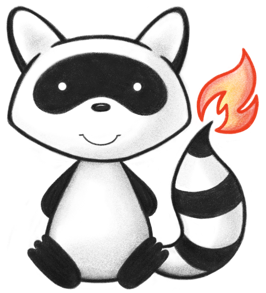
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum V3TriggerEventID { 037 038 /** 039 * Description: 040 */ 041 POLBTE004000UV, 042 /** 043 * Description: 044 */ 045 POLBTE004001UV, 046 /** 047 * Description: 048 */ 049 POLBTE004002UV, 050 /** 051 * Description: 052 */ 053 POLBTE004007UV, 054 /** 055 * Description: 056 */ 057 POLBTE004100UV, 058 /** 059 * Description: 060 */ 061 POLBTE004102UV, 062 /** 063 * Description: 064 */ 065 POLBTE004200UV, 066 /** 067 * Description: 068 */ 069 POLBTE004201UV, 070 /** 071 * Description: 072 */ 073 POLBTE004202UV, 074 /** 075 * Description: 076 */ 077 POLBTE004301UV, 078 /** 079 * Description: 080 */ 081 POLBTE004500UV, 082 /** 083 * added to help the parsers 084 */ 085 NULL; 086 087 public static V3TriggerEventID fromCode(String codeString) throws FHIRException { 088 if (codeString == null || "".equals(codeString)) 089 return null; 090 if ("POLB_TE004000UV".equals(codeString)) 091 return POLBTE004000UV; 092 if ("POLB_TE004001UV".equals(codeString)) 093 return POLBTE004001UV; 094 if ("POLB_TE004002UV".equals(codeString)) 095 return POLBTE004002UV; 096 if ("POLB_TE004007UV".equals(codeString)) 097 return POLBTE004007UV; 098 if ("POLB_TE004100UV".equals(codeString)) 099 return POLBTE004100UV; 100 if ("POLB_TE004102UV".equals(codeString)) 101 return POLBTE004102UV; 102 if ("POLB_TE004200UV".equals(codeString)) 103 return POLBTE004200UV; 104 if ("POLB_TE004201UV".equals(codeString)) 105 return POLBTE004201UV; 106 if ("POLB_TE004202UV".equals(codeString)) 107 return POLBTE004202UV; 108 if ("POLB_TE004301UV".equals(codeString)) 109 return POLBTE004301UV; 110 if ("POLB_TE004500UV".equals(codeString)) 111 return POLBTE004500UV; 112 throw new FHIRException("Unknown V3TriggerEventID code '" + codeString + "'"); 113 } 114 115 public String toCode() { 116 switch (this) { 117 case POLBTE004000UV: 118 return "POLB_TE004000UV"; 119 case POLBTE004001UV: 120 return "POLB_TE004001UV"; 121 case POLBTE004002UV: 122 return "POLB_TE004002UV"; 123 case POLBTE004007UV: 124 return "POLB_TE004007UV"; 125 case POLBTE004100UV: 126 return "POLB_TE004100UV"; 127 case POLBTE004102UV: 128 return "POLB_TE004102UV"; 129 case POLBTE004200UV: 130 return "POLB_TE004200UV"; 131 case POLBTE004201UV: 132 return "POLB_TE004201UV"; 133 case POLBTE004202UV: 134 return "POLB_TE004202UV"; 135 case POLBTE004301UV: 136 return "POLB_TE004301UV"; 137 case POLBTE004500UV: 138 return "POLB_TE004500UV"; 139 case NULL: 140 return null; 141 default: 142 return "?"; 143 } 144 } 145 146 public String getSystem() { 147 return "http://terminology.hl7.org/CodeSystem/v3-triggerEventID"; 148 } 149 150 public String getDefinition() { 151 switch (this) { 152 case POLBTE004000UV: 153 return "Description:"; 154 case POLBTE004001UV: 155 return "Description:"; 156 case POLBTE004002UV: 157 return "Description:"; 158 case POLBTE004007UV: 159 return "Description:"; 160 case POLBTE004100UV: 161 return "Description:"; 162 case POLBTE004102UV: 163 return "Description:"; 164 case POLBTE004200UV: 165 return "Description:"; 166 case POLBTE004201UV: 167 return "Description:"; 168 case POLBTE004202UV: 169 return "Description:"; 170 case POLBTE004301UV: 171 return "Description:"; 172 case POLBTE004500UV: 173 return "Description:"; 174 case NULL: 175 return null; 176 default: 177 return "?"; 178 } 179 } 180 181 public String getDisplay() { 182 switch (this) { 183 case POLBTE004000UV: 184 return "Result Status"; 185 case POLBTE004001UV: 186 return "Result Confirm"; 187 case POLBTE004002UV: 188 return "Result Reject"; 189 case POLBTE004007UV: 190 return "Result Tracking"; 191 case POLBTE004100UV: 192 return "Result in Progress"; 193 case POLBTE004102UV: 194 return "Result Activate"; 195 case POLBTE004200UV: 196 return "Result Complete with Fulfillment"; 197 case POLBTE004201UV: 198 return "Result Corrected"; 199 case POLBTE004202UV: 200 return "Result Complete"; 201 case POLBTE004301UV: 202 return "Result Abort"; 203 case POLBTE004500UV: 204 return "Result Nullify"; 205 case NULL: 206 return null; 207 default: 208 return "?"; 209 } 210 } 211 212}