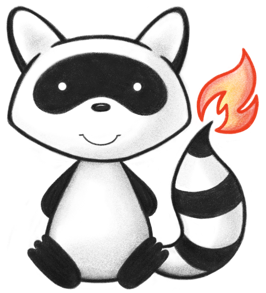
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum V3SubstanceAdminSubstitution { 037 038 /** 039 * Description: Substitution occurred or is permitted with another product that 040 * may potentially have different ingredients, but having the same biological 041 * and therapeutic effects. 042 */ 043 _ACTSUBSTANCEADMINSUBSTITUTIONCODE, 044 /** 045 * Description: Substitution occurred or is permitted with another bioequivalent 046 * and therapeutically equivalent product. 047 */ 048 E, 049 /** 050 * Description: 051 * 052 * 053 * Substitution occurred or is permitted with another product that is a: 054 * 055 * 056 * pharmaceutical alternative containing the same active ingredient but is 057 * formulated with different salt, ester pharmaceutical equivalent that has the 058 * same active ingredient, strength, dosage form and route of administration 059 * 060 * 061 * Examples: 062 * 063 * 064 * 065 * 066 * Pharmaceutical alternative: Erythromycin Ethylsuccinate for Erythromycin 067 * Stearate 068 * 069 * Pharmaceutical equivalent: Lisonpril for Zestril 070 */ 071 EC, 072 /** 073 * Description: 074 * 075 * 076 * Substitution occurred or is permitted between equivalent Brands but not 077 * Generics 078 * 079 * 080 * Examples: 081 * 082 * 083 * 084 * Zestril for Prinivil Coumadin for Jantoven 085 */ 086 BC, 087 /** 088 * Description: Substitution occurred or is permitted between equivalent 089 * Generics but not Brands 090 * 091 * 092 * Examples: 093 * 094 * 095 * 096 * Lisnopril (Lupin Corp) for Lisnopril (Wockhardt Corp) 097 */ 098 G, 099 /** 100 * Description: Substitution occurred or is permitted with another product 101 * having the same therapeutic objective and safety profile. 102 * 103 * 104 * Examples: 105 * 106 * 107 * 108 * ranitidine for Tagamet 109 */ 110 TE, 111 /** 112 * Description: Substitution occurred or is permitted between therapeutically 113 * equivalent Brands but not Generics > Examples: 114 * 115 * 116 * 117 * Zantac for Tagamet 118 */ 119 TB, 120 /** 121 * Description: Substitution occurred or is permitted between therapeutically 122 * equivalent Generics but not Brands > Examples: 123 * 124 * 125 * 126 * Ranitidine for cimetidine 127 */ 128 TG, 129 /** 130 * Description: This substitution was performed or is permitted based on 131 * formulary guidelines. 132 */ 133 F, 134 /** 135 * No substitution occurred or is permitted. 136 */ 137 N, 138 /** 139 * added to help the parsers 140 */ 141 NULL; 142 143 public static V3SubstanceAdminSubstitution fromCode(String codeString) throws FHIRException { 144 if (codeString == null || "".equals(codeString)) 145 return null; 146 if ("_ActSubstanceAdminSubstitutionCode".equals(codeString)) 147 return _ACTSUBSTANCEADMINSUBSTITUTIONCODE; 148 if ("E".equals(codeString)) 149 return E; 150 if ("EC".equals(codeString)) 151 return EC; 152 if ("BC".equals(codeString)) 153 return BC; 154 if ("G".equals(codeString)) 155 return G; 156 if ("TE".equals(codeString)) 157 return TE; 158 if ("TB".equals(codeString)) 159 return TB; 160 if ("TG".equals(codeString)) 161 return TG; 162 if ("F".equals(codeString)) 163 return F; 164 if ("N".equals(codeString)) 165 return N; 166 throw new FHIRException("Unknown V3SubstanceAdminSubstitution code '" + codeString + "'"); 167 } 168 169 public String toCode() { 170 switch (this) { 171 case _ACTSUBSTANCEADMINSUBSTITUTIONCODE: 172 return "_ActSubstanceAdminSubstitutionCode"; 173 case E: 174 return "E"; 175 case EC: 176 return "EC"; 177 case BC: 178 return "BC"; 179 case G: 180 return "G"; 181 case TE: 182 return "TE"; 183 case TB: 184 return "TB"; 185 case TG: 186 return "TG"; 187 case F: 188 return "F"; 189 case N: 190 return "N"; 191 case NULL: 192 return null; 193 default: 194 return "?"; 195 } 196 } 197 198 public String getSystem() { 199 return "http://terminology.hl7.org/CodeSystem/v3-substanceAdminSubstitution"; 200 } 201 202 public String getDefinition() { 203 switch (this) { 204 case _ACTSUBSTANCEADMINSUBSTITUTIONCODE: 205 return "Description: Substitution occurred or is permitted with another product that may potentially have different ingredients, but having the same biological and therapeutic effects."; 206 case E: 207 return "Description: Substitution occurred or is permitted with another bioequivalent and therapeutically equivalent product."; 208 case EC: 209 return "Description: \n \r\n\n Substitution occurred or is permitted with another product that is a:\r\n\n \n pharmaceutical alternative containing the same active ingredient but is formulated with different salt, ester\n pharmaceutical equivalent that has the same active ingredient, strength, dosage form and route of administration\n \n \n Examples: \n \r\n\n \n \n Pharmaceutical alternative: Erythromycin Ethylsuccinate for Erythromycin Stearate\n \n Pharmaceutical equivalent: Lisonpril for Zestril"; 210 case BC: 211 return "Description: \n \r\n\n Substitution occurred or is permitted between equivalent Brands but not Generics\r\n\n \n Examples: \n \r\n\n \n Zestril for Prinivil\n Coumadin for Jantoven"; 212 case G: 213 return "Description: Substitution occurred or is permitted between equivalent Generics but not Brands\r\n\n \n Examples: \n \r\n\n \n Lisnopril (Lupin Corp) for Lisnopril (Wockhardt Corp)"; 214 case TE: 215 return "Description: Substitution occurred or is permitted with another product having the same therapeutic objective and safety profile.\r\n\n \n Examples: \n \r\n\n \n ranitidine for Tagamet"; 216 case TB: 217 return "Description: Substitution occurred or is permitted between therapeutically equivalent Brands but not Generics\r\n>\n Examples: \n \r\n\n \n Zantac for Tagamet"; 218 case TG: 219 return "Description: Substitution occurred or is permitted between therapeutically equivalent Generics but not Brands\r\n>\n Examples: \n \r\n\n \n Ranitidine for cimetidine"; 220 case F: 221 return "Description: This substitution was performed or is permitted based on formulary guidelines."; 222 case N: 223 return "No substitution occurred or is permitted."; 224 case NULL: 225 return null; 226 default: 227 return "?"; 228 } 229 } 230 231 public String getDisplay() { 232 switch (this) { 233 case _ACTSUBSTANCEADMINSUBSTITUTIONCODE: 234 return "ActSubstanceAdminSubstitutionCode"; 235 case E: 236 return "equivalent"; 237 case EC: 238 return "equivalent composition"; 239 case BC: 240 return "brand composition"; 241 case G: 242 return "generic composition"; 243 case TE: 244 return "therapeutic alternative"; 245 case TB: 246 return "therapeutic brand"; 247 case TG: 248 return "therapeutic generic"; 249 case F: 250 return "formulary"; 251 case N: 252 return "none"; 253 case NULL: 254 return null; 255 default: 256 return "?"; 257 } 258 } 259 260}