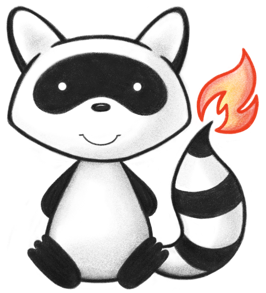
001package org.hl7.fhir.r5.terminologies.client; 002 003import java.util.HashSet; 004import java.util.Set; 005 006import org.hl7.fhir.r5.model.CanonicalResource; 007import org.hl7.fhir.r5.model.TerminologyCapabilities; 008import org.hl7.fhir.r5.model.ValueSet; 009 010public class TerminologyClientContext { 011 private String cacheId; 012 private boolean isTxCaching; 013 private int serverQueryCount = 0; 014 private final Set<String> cached = new HashSet<>(); 015 protected String server; 016 private ITerminologyClient client; 017 private TerminologyCapabilities txcaps; 018 019 public String getCacheId() { 020 return cacheId; 021 } 022 023 public void setCacheId(String cacheId) { 024 this.cacheId = cacheId; 025 } 026 027 public boolean isTxCaching() { 028 return isTxCaching; 029 } 030 031 public void setTxCaching(boolean isTxCaching) { 032 this.isTxCaching = isTxCaching; 033 } 034 035 public int getServerQueryCount() { 036 return serverQueryCount; 037 } 038 039 public void setServerQueryCount(int serverQueryCount) { 040 this.serverQueryCount = serverQueryCount; 041 } 042 043 public Set<String> getCached() { 044 return cached; 045 } 046 047 public String getServer() { 048 return server; 049 } 050 051 public void setServer(String server) { 052 this.server = server; 053 } 054 055 public ITerminologyClient getClient() { 056 return client; 057 } 058 059 public void setClient(ITerminologyClient client) { 060 this.client = client; 061 } 062 063 public TerminologyCapabilities getTxcaps() { 064 return txcaps; 065 } 066 067 public void setTxcaps(TerminologyCapabilities txcaps) { 068 this.txcaps = txcaps; 069 } 070 071 public void copy(TerminologyClientContext other) { 072 cacheId = other.cacheId; 073 isTxCaching = other.isTxCaching; 074 cached.addAll(other.cached); 075// tsServer = other.tsServer; 076 client = other.client; 077 txcaps = other.txcaps; 078 079 } 080 081 public boolean usingCache() { 082 return isTxCaching && cacheId != null; 083 } 084 085 public boolean alreadyCached(CanonicalResource cr) { 086 return cached.contains(cr.getVUrl()); 087 } 088 089 public void addToCache(CanonicalResource cr) { 090 cached.add(cr.getVUrl()); 091 } 092 093 094 095}