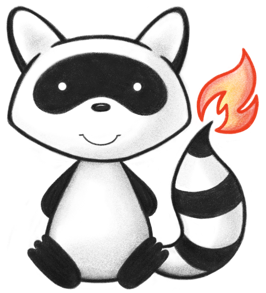
001package org.hl7.fhir.r5.model; 002 003 004/* 005 Copyright (c) 2011+, HL7, Inc. 006 All rights reserved. 007 008 Redistribution and use in source and binary forms, with or without modification, \ 009 are permitted provided that the following conditions are met: 010 011 * Redistributions of source code must retain the above copyright notice, this \ 012 list of conditions and the following disclaimer. 013 * Redistributions in binary form must reproduce the above copyright notice, \ 014 this list of conditions and the following disclaimer in the documentation \ 015 and/or other materials provided with the distribution. 016 * Neither the name of HL7 nor the names of its contributors may be used to 017 endorse or promote products derived from this software without specific 018 prior written permission. 019 020 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND \ 021 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED \ 022 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. \ 023 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, \ 024 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT \ 025 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR \ 026 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, \ 027 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) \ 028 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE \ 029 POSSIBILITY OF SUCH DAMAGE. 030 */ 031 032// Generated on Thu, Mar 23, 2023 19:59+1100 for FHIR v5.0.0 033 034import java.util.ArrayList; 035import java.util.Date; 036import java.util.List; 037import org.hl7.fhir.r5.model.Enumerations.*; 038import org.hl7.fhir.instance.model.api.IBaseDatatypeElement; 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.ICompositeType; 041import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.ChildOrder; 044import ca.uhn.fhir.model.api.annotation.DatatypeDef; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.Block; 047 048/** 049 * BackboneType Type: Base definition for the few data types that are allowed to carry modifier extensions. 050 */ 051@DatatypeDef(name="BackboneType") 052public abstract class BackboneType extends DataType implements IBaseBackboneElement { 053 054 /** 055 * May be used to represent additional information that is not part of the basic definition of the element and that modifies the understanding of the element in which it is contained and/or the understanding of the containing element's descendants. Usually modifier elements provide negation or qualification. To make the use of extensions safe and managable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer can define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension. Applications processing a resource are required to check for modifier extensions. 056 057Modifier extensions SHALL NOT change the meaning of any elements on Resource or DomainResource (including cannot change the meaning of modifierExtension itself). 058 */ 059 @Child(name = "modifierExtension", type = {Extension.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=true, summary=true) 060 @Description(shortDefinition="Extensions that cannot be ignored even if unrecognized", formalDefinition="May be used to represent additional information that is not part of the basic definition of the element and that modifies the understanding of the element in which it is contained and/or the understanding of the containing element's descendants. Usually modifier elements provide negation or qualification. To make the use of extensions safe and managable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer can define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension. Applications processing a resource are required to check for modifier extensions.\n\nModifier extensions SHALL NOT change the meaning of any elements on Resource or DomainResource (including cannot change the meaning of modifierExtension itself)." ) 061 protected List<Extension> modifierExtension; 062 063 private static final long serialVersionUID = -1431673179L; 064 065 /** 066 * Constructor 067 */ 068 public BackboneType() { 069 super(); 070 } 071 072 /** 073 * @return {@link #modifierExtension} (May be used to represent additional information that is not part of the basic definition of the element and that modifies the understanding of the element in which it is contained and/or the understanding of the containing element's descendants. Usually modifier elements provide negation or qualification. To make the use of extensions safe and managable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer can define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension. Applications processing a resource are required to check for modifier extensions. 074 075Modifier extensions SHALL NOT change the meaning of any elements on Resource or DomainResource (including cannot change the meaning of modifierExtension itself).) 076 */ 077 public List<Extension> getModifierExtension() { 078 if (this.modifierExtension == null) 079 this.modifierExtension = new ArrayList<Extension>(); 080 return this.modifierExtension; 081 } 082 083 /** 084 * @return Returns a reference to <code>this</code> for easy method chaining 085 */ 086 public BackboneType setModifierExtension(List<Extension> theModifierExtension) { 087 this.modifierExtension = theModifierExtension; 088 return this; 089 } 090 091 public boolean hasModifierExtension() { 092 if (this.modifierExtension == null) 093 return false; 094 for (Extension item : this.modifierExtension) 095 if (!item.isEmpty()) 096 return true; 097 return false; 098 } 099 100 public Extension addModifierExtension() { //3 101 Extension t = new Extension(); 102 if (this.modifierExtension == null) 103 this.modifierExtension = new ArrayList<Extension>(); 104 this.modifierExtension.add(t); 105 return t; 106 } 107 108 public BackboneType addModifierExtension(Extension t) { //3 109 if (t == null) 110 return this; 111 if (this.modifierExtension == null) 112 this.modifierExtension = new ArrayList<Extension>(); 113 this.modifierExtension.add(t); 114 return this; 115 } 116 117 /** 118 * @return The first repetition of repeating field {@link #modifierExtension}, creating it if it does not already exist {3} 119 */ 120 public Extension getModifierExtensionFirstRep() { 121 if (getModifierExtension().isEmpty()) { 122 addModifierExtension(); 123 } 124 return getModifierExtension().get(0); 125 } 126 127 protected void listChildren(List<Property> children) { 128 super.listChildren(children); 129 children.add(new Property("modifierExtension", "Extension", "May be used to represent additional information that is not part of the basic definition of the element and that modifies the understanding of the element in which it is contained and/or the understanding of the containing element's descendants. Usually modifier elements provide negation or qualification. To make the use of extensions safe and managable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer can define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension. Applications processing a resource are required to check for modifier extensions.\n\nModifier extensions SHALL NOT change the meaning of any elements on Resource or DomainResource (including cannot change the meaning of modifierExtension itself).", 0, java.lang.Integer.MAX_VALUE, modifierExtension)); 130 } 131 132 @Override 133 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 134 switch (_hash) { 135 case -298878168: /*modifierExtension*/ return new Property("modifierExtension", "Extension", "May be used to represent additional information that is not part of the basic definition of the element and that modifies the understanding of the element in which it is contained and/or the understanding of the containing element's descendants. Usually modifier elements provide negation or qualification. To make the use of extensions safe and managable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer can define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension. Applications processing a resource are required to check for modifier extensions.\n\nModifier extensions SHALL NOT change the meaning of any elements on Resource or DomainResource (including cannot change the meaning of modifierExtension itself).", 0, java.lang.Integer.MAX_VALUE, modifierExtension); 136 default: return super.getNamedProperty(_hash, _name, _checkValid); 137 } 138 139 } 140 141 @Override 142 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 143 switch (hash) { 144 case -298878168: /*modifierExtension*/ return this.modifierExtension == null ? new Base[0] : this.modifierExtension.toArray(new Base[this.modifierExtension.size()]); // Extension 145 default: return super.getProperty(hash, name, checkValid); 146 } 147 148 } 149 150 @Override 151 public Base setProperty(int hash, String name, Base value) throws FHIRException { 152 switch (hash) { 153 case -298878168: // modifierExtension 154 this.getModifierExtension().add(TypeConvertor.castToExtension(value)); // Extension 155 return value; 156 default: return super.setProperty(hash, name, value); 157 } 158 159 } 160 161 @Override 162 public Base setProperty(String name, Base value) throws FHIRException { 163 if (name.equals("modifierExtension")) { 164 this.getModifierExtension().add(TypeConvertor.castToExtension(value)); 165 } else 166 return super.setProperty(name, value); 167 return value; 168 } 169 170 @Override 171 public Base makeProperty(int hash, String name) throws FHIRException { 172 switch (hash) { 173 case -298878168: return addModifierExtension(); 174 default: return super.makeProperty(hash, name); 175 } 176 177 } 178 179 @Override 180 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 181 switch (hash) { 182 case -298878168: /*modifierExtension*/ return new String[] {"Extension"}; 183 default: return super.getTypesForProperty(hash, name); 184 } 185 186 } 187 188 @Override 189 public Base addChild(String name) throws FHIRException { 190 if (name.equals("modifierExtension")) { 191 return addModifierExtension(); 192 } 193 else 194 return super.addChild(name); 195 } 196 197 public String fhirType() { 198 return "BackboneType"; 199 200 } 201 202 public abstract BackboneType copy(); 203 204 public void copyValues(BackboneType dst) { 205 super.copyValues(dst); 206 if (modifierExtension != null) { 207 dst.modifierExtension = new ArrayList<Extension>(); 208 for (Extension i : modifierExtension) 209 dst.modifierExtension.add(i.copy()); 210 }; 211 } 212 213 @Override 214 public boolean equalsDeep(Base other_) { 215 if (!super.equalsDeep(other_)) 216 return false; 217 if (!(other_ instanceof BackboneType)) 218 return false; 219 BackboneType o = (BackboneType) other_; 220 return compareDeep(modifierExtension, o.modifierExtension, true); 221 } 222 223 @Override 224 public boolean equalsShallow(Base other_) { 225 if (!super.equalsShallow(other_)) 226 return false; 227 if (!(other_ instanceof BackboneType)) 228 return false; 229 BackboneType o = (BackboneType) other_; 230 return true; 231 } 232 233 public boolean isEmpty() { 234 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(modifierExtension); 235 } 236 237// Manual code (from Configuration.txt): 238public void checkNoModifiers(String noun, String verb) throws FHIRException { 239 if (hasModifierExtension()) { 240 throw new FHIRException("Found unknown Modifier Exceptions on "+noun+" doing "+verb); 241 } 242 243 } 244// end addition 245 246} 247