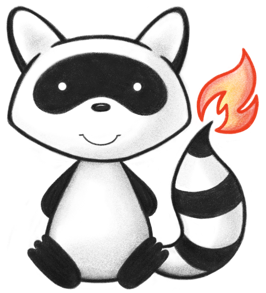
Class Bundle
- All Implemented Interfaces:
ca.uhn.fhir.model.api.IElement
,Serializable
,org.hl7.fhir.instance.model.api.IAnyResource
,org.hl7.fhir.instance.model.api.IBase
,org.hl7.fhir.instance.model.api.IBaseBundle
,org.hl7.fhir.instance.model.api.IBaseResource
- See Also:
-
Nested Class Summary
Nested ClassesModifier and TypeClassDescriptionstatic class
static class
static class
static class
static class
static enum
static class
static enum
static class
static enum
static class
-
Field Summary
FieldsModifier and TypeFieldDescriptionstatic final ca.uhn.fhir.rest.gclient.ReferenceClientParam
Fluent Client search parameter constant for compositionprotected List
<Bundle.BundleEntryComponent> An entry in a bundle resource - will either contain a resource or information about a resource (transactions and history only).protected Identifier
A persistent identifier for the bundle that won't change as a bundle is copied from server to server.static final ca.uhn.fhir.rest.gclient.TokenClientParam
Fluent Client search parameter constant for identifierstatic final ca.uhn.fhir.model.api.Include
Constant for fluent queries to be used to add include statements.static final ca.uhn.fhir.model.api.Include
Constant for fluent queries to be used to add include statements.protected List
<Bundle.BundleLinkComponent> A series of links that provide context to this bundle.static final ca.uhn.fhir.rest.gclient.ReferenceClientParam
Fluent Client search parameter constant for messageprotected Signature
Digital Signature - base64 encoded.static final String
Search parameter: compositionstatic final String
Search parameter: identifierstatic final String
Search parameter: messagestatic final String
Search parameter: timestampstatic final String
Search parameter: typeprotected InstantType
The date/time that the bundle was assembled - i.e.static final ca.uhn.fhir.rest.gclient.DateClientParam
Fluent Client search parameter constant for timestampprotected UnsignedIntType
If a set of search matches, this is the total number of entries of type 'match' across all pages in the search.protected Enumeration
<Bundle.BundleType> Indicates the purpose of this bundle - how it is intended to be used.static final ca.uhn.fhir.rest.gclient.TokenClientParam
Fluent Client search parameter constant for typeFields inherited from class org.hl7.fhir.r4.model.Resource
id, implicitRules, language, meta
Fields inherited from interface org.hl7.fhir.instance.model.api.IAnyResource
RES_ID, SP_RES_ID
Fields inherited from interface org.hl7.fhir.instance.model.api.IBaseBundle
LINK_NEXT, LINK_PREV, LINK_SELF
Fields inherited from interface org.hl7.fhir.instance.model.api.IBaseResource
INCLUDE_ALL, WILDCARD_ALL_SET
-
Constructor Summary
ConstructorsConstructorDescriptionBundle()
ConstructorBundle
(Enumeration<Bundle.BundleType> type) Constructor -
Method Summary
Modifier and TypeMethodDescriptionaddEntry()
addLink()
copy()
void
copyValues
(Bundle dst) boolean
equalsDeep
(Base other_) boolean
equalsShallow
(Base other_) fhirType()
getEntry()
getLink()
getLinkOrCreate
(String theRelation) getNamedProperty
(int _hash, String _name, boolean _checkValid) Base[]
getProperty
(int hash, String name, boolean checkValid) int
getTotal()
getType()
String[]
getTypesForProperty
(int hash, String name) boolean
hasEntry()
boolean
boolean
hasLink()
boolean
boolean
boolean
boolean
hasTotal()
boolean
boolean
hasType()
boolean
boolean
isEmpty()
protected void
listChildren
(List<Property> children) makeProperty
(int hash, String name) setEntry
(List<Bundle.BundleEntryComponent> theEntry) setIdentifier
(Identifier value) setLink
(List<Bundle.BundleLinkComponent> theLink) setProperty
(int hash, String name, Base value) setProperty
(String name, Base value) setSignature
(Signature value) setTimestamp
(Date value) setTimestampElement
(InstantType value) setTotal
(int value) setTotalElement
(UnsignedIntType value) setType
(Bundle.BundleType value) protected Bundle
Methods inherited from class org.hl7.fhir.r4.model.Resource
copyValues, getId, getIdBase, getIdElement, getIdPart, getImplicitRules, getImplicitRulesElement, getLanguage, getLanguage, getLanguageElement, getMeta, hasId, hasIdElement, hasImplicitRules, hasImplicitRulesElement, hasLanguage, hasLanguageElement, hasMeta, setId, setIdBase, setIdElement, setImplicitRules, setImplicitRulesElement, setLanguage, setLanguageElement, setMeta
Methods inherited from class org.hl7.fhir.r4.model.BaseResource
getStructureFhirVersionEnum, isResource, setId
Methods inherited from class org.hl7.fhir.r4.model.Base
castToAddress, castToAnnotation, castToAttachment, castToBase64Binary, castToBoolean, castToCanonical, castToCode, castToCodeableConcept, castToCoding, castToContactDetail, castToContactPoint, castToContributor, castToDataRequirement, castToDate, castToDateTime, castToDecimal, castToDosage, castToDuration, castToElementDefinition, castToExpression, castToExtension, castToHumanName, castToId, castToIdentifier, castToInstant, castToInteger, castToMarkdown, castToMarketingStatus, castToMeta, castToMoney, castToNarrative, castToOid, castToParameterDefinition, castToPeriod, castToPopulation, castToPositiveInt, castToProdCharacteristic, castToProductShelfLife, castToQuantity, castToRange, castToRatio, castToReference, castToRelatedArtifact, castToResource, castToSampledData, castToSignature, castToSimpleQuantity, castToString, castToSubstanceAmount, castToTime, castToTiming, castToTriggerDefinition, castToType, castToUnsignedInt, castToUri, castToUrl, castToUsageContext, castToXhtml, castToXhtmlString, children, clearUserData, compareDeep, compareDeep, compareDeep, compareDeep, compareValues, compareValues, copyValues, dateTimeValue, equals, getChildByName, getFormatCommentsPost, getFormatCommentsPre, getNamedProperty, getUserData, getUserInt, getUserString, getXhtml, hasFormatComment, hasPrimitiveValue, hasType, hasUserData, isBooleanPrimitive, isDateTime, isMetadataBased, isPrimitive, listChildrenByName, listChildrenByName, primitiveValue, setUserData, setUserDataINN
Methods inherited from class java.lang.Object
clone, equals, finalize, getClass, hashCode, notify, notifyAll, toString, wait, wait, wait
Methods inherited from interface org.hl7.fhir.instance.model.api.IAnyResource
getUserData, setUserData
Methods inherited from interface org.hl7.fhir.instance.model.api.IBase
getFormatCommentsPost, getFormatCommentsPre, hasFormatComment
Methods inherited from interface org.hl7.fhir.instance.model.api.IBaseResource
getMeta, getStructureFhirVersionEnum, isDeleted, setId
-
Field Details
-
identifier
A persistent identifier for the bundle that won't change as a bundle is copied from server to server. -
type
Indicates the purpose of this bundle - how it is intended to be used. -
timestamp
The date/time that the bundle was assembled - i.e. when the resources were placed in the bundle. -
total
If a set of search matches, this is the total number of entries of type 'match' across all pages in the search. It does not include search.mode = 'include' or 'outcome' entries and it does not provide a count of the number of entries in the Bundle. -
link
A series of links that provide context to this bundle. -
entry
An entry in a bundle resource - will either contain a resource or information about a resource (transactions and history only). -
signature
Digital Signature - base64 encoded. XML-DSig or a JWT. -
SP_IDENTIFIER
Search parameter: identifierDescription: Persistent identifier for the bundle
Type: token
Path: Bundle.identifier
- See Also:
-
IDENTIFIER
Fluent Client search parameter constant for identifierDescription: Persistent identifier for the bundle
Type: token
Path: Bundle.identifier
-
SP_COMPOSITION
Search parameter: compositionDescription: The first resource in the bundle, if the bundle type is "document" - this is a composition, and this parameter provides access to search its contents
Type: reference
Path: Bundle.entry(0).resource
- See Also:
-
COMPOSITION
Fluent Client search parameter constant for compositionDescription: The first resource in the bundle, if the bundle type is "document" - this is a composition, and this parameter provides access to search its contents
Type: reference
Path: Bundle.entry(0).resource
-
INCLUDE_COMPOSITION
Constant for fluent queries to be used to add include statements. Specifies the path value of "Bundle:composition". -
SP_TYPE
Search parameter: typeDescription: document | message | transaction | transaction-response | batch | batch-response | history | searchset | collection
Type: token
Path: Bundle.type
- See Also:
-
TYPE
Fluent Client search parameter constant for typeDescription: document | message | transaction | transaction-response | batch | batch-response | history | searchset | collection
Type: token
Path: Bundle.type
-
SP_MESSAGE
Search parameter: messageDescription: The first resource in the bundle, if the bundle type is "message" - this is a message header, and this parameter provides access to search its contents
Type: reference
Path: Bundle.entry(0).resource
- See Also:
-
MESSAGE
Fluent Client search parameter constant for messageDescription: The first resource in the bundle, if the bundle type is "message" - this is a message header, and this parameter provides access to search its contents
Type: reference
Path: Bundle.entry(0).resource
-
INCLUDE_MESSAGE
Constant for fluent queries to be used to add include statements. Specifies the path value of "Bundle:message". -
SP_TIMESTAMP
Search parameter: timestampDescription: When the bundle was assembled
Type: date
Path: Bundle.timestamp
- See Also:
-
TIMESTAMP
Fluent Client search parameter constant for timestampDescription: When the bundle was assembled
Type: date
Path: Bundle.timestamp
-
-
Constructor Details
-
Bundle
public Bundle()Constructor -
Bundle
Constructor
-
-
Method Details
-
getIdentifier
- Returns:
identifier
(A persistent identifier for the bundle that won't change as a bundle is copied from server to server.)
-
hasIdentifier
-
setIdentifier
- Parameters:
value
-identifier
(A persistent identifier for the bundle that won't change as a bundle is copied from server to server.)
-
getTypeElement
- Returns:
type
(Indicates the purpose of this bundle - how it is intended to be used.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value
-
hasTypeElement
-
hasType
-
setTypeElement
- Parameters:
value
-type
(Indicates the purpose of this bundle - how it is intended to be used.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value
-
getType
- Returns:
- Indicates the purpose of this bundle - how it is intended to be used.
-
setType
- Parameters:
value
- Indicates the purpose of this bundle - how it is intended to be used.
-
getTimestampElement
- Returns:
timestamp
(The date/time that the bundle was assembled - i.e. when the resources were placed in the bundle.). This is the underlying object with id, value and extensions. The accessor "getTimestamp" gives direct access to the value
-
hasTimestampElement
-
hasTimestamp
-
setTimestampElement
- Parameters:
value
-timestamp
(The date/time that the bundle was assembled - i.e. when the resources were placed in the bundle.). This is the underlying object with id, value and extensions. The accessor "getTimestamp" gives direct access to the value
-
getTimestamp
- Returns:
- The date/time that the bundle was assembled - i.e. when the resources were placed in the bundle.
-
setTimestamp
- Parameters:
value
- The date/time that the bundle was assembled - i.e. when the resources were placed in the bundle.
-
getTotalElement
- Returns:
total
(If a set of search matches, this is the total number of entries of type 'match' across all pages in the search. It does not include search.mode = 'include' or 'outcome' entries and it does not provide a count of the number of entries in the Bundle.). This is the underlying object with id, value and extensions. The accessor "getTotal" gives direct access to the value
-
hasTotalElement
-
hasTotal
-
setTotalElement
- Parameters:
value
-total
(If a set of search matches, this is the total number of entries of type 'match' across all pages in the search. It does not include search.mode = 'include' or 'outcome' entries and it does not provide a count of the number of entries in the Bundle.). This is the underlying object with id, value and extensions. The accessor "getTotal" gives direct access to the value
-
getTotal
- Returns:
- If a set of search matches, this is the total number of entries of type 'match' across all pages in the search. It does not include search.mode = 'include' or 'outcome' entries and it does not provide a count of the number of entries in the Bundle.
-
setTotal
- Parameters:
value
- If a set of search matches, this is the total number of entries of type 'match' across all pages in the search. It does not include search.mode = 'include' or 'outcome' entries and it does not provide a count of the number of entries in the Bundle.
-
getLink
- Returns:
link
(A series of links that provide context to this bundle.)
-
setLink
- Returns:
- Returns a reference to
this
for easy method chaining
-
hasLink
-
addLink
-
addLink
-
getLinkFirstRep
- Returns:
- The first repetition of repeating field
link
, creating it if it does not already exist
-
getEntry
- Returns:
entry
(An entry in a bundle resource - will either contain a resource or information about a resource (transactions and history only).)
-
setEntry
- Returns:
- Returns a reference to
this
for easy method chaining
-
hasEntry
-
addEntry
-
addEntry
-
getEntryFirstRep
- Returns:
- The first repetition of repeating field
entry
, creating it if it does not already exist
-
getSignature
- Returns:
signature
(Digital Signature - base64 encoded. XML-DSig or a JWT.)
-
hasSignature
-
setSignature
- Parameters:
value
-signature
(Digital Signature - base64 encoded. XML-DSig or a JWT.)
-
getLink
Returns thelink
which matches a givenrelation
. If no link is found which matches the given relation, returnsnull
. If more than one link is found which matches the given relation, returns the first matching BundleLinkComponent.- Parameters:
theRelation
- The relation, such as "next", or "self. See the constants such asIBaseBundle.LINK_SELF
andIBaseBundle.LINK_NEXT
.- Returns:
- Returns a matching BundleLinkComponent, or
null
- See Also:
-
getLinkOrCreate
Returns thelink
which matches a givenrelation
. If no link is found which matches the given relation, creates a new BundleLinkComponent with the given relation and adds it to this Bundle. If more than one link is found which matches the given relation, returns the first matching BundleLinkComponent.- Parameters:
theRelation
- The relation, such as "next", or "self. See the constants such asIBaseBundle.LINK_SELF
andIBaseBundle.LINK_NEXT
.- Returns:
- Returns a matching BundleLinkComponent, or
null
- See Also:
-
listChildren
- Overrides:
listChildren
in classResource
-
getNamedProperty
public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws org.hl7.fhir.exceptions.FHIRException - Overrides:
getNamedProperty
in classResource
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
getProperty
public Base[] getProperty(int hash, String name, boolean checkValid) throws org.hl7.fhir.exceptions.FHIRException - Overrides:
getProperty
in classResource
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
setProperty
public Base setProperty(int hash, String name, Base value) throws org.hl7.fhir.exceptions.FHIRException - Overrides:
setProperty
in classResource
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
setProperty
- Overrides:
setProperty
in classResource
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
makeProperty
- Overrides:
makeProperty
in classResource
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
getTypesForProperty
public String[] getTypesForProperty(int hash, String name) throws org.hl7.fhir.exceptions.FHIRException - Overrides:
getTypesForProperty
in classResource
- Throws:
org.hl7.fhir.exceptions.FHIRException
-
addChild
-
fhirType
-
copy
-
copyValues
-
typedCopy
-
equalsDeep
- Overrides:
equalsDeep
in classResource
-
equalsShallow
- Overrides:
equalsShallow
in classResource
-
isEmpty
-
getResourceType
- Specified by:
getResourceType
in classResource
-