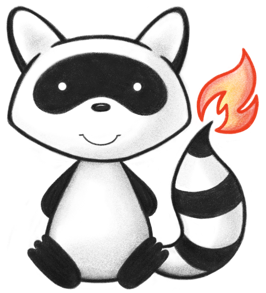
Interface IParser
- All Known Subinterfaces:
IJsonLikeParser
- All Known Implementing Classes:
BaseParser
,JsonParser
,NDJsonParser
,RDFParser
,XmlParser
Thread safety: Parsers are not guaranteed to be thread safe. Create a new parser instance for every thread or every message being parsed/encoded.
-
Method Summary
Modifier and TypeMethodDescriptionencodeResourceToString
(IBaseResource theResource) Encodes a resource using the parser's given encoding format.void
encodeResourceToWriter
(IBaseResource theResource, Writer theWriter) Encodes a resource using the parser's given encoding format.encodeToString
(IBase theElement) Encodes any FHIR element to a string.void
encodeToWriter
(IBase theElement, Writer theWriter) Encodes any FHIR element to a writer.Returns the value supplied tosetDontStripVersionsFromReferencesAtPaths(String...)
ornull
if no value has been set for this parser (in which case the default from theParserOptions
will be used}If not set to null (as is the default) this ID will be used as the ID in any resources encoded by this parserWhich encoding does this parser instance produce?List
<Class<? extends IBaseResource>> Gets the preferred types, as set usingsetPreferTypes(List)
If set totrue
(which is the default), resource references containing a version will have the version removed when the resource is encoded.
boolean
If set totrue
(default is false), the values supplied tosetEncodeElements(Set)
will not be applied to the root resource (typically a Bundle), but will be applied to any sub-resources contained within it (i.e.boolean
Returns true if resource IDs should be omittedboolean
Is the parser in "summary mode"? SeesetSummaryMode(boolean)
for informationparseResource
(InputStream theInputStream) Parses a resourceparseResource
(Reader theReader) Parses a resource<T extends IBaseResource>
TparseResource
(Class<T> theResourceType, InputStream theInputStream) Parses a resource<T extends IBaseResource>
TparseResource
(Class<T> theResourceType, Reader theReader) Parses a resource<T extends IBaseResource>
TparseResource
(Class<T> theResourceType, String theString) Parses a resourceparseResource
(String theMessageString) Parses a resourcesetDontEncodeElements
(Collection<String> theDontEncodeElements) If provided, specifies the elements which should NOT be encoded.setDontStripVersionsFromReferencesAtPaths
(String... thePaths) If supplied value(s), any resource references at the specified paths will have their resource versions encoded instead of being automatically stripped during the encoding process.If supplied value(s), any resource references at the specified paths will have their resource versions encoded instead of being automatically stripped during the encoding process.setEncodeElements
(Set<String> theEncodeElements) If provided, specifies the elements which should be encoded, to the exclusion of all others.void
setEncodeElementsAppliesToChildResourcesOnly
(boolean theEncodeElementsAppliesToChildResourcesOnly) If set totrue
(default is false), the values supplied tosetEncodeElements(Set)
will not be applied to the root resource (typically a Bundle), but will be applied to any sub-resources contained within it (i.e.setEncodeForceResourceId
(IIdType theForceResourceId) When encoding, force this resource ID to be encoded as the resource IDsetOmitResourceId
(boolean theOmitResourceId) If set totrue
(default isfalse
) the ID of any resources being encoded will not be included in the output.setOverrideResourceIdWithBundleEntryFullUrl
(Boolean theOverrideResourceIdWithBundleEntryFullUrl) If set totrue
(which is the default), the Bundle.entry.fullUrl will override the Bundle.entry.resource's resource id if the fullUrl is defined.setParserErrorHandler
(IParserErrorHandler theErrorHandler) Registers an error handler which will be invoked when any parse errors are foundvoid
setPreferTypes
(List<Class<? extends IBaseResource>> thePreferTypes) If set, when parsing resources the parser will try to use the given types when possible, in the order that they are provided (from highest to lowest priority).setPrettyPrint
(boolean thePrettyPrint) Sets the "pretty print" flag, meaning that the parser will encode resources with human-readable spacing and newlines between elements instead of condensing output as much as possible.setServerBaseUrl
(String theUrl) Sets the server's base URL used by this parser.setStripVersionsFromReferences
(Boolean theStripVersionsFromReferences) If set totrue
(which is the default), resource references containing a version will have the version removed when the resource is encoded.
setSummaryMode
(boolean theSummaryMode) If set totrue
(default isfalse
) only elements marked by the FHIR specification as being "summary elements" will be included.setSuppressNarratives
(boolean theSuppressNarratives) If set totrue
(default isfalse
), narratives will not be included in the encoded values.
-
Method Details
-
encodeResourceToString
Encodes a resource using the parser's given encoding format.- Parameters:
theResource
- The resource to encode. Must not be null.- Returns:
- A string representation of the encoding
- Throws:
DataFormatException
- If any invalid elements within the contents to be encoded prevent successful encoding.
-
encodeResourceToWriter
void encodeResourceToWriter(IBaseResource theResource, Writer theWriter) throws IOException, DataFormatException Encodes a resource using the parser's given encoding format.- Parameters:
theResource
- The resource to encode. Must not be null.theWriter
- The writer to write to.- Throws:
DataFormatException
- If any invalid elements within the contents to be encoded prevent successful encoding.IOException
-
encodeToString
Encodes any FHIR element to a string. If aresource object
is passed in, the resource will be encoded using standard FHIR encoding rules. If aprimitive datatype
is passed in, the string value of the primitive type is encoded. Any extensions on the primitive type are not encoded. If any other object is passed in, a fragment is encoded. The format of the fragment depends on the encoding:- JSON: The fragment is output as a simple JSON object, exactly as it would appear within an encoded resource.
- XML: The fragment is output as an XML element as it would appear within an encoded resource, however it is wrapped in an element called
<element>
in order to avoid producing a document with multiple root tags. - RDF/Turtle: This mode is not supported and will throw an
InternalErrorException
- Throws:
DataFormatException
- Since:
- 6.8.0
-
encodeToWriter
Encodes any FHIR element to a writer. If aresource object
is passed in, the resource will be encoded using standard FHIR encoding rules. If aprimitive datatype
is passed in, the string value of the primitive type is encoded. Any extensions on the primitive type are not encoded. If any other object is passed in, a fragment is encoded. The format of the fragment depends on the encoding:- JSON: The fragment is output as a simple JSON object, exactly as it would appear within an encoded resource.
- XML: The fragment is output as an XML element as it would appear within an encoded resource, however it is wrapped in an element called
<element>
in order to avoid producing a document with multiple root tags. - RDF/Turtle: This mode is not supported and will throw an
InternalErrorException
- Throws:
DataFormatException
IOException
- Since:
- 6.8.0
-
getEncodeForceResourceId
If not set to null (as is the default) this ID will be used as the ID in any resources encoded by this parser -
setEncodeForceResourceId
When encoding, force this resource ID to be encoded as the resource ID -
getEncoding
Which encoding does this parser instance produce? -
getPreferTypes
List<Class<? extends IBaseResource>> getPreferTypes()Gets the preferred types, as set usingsetPreferTypes(List)
- Returns:
- Returns the preferred types, or
null
- See Also:
-
setPreferTypes
If set, when parsing resources the parser will try to use the given types when possible, in the order that they are provided (from highest to lowest priority). For example, if a custom type which declares to implement the Patient resource is passed in here, and the parser is parsing a Bundle containing a Patient resource, the parser will use the given custom type.This feature is related to, but not the same as the
FhirContext.setDefaultTypeForProfile(String, Class)
feature.setDefaultTypeForProfile
is used to specify a type to be used when a resource explicitly declares support for a given profile. This feature specifies a type to be used irrespective of the profile declaration in the metadata statement.- Parameters:
thePreferTypes
- The preferred types, ornull
-
isOmitResourceId
boolean isOmitResourceId()Returns true if resource IDs should be omitted- Since:
- 1.1
- See Also:
-
setOmitResourceId
If set totrue
(default isfalse
) the ID of any resources being encoded will not be included in the output. Note that this does not apply to contained resources, only to root resources. In other words, if this is set totrue
, contained resources will still have local IDs but the outer/containing ID will not have an ID.If the resource being encoded is a Bundle or Parameters resource, this setting only applies to the outer resource being encoded, not any resources contained wihthin.
- Parameters:
theOmitResourceId
- Should resource IDs be omitted- Returns:
- Returns a reference to
this
parser so that method calls can be chained together - Since:
- 1.1
-
getStripVersionsFromReferences
If set totrue
(which is the default), resource references containing a version will have the version removed when the resource is encoded. This is generally good behaviour because in most situations, references from one resource to another should be to the resource by ID, not by ID and version. In some cases though, it may be desirable to preserve the version in resource links. In that case, this value should be set to
false
.- Returns:
- Returns the parser instance's configuration setting for stripping versions from resource references when
encoding. This method will retun
null
if no value is set, in which case the value from theParserOptions
will be used (default istrue
) - See Also:
-
setStripVersionsFromReferences
If set totrue
(which is the default), resource references containing a version will have the version removed when the resource is encoded. This is generally good behaviour because in most situations, references from one resource to another should be to the resource by ID, not by ID and version. In some cases though, it may be desirable to preserve the version in resource links. In that case, this value should be set to
false
.This method provides the ability to globally disable reference encoding. If finer-grained control is needed, use
setDontStripVersionsFromReferencesAtPaths(String...)
- Parameters:
theStripVersionsFromReferences
- Set this tofalse
to prevent the parser from removing resource versions from references (or
null
to apply the default setting from theParserOptions
- Returns:
- Returns a reference to
this
parser so that method calls can be chained together - See Also:
-
isSummaryMode
boolean isSummaryMode()Is the parser in "summary mode"? SeesetSummaryMode(boolean)
for information -
setSummaryMode
If set totrue
(default isfalse
) only elements marked by the FHIR specification as being "summary elements" will be included.- Returns:
- Returns a reference to
this
parser so that method calls can be chained together
-
parseResource
<T extends IBaseResource> T parseResource(Class<T> theResourceType, Reader theReader) throws DataFormatException Parses a resource- Parameters:
theResourceType
- The resource type to use. This can be used to explicitly specify a class which extends a built-in type (e.g. a custom type extending the default Patient class)theReader
- The reader to parse input from. Note that the Reader will not be closed by the parser upon completion.- Returns:
- A parsed resource
- Throws:
DataFormatException
- If the resource can not be parsed because the data is not recognized or invalid for any reason
-
parseResource
<T extends IBaseResource> T parseResource(Class<T> theResourceType, InputStream theInputStream) throws DataFormatException Parses a resource- Parameters:
theResourceType
- The resource type to use. This can be used to explicitly specify a class which extends a built-in type (e.g. a custom type extending the default Patient class)theInputStream
- The InputStream to parse input from, with an implied charset of UTF-8. Note that the InputStream will not be closed by the parser upon completion.- Returns:
- A parsed resource
- Throws:
DataFormatException
- If the resource can not be parsed because the data is not recognized or invalid for any reason
-
parseResource
<T extends IBaseResource> T parseResource(Class<T> theResourceType, String theString) throws DataFormatException Parses a resource- Parameters:
theResourceType
- The resource type to use. This can be used to explicitly specify a class which extends a built-in type (e.g. a custom type extending the default Patient class)theString
- The string to parse- Returns:
- A parsed resource
- Throws:
DataFormatException
- If the resource can not be parsed because the data is not recognized or invalid for any reason
-
parseResource
Parses a resource- Parameters:
theReader
- The reader to parse input from. Note that the Reader will not be closed by the parser upon completion.- Returns:
- A parsed resource. Note that the returned object will be an instance of
IResource
orIAnyResource
depending on the specific FhirContext which created this parser. - Throws:
DataFormatException
- If the resource can not be parsed because the data is not recognized or invalid for any reasonConfigurationException
-
parseResource
IBaseResource parseResource(InputStream theInputStream) throws ConfigurationException, DataFormatException Parses a resource- Parameters:
theInputStream
- The InputStream to parse input from (charset is assumed to be UTF-8). Note that the stream will not be closed by the parser upon completion.- Returns:
- A parsed resource. Note that the returned object will be an instance of
IResource
orIAnyResource
depending on the specific FhirContext which created this parser. - Throws:
DataFormatException
- If the resource can not be parsed because the data is not recognized or invalid for any reasonConfigurationException
-
parseResource
IBaseResource parseResource(String theMessageString) throws ConfigurationException, DataFormatException Parses a resource- Parameters:
theMessageString
- The string to parse- Returns:
- A parsed resource. Note that the returned object will be an instance of
IResource
orIAnyResource
depending on the specific FhirContext which created this parser. - Throws:
DataFormatException
- If the resource can not be parsed because the data is not recognized or invalid for any reasonConfigurationException
-
setDontEncodeElements
If provided, specifies the elements which should NOT be encoded. Valid values for this field would include:- Patient - Don't encode patient and all its children
- Patient.name - Don't encode the patient's name
- Patient.name.family - Don't encode the patient's family name
- *.text - Don't encode the text element on any resource (only the very first position may contain a wildcard)
DSTU2 note: Note that values including meta, such as
Patient.meta
will work for DSTU2 parsers, but values with subelements on meta such asPatient.meta.lastUpdated
will only work in DSTU3+ mode.- Parameters:
theDontEncodeElements
- The elements to encode- See Also:
-
setEncodeElements
If provided, specifies the elements which should be encoded, to the exclusion of all others. Valid values for this field would include:- Patient - Encode patient and all its children
- Patient.name - Encode only the patient's name
- Patient.name.family - Encode only the patient's family name
- *.text - Encode the text element on any resource (only the very first position may contain a wildcard)
- *.(mandatory) - This is a special case which causes any mandatory fields (min > 0) to be encoded
- Parameters:
theEncodeElements
- The elements to encode- See Also:
-
isEncodeElementsAppliesToChildResourcesOnly
If set totrue
(default is false), the values supplied tosetEncodeElements(Set)
will not be applied to the root resource (typically a Bundle), but will be applied to any sub-resources contained within it (i.e. search result resources in that bundle) -
setEncodeElementsAppliesToChildResourcesOnly
void setEncodeElementsAppliesToChildResourcesOnly(boolean theEncodeElementsAppliesToChildResourcesOnly) If set totrue
(default is false), the values supplied tosetEncodeElements(Set)
will not be applied to the root resource (typically a Bundle), but will be applied to any sub-resources contained within it (i.e. search result resources in that bundle) -
setParserErrorHandler
Registers an error handler which will be invoked when any parse errors are found- Parameters:
theErrorHandler
- The error handler to set. Must not be null.
-
setPrettyPrint
Sets the "pretty print" flag, meaning that the parser will encode resources with human-readable spacing and newlines between elements instead of condensing output as much as possible.- Parameters:
thePrettyPrint
- The flag- Returns:
- Returns an instance of
this
parser so that method calls can be chained together
-
setServerBaseUrl
Sets the server's base URL used by this parser. If a value is set, resource references will be turned into relative references if they are provided as absolute URLs but have a base matching the given base.- Parameters:
theUrl
- The base URL, e.g. "http://example.com/base"- Returns:
- Returns an instance of
this
parser so that method calls can be chained together
-
setOverrideResourceIdWithBundleEntryFullUrl
IParser setOverrideResourceIdWithBundleEntryFullUrl(Boolean theOverrideResourceIdWithBundleEntryFullUrl) If set totrue
(which is the default), the Bundle.entry.fullUrl will override the Bundle.entry.resource's resource id if the fullUrl is defined. This behavior happens when parsing the source data into a Bundle object. Set this tofalse
if this is not the desired behavior (e.g. the client code wishes to perform additional validation checks between the fullUrl and the resource id).- Parameters:
theOverrideResourceIdWithBundleEntryFullUrl
- Set this tofalse
to prevent the parser from overriding resource ids with the Bundle.entry.fullUrl (ornull
to apply the default setting from theParserOptions
)- Returns:
- Returns a reference to
this
parser so that method calls can be chained together - See Also:
-
setSuppressNarratives
If set totrue
(default isfalse
), narratives will not be included in the encoded values. -
getDontStripVersionsFromReferencesAtPaths
Returns the value supplied tosetDontStripVersionsFromReferencesAtPaths(String...)
ornull
if no value has been set for this parser (in which case the default from theParserOptions
will be used}- See Also:
-
setDontStripVersionsFromReferencesAtPaths
If supplied value(s), any resource references at the specified paths will have their resource versions encoded instead of being automatically stripped during the encoding process. This setting has no effect on the parsing process.This method provides a finer-grained level of control than
setStripVersionsFromReferences(Boolean)
and any paths specified by this method will be encoded even ifsetStripVersionsFromReferences(Boolean)
has been set totrue
(which is the default)- Parameters:
thePaths
- A collection of paths for which the resource versions will not be removed automatically when serializing, e.g. "Patient.managingOrganization" or "AuditEvent.object.reference". Note that only resource name and field names with dots separating is allowed here (no repetition indicators, FluentPath expressions, etc.). Set tonull
to use the value set in theParserOptions
- Returns:
- Returns a reference to
this
parser so that method calls can be chained together - See Also:
-
setDontStripVersionsFromReferencesAtPaths
If supplied value(s), any resource references at the specified paths will have their resource versions encoded instead of being automatically stripped during the encoding process. This setting has no effect on the parsing process.This method provides a finer-grained level of control than
setStripVersionsFromReferences(Boolean)
and any paths specified by this method will be encoded even ifsetStripVersionsFromReferences(Boolean)
has been set totrue
(which is the default)- Parameters:
thePaths
- A collection of paths for which the resource versions will not be removed automatically when serializing, e.g. "Patient.managingOrganization" or "AuditEvent.object.reference". Note that only resource name and field names with dots separating is allowed here (no repetition indicators, FluentPath expressions, etc.). Set tonull
to use the value set in theParserOptions
- Returns:
- Returns a reference to
this
parser so that method calls can be chained together - See Also:
-