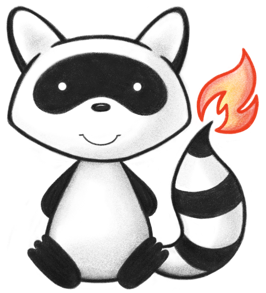
Package org.hl7.fhir.r5.context
Interface IWorkerContext
- All Known Implementing Classes:
BaseWorkerContext
,HapiWorkerContext
,SimpleWorkerContext
public interface IWorkerContext
This is the standard interface used for access to underlying FHIR
services through the tools and utilities provided by the reference
implementation.
The functionality it provides is
- get access to canonical resources,terminology services, and validator
(you can't create a validator directly because it needs access
to the right context for their information)
- find resources that the tools need to carry out their tasks
- provide access to terminology services they need.
(typically, these terminology service requests are just
passed through to the local implementation's terminology
service)
- Author:
- Grahame
-
Nested Class Summary
Nested ClassesModifier and TypeInterfaceDescriptionstatic class
static interface
static interface
static class
-
Method Summary
Modifier and TypeMethodDescriptionvoid
cachePackage
(PackageInformation packageInfo) Inform the cache about package dependencies.void
cacheResource
(Resource res) cache a resource for later retrieval using fetchResource.void
cacheResourceFromPackage
(Resource res, PackageInformation packageInfo) cache a resource for later retrieval using fetchResource.org.hl7.fhir.utilities.TimeTracker
clock()
expandVS
(Resource src, ElementDefinition.ElementDefinitionBindingComponent binding, boolean cacheOk, boolean heiarchical) ValueSet Expansion - see $expand, but resolves the binding firstexpandVS
(ValueSet.ConceptSetComponent inc, boolean hierarchical, boolean noInactive) Value set expanion inside the internal expansion engine - used for references to supported system (see "supportsSystem") for which there is no value set.ValueSet Expansion - see $expandValueSet Expansion - see $expandfetchCodeSystem
(String system) Find the code system definition for the nominated system uri.fetchCodeSystem
(String system, String version) <T extends Resource>
TfetchResource
(Class<T> class_, String uri) Find an identified resource.<T extends Resource>
TfetchResource
(Class<T> class_, String uri, String version) <T extends Resource>
TfetchResource
(Class<T> class_, String uri, Resource sourceOfReference) has the same functionality as fetchResource, but passes in information about the source of the reference (this may affect resolution of version)fetchResourceById
(String type, String uri) Variation of fetchResource when you have a string type, and don't need the right class The URI can have one of 3 formats: - a full URL e.g.<T extends Resource>
TfetchResourceRaw
(Class<T> class_, String uri) fetchResourcesByType
(Class<T> class_) Fetch all the resources of a particular type.<T extends Resource>
TfetchResourceWithException
(Class<T> class_, String uri) <T extends Resource>
TfetchResourceWithException
(Class<T> class_, String uri, Resource sourceOfReference) fetchSupplementedCodeSystem
(String system) Like fetchCodeSystem, except that the context will find any CodeSysetm supplements and merge them into thefetchSupplementedCodeSystem
(String system, String version) fetchTypeDefinition
(String typeName) This is a short cut for fetchResource(StructureDefinition.class, ...) but it accepts a typename - that is, it resolves based on StructureDefinition.type or StructureDefinition.url.This finds all the structure definitions that have the given typeNameformatMessage
(String theMessage, Object... theMessageArguments) Access to the contexts internationalised error messagesformatMessagePlural
(Integer pluralNum, String theMessage, Object... theMessageArguments) byte[]
getBinaryForKey
(String binaryKey) Returns the binary for the keyReturns a set of keys that can be used to get binaries from this context.int
Set the expansion parameters passed through the terminology server when txServer calls are made Note that the Validation Options override these when they are specified on validateCodeget/set the locale used when creating messages todo: what's the difference?getPackage
(String id, String ver) getPackageForUrl
(String url) getProfiledElementBuilder
(PEBuilder.PEElementPropertiesPolicy elementProps, boolean fixedProps) org.fhir.ucum.UcumService
Get the UCUM service that provides access to units of measure reasoning services This service might not be availableGet the version of the definitions loaded in context This *does not* have to be 5.0 (R5) - the context can load other versionsboolean
hasBinaryKey
(String binaryKey) Returns true if this worker context contains a binary for this key.boolean
hasPackage
(String id, String ver) boolean
hasPackage
(PackageInformation pack) <T extends Resource>
booleanhasResource
(Class<T> class_, String uri) find whether a resource is available.boolean
boolean
int
loadFromPackage
(org.hl7.fhir.utilities.npm.NpmPackage pi, IWorkerContext.IContextResourceLoader loader) Load relevant resources of the appropriate types (as specified by the loader) from the nominated package note that the package system uses lazy loading; the loader will be called later when the classes that use the context need the relevant resourceint
loadFromPackage
(org.hl7.fhir.utilities.npm.NpmPackage pi, IWorkerContext.IContextResourceLoader loader, List<String> types) Deprecated.int
loadFromPackageAndDependencies
(org.hl7.fhir.utilities.npm.NpmPackage pi, IWorkerContext.IContextResourceLoader loader, org.hl7.fhir.utilities.npm.BasePackageCacheManager pcm) Load relevant resources of the appropriate types (as specified by the loader) from the nominated package note that the package system uses lazy loading; the loader will be called later when the classes that use the context need the relevant resource This method also loads all the packages that the package depends on (recursively)Get a validator that can check whether a resource is validsetClientRetryCount
(int value) void
setExpansionProfile
(Parameters expParameters) Get the expansion parameters passed through the terminology server when txServer calls are made Note that the Validation Options override these when they are specified on validateCodevoid
setForPublication
(boolean value) void
void
setPackageTracker
(IWorkerContextManager.IPackageLoadingTracker packageTracker) void
setUcumService
(org.fhir.ucum.UcumService ucumService) void
setValidationMessageLanguage
(Locale locale) boolean
supportsSystem
(String system) True if the underlying terminology service provider will do expansion and code validation for the terminology.org.hl7.fhir.utilities.TranslationServices
validateCode
(org.hl7.fhir.utilities.validation.ValidationOptions options, String system, String version, String code, String display) Validation of a code - consult the terminology infrstructure and/or service to see whether it is known.validateCode
(org.hl7.fhir.utilities.validation.ValidationOptions options, String system, String version, String code, String display, ValueSet vs) Validation of a code - consult the terminology infrstructure and/or service to see whether it is known.validateCode
(org.hl7.fhir.utilities.validation.ValidationOptions options, String code, ValueSet vs) Validation of a code - consult the terminology infrstructure and/or service to see whether it is known.validateCode
(org.hl7.fhir.utilities.validation.ValidationOptions options, CodeableConcept code, ValueSet vs) Validation of a code - consult the terminology infrstructure and/or service to see whether it is known.validateCode
(org.hl7.fhir.utilities.validation.ValidationOptions options, Coding code, ValueSet vs) Validation of a code - consult the terminology infrstructure and/or service to see whether it is known.validateCode
(org.hl7.fhir.utilities.validation.ValidationOptions options, Coding code, ValueSet vs, ValidationContextCarrier ctxt) See comments in ValidationContextCarrier.void
validateCodeBatch
(org.hl7.fhir.utilities.validation.ValidationOptions options, List<? extends IWorkerContext.CodingValidationRequest> codes, ValueSet vs) Batch validate code - reduce latency and do a bunch of codes in a single server call.void
validateCodeBatchByRef
(org.hl7.fhir.utilities.validation.ValidationOptions options, List<? extends IWorkerContext.CodingValidationRequest> codes, String vsUrl)
-
Method Details
-
getVersion
Get the version of the definitions loaded in context This *does not* have to be 5.0 (R5) - the context can load other versions- Returns:
-
getUcumService
org.fhir.ucum.UcumService getUcumService()Get the UCUM service that provides access to units of measure reasoning services This service might not be available- Returns:
-
setUcumService
-
newValidator
Get a validator that can check whether a resource is valid- Returns:
- a prepared generator
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
fetchResource
Find an identified resource. The most common use of this is to access the the standard conformance resources that are part of the standard - structure definitions, value sets, concept maps, etc. Also, the narrative generator uses this, and may access any kind of resource The URI is called speculatively for things that might exist, so not finding a matching resource, return null, not an error The URI can have one of 3 formats: - a full URL e.g. http://acme.org/fhir/ValueSet/[id] - a relative URL e.g. ValueSet/[id] - a logical id e.g. [id] It's an error if the second form doesn't agree with class_. It's an error if class_ is null for the last form class can be Resource, DomainResource or CanonicalResource, which means resource of all kinds- Parameters:
resource
-Reference
-- Returns:
- Throws:
org.hl7.fhir.exceptions.FHIRException
Exception
-
fetchResourceRaw
-
fetchResourceWithException
<T extends Resource> T fetchResourceWithException(Class<T> class_, String uri) throws org.hl7.fhir.exceptions.FHIRException - Throws:
org.hl7.fhir.exceptions.FHIRException
-
fetchResourceWithException
<T extends Resource> T fetchResourceWithException(Class<T> class_, String uri, Resource sourceOfReference) throws org.hl7.fhir.exceptions.FHIRException - Throws:
org.hl7.fhir.exceptions.FHIRException
-
fetchResource
-
fetchResource
has the same functionality as fetchResource, but passes in information about the source of the reference (this may affect resolution of version)- Type Parameters:
T
-- Parameters:
class_
-uri
-canonicalForSource
-- Returns:
-
fetchResourcesByType
Fetch all the resources of a particular type. if class == (null | Resource | DomainResource | CanonicalResource) return everything- Type Parameters:
T
-- Parameters:
class_
-uri
-canonicalForSource
-- Returns:
-
fetchResourceById
Variation of fetchResource when you have a string type, and don't need the right class The URI can have one of 3 formats: - a full URL e.g. http://acme.org/fhir/ValueSet/[id] - a relative URL e.g. ValueSet/[id] - a logical id e.g. [id] if type == null, the URI can't be a simple logical id- Parameters:
type
-uri
-- Returns:
-
hasResource
find whether a resource is available. Implementations of the interface can assume that if hasResource ruturns true, the resource will usually be fetched subsequently- Parameters:
class_
-uri
-- Returns:
-
cacheResource
cache a resource for later retrieval using fetchResource. Note that various context implementations will have their own ways of loading rseources, and not all need implement cacheResource. If the resource is loaded out of a package, call cacheResourceFromPackage instead- Parameters:
res
-- Throws:
org.hl7.fhir.exceptions.FHIRException
-
cacheResourceFromPackage
void cacheResourceFromPackage(Resource res, PackageInformation packageInfo) throws org.hl7.fhir.exceptions.FHIRException cache a resource for later retrieval using fetchResource. The package information is used to help manage the cache internally, and to help with reference resolution. Packages should be define using cachePackage (but don't have to be) Note that various context implementations will have their own ways of loading rseources, and not all need implement cacheResource- Parameters:
res
-- Throws:
org.hl7.fhir.exceptions.FHIRException
-
cachePackage
Inform the cache about package dependencies. This can be used to help resolve references Note that the cache doesn't load dependencies- Parameters:
packageInfo
-
-
getResourceNames
- Returns:
- a list of the resource names defined for this version
-
getResourceNamesAsSet
- Returns:
- a set of the resource names defined for this version
-
getExpansionParameters
Set the expansion parameters passed through the terminology server when txServer calls are made Note that the Validation Options override these when they are specified on validateCode -
setExpansionProfile
Get the expansion parameters passed through the terminology server when txServer calls are made Note that the Validation Options override these when they are specified on validateCode -
fetchCodeSystem
Find the code system definition for the nominated system uri. return null if there isn't one (then the tool might try supportsSystem) This is a short cut for fetchResource(CodeSystem.class...)- Parameters:
system
-- Returns:
-
fetchCodeSystem
-
fetchSupplementedCodeSystem
Like fetchCodeSystem, except that the context will find any CodeSysetm supplements and merge them into the- Parameters:
system
-- Returns:
-
fetchSupplementedCodeSystem
-
supportsSystem
True if the underlying terminology service provider will do expansion and code validation for the terminology. Corresponds to the extension http://hl7.org/fhir/StructureDefinition/capabilitystatement-supported-system in the Conformance resource Not that not all supported code systems have an available CodeSystem resource- Parameters:
system
-- Returns:
- Throws:
Exception
org.hl7.fhir.exceptions.TerminologyServiceException
-
expandVS
ValueSet Expansion - see $expand- Parameters:
source
-- Returns:
-
expandVS
ValueSetExpansionOutcome expandVS(ValueSet source, boolean cacheOk, boolean heiarchical, boolean incompleteOk) ValueSet Expansion - see $expand- Parameters:
source
-- Returns:
-
expandVS
ValueSetExpansionOutcome expandVS(Resource src, ElementDefinition.ElementDefinitionBindingComponent binding, boolean cacheOk, boolean heiarchical) throws org.hl7.fhir.exceptions.FHIRException ValueSet Expansion - see $expand, but resolves the binding first- Parameters:
source
-- Returns:
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
expandVS
ValueSetExpansionOutcome expandVS(ValueSet.ConceptSetComponent inc, boolean hierarchical, boolean noInactive) throws org.hl7.fhir.exceptions.TerminologyServiceException Value set expanion inside the internal expansion engine - used for references to supported system (see "supportsSystem") for which there is no value set.- Parameters:
inc
-- Returns:
- Throws:
org.hl7.fhir.exceptions.FHIRException
org.hl7.fhir.exceptions.TerminologyServiceException
-
getLocale
get/set the locale used when creating messages todo: what's the difference?- Returns:
-
setLocale
-
setValidationMessageLanguage
-
formatMessage
Access to the contexts internationalised error messages- Parameters:
theMessage
-theMessageArguments
-- Returns:
-
formatMessagePlural
-
validateCode
IWorkerContext.ValidationResult validateCode(org.hl7.fhir.utilities.validation.ValidationOptions options, String code, ValueSet vs) Validation of a code - consult the terminology infrstructure and/or service to see whether it is known. If known, return a description of it note: always return a result, with either an error or a code description corresponds to 2 terminology service calls: $validate-code and $lookup in this case, the system will be inferred from the value set. It's an error to call this one without the value set- Parameters:
options
- - validation options (required)code
- he code to validate (required)vs
- the applicable valueset (required)- Returns:
-
validateCode
IWorkerContext.ValidationResult validateCode(org.hl7.fhir.utilities.validation.ValidationOptions options, String system, String version, String code, String display) Validation of a code - consult the terminology infrstructure and/or service to see whether it is known. If known, return a description of it note: always return a result, with either an error or a code description corresponds to 2 terminology service calls: $validate-code and $lookup- Parameters:
options
- - validation options (required)system
- - equals Coding.system (required)code
- - equals Coding.code (required)display
- - equals Coding.display (optional)- Returns:
-
validateCode
IWorkerContext.ValidationResult validateCode(org.hl7.fhir.utilities.validation.ValidationOptions options, String system, String version, String code, String display, ValueSet vs) Validation of a code - consult the terminology infrstructure and/or service to see whether it is known. If known, return a description of it note: always return a result, with either an error or a code description corresponds to 2 terminology service calls: $validate-code and $lookup- Parameters:
options
- - validation options (required)system
- - equals Coding.system (required)code
- - equals Coding.code (required)display
- - equals Coding.display (optional)vs
- the applicable valueset (optional)- Returns:
-
validateCode
IWorkerContext.ValidationResult validateCode(org.hl7.fhir.utilities.validation.ValidationOptions options, CodeableConcept code, ValueSet vs) Validation of a code - consult the terminology infrstructure and/or service to see whether it is known. If known, return a description of it note: always return a result, with either an error or a code description corresponds to 2 terminology service calls: $validate-code and $lookup Note that this doesn't validate binding strength (e.g. is just text allowed?)- Parameters:
options
- - validation options (required)code
- - CodeableConcept to validatevs
- the applicable valueset (optional)- Returns:
-
validateCode
IWorkerContext.ValidationResult validateCode(org.hl7.fhir.utilities.validation.ValidationOptions options, Coding code, ValueSet vs) Validation of a code - consult the terminology infrstructure and/or service to see whether it is known. If known, return a description of it note: always return a result, with either an error or a code description corresponds to 2 terminology service calls: $validate-code and $lookup in this case, the system will be inferred from the value set. It's an error to call this one without the value set- Parameters:
options
- - validation options (required)code
- - Coding to validatevs
- the applicable valueset (optional)- Returns:
-
validateCode
IWorkerContext.ValidationResult validateCode(org.hl7.fhir.utilities.validation.ValidationOptions options, Coding code, ValueSet vs, ValidationContextCarrier ctxt) See comments in ValidationContextCarrier. This is called when there might be additional value sets etc available in the context, but we don't want to pre-process them.- Parameters:
options
-code
-vs
-ctxt
-- Returns:
-
validateCodeBatch
void validateCodeBatch(org.hl7.fhir.utilities.validation.ValidationOptions options, List<? extends IWorkerContext.CodingValidationRequest> codes, ValueSet vs) Batch validate code - reduce latency and do a bunch of codes in a single server call. Each is the same as a validateCode- Parameters:
options
-codes
-vs
-
-
validateCodeBatchByRef
void validateCodeBatchByRef(org.hl7.fhir.utilities.validation.ValidationOptions options, List<? extends IWorkerContext.CodingValidationRequest> codes, String vsUrl) -
getNSUrlMap
-
translator
org.hl7.fhir.utilities.TranslationServices translator() -
setLogger
-
getLogger
-
isNoTerminologyServer
boolean isNoTerminologyServer() -
getCodeSystemsUsed
-
getClientRetryCount
int getClientRetryCount() -
setClientRetryCount
-
clock
org.hl7.fhir.utilities.TimeTracker clock() -
fetchTypeDefinition
This is a short cut for fetchResource(StructureDefinition.class, ...) but it accepts a typename - that is, it resolves based on StructureDefinition.type or StructureDefinition.url. This only resolves to http://hl7.org/fhir/StructureDefinition/{typename}- Parameters:
typeName
-- Returns:
-
fetchTypeDefinitions
This finds all the structure definitions that have the given typeName- Parameters:
typeName
-- Returns:
-
getBinaryKeysAsSet
Returns a set of keys that can be used to get binaries from this context. The binaries come from the loaded packages (mostly the pubpack)- Returns:
- a set of binaries or null
-
hasBinaryKey
Returns true if this worker context contains a binary for this key.- Parameters:
binaryKey
-- Returns:
- true if binary is available for this key
-
getBinaryForKey
Returns the binary for the key- Parameters:
binaryKey
-- Returns:
-
loadFromPackage
int loadFromPackage(org.hl7.fhir.utilities.npm.NpmPackage pi, IWorkerContext.IContextResourceLoader loader) throws FileNotFoundException, IOException, org.hl7.fhir.exceptions.FHIRException Load relevant resources of the appropriate types (as specified by the loader) from the nominated package note that the package system uses lazy loading; the loader will be called later when the classes that use the context need the relevant resource- Parameters:
pi
- - the package to loadloader
- - an implemenation of IContextResourceLoader that knows how to read the resources in the package (e.g. for the appropriate version).- Returns:
- the number of resources loaded
- Throws:
FileNotFoundException
IOException
org.hl7.fhir.exceptions.FHIRException
-
loadFromPackage
@Deprecated int loadFromPackage(org.hl7.fhir.utilities.npm.NpmPackage pi, IWorkerContext.IContextResourceLoader loader, List<String> types) throws FileNotFoundException, IOException, org.hl7.fhir.exceptions.FHIRException Deprecated.Load relevant resources of the appropriate types (as specified by the loader) from the nominated package note that the package system uses lazy loading; the loader will be called later when the classes that use the context need the relevant resource Deprecated - use the simpler method where the types come from the loader.- Parameters:
pi
- - the package to loadloader
- - an implemenation of IContextResourceLoader that knows how to read the resources in the package (e.g. for the appropriate version).types
- - which types of resources to load- Returns:
- the number of resources loaded
- Throws:
FileNotFoundException
IOException
org.hl7.fhir.exceptions.FHIRException
-
loadFromPackageAndDependencies
int loadFromPackageAndDependencies(org.hl7.fhir.utilities.npm.NpmPackage pi, IWorkerContext.IContextResourceLoader loader, org.hl7.fhir.utilities.npm.BasePackageCacheManager pcm) throws FileNotFoundException, IOException, org.hl7.fhir.exceptions.FHIRException Load relevant resources of the appropriate types (as specified by the loader) from the nominated package note that the package system uses lazy loading; the loader will be called later when the classes that use the context need the relevant resource This method also loads all the packages that the package depends on (recursively)- Parameters:
pi
- - the package to loadloader
- - an implemenation of IContextResourceLoader that knows how to read the resources in the package (e.g. for the appropriate version).pcm
- - used to find and load additional dependencies- Returns:
- the number of resources loaded
- Throws:
FileNotFoundException
IOException
org.hl7.fhir.exceptions.FHIRException
-
hasPackage
-
hasPackage
-
getPackage
-
getPackageForUrl
-
getPackageTracker
-
setPackageTracker
-
getSpecUrl
-
getProfiledElementBuilder
PEBuilder getProfiledElementBuilder(PEBuilder.PEElementPropertiesPolicy elementProps, boolean fixedProps) -
isForPublication
boolean isForPublication() -
setForPublication
-