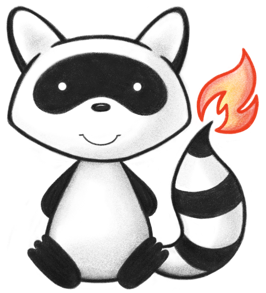
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum V3StyleType { 037 038 /** 039 * Defines font rendering characteristics 040 */ 041 _FONTSTYLE, 042 /** 043 * Render with a bold font 044 */ 045 BOLD, 046 /** 047 * Render with with some type of emphasis 048 */ 049 EMPHASIS, 050 /** 051 * Render italicized 052 */ 053 ITALICS, 054 /** 055 * Render with an underline font 056 */ 057 UNDERLINE, 058 /** 059 * Defines list rendering characteristics 060 */ 061 _LISTSTYLE, 062 /** 063 * Defines rendering characteristics for ordered lists 064 */ 065 _ORDEREDLISTSTYLE, 066 /** 067 * List is ordered using Arabic numerals: 1, 2, 3 068 */ 069 ARABIC, 070 /** 071 * List is ordered using big alpha characters: A, B, C 072 */ 073 BIGALPHA, 074 /** 075 * List is ordered using big Roman numerals: I, II, III 076 */ 077 BIGROMAN, 078 /** 079 * List is order using little alpha characters: a, b, c 080 */ 081 LITTLEALPHA, 082 /** 083 * List is ordered using little Roman numerals: i, ii, iii 084 */ 085 LITTLEROMAN, 086 /** 087 * Defines rendering characteristics for unordered lists 088 */ 089 _UNORDEREDLISTSTYLE, 090 /** 091 * List bullets are hollow discs 092 */ 093 CIRCLE, 094 /** 095 * List bullets are simple solid discs 096 */ 097 DISC, 098 /** 099 * List bullets are solid squares 100 */ 101 SQUARE, 102 /** 103 * Defines table cell rendering characteristics 104 */ 105 _TABLERULESTYLE, 106 /** 107 * Render cell with rule on bottom 108 */ 109 BOTRULE, 110 /** 111 * Render cell with left-sided rule 112 */ 113 LRULE, 114 /** 115 * Render cell with right-sided rule 116 */ 117 RRULE, 118 /** 119 * Render cell with rule on top 120 */ 121 TOPRULE, 122 /** 123 * added to help the parsers 124 */ 125 NULL; 126 127 public static V3StyleType fromCode(String codeString) throws FHIRException { 128 if (codeString == null || "".equals(codeString)) 129 return null; 130 if ("_FontStyle".equals(codeString)) 131 return _FONTSTYLE; 132 if ("bold".equals(codeString)) 133 return BOLD; 134 if ("emphasis".equals(codeString)) 135 return EMPHASIS; 136 if ("italics".equals(codeString)) 137 return ITALICS; 138 if ("underline".equals(codeString)) 139 return UNDERLINE; 140 if ("_ListStyle".equals(codeString)) 141 return _LISTSTYLE; 142 if ("_OrderedListStyle".equals(codeString)) 143 return _ORDEREDLISTSTYLE; 144 if ("Arabic".equals(codeString)) 145 return ARABIC; 146 if ("BigAlpha".equals(codeString)) 147 return BIGALPHA; 148 if ("BigRoman".equals(codeString)) 149 return BIGROMAN; 150 if ("LittleAlpha".equals(codeString)) 151 return LITTLEALPHA; 152 if ("LittleRoman".equals(codeString)) 153 return LITTLEROMAN; 154 if ("_UnorderedListStyle".equals(codeString)) 155 return _UNORDEREDLISTSTYLE; 156 if ("Circle".equals(codeString)) 157 return CIRCLE; 158 if ("Disc".equals(codeString)) 159 return DISC; 160 if ("Square".equals(codeString)) 161 return SQUARE; 162 if ("_TableRuleStyle".equals(codeString)) 163 return _TABLERULESTYLE; 164 if ("Botrule".equals(codeString)) 165 return BOTRULE; 166 if ("Lrule".equals(codeString)) 167 return LRULE; 168 if ("Rrule".equals(codeString)) 169 return RRULE; 170 if ("Toprule".equals(codeString)) 171 return TOPRULE; 172 throw new FHIRException("Unknown V3StyleType code '" + codeString + "'"); 173 } 174 175 public String toCode() { 176 switch (this) { 177 case _FONTSTYLE: 178 return "_FontStyle"; 179 case BOLD: 180 return "bold"; 181 case EMPHASIS: 182 return "emphasis"; 183 case ITALICS: 184 return "italics"; 185 case UNDERLINE: 186 return "underline"; 187 case _LISTSTYLE: 188 return "_ListStyle"; 189 case _ORDEREDLISTSTYLE: 190 return "_OrderedListStyle"; 191 case ARABIC: 192 return "Arabic"; 193 case BIGALPHA: 194 return "BigAlpha"; 195 case BIGROMAN: 196 return "BigRoman"; 197 case LITTLEALPHA: 198 return "LittleAlpha"; 199 case LITTLEROMAN: 200 return "LittleRoman"; 201 case _UNORDEREDLISTSTYLE: 202 return "_UnorderedListStyle"; 203 case CIRCLE: 204 return "Circle"; 205 case DISC: 206 return "Disc"; 207 case SQUARE: 208 return "Square"; 209 case _TABLERULESTYLE: 210 return "_TableRuleStyle"; 211 case BOTRULE: 212 return "Botrule"; 213 case LRULE: 214 return "Lrule"; 215 case RRULE: 216 return "Rrule"; 217 case TOPRULE: 218 return "Toprule"; 219 case NULL: 220 return null; 221 default: 222 return "?"; 223 } 224 } 225 226 public String getSystem() { 227 return "http://terminology.hl7.org/CodeSystem/v3-styleType"; 228 } 229 230 public String getDefinition() { 231 switch (this) { 232 case _FONTSTYLE: 233 return "Defines font rendering characteristics"; 234 case BOLD: 235 return "Render with a bold font"; 236 case EMPHASIS: 237 return "Render with with some type of emphasis"; 238 case ITALICS: 239 return "Render italicized"; 240 case UNDERLINE: 241 return "Render with an underline font"; 242 case _LISTSTYLE: 243 return "Defines list rendering characteristics"; 244 case _ORDEREDLISTSTYLE: 245 return "Defines rendering characteristics for ordered lists"; 246 case ARABIC: 247 return "List is ordered using Arabic numerals: 1, 2, 3"; 248 case BIGALPHA: 249 return "List is ordered using big alpha characters: A, B, C"; 250 case BIGROMAN: 251 return "List is ordered using big Roman numerals: I, II, III"; 252 case LITTLEALPHA: 253 return "List is order using little alpha characters: a, b, c"; 254 case LITTLEROMAN: 255 return "List is ordered using little Roman numerals: i, ii, iii"; 256 case _UNORDEREDLISTSTYLE: 257 return "Defines rendering characteristics for unordered lists"; 258 case CIRCLE: 259 return "List bullets are hollow discs"; 260 case DISC: 261 return "List bullets are simple solid discs"; 262 case SQUARE: 263 return "List bullets are solid squares"; 264 case _TABLERULESTYLE: 265 return "Defines table cell rendering characteristics"; 266 case BOTRULE: 267 return "Render cell with rule on bottom"; 268 case LRULE: 269 return "Render cell with left-sided rule"; 270 case RRULE: 271 return "Render cell with right-sided rule"; 272 case TOPRULE: 273 return "Render cell with rule on top"; 274 case NULL: 275 return null; 276 default: 277 return "?"; 278 } 279 } 280 281 public String getDisplay() { 282 switch (this) { 283 case _FONTSTYLE: 284 return "Font Style"; 285 case BOLD: 286 return "Bold Font"; 287 case EMPHASIS: 288 return "Emphasised Font"; 289 case ITALICS: 290 return "Italics Font"; 291 case UNDERLINE: 292 return "Underline Font"; 293 case _LISTSTYLE: 294 return "List Style"; 295 case _ORDEREDLISTSTYLE: 296 return "Ordered List Style"; 297 case ARABIC: 298 return "Arabic"; 299 case BIGALPHA: 300 return "Big Alpha"; 301 case BIGROMAN: 302 return "Big Roman"; 303 case LITTLEALPHA: 304 return "Little Alpha"; 305 case LITTLEROMAN: 306 return "Little Roman"; 307 case _UNORDEREDLISTSTYLE: 308 return "Unordered List Style"; 309 case CIRCLE: 310 return "Circle"; 311 case DISC: 312 return "Disc"; 313 case SQUARE: 314 return "Square"; 315 case _TABLERULESTYLE: 316 return "Table Rule Style"; 317 case BOTRULE: 318 return "Bottom Rule"; 319 case LRULE: 320 return "Left-sided rule"; 321 case RRULE: 322 return "Right-sided rule"; 323 case TOPRULE: 324 return "Top Rule"; 325 case NULL: 326 return null; 327 default: 328 return "?"; 329 } 330 } 331 332}