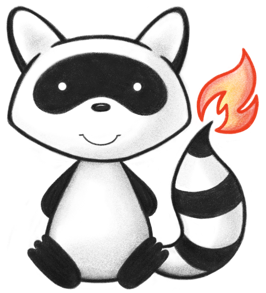
001package org.hl7.fhir.r5.patterns; 002 003 004 005 006import java.util.Date; 007import java.util.List; 008 009import org.hl7.fhir.exceptions.FHIRException; 010 011/* 012 Copyright (c) 2011+, HL7, Inc. 013 All rights reserved. 014 015 Redistribution and use in source and binary forms, with or without modification, 016 are permitted provided that the following conditions are met: 017 018 * Redistributions of source code must retain the above copyright notice, this 019 list of conditions and the following disclaimer. 020 * Redistributions in binary form must reproduce the above copyright notice, 021 this list of conditions and the following disclaimer in the documentation 022 and/or other materials provided with the distribution. 023 * Neither the name of HL7 nor the names of its contributors may be used to 024 endorse or promote products derived from this software without specific 025 prior written permission. 026 027 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 028 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 029 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 030 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 031 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 032 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 033 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 034 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 035 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 036 POSSIBILITY OF SUCH DAMAGE. 037 038*/ 039 040// Generated on Wed, May 8, 2019 10:40+1000 for FHIR v4.1.0 041import org.hl7.fhir.r5.model.Base; 042import org.hl7.fhir.r5.model.CodeableConcept; 043import org.hl7.fhir.r5.model.Configuration; 044import org.hl7.fhir.r5.model.DataType; 045import org.hl7.fhir.r5.model.DateTimeType; 046import org.hl7.fhir.r5.model.EnumFactory; 047import org.hl7.fhir.r5.model.Enumeration; 048import org.hl7.fhir.r5.model.Identifier; 049import org.hl7.fhir.r5.model.InstantType; 050import org.hl7.fhir.r5.model.Period; 051import org.hl7.fhir.r5.model.PrimitiveType; 052import org.hl7.fhir.r5.model.Reference; 053import org.hl7.fhir.r5.model.StringType; 054import org.hl7.fhir.r5.model.Timing; 055/** 056 * Who What When Where Why - Common pattern for all resources that deals with attribution. 057 */ 058public interface Fivews extends PatternBase { 059 060 public enum CanonicalStatus { 061 /** 062 * The resource was created in error, and should not be treated as valid (note: in many cases, for various data integrity related reasons, the information cannot be removed from the record) 063 */ 064 ERROR, 065 /** 066 * The resource describes an action or plan that is proposed, and not yet approved by the participants 067 */ 068 PROPOSED, 069 /** 070 * The resource describes a course of action that is planned and agreed/approved, but at the time of recording was still future 071 */ 072 PLANNED, 073 /** 074 * The information in the resource is still being prepared and edited 075 */ 076 DRAFT, 077 /** 078 * A fulfiller has been asked to perform this action, but it has not yet occurred 079 */ 080 REQUESTED, 081 /** 082 * The fulfiller has received the request, but not yet agreed to carry out the action 083 */ 084 RECEIVED, 085 /** 086 * The fulfiller chose not to perform the action 087 */ 088 DECLINED, 089 /** 090 * The fulfiller has decided to perform the action, and plans are in train to do this in the future 091 */ 092 ACCEPTED, 093 /** 094 * The pre-conditions for the action are all fulfilled, and it is imminent 095 */ 096 ARRIVED, 097 /** 098 * The resource describes information that is currently valid or a process that is presently occuring 099 */ 100 ACTIVE, 101 /** 102 * The process described/requested in this resource has been halted for some reason 103 */ 104 SUSPENDED, 105 /** 106 * The process described/requested in the resource could not be completed, and no further action is planned 107 */ 108 FAILED, 109 /** 110 * The information in this resource has been replaced by information in another resource 111 */ 112 REPLACED, 113 /** 114 * The process described/requested in the resource has been completed, and no further action is planned 115 */ 116 COMPLETE, 117 /** 118 * The resource describes information that is no longer valid or a process that is stopped occurring 119 */ 120 INACTIVE, 121 /** 122 * The process described/requested in the resource did not complete - usually due to some workflow error, and no further action is planned 123 */ 124 ABANDONED, 125 /** 126 * Authoring system does not know the status 127 */ 128 UNKNOWN, 129 /** 130 * The information in this resource is not yet approved 131 */ 132 UNCONFIRMED, 133 /** 134 * The information in this resource is approved 135 */ 136 CONFIRMED, 137 /** 138 * The issue identified by this resource is no longer of concern 139 */ 140 RESOLVED, 141 /** 142 * This information has been ruled out by testing and evaluation 143 */ 144 REFUTED, 145 /** 146 * Potentially true? 147 */ 148 DIFFERENTIAL, 149 /** 150 * This information is still being assembled 151 */ 152 PARTIAL, 153 /** 154 * not available at this time/location 155 */ 156 BUSYUNAVAILABLE, 157 /** 158 * Free for scheduling 159 */ 160 FREE, 161 /** 162 * Ready to act 163 */ 164 ONTARGET, 165 /** 166 * Ahead of the planned timelines 167 */ 168 AHEADOFTARGET, 169 /** 170 * 171 */ 172 BEHINDTARGET, 173 /** 174 * Behind the planned timelines 175 */ 176 NOTREADY, 177 /** 178 * The device transducer is disconnected 179 */ 180 TRANSDUCDISCON, 181 /** 182 * The hardware is disconnected 183 */ 184 HWDISCON, 185 /** 186 * added to help the parsers with the generic types 187 */ 188 NULL; 189 public static CanonicalStatus fromCode(String codeString) throws FHIRException { 190 if (codeString == null || "".equals(codeString)) 191 return null; 192 if ("error".equals(codeString)) 193 return ERROR; 194 if ("proposed".equals(codeString)) 195 return PROPOSED; 196 if ("planned".equals(codeString)) 197 return PLANNED; 198 if ("draft".equals(codeString)) 199 return DRAFT; 200 if ("requested".equals(codeString)) 201 return REQUESTED; 202 if ("received".equals(codeString)) 203 return RECEIVED; 204 if ("declined".equals(codeString)) 205 return DECLINED; 206 if ("accepted".equals(codeString)) 207 return ACCEPTED; 208 if ("arrived".equals(codeString)) 209 return ARRIVED; 210 if ("active".equals(codeString)) 211 return ACTIVE; 212 if ("suspended".equals(codeString)) 213 return SUSPENDED; 214 if ("failed".equals(codeString)) 215 return FAILED; 216 if ("replaced".equals(codeString)) 217 return REPLACED; 218 if ("complete".equals(codeString)) 219 return COMPLETE; 220 if ("inactive".equals(codeString)) 221 return INACTIVE; 222 if ("abandoned".equals(codeString)) 223 return ABANDONED; 224 if ("unknown".equals(codeString)) 225 return UNKNOWN; 226 if ("unconfirmed".equals(codeString)) 227 return UNCONFIRMED; 228 if ("confirmed".equals(codeString)) 229 return CONFIRMED; 230 if ("resolved".equals(codeString)) 231 return RESOLVED; 232 if ("refuted".equals(codeString)) 233 return REFUTED; 234 if ("differential".equals(codeString)) 235 return DIFFERENTIAL; 236 if ("partial".equals(codeString)) 237 return PARTIAL; 238 if ("busy-unavailable".equals(codeString)) 239 return BUSYUNAVAILABLE; 240 if ("free".equals(codeString)) 241 return FREE; 242 if ("on-target".equals(codeString)) 243 return ONTARGET; 244 if ("ahead-of-target".equals(codeString)) 245 return AHEADOFTARGET; 246 if ("behind-target".equals(codeString)) 247 return BEHINDTARGET; 248 if ("not-ready".equals(codeString)) 249 return NOTREADY; 250 if ("transduc-discon".equals(codeString)) 251 return TRANSDUCDISCON; 252 if ("hw-discon".equals(codeString)) 253 return HWDISCON; 254 if (Configuration.isAcceptInvalidEnums()) 255 return null; 256 else 257 throw new FHIRException("Unknown CanonicalStatus code '"+codeString+"'"); 258 } 259 public String toCode() { 260 switch (this) { 261 case ERROR: return "error"; 262 case PROPOSED: return "proposed"; 263 case PLANNED: return "planned"; 264 case DRAFT: return "draft"; 265 case REQUESTED: return "requested"; 266 case RECEIVED: return "received"; 267 case DECLINED: return "declined"; 268 case ACCEPTED: return "accepted"; 269 case ARRIVED: return "arrived"; 270 case ACTIVE: return "active"; 271 case SUSPENDED: return "suspended"; 272 case FAILED: return "failed"; 273 case REPLACED: return "replaced"; 274 case COMPLETE: return "complete"; 275 case INACTIVE: return "inactive"; 276 case ABANDONED: return "abandoned"; 277 case UNKNOWN: return "unknown"; 278 case UNCONFIRMED: return "unconfirmed"; 279 case CONFIRMED: return "confirmed"; 280 case RESOLVED: return "resolved"; 281 case REFUTED: return "refuted"; 282 case DIFFERENTIAL: return "differential"; 283 case PARTIAL: return "partial"; 284 case BUSYUNAVAILABLE: return "busy-unavailable"; 285 case FREE: return "free"; 286 case ONTARGET: return "on-target"; 287 case AHEADOFTARGET: return "ahead-of-target"; 288 case BEHINDTARGET: return "behind-target"; 289 case NOTREADY: return "not-ready"; 290 case TRANSDUCDISCON: return "transduc-discon"; 291 case HWDISCON: return "hw-discon"; 292 case NULL: return null; 293 default: return "?"; 294 } 295 } 296 public String getSystem() { 297 switch (this) { 298 case ERROR: return "http://hl7.org/fhir/resource-status"; 299 case PROPOSED: return "http://hl7.org/fhir/resource-status"; 300 case PLANNED: return "http://hl7.org/fhir/resource-status"; 301 case DRAFT: return "http://hl7.org/fhir/resource-status"; 302 case REQUESTED: return "http://hl7.org/fhir/resource-status"; 303 case RECEIVED: return "http://hl7.org/fhir/resource-status"; 304 case DECLINED: return "http://hl7.org/fhir/resource-status"; 305 case ACCEPTED: return "http://hl7.org/fhir/resource-status"; 306 case ARRIVED: return "http://hl7.org/fhir/resource-status"; 307 case ACTIVE: return "http://hl7.org/fhir/resource-status"; 308 case SUSPENDED: return "http://hl7.org/fhir/resource-status"; 309 case FAILED: return "http://hl7.org/fhir/resource-status"; 310 case REPLACED: return "http://hl7.org/fhir/resource-status"; 311 case COMPLETE: return "http://hl7.org/fhir/resource-status"; 312 case INACTIVE: return "http://hl7.org/fhir/resource-status"; 313 case ABANDONED: return "http://hl7.org/fhir/resource-status"; 314 case UNKNOWN: return "http://hl7.org/fhir/resource-status"; 315 case UNCONFIRMED: return "http://hl7.org/fhir/resource-status"; 316 case CONFIRMED: return "http://hl7.org/fhir/resource-status"; 317 case RESOLVED: return "http://hl7.org/fhir/resource-status"; 318 case REFUTED: return "http://hl7.org/fhir/resource-status"; 319 case DIFFERENTIAL: return "http://hl7.org/fhir/resource-status"; 320 case PARTIAL: return "http://hl7.org/fhir/resource-status"; 321 case BUSYUNAVAILABLE: return "http://hl7.org/fhir/resource-status"; 322 case FREE: return "http://hl7.org/fhir/resource-status"; 323 case ONTARGET: return "http://hl7.org/fhir/resource-status"; 324 case AHEADOFTARGET: return "http://hl7.org/fhir/resource-status"; 325 case BEHINDTARGET: return "http://hl7.org/fhir/resource-status"; 326 case NOTREADY: return "http://hl7.org/fhir/resource-status"; 327 case TRANSDUCDISCON: return "http://hl7.org/fhir/resource-status"; 328 case HWDISCON: return "http://hl7.org/fhir/resource-status"; 329 case NULL: return null; 330 default: return "?"; 331 } 332 } 333 public String getDefinition() { 334 switch (this) { 335 case ERROR: return "The resource was created in error, and should not be treated as valid (note: in many cases, for various data integrity related reasons, the information cannot be removed from the record)"; 336 case PROPOSED: return "The resource describes an action or plan that is proposed, and not yet approved by the participants"; 337 case PLANNED: return "The resource describes a course of action that is planned and agreed/approved, but at the time of recording was still future"; 338 case DRAFT: return "The information in the resource is still being prepared and edited"; 339 case REQUESTED: return "A fulfiller has been asked to perform this action, but it has not yet occurred"; 340 case RECEIVED: return "The fulfiller has received the request, but not yet agreed to carry out the action"; 341 case DECLINED: return "The fulfiller chose not to perform the action"; 342 case ACCEPTED: return "The fulfiller has decided to perform the action, and plans are in train to do this in the future"; 343 case ARRIVED: return "The pre-conditions for the action are all fulfilled, and it is imminent"; 344 case ACTIVE: return "The resource describes information that is currently valid or a process that is presently occuring"; 345 case SUSPENDED: return "The process described/requested in this resource has been halted for some reason"; 346 case FAILED: return "The process described/requested in the resource could not be completed, and no further action is planned"; 347 case REPLACED: return "The information in this resource has been replaced by information in another resource"; 348 case COMPLETE: return "The process described/requested in the resource has been completed, and no further action is planned"; 349 case INACTIVE: return "The resource describes information that is no longer valid or a process that is stopped occurring"; 350 case ABANDONED: return "The process described/requested in the resource did not complete - usually due to some workflow error, and no further action is planned"; 351 case UNKNOWN: return "Authoring system does not know the status"; 352 case UNCONFIRMED: return "The information in this resource is not yet approved"; 353 case CONFIRMED: return "The information in this resource is approved"; 354 case RESOLVED: return "The issue identified by this resource is no longer of concern"; 355 case REFUTED: return "This information has been ruled out by testing and evaluation"; 356 case DIFFERENTIAL: return "Potentially true?"; 357 case PARTIAL: return "This information is still being assembled"; 358 case BUSYUNAVAILABLE: return "not available at this time/location"; 359 case FREE: return "Free for scheduling"; 360 case ONTARGET: return "Ready to act"; 361 case AHEADOFTARGET: return "Ahead of the planned timelines"; 362 case BEHINDTARGET: return ""; 363 case NOTREADY: return "Behind the planned timelines"; 364 case TRANSDUCDISCON: return "The device transducer is disconnected"; 365 case HWDISCON: return "The hardware is disconnected"; 366 case NULL: return null; 367 default: return "?"; 368 } 369 } 370 public String getDisplay() { 371 switch (this) { 372 case ERROR: return "error"; 373 case PROPOSED: return "proposed"; 374 case PLANNED: return "planned"; 375 case DRAFT: return "draft"; 376 case REQUESTED: return "requested"; 377 case RECEIVED: return "received"; 378 case DECLINED: return "declined"; 379 case ACCEPTED: return "accepted"; 380 case ARRIVED: return "arrived"; 381 case ACTIVE: return "active"; 382 case SUSPENDED: return "suspended"; 383 case FAILED: return "failed"; 384 case REPLACED: return "replaced"; 385 case COMPLETE: return "complete"; 386 case INACTIVE: return "inactive"; 387 case ABANDONED: return "abandoned"; 388 case UNKNOWN: return "unknown"; 389 case UNCONFIRMED: return "unconfirmed"; 390 case CONFIRMED: return "confirmed"; 391 case RESOLVED: return "resolved"; 392 case REFUTED: return "refuted"; 393 case DIFFERENTIAL: return "differential"; 394 case PARTIAL: return "partial"; 395 case BUSYUNAVAILABLE: return "busy-unavailable"; 396 case FREE: return "free"; 397 case ONTARGET: return "on-target"; 398 case AHEADOFTARGET: return "ahead-of-target"; 399 case BEHINDTARGET: return "behind-target"; 400 case NOTREADY: return "not-ready"; 401 case TRANSDUCDISCON: return "transduc-discon"; 402 case HWDISCON: return "hw-discon"; 403 case NULL: return null; 404 default: return "?"; 405 } 406 } 407 } 408 409 public class CanonicalStatusEnumFactory implements EnumFactory<CanonicalStatus> { 410 public CanonicalStatus fromCode(String codeString) throws IllegalArgumentException { 411 if (codeString == null || "".equals(codeString)) 412 if (codeString == null || "".equals(codeString)) 413 return null; 414 if ("error".equals(codeString)) 415 return CanonicalStatus.ERROR; 416 if ("proposed".equals(codeString)) 417 return CanonicalStatus.PROPOSED; 418 if ("planned".equals(codeString)) 419 return CanonicalStatus.PLANNED; 420 if ("draft".equals(codeString)) 421 return CanonicalStatus.DRAFT; 422 if ("requested".equals(codeString)) 423 return CanonicalStatus.REQUESTED; 424 if ("received".equals(codeString)) 425 return CanonicalStatus.RECEIVED; 426 if ("declined".equals(codeString)) 427 return CanonicalStatus.DECLINED; 428 if ("accepted".equals(codeString)) 429 return CanonicalStatus.ACCEPTED; 430 if ("arrived".equals(codeString)) 431 return CanonicalStatus.ARRIVED; 432 if ("active".equals(codeString)) 433 return CanonicalStatus.ACTIVE; 434 if ("suspended".equals(codeString)) 435 return CanonicalStatus.SUSPENDED; 436 if ("failed".equals(codeString)) 437 return CanonicalStatus.FAILED; 438 if ("replaced".equals(codeString)) 439 return CanonicalStatus.REPLACED; 440 if ("complete".equals(codeString)) 441 return CanonicalStatus.COMPLETE; 442 if ("inactive".equals(codeString)) 443 return CanonicalStatus.INACTIVE; 444 if ("abandoned".equals(codeString)) 445 return CanonicalStatus.ABANDONED; 446 if ("unknown".equals(codeString)) 447 return CanonicalStatus.UNKNOWN; 448 if ("unconfirmed".equals(codeString)) 449 return CanonicalStatus.UNCONFIRMED; 450 if ("confirmed".equals(codeString)) 451 return CanonicalStatus.CONFIRMED; 452 if ("resolved".equals(codeString)) 453 return CanonicalStatus.RESOLVED; 454 if ("refuted".equals(codeString)) 455 return CanonicalStatus.REFUTED; 456 if ("differential".equals(codeString)) 457 return CanonicalStatus.DIFFERENTIAL; 458 if ("partial".equals(codeString)) 459 return CanonicalStatus.PARTIAL; 460 if ("busy-unavailable".equals(codeString)) 461 return CanonicalStatus.BUSYUNAVAILABLE; 462 if ("free".equals(codeString)) 463 return CanonicalStatus.FREE; 464 if ("on-target".equals(codeString)) 465 return CanonicalStatus.ONTARGET; 466 if ("ahead-of-target".equals(codeString)) 467 return CanonicalStatus.AHEADOFTARGET; 468 if ("behind-target".equals(codeString)) 469 return CanonicalStatus.BEHINDTARGET; 470 if ("not-ready".equals(codeString)) 471 return CanonicalStatus.NOTREADY; 472 if ("transduc-discon".equals(codeString)) 473 return CanonicalStatus.TRANSDUCDISCON; 474 if ("hw-discon".equals(codeString)) 475 return CanonicalStatus.HWDISCON; 476 throw new IllegalArgumentException("Unknown CanonicalStatus code '"+codeString+"'"); 477 } 478 public Enumeration<CanonicalStatus> fromType(PrimitiveType<?> code) throws FHIRException { 479 if (code == null) 480 return null; 481 if (code.isEmpty()) 482 return new Enumeration<CanonicalStatus>(this, CanonicalStatus.NULL, code); 483 String codeString = ((PrimitiveType) code).asStringValue(); 484 if (codeString == null || "".equals(codeString)) 485 return new Enumeration<CanonicalStatus>(this, CanonicalStatus.NULL, code); 486 if ("error".equals(codeString)) 487 return new Enumeration<CanonicalStatus>(this, CanonicalStatus.ERROR, code); 488 if ("proposed".equals(codeString)) 489 return new Enumeration<CanonicalStatus>(this, CanonicalStatus.PROPOSED, code); 490 if ("planned".equals(codeString)) 491 return new Enumeration<CanonicalStatus>(this, CanonicalStatus.PLANNED, code); 492 if ("draft".equals(codeString)) 493 return new Enumeration<CanonicalStatus>(this, CanonicalStatus.DRAFT, code); 494 if ("requested".equals(codeString)) 495 return new Enumeration<CanonicalStatus>(this, CanonicalStatus.REQUESTED, code); 496 if ("received".equals(codeString)) 497 return new Enumeration<CanonicalStatus>(this, CanonicalStatus.RECEIVED, code); 498 if ("declined".equals(codeString)) 499 return new Enumeration<CanonicalStatus>(this, CanonicalStatus.DECLINED, code); 500 if ("accepted".equals(codeString)) 501 return new Enumeration<CanonicalStatus>(this, CanonicalStatus.ACCEPTED, code); 502 if ("arrived".equals(codeString)) 503 return new Enumeration<CanonicalStatus>(this, CanonicalStatus.ARRIVED, code); 504 if ("active".equals(codeString)) 505 return new Enumeration<CanonicalStatus>(this, CanonicalStatus.ACTIVE, code); 506 if ("suspended".equals(codeString)) 507 return new Enumeration<CanonicalStatus>(this, CanonicalStatus.SUSPENDED, code); 508 if ("failed".equals(codeString)) 509 return new Enumeration<CanonicalStatus>(this, CanonicalStatus.FAILED, code); 510 if ("replaced".equals(codeString)) 511 return new Enumeration<CanonicalStatus>(this, CanonicalStatus.REPLACED, code); 512 if ("complete".equals(codeString)) 513 return new Enumeration<CanonicalStatus>(this, CanonicalStatus.COMPLETE, code); 514 if ("inactive".equals(codeString)) 515 return new Enumeration<CanonicalStatus>(this, CanonicalStatus.INACTIVE, code); 516 if ("abandoned".equals(codeString)) 517 return new Enumeration<CanonicalStatus>(this, CanonicalStatus.ABANDONED, code); 518 if ("unknown".equals(codeString)) 519 return new Enumeration<CanonicalStatus>(this, CanonicalStatus.UNKNOWN, code); 520 if ("unconfirmed".equals(codeString)) 521 return new Enumeration<CanonicalStatus>(this, CanonicalStatus.UNCONFIRMED, code); 522 if ("confirmed".equals(codeString)) 523 return new Enumeration<CanonicalStatus>(this, CanonicalStatus.CONFIRMED, code); 524 if ("resolved".equals(codeString)) 525 return new Enumeration<CanonicalStatus>(this, CanonicalStatus.RESOLVED, code); 526 if ("refuted".equals(codeString)) 527 return new Enumeration<CanonicalStatus>(this, CanonicalStatus.REFUTED, code); 528 if ("differential".equals(codeString)) 529 return new Enumeration<CanonicalStatus>(this, CanonicalStatus.DIFFERENTIAL, code); 530 if ("partial".equals(codeString)) 531 return new Enumeration<CanonicalStatus>(this, CanonicalStatus.PARTIAL, code); 532 if ("busy-unavailable".equals(codeString)) 533 return new Enumeration<CanonicalStatus>(this, CanonicalStatus.BUSYUNAVAILABLE, code); 534 if ("free".equals(codeString)) 535 return new Enumeration<CanonicalStatus>(this, CanonicalStatus.FREE, code); 536 if ("on-target".equals(codeString)) 537 return new Enumeration<CanonicalStatus>(this, CanonicalStatus.ONTARGET, code); 538 if ("ahead-of-target".equals(codeString)) 539 return new Enumeration<CanonicalStatus>(this, CanonicalStatus.AHEADOFTARGET, code); 540 if ("behind-target".equals(codeString)) 541 return new Enumeration<CanonicalStatus>(this, CanonicalStatus.BEHINDTARGET, code); 542 if ("not-ready".equals(codeString)) 543 return new Enumeration<CanonicalStatus>(this, CanonicalStatus.NOTREADY, code); 544 if ("transduc-discon".equals(codeString)) 545 return new Enumeration<CanonicalStatus>(this, CanonicalStatus.TRANSDUCDISCON, code); 546 if ("hw-discon".equals(codeString)) 547 return new Enumeration<CanonicalStatus>(this, CanonicalStatus.HWDISCON, code); 548 throw new FHIRException("Unknown CanonicalStatus code '"+codeString+"'"); 549 } 550 public String toCode(CanonicalStatus code) { 551 if (code == CanonicalStatus.ERROR) 552 return "error"; 553 if (code == CanonicalStatus.PROPOSED) 554 return "proposed"; 555 if (code == CanonicalStatus.PLANNED) 556 return "planned"; 557 if (code == CanonicalStatus.DRAFT) 558 return "draft"; 559 if (code == CanonicalStatus.REQUESTED) 560 return "requested"; 561 if (code == CanonicalStatus.RECEIVED) 562 return "received"; 563 if (code == CanonicalStatus.DECLINED) 564 return "declined"; 565 if (code == CanonicalStatus.ACCEPTED) 566 return "accepted"; 567 if (code == CanonicalStatus.ARRIVED) 568 return "arrived"; 569 if (code == CanonicalStatus.ACTIVE) 570 return "active"; 571 if (code == CanonicalStatus.SUSPENDED) 572 return "suspended"; 573 if (code == CanonicalStatus.FAILED) 574 return "failed"; 575 if (code == CanonicalStatus.REPLACED) 576 return "replaced"; 577 if (code == CanonicalStatus.COMPLETE) 578 return "complete"; 579 if (code == CanonicalStatus.INACTIVE) 580 return "inactive"; 581 if (code == CanonicalStatus.ABANDONED) 582 return "abandoned"; 583 if (code == CanonicalStatus.UNKNOWN) 584 return "unknown"; 585 if (code == CanonicalStatus.UNCONFIRMED) 586 return "unconfirmed"; 587 if (code == CanonicalStatus.CONFIRMED) 588 return "confirmed"; 589 if (code == CanonicalStatus.RESOLVED) 590 return "resolved"; 591 if (code == CanonicalStatus.REFUTED) 592 return "refuted"; 593 if (code == CanonicalStatus.DIFFERENTIAL) 594 return "differential"; 595 if (code == CanonicalStatus.PARTIAL) 596 return "partial"; 597 if (code == CanonicalStatus.BUSYUNAVAILABLE) 598 return "busy-unavailable"; 599 if (code == CanonicalStatus.FREE) 600 return "free"; 601 if (code == CanonicalStatus.ONTARGET) 602 return "on-target"; 603 if (code == CanonicalStatus.AHEADOFTARGET) 604 return "ahead-of-target"; 605 if (code == CanonicalStatus.BEHINDTARGET) 606 return "behind-target"; 607 if (code == CanonicalStatus.NOTREADY) 608 return "not-ready"; 609 if (code == CanonicalStatus.TRANSDUCDISCON) 610 return "transduc-discon"; 611 if (code == CanonicalStatus.HWDISCON) 612 return "hw-discon"; 613 return "?"; 614 } 615 public String toSystem(CanonicalStatus code) { 616 return code.getSystem(); 617 } 618 } 619 620 /** 621 * @return {@link #identifier} (Business Identifier.) 622 */ 623 public List<Identifier> getIdentifier() throws FHIRException; 624 625 /** 626 * @return Returns a reference to <code>this</code> for easy method chaining 627 */ 628 public Fivews setIdentifier(List<Identifier> theIdentifier) throws FHIRException; 629 630 /** 631 * @return whether there is more than zero values for identifier 632 */ 633 public boolean hasIdentifier(); 634 /** 635 * @return minimum allowed cardinality for identifier. Note that with patterns, this may be different for the underlying resource 636 */ 637 public int getIdentifierMin(); 638 /** 639 * @return maximum allowed cardinality for identifier. Note that with patterns, this may be different for the underlying resource 640 */ 641 public int getIdentifierMax(); 642 643 public Identifier addIdentifier() throws FHIRException; 644 645 public Fivews addIdentifier(Identifier t) throws FHIRException; 646 647 /** 648 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 649 */ 650 public Identifier getIdentifierFirstRep() throws FHIRException; 651 652 /** 653 * @return {@link #version} (Identifier for this version.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 654 */ 655 public StringType getVersionElement() throws FHIRException; 656 657 /** 658 * @return whether there is more than zero values for version 659 */ 660 public boolean hasVersion(); 661 /** 662 * @return minimum allowed cardinality for version. Note that with patterns, this may be different for the underlying resource 663 */ 664 public int getVersionMin() throws FHIRException; 665 /** 666 * @return maximum allowed cardinality for version. Note that with patterns, this may be different for the underlying resource 667 */ 668 public int getVersionMax() throws FHIRException; 669 public boolean hasVersionElement(); 670 671 /** 672 * @param value {@link #version} (Identifier for this version.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 673 */ 674 public Fivews setVersionElement(StringType value) throws FHIRException; 675 676 /** 677 * @return Identifier for this version. 678 */ 679 public String getVersion() throws FHIRException; 680 681 /** 682 * @param value Identifier for this version. 683 */ 684 public Fivews setVersion(String value) throws FHIRException; 685 686 /** 687 * @return {@link #status} (Status Field.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 688 */ 689 public Enumeration<CanonicalStatus> getStatusElement() throws FHIRException; 690 691 /** 692 * @return whether there is more than zero values for status 693 */ 694 public boolean hasStatus(); 695 /** 696 * @return minimum allowed cardinality for status. Note that with patterns, this may be different for the underlying resource 697 */ 698 public int getStatusMin() throws FHIRException; 699 /** 700 * @return maximum allowed cardinality for status. Note that with patterns, this may be different for the underlying resource 701 */ 702 public int getStatusMax() throws FHIRException; 703 public boolean hasStatusElement(); 704 705 /** 706 * @param value {@link #status} (Status Field.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 707 */ 708 public Fivews setStatusElement(Enumeration<CanonicalStatus> value) throws FHIRException; 709 710 /** 711 * @return Status Field. 712 */ 713 public CanonicalStatus getStatus() throws FHIRException; 714 715 /** 716 * @param value Status Field. 717 */ 718 public Fivews setStatus(CanonicalStatus value) throws FHIRException; 719 720 /** 721 * @return {@link #class_} (Classifier Field.) 722 */ 723 public List<CodeableConcept> getClass_() throws FHIRException; 724 725 /** 726 * @return Returns a reference to <code>this</code> for easy method chaining 727 */ 728 public Fivews setClass_(List<CodeableConcept> theClass_) throws FHIRException; 729 730 /** 731 * @return whether there is more than zero values for class_ 732 */ 733 public boolean hasClass_(); 734 /** 735 * @return minimum allowed cardinality for class_. Note that with patterns, this may be different for the underlying resource 736 */ 737 public int getClass_Min(); 738 /** 739 * @return maximum allowed cardinality for class_. Note that with patterns, this may be different for the underlying resource 740 */ 741 public int getClass_Max(); 742 743 public CodeableConcept addClass_() throws FHIRException; 744 745 public Fivews addClass_(CodeableConcept t) throws FHIRException; 746 747 /** 748 * @return The first repetition of repeating field {@link #class_}, creating it if it does not already exist 749 */ 750 public CodeableConcept getClass_FirstRep() throws FHIRException; 751 752 /** 753 * @return {@link #grade} (A field that indicates the potential impact of the content of the resource.) 754 */ 755 public CodeableConcept getGrade() throws FHIRException ; 756 757 /** 758 * @return whether there is more than zero values for grade 759 */ 760 public boolean hasGrade(); 761 /** 762 * @return minimum allowed cardinality for grade. Note that with patterns, this may be different for the underlying resource 763 */ 764 public int getGradeMin(); 765 /** 766 * @return maximum allowed cardinality for grade. Note that with patterns, this may be different for the underlying resource 767 */ 768 public int getGradeMax(); 769 /** 770 * @param value {@link #grade} (A field that indicates the potential impact of the content of the resource.) 771 */ 772 public Fivews setGrade(CodeableConcept value) throws FHIRException; 773 774 /** 775 * @return {@link #what} (what this resource is about.) 776 */ 777 public DataType getWhat() throws FHIRException ; 778 779 /** 780 * @return {@link #what} (what this resource is about.) 781 */ 782 public CodeableConcept getWhatCodeableConcept() throws FHIRException; 783 784 public boolean hasWhatCodeableConcept(); 785 786 /** 787 * @return {@link #what} (what this resource is about.) 788 */ 789 public Reference getWhatReference() throws FHIRException; 790 791 public boolean hasWhatReference(); 792 793 /** 794 * @return whether there is more than zero values for what 795 */ 796 public boolean hasWhat(); 797 /** 798 * @return minimum allowed cardinality for what. Note that with patterns, this may be different for the underlying resource 799 */ 800 public int getWhatMin(); 801 /** 802 * @return maximum allowed cardinality for what. Note that with patterns, this may be different for the underlying resource 803 */ 804 public int getWhatMax(); 805 /** 806 * @param value {@link #what} (what this resource is about.) 807 */ 808 public Fivews setWhat(DataType value) throws FHIRException; 809 810 /** 811 * @return {@link #subject} (Who this resource is about.) 812 */ 813 public List<Reference> getSubject() throws FHIRException; 814 815 /** 816 * @return Returns a reference to <code>this</code> for easy method chaining 817 */ 818 public Fivews setSubject(List<Reference> theSubject) throws FHIRException; 819 820 /** 821 * @return whether there is more than zero values for subject 822 */ 823 public boolean hasSubject(); 824 /** 825 * @return minimum allowed cardinality for subject. Note that with patterns, this may be different for the underlying resource 826 */ 827 public int getSubjectMin(); 828 /** 829 * @return maximum allowed cardinality for subject. Note that with patterns, this may be different for the underlying resource 830 */ 831 public int getSubjectMax(); 832 833 public Reference addSubject() throws FHIRException; 834 835 public Fivews addSubject(Reference t) throws FHIRException; 836 837 /** 838 * @return The first repetition of repeating field {@link #subject}, creating it if it does not already exist 839 */ 840 public Reference getSubjectFirstRep() throws FHIRException; 841 842 /** 843 * @return {@link #context} (a resource that gives context for the work described in this resource (usually Encounter or EpisodeOfCare).) 844 */ 845 public Reference getContext() throws FHIRException ; 846 847 /** 848 * @return whether there is more than zero values for context 849 */ 850 public boolean hasContext(); 851 /** 852 * @return minimum allowed cardinality for context. Note that with patterns, this may be different for the underlying resource 853 */ 854 public int getContextMin(); 855 /** 856 * @return maximum allowed cardinality for context. Note that with patterns, this may be different for the underlying resource 857 */ 858 public int getContextMax(); 859 /** 860 * @param value {@link #context} (a resource that gives context for the work described in this resource (usually Encounter or EpisodeOfCare).) 861 */ 862 public Fivews setContext(Reference value) throws FHIRException; 863 864 /** 865 * @return {@link #init} (when the work described in this resource was started (or will be).). This is the underlying object with id, value and extensions. The accessor "getInit" gives direct access to the value 866 */ 867 public DateTimeType getInitElement() throws FHIRException; 868 869 /** 870 * @return whether there is more than zero values for init 871 */ 872 public boolean hasInit(); 873 /** 874 * @return minimum allowed cardinality for init. Note that with patterns, this may be different for the underlying resource 875 */ 876 public int getInitMin() throws FHIRException; 877 /** 878 * @return maximum allowed cardinality for init. Note that with patterns, this may be different for the underlying resource 879 */ 880 public int getInitMax() throws FHIRException; 881 public boolean hasInitElement(); 882 883 /** 884 * @param value {@link #init} (when the work described in this resource was started (or will be).). This is the underlying object with id, value and extensions. The accessor "getInit" gives direct access to the value 885 */ 886 public Fivews setInitElement(DateTimeType value) throws FHIRException; 887 888 /** 889 * @return when the work described in this resource was started (or will be). 890 */ 891 public Date getInit() throws FHIRException; 892 893 /** 894 * @param value when the work described in this resource was started (or will be). 895 */ 896 public Fivews setInit(Date value) throws FHIRException; 897 898 /** 899 * @return {@link #planned} (when this resource is planned to occur.) 900 */ 901 public List<Timing> getPlanned() throws FHIRException; 902 903 /** 904 * @return Returns a reference to <code>this</code> for easy method chaining 905 */ 906 public Fivews setPlanned(List<Timing> thePlanned) throws FHIRException; 907 908 /** 909 * @return whether there is more than zero values for planned 910 */ 911 public boolean hasPlanned(); 912 /** 913 * @return minimum allowed cardinality for planned. Note that with patterns, this may be different for the underlying resource 914 */ 915 public int getPlannedMin(); 916 /** 917 * @return maximum allowed cardinality for planned. Note that with patterns, this may be different for the underlying resource 918 */ 919 public int getPlannedMax(); 920 921 public Timing addPlanned() throws FHIRException; 922 923 public Fivews addPlanned(Timing t) throws FHIRException; 924 925 /** 926 * @return The first repetition of repeating field {@link #planned}, creating it if it does not already exist 927 */ 928 public Timing getPlannedFirstRep() throws FHIRException; 929 930 /** 931 * @return {@link #done} (when the work described in this resource was completed (or will be).) 932 */ 933 public DataType getDone() throws FHIRException ; 934 935 /** 936 * @return {@link #done} (when the work described in this resource was completed (or will be).) 937 */ 938 public DateTimeType getDoneDateTimeType() throws FHIRException; 939 940 public boolean hasDoneDateTimeType(); 941 942 /** 943 * @return {@link #done} (when the work described in this resource was completed (or will be).) 944 */ 945 public Period getDonePeriod() throws FHIRException; 946 947 public boolean hasDonePeriod(); 948 949 /** 950 * @return whether there is more than zero values for done 951 */ 952 public boolean hasDone(); 953 /** 954 * @return minimum allowed cardinality for done. Note that with patterns, this may be different for the underlying resource 955 */ 956 public int getDoneMin(); 957 /** 958 * @return maximum allowed cardinality for done. Note that with patterns, this may be different for the underlying resource 959 */ 960 public int getDoneMax(); 961 /** 962 * @param value {@link #done} (when the work described in this resource was completed (or will be).) 963 */ 964 public Fivews setDone(DataType value) throws FHIRException; 965 966 /** 967 * @return {@link #recorded} (when this resource itself was created.). This is the underlying object with id, value and extensions. The accessor "getRecorded" gives direct access to the value 968 */ 969 public InstantType getRecordedElement() throws FHIRException; 970 971 /** 972 * @return whether there is more than zero values for recorded 973 */ 974 public boolean hasRecorded(); 975 /** 976 * @return minimum allowed cardinality for recorded. Note that with patterns, this may be different for the underlying resource 977 */ 978 public int getRecordedMin() throws FHIRException; 979 /** 980 * @return maximum allowed cardinality for recorded. Note that with patterns, this may be different for the underlying resource 981 */ 982 public int getRecordedMax() throws FHIRException; 983 public boolean hasRecordedElement(); 984 985 /** 986 * @param value {@link #recorded} (when this resource itself was created.). This is the underlying object with id, value and extensions. The accessor "getRecorded" gives direct access to the value 987 */ 988 public Fivews setRecordedElement(InstantType value) throws FHIRException; 989 990 /** 991 * @return when this resource itself was created. 992 */ 993 public Date getRecorded() throws FHIRException; 994 995 /** 996 * @param value when this resource itself was created. 997 */ 998 public Fivews setRecorded(Date value) throws FHIRException; 999 1000 /** 1001 * @return {@link #author} (who authored the content of the resource.) 1002 */ 1003 public List<Reference> getAuthor() throws FHIRException; 1004 1005 /** 1006 * @return Returns a reference to <code>this</code> for easy method chaining 1007 */ 1008 public Fivews setAuthor(List<Reference> theAuthor) throws FHIRException; 1009 1010 /** 1011 * @return whether there is more than zero values for author 1012 */ 1013 public boolean hasAuthor(); 1014 /** 1015 * @return minimum allowed cardinality for author. Note that with patterns, this may be different for the underlying resource 1016 */ 1017 public int getAuthorMin(); 1018 /** 1019 * @return maximum allowed cardinality for author. Note that with patterns, this may be different for the underlying resource 1020 */ 1021 public int getAuthorMax(); 1022 1023 public Reference addAuthor() throws FHIRException; 1024 1025 public Fivews addAuthor(Reference t) throws FHIRException; 1026 1027 /** 1028 * @return The first repetition of repeating field {@link #author}, creating it if it does not already exist 1029 */ 1030 public Reference getAuthorFirstRep() throws FHIRException; 1031 1032 /** 1033 * @return {@link #source} (Who provided the information in this resource.) 1034 */ 1035 public List<Reference> getSource() throws FHIRException; 1036 1037 /** 1038 * @return Returns a reference to <code>this</code> for easy method chaining 1039 */ 1040 public Fivews setSource(List<Reference> theSource) throws FHIRException; 1041 1042 /** 1043 * @return whether there is more than zero values for source 1044 */ 1045 public boolean hasSource(); 1046 /** 1047 * @return minimum allowed cardinality for source. Note that with patterns, this may be different for the underlying resource 1048 */ 1049 public int getSourceMin(); 1050 /** 1051 * @return maximum allowed cardinality for source. Note that with patterns, this may be different for the underlying resource 1052 */ 1053 public int getSourceMax(); 1054 1055 public Reference addSource() throws FHIRException; 1056 1057 public Fivews addSource(Reference t) throws FHIRException; 1058 1059 /** 1060 * @return The first repetition of repeating field {@link #source}, creating it if it does not already exist 1061 */ 1062 public Reference getSourceFirstRep() throws FHIRException; 1063 1064 /** 1065 * @return {@link #actor} (who did the work described the resource (or will do).) 1066 */ 1067 public List<Reference> getActor() throws FHIRException; 1068 1069 /** 1070 * @return Returns a reference to <code>this</code> for easy method chaining 1071 */ 1072 public Fivews setActor(List<Reference> theActor) throws FHIRException; 1073 1074 /** 1075 * @return whether there is more than zero values for actor 1076 */ 1077 public boolean hasActor(); 1078 /** 1079 * @return minimum allowed cardinality for actor. Note that with patterns, this may be different for the underlying resource 1080 */ 1081 public int getActorMin(); 1082 /** 1083 * @return maximum allowed cardinality for actor. Note that with patterns, this may be different for the underlying resource 1084 */ 1085 public int getActorMax(); 1086 1087 public Reference addActor() throws FHIRException; 1088 1089 public Fivews addActor(Reference t) throws FHIRException; 1090 1091 /** 1092 * @return The first repetition of repeating field {@link #actor}, creating it if it does not already exist 1093 */ 1094 public Reference getActorFirstRep() throws FHIRException; 1095 1096 /** 1097 * @return {@link #cause} (who prompted the work described in the resource.) 1098 */ 1099 public List<Reference> getCause() throws FHIRException; 1100 1101 /** 1102 * @return Returns a reference to <code>this</code> for easy method chaining 1103 */ 1104 public Fivews setCause(List<Reference> theCause) throws FHIRException; 1105 1106 /** 1107 * @return whether there is more than zero values for cause 1108 */ 1109 public boolean hasCause(); 1110 /** 1111 * @return minimum allowed cardinality for cause. Note that with patterns, this may be different for the underlying resource 1112 */ 1113 public int getCauseMin(); 1114 /** 1115 * @return maximum allowed cardinality for cause. Note that with patterns, this may be different for the underlying resource 1116 */ 1117 public int getCauseMax(); 1118 1119 public Reference addCause() throws FHIRException; 1120 1121 public Fivews addCause(Reference t) throws FHIRException; 1122 1123 /** 1124 * @return The first repetition of repeating field {@link #cause}, creating it if it does not already exist 1125 */ 1126 public Reference getCauseFirstRep() throws FHIRException; 1127 1128 /** 1129 * @return {@link #witness} (who attests to the content of the resource (individual or org).) 1130 */ 1131 public List<Reference> getWitness() throws FHIRException; 1132 1133 /** 1134 * @return Returns a reference to <code>this</code> for easy method chaining 1135 */ 1136 public Fivews setWitness(List<Reference> theWitness) throws FHIRException; 1137 1138 /** 1139 * @return whether there is more than zero values for witness 1140 */ 1141 public boolean hasWitness(); 1142 /** 1143 * @return minimum allowed cardinality for witness. Note that with patterns, this may be different for the underlying resource 1144 */ 1145 public int getWitnessMin(); 1146 /** 1147 * @return maximum allowed cardinality for witness. Note that with patterns, this may be different for the underlying resource 1148 */ 1149 public int getWitnessMax(); 1150 1151 public Reference addWitness() throws FHIRException; 1152 1153 public Fivews addWitness(Reference t) throws FHIRException; 1154 1155 /** 1156 * @return The first repetition of repeating field {@link #witness}, creating it if it does not already exist 1157 */ 1158 public Reference getWitnessFirstRep() throws FHIRException; 1159 1160 /** 1161 * @return {@link #who} (An actor involved in the work described by this resource.) 1162 */ 1163 public List<Reference> getWho() throws FHIRException; 1164 1165 /** 1166 * @return Returns a reference to <code>this</code> for easy method chaining 1167 */ 1168 public Fivews setWho(List<Reference> theWho) throws FHIRException; 1169 1170 /** 1171 * @return whether there is more than zero values for who 1172 */ 1173 public boolean hasWho(); 1174 /** 1175 * @return minimum allowed cardinality for who. Note that with patterns, this may be different for the underlying resource 1176 */ 1177 public int getWhoMin(); 1178 /** 1179 * @return maximum allowed cardinality for who. Note that with patterns, this may be different for the underlying resource 1180 */ 1181 public int getWhoMax(); 1182 1183 public Reference addWho() throws FHIRException; 1184 1185 public Fivews addWho(Reference t) throws FHIRException; 1186 1187 /** 1188 * @return The first repetition of repeating field {@link #who}, creating it if it does not already exist 1189 */ 1190 public Reference getWhoFirstRep() throws FHIRException; 1191 1192 /** 1193 * @return {@link #where} (The location of the work described.) 1194 */ 1195 public List<DataType> getWhere() throws FHIRException; 1196 1197 /** 1198 * @return Returns a reference to <code>this</code> for easy method chaining 1199 */ 1200 public Fivews setWhere(List<DataType> theWhere) throws FHIRException; 1201 1202 /** 1203 * @return whether there is more than zero values for where 1204 */ 1205 public boolean hasWhere(); 1206 /** 1207 * @return minimum allowed cardinality for where. Note that with patterns, this may be different for the underlying resource 1208 */ 1209 public int getWhereMin(); 1210 /** 1211 * @return maximum allowed cardinality for where. Note that with patterns, this may be different for the underlying resource 1212 */ 1213 public int getWhereMax(); 1214 1215 public DataType addWhere() throws FHIRException; 1216 1217 public Fivews addWhere(DataType t) throws FHIRException; 1218 1219 /** 1220 * @return The first repetition of repeating field {@link #where}, creating it if it does not already exist 1221 */ 1222 public DataType getWhereFirstRep() throws FHIRException; 1223 1224 /** 1225 * @return {@link #why} (Why this work was done.) 1226 */ 1227 public List<DataType> getWhy() throws FHIRException; 1228 1229 /** 1230 * @return Returns a reference to <code>this</code> for easy method chaining 1231 */ 1232 public Fivews setWhy(List<DataType> theWhy) throws FHIRException; 1233 1234 /** 1235 * @return whether there is more than zero values for why 1236 */ 1237 public boolean hasWhy(); 1238 /** 1239 * @return minimum allowed cardinality for why. Note that with patterns, this may be different for the underlying resource 1240 */ 1241 public int getWhyMin(); 1242 /** 1243 * @return maximum allowed cardinality for why. Note that with patterns, this may be different for the underlying resource 1244 */ 1245 public int getWhyMax(); 1246 1247 public DataType addWhy() throws FHIRException; 1248 1249 public Fivews addWhy(DataType t) throws FHIRException; 1250 1251 /** 1252 * @return The first repetition of repeating field {@link #why}, creating it if it does not already exist 1253 */ 1254 public DataType getWhyFirstRep() throws FHIRException; 1255 1256 public String fhirType(); 1257 1258 1259}