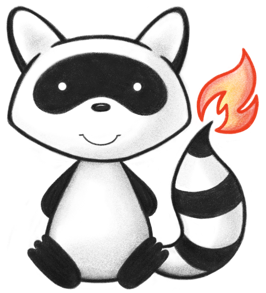
001package org.hl7.fhir.r5.utils.formats; 002 003import java.io.File; 004import java.io.FileInputStream; 005import java.io.FileOutputStream; 006import java.util.ArrayList; 007import java.util.List; 008 009import javax.sound.sampled.FloatControl.Type; 010 011import org.hl7.fhir.r5.formats.JsonParser; 012import org.hl7.fhir.r5.formats.XmlParser; 013import org.hl7.fhir.r5.formats.IParser.OutputStyle; 014import org.hl7.fhir.r5.model.Resource; 015 016public class ResourceFolderVisitor { 017 018 public interface IResourceObserver { 019 public boolean visitResource(String filename, Resource resource); 020 } 021 022 private IResourceObserver observer; 023 private List<String> types = new ArrayList<>(); 024 025 public ResourceFolderVisitor(IResourceObserver observer) { 026 super(); 027 this.observer = observer; 028 } 029 030 public ResourceFolderVisitor(IResourceObserver observer, String... types) { 031 super(); 032 this.observer = observer; 033 for (String t : types) { 034 this.types.add(t); 035 } 036 } 037 038 039 public void visit(String folder) { 040 visit(new File(folder)); 041 } 042 043 public void visit(File file) { 044 for (File f : file.listFiles()) { 045 if (f.isDirectory()) { 046 visit(f); 047 } else if (f.getName().endsWith(".xml")) { 048 try { 049 Resource res = new XmlParser().parse(new FileInputStream(f)); 050 if (types.isEmpty() || types.contains(res.fhirType())) { 051 if (observer.visitResource(f.getAbsolutePath(), res)) { 052 new XmlParser().setOutputStyle(OutputStyle.PRETTY).compose(new FileOutputStream(f), res); 053 } 054 } 055 } catch (Exception e) { 056 } 057 } else if (f.getName().endsWith(".json")) { 058 try { 059 Resource res = new JsonParser().parse(new FileInputStream(f)); 060 if (types.isEmpty() || types.contains(res.fhirType())) { 061 if (observer.visitResource(f.getAbsolutePath(), res)) { 062 new JsonParser().setOutputStyle(OutputStyle.PRETTY).compose(new FileOutputStream(f), res); 063 } 064 } 065 } catch (Exception e) { 066 } 067 } 068 } 069 } 070 071 072}