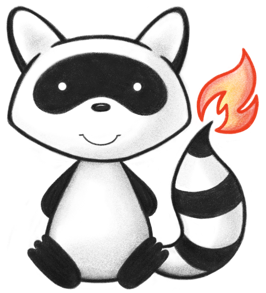
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.r5.model.Enumerations.*; 038import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.ICompositeType; 041import ca.uhn.fhir.model.api.annotation.ResourceDef; 042import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 043import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.ChildOrder; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.Block; 048 049/** 050 * Basic is used for handling concepts not yet defined in FHIR, narrative-only resources that don't map to an existing resource, and custom resources not appropriate for inclusion in the FHIR specification. 051 */ 052@ResourceDef(name="Basic", profile="http://hl7.org/fhir/StructureDefinition/Basic") 053public class Basic extends DomainResource { 054 055 /** 056 * Identifier assigned to the resource for business purposes, outside the context of FHIR. 057 */ 058 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 059 @Description(shortDefinition="Business identifier", formalDefinition="Identifier assigned to the resource for business purposes, outside the context of FHIR." ) 060 protected List<Identifier> identifier; 061 062 /** 063 * Identifies the 'type' of resource - equivalent to the resource name for other resources. 064 */ 065 @Child(name = "code", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=true, summary=true) 066 @Description(shortDefinition="Kind of Resource", formalDefinition="Identifies the 'type' of resource - equivalent to the resource name for other resources." ) 067 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/basic-resource-type") 068 protected CodeableConcept code; 069 070 /** 071 * Identifies the patient, practitioner, device or any other resource that is the "focus" of this resource. 072 */ 073 @Child(name = "subject", type = {Reference.class}, order=2, min=0, max=1, modifier=false, summary=true) 074 @Description(shortDefinition="Identifies the focus of this resource", formalDefinition="Identifies the patient, practitioner, device or any other resource that is the \"focus\" of this resource." ) 075 protected Reference subject; 076 077 /** 078 * Identifies when the resource was first created. 079 */ 080 @Child(name = "created", type = {DateTimeType.class}, order=3, min=0, max=1, modifier=false, summary=true) 081 @Description(shortDefinition="When created", formalDefinition="Identifies when the resource was first created." ) 082 protected DateTimeType created; 083 084 /** 085 * Indicates who was responsible for creating the resource instance. 086 */ 087 @Child(name = "author", type = {Practitioner.class, PractitionerRole.class, Patient.class, RelatedPerson.class, Organization.class, Device.class, CareTeam.class}, order=4, min=0, max=1, modifier=false, summary=true) 088 @Description(shortDefinition="Who created", formalDefinition="Indicates who was responsible for creating the resource instance." ) 089 protected Reference author; 090 091 private static final long serialVersionUID = -1635508686L; 092 093 /** 094 * Constructor 095 */ 096 public Basic() { 097 super(); 098 } 099 100 /** 101 * Constructor 102 */ 103 public Basic(CodeableConcept code) { 104 super(); 105 this.setCode(code); 106 } 107 108 /** 109 * @return {@link #identifier} (Identifier assigned to the resource for business purposes, outside the context of FHIR.) 110 */ 111 public List<Identifier> getIdentifier() { 112 if (this.identifier == null) 113 this.identifier = new ArrayList<Identifier>(); 114 return this.identifier; 115 } 116 117 /** 118 * @return Returns a reference to <code>this</code> for easy method chaining 119 */ 120 public Basic setIdentifier(List<Identifier> theIdentifier) { 121 this.identifier = theIdentifier; 122 return this; 123 } 124 125 public boolean hasIdentifier() { 126 if (this.identifier == null) 127 return false; 128 for (Identifier item : this.identifier) 129 if (!item.isEmpty()) 130 return true; 131 return false; 132 } 133 134 public Identifier addIdentifier() { //3 135 Identifier t = new Identifier(); 136 if (this.identifier == null) 137 this.identifier = new ArrayList<Identifier>(); 138 this.identifier.add(t); 139 return t; 140 } 141 142 public Basic addIdentifier(Identifier t) { //3 143 if (t == null) 144 return this; 145 if (this.identifier == null) 146 this.identifier = new ArrayList<Identifier>(); 147 this.identifier.add(t); 148 return this; 149 } 150 151 /** 152 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist {3} 153 */ 154 public Identifier getIdentifierFirstRep() { 155 if (getIdentifier().isEmpty()) { 156 addIdentifier(); 157 } 158 return getIdentifier().get(0); 159 } 160 161 /** 162 * @return {@link #code} (Identifies the 'type' of resource - equivalent to the resource name for other resources.) 163 */ 164 public CodeableConcept getCode() { 165 if (this.code == null) 166 if (Configuration.errorOnAutoCreate()) 167 throw new Error("Attempt to auto-create Basic.code"); 168 else if (Configuration.doAutoCreate()) 169 this.code = new CodeableConcept(); // cc 170 return this.code; 171 } 172 173 public boolean hasCode() { 174 return this.code != null && !this.code.isEmpty(); 175 } 176 177 /** 178 * @param value {@link #code} (Identifies the 'type' of resource - equivalent to the resource name for other resources.) 179 */ 180 public Basic setCode(CodeableConcept value) { 181 this.code = value; 182 return this; 183 } 184 185 /** 186 * @return {@link #subject} (Identifies the patient, practitioner, device or any other resource that is the "focus" of this resource.) 187 */ 188 public Reference getSubject() { 189 if (this.subject == null) 190 if (Configuration.errorOnAutoCreate()) 191 throw new Error("Attempt to auto-create Basic.subject"); 192 else if (Configuration.doAutoCreate()) 193 this.subject = new Reference(); // cc 194 return this.subject; 195 } 196 197 public boolean hasSubject() { 198 return this.subject != null && !this.subject.isEmpty(); 199 } 200 201 /** 202 * @param value {@link #subject} (Identifies the patient, practitioner, device or any other resource that is the "focus" of this resource.) 203 */ 204 public Basic setSubject(Reference value) { 205 this.subject = value; 206 return this; 207 } 208 209 /** 210 * @return {@link #created} (Identifies when the resource was first created.). This is the underlying object with id, value and extensions. The accessor "getCreated" gives direct access to the value 211 */ 212 public DateTimeType getCreatedElement() { 213 if (this.created == null) 214 if (Configuration.errorOnAutoCreate()) 215 throw new Error("Attempt to auto-create Basic.created"); 216 else if (Configuration.doAutoCreate()) 217 this.created = new DateTimeType(); // bb 218 return this.created; 219 } 220 221 public boolean hasCreatedElement() { 222 return this.created != null && !this.created.isEmpty(); 223 } 224 225 public boolean hasCreated() { 226 return this.created != null && !this.created.isEmpty(); 227 } 228 229 /** 230 * @param value {@link #created} (Identifies when the resource was first created.). This is the underlying object with id, value and extensions. The accessor "getCreated" gives direct access to the value 231 */ 232 public Basic setCreatedElement(DateTimeType value) { 233 this.created = value; 234 return this; 235 } 236 237 /** 238 * @return Identifies when the resource was first created. 239 */ 240 public Date getCreated() { 241 return this.created == null ? null : this.created.getValue(); 242 } 243 244 /** 245 * @param value Identifies when the resource was first created. 246 */ 247 public Basic setCreated(Date value) { 248 if (value == null) 249 this.created = null; 250 else { 251 if (this.created == null) 252 this.created = new DateTimeType(); 253 this.created.setValue(value); 254 } 255 return this; 256 } 257 258 /** 259 * @return {@link #author} (Indicates who was responsible for creating the resource instance.) 260 */ 261 public Reference getAuthor() { 262 if (this.author == null) 263 if (Configuration.errorOnAutoCreate()) 264 throw new Error("Attempt to auto-create Basic.author"); 265 else if (Configuration.doAutoCreate()) 266 this.author = new Reference(); // cc 267 return this.author; 268 } 269 270 public boolean hasAuthor() { 271 return this.author != null && !this.author.isEmpty(); 272 } 273 274 /** 275 * @param value {@link #author} (Indicates who was responsible for creating the resource instance.) 276 */ 277 public Basic setAuthor(Reference value) { 278 this.author = value; 279 return this; 280 } 281 282 protected void listChildren(List<Property> children) { 283 super.listChildren(children); 284 children.add(new Property("identifier", "Identifier", "Identifier assigned to the resource for business purposes, outside the context of FHIR.", 0, java.lang.Integer.MAX_VALUE, identifier)); 285 children.add(new Property("code", "CodeableConcept", "Identifies the 'type' of resource - equivalent to the resource name for other resources.", 0, 1, code)); 286 children.add(new Property("subject", "Reference(Any)", "Identifies the patient, practitioner, device or any other resource that is the \"focus\" of this resource.", 0, 1, subject)); 287 children.add(new Property("created", "dateTime", "Identifies when the resource was first created.", 0, 1, created)); 288 children.add(new Property("author", "Reference(Practitioner|PractitionerRole|Patient|RelatedPerson|Organization|Device|CareTeam)", "Indicates who was responsible for creating the resource instance.", 0, 1, author)); 289 } 290 291 @Override 292 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 293 switch (_hash) { 294 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifier assigned to the resource for business purposes, outside the context of FHIR.", 0, java.lang.Integer.MAX_VALUE, identifier); 295 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "Identifies the 'type' of resource - equivalent to the resource name for other resources.", 0, 1, code); 296 case -1867885268: /*subject*/ return new Property("subject", "Reference(Any)", "Identifies the patient, practitioner, device or any other resource that is the \"focus\" of this resource.", 0, 1, subject); 297 case 1028554472: /*created*/ return new Property("created", "dateTime", "Identifies when the resource was first created.", 0, 1, created); 298 case -1406328437: /*author*/ return new Property("author", "Reference(Practitioner|PractitionerRole|Patient|RelatedPerson|Organization|Device|CareTeam)", "Indicates who was responsible for creating the resource instance.", 0, 1, author); 299 default: return super.getNamedProperty(_hash, _name, _checkValid); 300 } 301 302 } 303 304 @Override 305 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 306 switch (hash) { 307 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 308 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 309 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 310 case 1028554472: /*created*/ return this.created == null ? new Base[0] : new Base[] {this.created}; // DateTimeType 311 case -1406328437: /*author*/ return this.author == null ? new Base[0] : new Base[] {this.author}; // Reference 312 default: return super.getProperty(hash, name, checkValid); 313 } 314 315 } 316 317 @Override 318 public Base setProperty(int hash, String name, Base value) throws FHIRException { 319 switch (hash) { 320 case -1618432855: // identifier 321 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); // Identifier 322 return value; 323 case 3059181: // code 324 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 325 return value; 326 case -1867885268: // subject 327 this.subject = TypeConvertor.castToReference(value); // Reference 328 return value; 329 case 1028554472: // created 330 this.created = TypeConvertor.castToDateTime(value); // DateTimeType 331 return value; 332 case -1406328437: // author 333 this.author = TypeConvertor.castToReference(value); // Reference 334 return value; 335 default: return super.setProperty(hash, name, value); 336 } 337 338 } 339 340 @Override 341 public Base setProperty(String name, Base value) throws FHIRException { 342 if (name.equals("identifier")) { 343 this.getIdentifier().add(TypeConvertor.castToIdentifier(value)); 344 } else if (name.equals("code")) { 345 this.code = TypeConvertor.castToCodeableConcept(value); // CodeableConcept 346 } else if (name.equals("subject")) { 347 this.subject = TypeConvertor.castToReference(value); // Reference 348 } else if (name.equals("created")) { 349 this.created = TypeConvertor.castToDateTime(value); // DateTimeType 350 } else if (name.equals("author")) { 351 this.author = TypeConvertor.castToReference(value); // Reference 352 } else 353 return super.setProperty(name, value); 354 return value; 355 } 356 357 @Override 358 public Base makeProperty(int hash, String name) throws FHIRException { 359 switch (hash) { 360 case -1618432855: return addIdentifier(); 361 case 3059181: return getCode(); 362 case -1867885268: return getSubject(); 363 case 1028554472: return getCreatedElement(); 364 case -1406328437: return getAuthor(); 365 default: return super.makeProperty(hash, name); 366 } 367 368 } 369 370 @Override 371 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 372 switch (hash) { 373 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 374 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 375 case -1867885268: /*subject*/ return new String[] {"Reference"}; 376 case 1028554472: /*created*/ return new String[] {"dateTime"}; 377 case -1406328437: /*author*/ return new String[] {"Reference"}; 378 default: return super.getTypesForProperty(hash, name); 379 } 380 381 } 382 383 @Override 384 public Base addChild(String name) throws FHIRException { 385 if (name.equals("identifier")) { 386 return addIdentifier(); 387 } 388 else if (name.equals("code")) { 389 this.code = new CodeableConcept(); 390 return this.code; 391 } 392 else if (name.equals("subject")) { 393 this.subject = new Reference(); 394 return this.subject; 395 } 396 else if (name.equals("created")) { 397 throw new FHIRException("Cannot call addChild on a singleton property Basic.created"); 398 } 399 else if (name.equals("author")) { 400 this.author = new Reference(); 401 return this.author; 402 } 403 else 404 return super.addChild(name); 405 } 406 407 public String fhirType() { 408 return "Basic"; 409 410 } 411 412 public Basic copy() { 413 Basic dst = new Basic(); 414 copyValues(dst); 415 return dst; 416 } 417 418 public void copyValues(Basic dst) { 419 super.copyValues(dst); 420 if (identifier != null) { 421 dst.identifier = new ArrayList<Identifier>(); 422 for (Identifier i : identifier) 423 dst.identifier.add(i.copy()); 424 }; 425 dst.code = code == null ? null : code.copy(); 426 dst.subject = subject == null ? null : subject.copy(); 427 dst.created = created == null ? null : created.copy(); 428 dst.author = author == null ? null : author.copy(); 429 } 430 431 protected Basic typedCopy() { 432 return copy(); 433 } 434 435 @Override 436 public boolean equalsDeep(Base other_) { 437 if (!super.equalsDeep(other_)) 438 return false; 439 if (!(other_ instanceof Basic)) 440 return false; 441 Basic o = (Basic) other_; 442 return compareDeep(identifier, o.identifier, true) && compareDeep(code, o.code, true) && compareDeep(subject, o.subject, true) 443 && compareDeep(created, o.created, true) && compareDeep(author, o.author, true); 444 } 445 446 @Override 447 public boolean equalsShallow(Base other_) { 448 if (!super.equalsShallow(other_)) 449 return false; 450 if (!(other_ instanceof Basic)) 451 return false; 452 Basic o = (Basic) other_; 453 return compareValues(created, o.created, true); 454 } 455 456 public boolean isEmpty() { 457 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, code, subject 458 , created, author); 459 } 460 461 @Override 462 public ResourceType getResourceType() { 463 return ResourceType.Basic; 464 } 465 466 /** 467 * Search parameter: <b>author</b> 468 * <p> 469 * Description: <b>Who created</b><br> 470 * Type: <b>reference</b><br> 471 * Path: <b>Basic.author</b><br> 472 * </p> 473 */ 474 @SearchParamDefinition(name="author", path="Basic.author", description="Who created", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for RelatedPerson") }, target={CareTeam.class, Device.class, Organization.class, Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class } ) 475 public static final String SP_AUTHOR = "author"; 476 /** 477 * <b>Fluent Client</b> search parameter constant for <b>author</b> 478 * <p> 479 * Description: <b>Who created</b><br> 480 * Type: <b>reference</b><br> 481 * Path: <b>Basic.author</b><br> 482 * </p> 483 */ 484 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam AUTHOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_AUTHOR); 485 486/** 487 * Constant for fluent queries to be used to add include statements. Specifies 488 * the path value of "<b>Basic:author</b>". 489 */ 490 public static final ca.uhn.fhir.model.api.Include INCLUDE_AUTHOR = new ca.uhn.fhir.model.api.Include("Basic:author").toLocked(); 491 492 /** 493 * Search parameter: <b>created</b> 494 * <p> 495 * Description: <b>When created</b><br> 496 * Type: <b>date</b><br> 497 * Path: <b>Basic.created</b><br> 498 * </p> 499 */ 500 @SearchParamDefinition(name="created", path="Basic.created", description="When created", type="date" ) 501 public static final String SP_CREATED = "created"; 502 /** 503 * <b>Fluent Client</b> search parameter constant for <b>created</b> 504 * <p> 505 * Description: <b>When created</b><br> 506 * Type: <b>date</b><br> 507 * Path: <b>Basic.created</b><br> 508 * </p> 509 */ 510 public static final ca.uhn.fhir.rest.gclient.DateClientParam CREATED = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_CREATED); 511 512 /** 513 * Search parameter: <b>subject</b> 514 * <p> 515 * Description: <b>Identifies the focus of this resource</b><br> 516 * Type: <b>reference</b><br> 517 * Path: <b>Basic.subject</b><br> 518 * </p> 519 */ 520 @SearchParamDefinition(name="subject", path="Basic.subject", description="Identifies the focus of this resource", type="reference", target={Account.class, ActivityDefinition.class, ActorDefinition.class, AdministrableProductDefinition.class, AdverseEvent.class, AllergyIntolerance.class, Appointment.class, AppointmentResponse.class, ArtifactAssessment.class, AuditEvent.class, Basic.class, Binary.class, BiologicallyDerivedProduct.class, BiologicallyDerivedProductDispense.class, BodyStructure.class, Bundle.class, CapabilityStatement.class, CarePlan.class, CareTeam.class, ChargeItem.class, ChargeItemDefinition.class, Citation.class, Claim.class, ClaimResponse.class, ClinicalImpression.class, ClinicalUseDefinition.class, CodeSystem.class, Communication.class, CommunicationRequest.class, CompartmentDefinition.class, Composition.class, ConceptMap.class, Condition.class, ConditionDefinition.class, Consent.class, Contract.class, Coverage.class, CoverageEligibilityRequest.class, CoverageEligibilityResponse.class, DetectedIssue.class, Device.class, DeviceAssociation.class, DeviceDefinition.class, DeviceDispense.class, DeviceMetric.class, DeviceRequest.class, DeviceUsage.class, DiagnosticReport.class, DocumentReference.class, Encounter.class, EncounterHistory.class, Endpoint.class, EnrollmentRequest.class, EnrollmentResponse.class, EpisodeOfCare.class, EventDefinition.class, Evidence.class, EvidenceReport.class, EvidenceVariable.class, ExampleScenario.class, ExplanationOfBenefit.class, FamilyMemberHistory.class, Flag.class, FormularyItem.class, GenomicStudy.class, Goal.class, GraphDefinition.class, Group.class, GuidanceResponse.class, HealthcareService.class, ImagingSelection.class, ImagingStudy.class, Immunization.class, ImmunizationEvaluation.class, ImmunizationRecommendation.class, ImplementationGuide.class, Ingredient.class, InsurancePlan.class, InventoryItem.class, InventoryReport.class, Invoice.class, Library.class, Linkage.class, ListResource.class, Location.class, ManufacturedItemDefinition.class, Measure.class, MeasureReport.class, Medication.class, MedicationAdministration.class, MedicationDispense.class, MedicationKnowledge.class, MedicationRequest.class, MedicationStatement.class, MedicinalProductDefinition.class, MessageDefinition.class, MessageHeader.class, MolecularSequence.class, NamingSystem.class, NutritionIntake.class, NutritionOrder.class, NutritionProduct.class, Observation.class, ObservationDefinition.class, OperationDefinition.class, OperationOutcome.class, Organization.class, OrganizationAffiliation.class, PackagedProductDefinition.class, Parameters.class, Patient.class, PaymentNotice.class, PaymentReconciliation.class, Permission.class, Person.class, PlanDefinition.class, Practitioner.class, PractitionerRole.class, Procedure.class, Provenance.class, Questionnaire.class, QuestionnaireResponse.class, RegulatedAuthorization.class, RelatedPerson.class, RequestOrchestration.class, Requirements.class, ResearchStudy.class, ResearchSubject.class, RiskAssessment.class, Schedule.class, SearchParameter.class, ServiceRequest.class, Slot.class, Specimen.class, SpecimenDefinition.class, StructureDefinition.class, StructureMap.class, Subscription.class, SubscriptionStatus.class, SubscriptionTopic.class, Substance.class, SubstanceDefinition.class, SubstanceNucleicAcid.class, SubstancePolymer.class, SubstanceProtein.class, SubstanceReferenceInformation.class, SubstanceSourceMaterial.class, SupplyDelivery.class, SupplyRequest.class, Task.class, TerminologyCapabilities.class, TestPlan.class, TestReport.class, TestScript.class, Transport.class, ValueSet.class, VerificationResult.class, VisionPrescription.class } ) 521 public static final String SP_SUBJECT = "subject"; 522 /** 523 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 524 * <p> 525 * Description: <b>Identifies the focus of this resource</b><br> 526 * Type: <b>reference</b><br> 527 * Path: <b>Basic.subject</b><br> 528 * </p> 529 */ 530 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 531 532/** 533 * Constant for fluent queries to be used to add include statements. Specifies 534 * the path value of "<b>Basic:subject</b>". 535 */ 536 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("Basic:subject").toLocked(); 537 538 /** 539 * Search parameter: <b>code</b> 540 * <p> 541 * Description: <b>Multiple Resources: 542 543* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted 544* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance 545* [AuditEvent](auditevent.html): More specific code for the event 546* [Basic](basic.html): Kind of Resource 547* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code 548* [Condition](condition.html): Code for the condition 549* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc. 550* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered 551* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result 552* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code 553* [ImagingSelection](imagingselection.html): The imaging selection status 554* [List](list.html): What the purpose of this list is 555* [Medication](medication.html): Returns medications for a specific code 556* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code 557* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code 558* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code 559* [MedicationStatement](medicationstatement.html): Return statements of this medication code 560* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake 561* [Observation](observation.html): The code of the observation type 562* [Procedure](procedure.html): A code to identify a procedure 563* [RequestOrchestration](requestorchestration.html): The code of the request orchestration 564* [Task](task.html): Search by task code 565</b><br> 566 * Type: <b>token</b><br> 567 * Path: <b>AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code</b><br> 568 * </p> 569 */ 570 @SearchParamDefinition(name="code", path="AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code", description="Multiple Resources: \r\n\r\n* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted\r\n* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance\r\n* [AuditEvent](auditevent.html): More specific code for the event\r\n* [Basic](basic.html): Kind of Resource\r\n* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code\r\n* [Condition](condition.html): Code for the condition\r\n* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc.\r\n* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered\r\n* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code\r\n* [ImagingSelection](imagingselection.html): The imaging selection status\r\n* [List](list.html): What the purpose of this list is\r\n* [Medication](medication.html): Returns medications for a specific code\r\n* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code\r\n* [MedicationStatement](medicationstatement.html): Return statements of this medication code\r\n* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake\r\n* [Observation](observation.html): The code of the observation type\r\n* [Procedure](procedure.html): A code to identify a procedure\r\n* [RequestOrchestration](requestorchestration.html): The code of the request orchestration\r\n* [Task](task.html): Search by task code\r\n", type="token" ) 571 public static final String SP_CODE = "code"; 572 /** 573 * <b>Fluent Client</b> search parameter constant for <b>code</b> 574 * <p> 575 * Description: <b>Multiple Resources: 576 577* [AdverseEvent](adverseevent.html): Event or incident that occurred or was averted 578* [AllergyIntolerance](allergyintolerance.html): Code that identifies the allergy or intolerance 579* [AuditEvent](auditevent.html): More specific code for the event 580* [Basic](basic.html): Kind of Resource 581* [ChargeItem](chargeitem.html): A code that identifies the charge, like a billing code 582* [Condition](condition.html): Code for the condition 583* [DetectedIssue](detectedissue.html): Issue Type, e.g. drug-drug, duplicate therapy, etc. 584* [DeviceRequest](devicerequest.html): Code for what is being requested/ordered 585* [DiagnosticReport](diagnosticreport.html): The code for the report, as opposed to codes for the atomic results, which are the names on the observation resource referred to from the result 586* [FamilyMemberHistory](familymemberhistory.html): A search by a condition code 587* [ImagingSelection](imagingselection.html): The imaging selection status 588* [List](list.html): What the purpose of this list is 589* [Medication](medication.html): Returns medications for a specific code 590* [MedicationAdministration](medicationadministration.html): Return administrations of this medication code 591* [MedicationDispense](medicationdispense.html): Returns dispenses of this medicine code 592* [MedicationRequest](medicationrequest.html): Return prescriptions of this medication code 593* [MedicationStatement](medicationstatement.html): Return statements of this medication code 594* [NutritionIntake](nutritionintake.html): Returns statements of this code of NutritionIntake 595* [Observation](observation.html): The code of the observation type 596* [Procedure](procedure.html): A code to identify a procedure 597* [RequestOrchestration](requestorchestration.html): The code of the request orchestration 598* [Task](task.html): Search by task code 599</b><br> 600 * Type: <b>token</b><br> 601 * Path: <b>AdverseEvent.code | AllergyIntolerance.code | AllergyIntolerance.reaction.substance | AuditEvent.code | Basic.code | ChargeItem.code | Condition.code | DetectedIssue.code | DeviceRequest.code.concept | DiagnosticReport.code | FamilyMemberHistory.condition.code | ImagingSelection.status | List.code | Medication.code | MedicationAdministration.medication.concept | MedicationDispense.medication.concept | MedicationRequest.medication.concept | MedicationStatement.medication.concept | NutritionIntake.code | Observation.code | Procedure.code | RequestOrchestration.code | Task.code</b><br> 602 * </p> 603 */ 604 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 605 606 /** 607 * Search parameter: <b>identifier</b> 608 * <p> 609 * Description: <b>Multiple Resources: 610 611* [Account](account.html): Account number 612* [AdverseEvent](adverseevent.html): Business identifier for the event 613* [AllergyIntolerance](allergyintolerance.html): External ids for this item 614* [Appointment](appointment.html): An Identifier of the Appointment 615* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 616* [Basic](basic.html): Business identifier 617* [BodyStructure](bodystructure.html): Bodystructure identifier 618* [CarePlan](careplan.html): External Ids for this plan 619* [CareTeam](careteam.html): External Ids for this team 620* [ChargeItem](chargeitem.html): Business Identifier for item 621* [Claim](claim.html): The primary identifier of the financial resource 622* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 623* [ClinicalImpression](clinicalimpression.html): Business identifier 624* [Communication](communication.html): Unique identifier 625* [CommunicationRequest](communicationrequest.html): Unique identifier 626* [Composition](composition.html): Version-independent identifier for the Composition 627* [Condition](condition.html): A unique identifier of the condition record 628* [Consent](consent.html): Identifier for this record (external references) 629* [Contract](contract.html): The identity of the contract 630* [Coverage](coverage.html): The primary identifier of the insured and the coverage 631* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 632* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 633* [DetectedIssue](detectedissue.html): Unique id for the detected issue 634* [DeviceRequest](devicerequest.html): Business identifier for request/order 635* [DeviceUsage](deviceusage.html): Search by identifier 636* [DiagnosticReport](diagnosticreport.html): An identifier for the report 637* [DocumentReference](documentreference.html): Identifier of the attachment binary 638* [Encounter](encounter.html): Identifier(s) by which this encounter is known 639* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 640* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 641* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 642* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 643* [Flag](flag.html): Business identifier 644* [Goal](goal.html): External Ids for this goal 645* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 646* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 647* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 648* [Immunization](immunization.html): Business identifier 649* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 650* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 651* [Invoice](invoice.html): Business Identifier for item 652* [List](list.html): Business identifier 653* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 654* [Medication](medication.html): Returns medications with this external identifier 655* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 656* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 657* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 658* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 659* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 660* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 661* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 662* [Observation](observation.html): The unique id for a particular observation 663* [Person](person.html): A person Identifier 664* [Procedure](procedure.html): A unique identifier for a procedure 665* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 666* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 667* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 668* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 669* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 670* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 671* [Specimen](specimen.html): The unique identifier associated with the specimen 672* [SupplyDelivery](supplydelivery.html): External identifier 673* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 674* [Task](task.html): Search for a task instance by its business identifier 675* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 676</b><br> 677 * Type: <b>token</b><br> 678 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 679 * </p> 680 */ 681 @SearchParamDefinition(name="identifier", path="Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier", description="Multiple Resources: \r\n\r\n* [Account](account.html): Account number\r\n* [AdverseEvent](adverseevent.html): Business identifier for the event\r\n* [AllergyIntolerance](allergyintolerance.html): External ids for this item\r\n* [Appointment](appointment.html): An Identifier of the Appointment\r\n* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response\r\n* [Basic](basic.html): Business identifier\r\n* [BodyStructure](bodystructure.html): Bodystructure identifier\r\n* [CarePlan](careplan.html): External Ids for this plan\r\n* [CareTeam](careteam.html): External Ids for this team\r\n* [ChargeItem](chargeitem.html): Business Identifier for item\r\n* [Claim](claim.html): The primary identifier of the financial resource\r\n* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse\r\n* [ClinicalImpression](clinicalimpression.html): Business identifier\r\n* [Communication](communication.html): Unique identifier\r\n* [CommunicationRequest](communicationrequest.html): Unique identifier\r\n* [Composition](composition.html): Version-independent identifier for the Composition\r\n* [Condition](condition.html): A unique identifier of the condition record\r\n* [Consent](consent.html): Identifier for this record (external references)\r\n* [Contract](contract.html): The identity of the contract\r\n* [Coverage](coverage.html): The primary identifier of the insured and the coverage\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier\r\n* [DetectedIssue](detectedissue.html): Unique id for the detected issue\r\n* [DeviceRequest](devicerequest.html): Business identifier for request/order\r\n* [DeviceUsage](deviceusage.html): Search by identifier\r\n* [DiagnosticReport](diagnosticreport.html): An identifier for the report\r\n* [DocumentReference](documentreference.html): Identifier of the attachment binary\r\n* [Encounter](encounter.html): Identifier(s) by which this encounter is known\r\n* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment\r\n* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit\r\n* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier\r\n* [Flag](flag.html): Business identifier\r\n* [Goal](goal.html): External Ids for this goal\r\n* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response\r\n* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection\r\n* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID\r\n* [Immunization](immunization.html): Business identifier\r\n* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier\r\n* [Invoice](invoice.html): Business Identifier for item\r\n* [List](list.html): Business identifier\r\n* [MeasureReport](measurereport.html): External identifier of the measure report to be returned\r\n* [Medication](medication.html): Returns medications with this external identifier\r\n* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier\r\n* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier\r\n* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier\r\n* [MedicationStatement](medicationstatement.html): Return statements with this external identifier\r\n* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence\r\n* [NutritionIntake](nutritionintake.html): Return statements with this external identifier\r\n* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier\r\n* [Observation](observation.html): The unique id for a particular observation\r\n* [Person](person.html): A person Identifier\r\n* [Procedure](procedure.html): A unique identifier for a procedure\r\n* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response\r\n* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson\r\n* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration\r\n* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study\r\n* [RiskAssessment](riskassessment.html): Unique identifier for the assessment\r\n* [ServiceRequest](servicerequest.html): Identifiers assigned to this order\r\n* [Specimen](specimen.html): The unique identifier associated with the specimen\r\n* [SupplyDelivery](supplydelivery.html): External identifier\r\n* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest\r\n* [Task](task.html): Search for a task instance by its business identifier\r\n* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier\r\n", type="token" ) 682 public static final String SP_IDENTIFIER = "identifier"; 683 /** 684 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 685 * <p> 686 * Description: <b>Multiple Resources: 687 688* [Account](account.html): Account number 689* [AdverseEvent](adverseevent.html): Business identifier for the event 690* [AllergyIntolerance](allergyintolerance.html): External ids for this item 691* [Appointment](appointment.html): An Identifier of the Appointment 692* [AppointmentResponse](appointmentresponse.html): An Identifier in this appointment response 693* [Basic](basic.html): Business identifier 694* [BodyStructure](bodystructure.html): Bodystructure identifier 695* [CarePlan](careplan.html): External Ids for this plan 696* [CareTeam](careteam.html): External Ids for this team 697* [ChargeItem](chargeitem.html): Business Identifier for item 698* [Claim](claim.html): The primary identifier of the financial resource 699* [ClaimResponse](claimresponse.html): The identity of the ClaimResponse 700* [ClinicalImpression](clinicalimpression.html): Business identifier 701* [Communication](communication.html): Unique identifier 702* [CommunicationRequest](communicationrequest.html): Unique identifier 703* [Composition](composition.html): Version-independent identifier for the Composition 704* [Condition](condition.html): A unique identifier of the condition record 705* [Consent](consent.html): Identifier for this record (external references) 706* [Contract](contract.html): The identity of the contract 707* [Coverage](coverage.html): The primary identifier of the insured and the coverage 708* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The business identifier of the Eligibility 709* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The business identifier 710* [DetectedIssue](detectedissue.html): Unique id for the detected issue 711* [DeviceRequest](devicerequest.html): Business identifier for request/order 712* [DeviceUsage](deviceusage.html): Search by identifier 713* [DiagnosticReport](diagnosticreport.html): An identifier for the report 714* [DocumentReference](documentreference.html): Identifier of the attachment binary 715* [Encounter](encounter.html): Identifier(s) by which this encounter is known 716* [EnrollmentRequest](enrollmentrequest.html): The business identifier of the Enrollment 717* [EpisodeOfCare](episodeofcare.html): Business Identifier(s) relevant for this EpisodeOfCare 718* [ExplanationOfBenefit](explanationofbenefit.html): The business identifier of the Explanation of Benefit 719* [FamilyMemberHistory](familymemberhistory.html): A search by a record identifier 720* [Flag](flag.html): Business identifier 721* [Goal](goal.html): External Ids for this goal 722* [GuidanceResponse](guidanceresponse.html): The identifier of the guidance response 723* [ImagingSelection](imagingselection.html): Identifiers for the imaging selection 724* [ImagingStudy](imagingstudy.html): Identifiers for the Study, such as DICOM Study Instance UID 725* [Immunization](immunization.html): Business identifier 726* [ImmunizationEvaluation](immunizationevaluation.html): ID of the evaluation 727* [ImmunizationRecommendation](immunizationrecommendation.html): Business identifier 728* [Invoice](invoice.html): Business Identifier for item 729* [List](list.html): Business identifier 730* [MeasureReport](measurereport.html): External identifier of the measure report to be returned 731* [Medication](medication.html): Returns medications with this external identifier 732* [MedicationAdministration](medicationadministration.html): Return administrations with this external identifier 733* [MedicationDispense](medicationdispense.html): Returns dispenses with this external identifier 734* [MedicationRequest](medicationrequest.html): Return prescriptions with this external identifier 735* [MedicationStatement](medicationstatement.html): Return statements with this external identifier 736* [MolecularSequence](molecularsequence.html): The unique identity for a particular sequence 737* [NutritionIntake](nutritionintake.html): Return statements with this external identifier 738* [NutritionOrder](nutritionorder.html): Return nutrition orders with this external identifier 739* [Observation](observation.html): The unique id for a particular observation 740* [Person](person.html): A person Identifier 741* [Procedure](procedure.html): A unique identifier for a procedure 742* [QuestionnaireResponse](questionnaireresponse.html): The unique identifier for the questionnaire response 743* [RelatedPerson](relatedperson.html): An Identifier of the RelatedPerson 744* [RequestOrchestration](requestorchestration.html): External identifiers for the request orchestration 745* [ResearchSubject](researchsubject.html): Business Identifier for research subject in a study 746* [RiskAssessment](riskassessment.html): Unique identifier for the assessment 747* [ServiceRequest](servicerequest.html): Identifiers assigned to this order 748* [Specimen](specimen.html): The unique identifier associated with the specimen 749* [SupplyDelivery](supplydelivery.html): External identifier 750* [SupplyRequest](supplyrequest.html): Business Identifier for SupplyRequest 751* [Task](task.html): Search for a task instance by its business identifier 752* [VisionPrescription](visionprescription.html): Return prescriptions with this external identifier 753</b><br> 754 * Type: <b>token</b><br> 755 * Path: <b>Account.identifier | AdverseEvent.identifier | AllergyIntolerance.identifier | Appointment.identifier | AppointmentResponse.identifier | Basic.identifier | BodyStructure.identifier | CarePlan.identifier | CareTeam.identifier | ChargeItem.identifier | Claim.identifier | ClaimResponse.identifier | ClinicalImpression.identifier | Communication.identifier | CommunicationRequest.identifier | Composition.identifier | Condition.identifier | Consent.identifier | Contract.identifier | Coverage.identifier | CoverageEligibilityRequest.identifier | CoverageEligibilityResponse.identifier | DetectedIssue.identifier | DeviceRequest.identifier | DeviceUsage.identifier | DiagnosticReport.identifier | DocumentReference.identifier | Encounter.identifier | EnrollmentRequest.identifier | EpisodeOfCare.identifier | ExplanationOfBenefit.identifier | FamilyMemberHistory.identifier | Flag.identifier | Goal.identifier | GuidanceResponse.identifier | ImagingSelection.identifier | ImagingStudy.identifier | Immunization.identifier | ImmunizationEvaluation.identifier | ImmunizationRecommendation.identifier | Invoice.identifier | List.identifier | MeasureReport.identifier | Medication.identifier | MedicationAdministration.identifier | MedicationDispense.identifier | MedicationRequest.identifier | MedicationStatement.identifier | MolecularSequence.identifier | NutritionIntake.identifier | NutritionOrder.identifier | Observation.identifier | Person.identifier | Procedure.identifier | QuestionnaireResponse.identifier | RelatedPerson.identifier | RequestOrchestration.identifier | ResearchSubject.identifier | RiskAssessment.identifier | ServiceRequest.identifier | Specimen.identifier | SupplyDelivery.identifier | SupplyRequest.identifier | Task.identifier | VisionPrescription.identifier</b><br> 756 * </p> 757 */ 758 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 759 760 /** 761 * Search parameter: <b>patient</b> 762 * <p> 763 * Description: <b>Multiple Resources: 764 765* [Account](account.html): The entity that caused the expenses 766* [AdverseEvent](adverseevent.html): Subject impacted by event 767* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 768* [Appointment](appointment.html): One of the individuals of the appointment is this patient 769* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 770* [AuditEvent](auditevent.html): Where the activity involved patient data 771* [Basic](basic.html): Identifies the focus of this resource 772* [BodyStructure](bodystructure.html): Who this is about 773* [CarePlan](careplan.html): Who the care plan is for 774* [CareTeam](careteam.html): Who care team is for 775* [ChargeItem](chargeitem.html): Individual service was done for/to 776* [Claim](claim.html): Patient receiving the products or services 777* [ClaimResponse](claimresponse.html): The subject of care 778* [ClinicalImpression](clinicalimpression.html): Patient assessed 779* [Communication](communication.html): Focus of message 780* [CommunicationRequest](communicationrequest.html): Focus of message 781* [Composition](composition.html): Who and/or what the composition is about 782* [Condition](condition.html): Who has the condition? 783* [Consent](consent.html): Who the consent applies to 784* [Contract](contract.html): The identity of the subject of the contract (if a patient) 785* [Coverage](coverage.html): Retrieve coverages for a patient 786* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 787* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 788* [DetectedIssue](detectedissue.html): Associated patient 789* [DeviceRequest](devicerequest.html): Individual the service is ordered for 790* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 791* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 792* [DocumentReference](documentreference.html): Who/what is the subject of the document 793* [Encounter](encounter.html): The patient present at the encounter 794* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 795* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 796* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 797* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 798* [Flag](flag.html): The identity of a subject to list flags for 799* [Goal](goal.html): Who this goal is intended for 800* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 801* [ImagingSelection](imagingselection.html): Who the study is about 802* [ImagingStudy](imagingstudy.html): Who the study is about 803* [Immunization](immunization.html): The patient for the vaccination record 804* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 805* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 806* [Invoice](invoice.html): Recipient(s) of goods and services 807* [List](list.html): If all resources have the same subject 808* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 809* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 810* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 811* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 812* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 813* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 814* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 815* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 816* [Observation](observation.html): The subject that the observation is about (if patient) 817* [Person](person.html): The Person links to this Patient 818* [Procedure](procedure.html): Search by subject - a patient 819* [Provenance](provenance.html): Where the activity involved patient data 820* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 821* [RelatedPerson](relatedperson.html): The patient this related person is related to 822* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 823* [ResearchSubject](researchsubject.html): Who or what is part of study 824* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 825* [ServiceRequest](servicerequest.html): Search by subject - a patient 826* [Specimen](specimen.html): The patient the specimen comes from 827* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 828* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 829* [Task](task.html): Search by patient 830* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 831</b><br> 832 * Type: <b>reference</b><br> 833 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 834 * </p> 835 */ 836 @SearchParamDefinition(name="patient", path="Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient", description="Multiple Resources: \r\n\r\n* [Account](account.html): The entity that caused the expenses\r\n* [AdverseEvent](adverseevent.html): Subject impacted by event\r\n* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for\r\n* [Appointment](appointment.html): One of the individuals of the appointment is this patient\r\n* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient\r\n* [AuditEvent](auditevent.html): Where the activity involved patient data\r\n* [Basic](basic.html): Identifies the focus of this resource\r\n* [BodyStructure](bodystructure.html): Who this is about\r\n* [CarePlan](careplan.html): Who the care plan is for\r\n* [CareTeam](careteam.html): Who care team is for\r\n* [ChargeItem](chargeitem.html): Individual service was done for/to\r\n* [Claim](claim.html): Patient receiving the products or services\r\n* [ClaimResponse](claimresponse.html): The subject of care\r\n* [ClinicalImpression](clinicalimpression.html): Patient assessed\r\n* [Communication](communication.html): Focus of message\r\n* [CommunicationRequest](communicationrequest.html): Focus of message\r\n* [Composition](composition.html): Who and/or what the composition is about\r\n* [Condition](condition.html): Who has the condition?\r\n* [Consent](consent.html): Who the consent applies to\r\n* [Contract](contract.html): The identity of the subject of the contract (if a patient)\r\n* [Coverage](coverage.html): Retrieve coverages for a patient\r\n* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient\r\n* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient\r\n* [DetectedIssue](detectedissue.html): Associated patient\r\n* [DeviceRequest](devicerequest.html): Individual the service is ordered for\r\n* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device\r\n* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient\r\n* [DocumentReference](documentreference.html): Who/what is the subject of the document\r\n* [Encounter](encounter.html): The patient present at the encounter\r\n* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled\r\n* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care\r\n* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient\r\n* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for\r\n* [Flag](flag.html): The identity of a subject to list flags for\r\n* [Goal](goal.html): Who this goal is intended for\r\n* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results\r\n* [ImagingSelection](imagingselection.html): Who the study is about\r\n* [ImagingStudy](imagingstudy.html): Who the study is about\r\n* [Immunization](immunization.html): The patient for the vaccination record\r\n* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated\r\n* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for\r\n* [Invoice](invoice.html): Recipient(s) of goods and services\r\n* [List](list.html): If all resources have the same subject\r\n* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for\r\n* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for\r\n* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for\r\n* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient\r\n* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient.\r\n* [MolecularSequence](molecularsequence.html): The subject that the sequence is about\r\n* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient.\r\n* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement\r\n* [Observation](observation.html): The subject that the observation is about (if patient)\r\n* [Person](person.html): The Person links to this Patient\r\n* [Procedure](procedure.html): Search by subject - a patient\r\n* [Provenance](provenance.html): Where the activity involved patient data\r\n* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response\r\n* [RelatedPerson](relatedperson.html): The patient this related person is related to\r\n* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations\r\n* [ResearchSubject](researchsubject.html): Who or what is part of study\r\n* [RiskAssessment](riskassessment.html): Who/what does assessment apply to?\r\n* [ServiceRequest](servicerequest.html): Search by subject - a patient\r\n* [Specimen](specimen.html): The patient the specimen comes from\r\n* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied\r\n* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined\r\n* [Task](task.html): Search by patient\r\n* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for\r\n", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Patient.class } ) 837 public static final String SP_PATIENT = "patient"; 838 /** 839 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 840 * <p> 841 * Description: <b>Multiple Resources: 842 843* [Account](account.html): The entity that caused the expenses 844* [AdverseEvent](adverseevent.html): Subject impacted by event 845* [AllergyIntolerance](allergyintolerance.html): Who the sensitivity is for 846* [Appointment](appointment.html): One of the individuals of the appointment is this patient 847* [AppointmentResponse](appointmentresponse.html): This Response is for this Patient 848* [AuditEvent](auditevent.html): Where the activity involved patient data 849* [Basic](basic.html): Identifies the focus of this resource 850* [BodyStructure](bodystructure.html): Who this is about 851* [CarePlan](careplan.html): Who the care plan is for 852* [CareTeam](careteam.html): Who care team is for 853* [ChargeItem](chargeitem.html): Individual service was done for/to 854* [Claim](claim.html): Patient receiving the products or services 855* [ClaimResponse](claimresponse.html): The subject of care 856* [ClinicalImpression](clinicalimpression.html): Patient assessed 857* [Communication](communication.html): Focus of message 858* [CommunicationRequest](communicationrequest.html): Focus of message 859* [Composition](composition.html): Who and/or what the composition is about 860* [Condition](condition.html): Who has the condition? 861* [Consent](consent.html): Who the consent applies to 862* [Contract](contract.html): The identity of the subject of the contract (if a patient) 863* [Coverage](coverage.html): Retrieve coverages for a patient 864* [CoverageEligibilityRequest](coverageeligibilityrequest.html): The reference to the patient 865* [CoverageEligibilityResponse](coverageeligibilityresponse.html): The reference to the patient 866* [DetectedIssue](detectedissue.html): Associated patient 867* [DeviceRequest](devicerequest.html): Individual the service is ordered for 868* [DeviceUsage](deviceusage.html): Search by patient who used / uses the device 869* [DiagnosticReport](diagnosticreport.html): The subject of the report if a patient 870* [DocumentReference](documentreference.html): Who/what is the subject of the document 871* [Encounter](encounter.html): The patient present at the encounter 872* [EnrollmentRequest](enrollmentrequest.html): The party to be enrolled 873* [EpisodeOfCare](episodeofcare.html): The patient who is the focus of this episode of care 874* [ExplanationOfBenefit](explanationofbenefit.html): The reference to the patient 875* [FamilyMemberHistory](familymemberhistory.html): The identity of a subject to list family member history items for 876* [Flag](flag.html): The identity of a subject to list flags for 877* [Goal](goal.html): Who this goal is intended for 878* [GuidanceResponse](guidanceresponse.html): The identity of a patient to search for guidance response results 879* [ImagingSelection](imagingselection.html): Who the study is about 880* [ImagingStudy](imagingstudy.html): Who the study is about 881* [Immunization](immunization.html): The patient for the vaccination record 882* [ImmunizationEvaluation](immunizationevaluation.html): The patient being evaluated 883* [ImmunizationRecommendation](immunizationrecommendation.html): Who this profile is for 884* [Invoice](invoice.html): Recipient(s) of goods and services 885* [List](list.html): If all resources have the same subject 886* [MeasureReport](measurereport.html): The identity of a patient to search for individual measure report results for 887* [MedicationAdministration](medicationadministration.html): The identity of a patient to list administrations for 888* [MedicationDispense](medicationdispense.html): The identity of a patient to list dispenses for 889* [MedicationRequest](medicationrequest.html): Returns prescriptions for a specific patient 890* [MedicationStatement](medicationstatement.html): Returns statements for a specific patient. 891* [MolecularSequence](molecularsequence.html): The subject that the sequence is about 892* [NutritionIntake](nutritionintake.html): Returns statements for a specific patient. 893* [NutritionOrder](nutritionorder.html): The identity of the individual or set of individuals who requires the diet, formula or nutritional supplement 894* [Observation](observation.html): The subject that the observation is about (if patient) 895* [Person](person.html): The Person links to this Patient 896* [Procedure](procedure.html): Search by subject - a patient 897* [Provenance](provenance.html): Where the activity involved patient data 898* [QuestionnaireResponse](questionnaireresponse.html): The patient that is the subject of the questionnaire response 899* [RelatedPerson](relatedperson.html): The patient this related person is related to 900* [RequestOrchestration](requestorchestration.html): The identity of a patient to search for request orchestrations 901* [ResearchSubject](researchsubject.html): Who or what is part of study 902* [RiskAssessment](riskassessment.html): Who/what does assessment apply to? 903* [ServiceRequest](servicerequest.html): Search by subject - a patient 904* [Specimen](specimen.html): The patient the specimen comes from 905* [SupplyDelivery](supplydelivery.html): Patient for whom the item is supplied 906* [SupplyRequest](supplyrequest.html): The patient or subject for whom the supply is destined 907* [Task](task.html): Search by patient 908* [VisionPrescription](visionprescription.html): The identity of a patient to list dispenses for 909</b><br> 910 * Type: <b>reference</b><br> 911 * Path: <b>Account.subject.where(resolve() is Patient) | AdverseEvent.subject.where(resolve() is Patient) | AllergyIntolerance.patient | Appointment.participant.actor.where(resolve() is Patient) | Appointment.subject.where(resolve() is Patient) | AppointmentResponse.actor.where(resolve() is Patient) | AuditEvent.patient | Basic.subject.where(resolve() is Patient) | BodyStructure.patient | CarePlan.subject.where(resolve() is Patient) | CareTeam.subject.where(resolve() is Patient) | ChargeItem.subject.where(resolve() is Patient) | Claim.patient | ClaimResponse.patient | ClinicalImpression.subject.where(resolve() is Patient) | Communication.subject.where(resolve() is Patient) | CommunicationRequest.subject.where(resolve() is Patient) | Composition.subject.where(resolve() is Patient) | Condition.subject.where(resolve() is Patient) | Consent.subject.where(resolve() is Patient) | Contract.subject.where(resolve() is Patient) | Coverage.beneficiary | CoverageEligibilityRequest.patient | CoverageEligibilityResponse.patient | DetectedIssue.subject.where(resolve() is Patient) | DeviceRequest.subject.where(resolve() is Patient) | DeviceUsage.patient | DiagnosticReport.subject.where(resolve() is Patient) | DocumentReference.subject.where(resolve() is Patient) | Encounter.subject.where(resolve() is Patient) | EnrollmentRequest.candidate | EpisodeOfCare.patient | ExplanationOfBenefit.patient | FamilyMemberHistory.patient | Flag.subject.where(resolve() is Patient) | Goal.subject.where(resolve() is Patient) | GuidanceResponse.subject.where(resolve() is Patient) | ImagingSelection.subject.where(resolve() is Patient) | ImagingStudy.subject.where(resolve() is Patient) | Immunization.patient | ImmunizationEvaluation.patient | ImmunizationRecommendation.patient | Invoice.subject.where(resolve() is Patient) | List.subject.where(resolve() is Patient) | MeasureReport.subject.where(resolve() is Patient) | MedicationAdministration.subject.where(resolve() is Patient) | MedicationDispense.subject.where(resolve() is Patient) | MedicationRequest.subject.where(resolve() is Patient) | MedicationStatement.subject.where(resolve() is Patient) | MolecularSequence.subject.where(resolve() is Patient) | NutritionIntake.subject.where(resolve() is Patient) | NutritionOrder.subject.where(resolve() is Patient) | Observation.subject.where(resolve() is Patient) | Person.link.target.where(resolve() is Patient) | Procedure.subject.where(resolve() is Patient) | Provenance.patient | QuestionnaireResponse.subject.where(resolve() is Patient) | RelatedPerson.patient | RequestOrchestration.subject.where(resolve() is Patient) | ResearchSubject.subject.where(resolve() is Patient) | RiskAssessment.subject.where(resolve() is Patient) | ServiceRequest.subject.where(resolve() is Patient) | Specimen.subject.where(resolve() is Patient) | SupplyDelivery.patient | SupplyRequest.deliverFor | Task.for.where(resolve() is Patient) | VisionPrescription.patient</b><br> 912 * </p> 913 */ 914 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 915 916/** 917 * Constant for fluent queries to be used to add include statements. Specifies 918 * the path value of "<b>Basic:patient</b>". 919 */ 920 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("Basic:patient").toLocked(); 921 922 923} 924