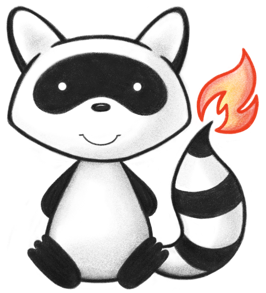
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum V3DataOperation { 037 038 /** 039 * Description:Act on an object or objects. 040 */ 041 OPERATE, 042 /** 043 * Description:Fundamental operation in an Information System (IS) that results 044 * only in the act of bringing an object into existence. Note: The preceding 045 * definition is taken from the HL7 RBAC specification. There is no restriction 046 * on how the operation is invoked, e.g., via a user interface. For an HL7 Act, 047 * the state transitions per the HL7 Reference Information Model. 048 */ 049 CREATE, 050 /** 051 * Description:Fundamental operation in an Information System (IS) that results 052 * only in the removal of information about an object from memory or storage. 053 * Note: The preceding definition is taken from the HL7 RBAC specification. 054 * There is no restriction on how the operation is invoked, e.g., via a user 055 * interface. 056 */ 057 DELETE, 058 /** 059 * Description:Fundamental operation in an IS that results only in initiating 060 * performance of a single or set of programs (i.e., software objects). Note: 061 * The preceding definition is taken from the HL7 RBAC specification. There is 062 * no restriction on how the operation is invoked, e.g., via a user interface. 063 */ 064 EXECUTE, 065 /** 066 * Description:Fundamental operation in an Information System (IS) that results 067 * only in the flow of information about an object to a subject. Note: The 068 * preceding definition is taken from the HL7 RBAC specification. There is no 069 * restriction on how the operation is invoked, e.g., via a user interface. 070 */ 071 READ, 072 /** 073 * Definition:Fundamental operation in an Information System (IS) that results 074 * only in the revision or alteration of an object. Note: The preceding 075 * definition is taken from the HL7 RBAC specification. There is no restriction 076 * on how the operation is invoked, e.g., via a user interface. 077 */ 078 UPDATE, 079 /** 080 * Description:Fundamental operation in an Information System (IS) that results 081 * only in the addition of information to an object already in existence. Note: 082 * The preceding definition is taken from the HL7 RBAC specification. There is 083 * no restriction on how the operation is invoked, e.g., via a user interface. 084 */ 085 APPEND, 086 /** 087 * Description:Change the status of an object representing an Act. 088 */ 089 MODIFYSTATUS, 090 /** 091 * Description:Change the status of an object representing an Act to "aborted", 092 * i.e., terminated prior to the originally intended completion. For an HL7 Act, 093 * the state transitions per the HL7 Reference Information Model. 094 */ 095 ABORT, 096 /** 097 * Description:Change the status of an object representing an Act to "active", 098 * i.e., so it can be performed or is being performed, for the first time. 099 * (Contrast with REACTIVATE.) For an HL7 Act, the state transitions per the HL7 100 * Reference Information Model. 101 */ 102 ACTIVATE, 103 /** 104 * Description:Change the status of an object representing an Act to 105 * "cancelled", i.e., abandoned before activation. For an HL7 Act, the state 106 * transitions per the HL7 Reference Information Model. 107 */ 108 CANCEL, 109 /** 110 * Description:Change the status of an object representing an Act to 111 * "completed", i.e., terminated normally after all of its constituents have 112 * been performed. For an HL7 Act, the state transitions per the HL7 Reference 113 * Information Model. 114 */ 115 COMPLETE, 116 /** 117 * Description:Change the status of an object representing an Act to "held", 118 * i.e., put aside an Act that is still in preparatory stages. No action can 119 * occur until the Act is released. For an HL7 Act, the state transitions per 120 * the HL7 Reference Information Model. 121 */ 122 HOLD, 123 /** 124 * Description:Change the status of an object representing an Act to a normal 125 * state. For an HL7 Act, the state transitions per the HL7 Reference 126 * Information Model. 127 */ 128 JUMP, 129 /** 130 * Description:Change the status of an object representing an Act to 131 * "nullified", i.e., treat as though it never existed. For an HL7 Act, the 132 * state transitions per the HL7 Reference Information Model. 133 */ 134 NULLIFY, 135 /** 136 * Description:Change the status of an object representing an Act to "obsolete" 137 * when it has been replaced by a new instance. For an HL7 Act, the state 138 * transitions per the HL7 Reference Information Model. 139 */ 140 OBSOLETE, 141 /** 142 * Description:Change the status of a formerly active object representing an Act 143 * to "active", i.e., so it can again be performed or is being performed. 144 * (Contrast with ACTIVATE.) For an HL7 Act, the state transitions per the HL7 145 * Reference Information Model. 146 */ 147 REACTIVATE, 148 /** 149 * Description:Change the status of an object representing an Act so it is no 150 * longer "held", i.e., allow action to occur. For an HL7 Act, the state 151 * transitions per the HL7 Reference Information Model. 152 */ 153 RELEASE, 154 /** 155 * Description:Change the status of a suspended object representing an Act to 156 * "active", i.e., so it can be performed or is being performed. For an HL7 Act, 157 * the state transitions per the HL7 Reference Information Model. 158 */ 159 RESUME, 160 /** 161 * Definition:Change the status of an object representing an Act to suspended, 162 * i.e., so it is temporarily not in service. 163 */ 164 SUSPEND, 165 /** 166 * added to help the parsers 167 */ 168 NULL; 169 170 public static V3DataOperation fromCode(String codeString) throws FHIRException { 171 if (codeString == null || "".equals(codeString)) 172 return null; 173 if ("OPERATE".equals(codeString)) 174 return OPERATE; 175 if ("CREATE".equals(codeString)) 176 return CREATE; 177 if ("DELETE".equals(codeString)) 178 return DELETE; 179 if ("EXECUTE".equals(codeString)) 180 return EXECUTE; 181 if ("READ".equals(codeString)) 182 return READ; 183 if ("UPDATE".equals(codeString)) 184 return UPDATE; 185 if ("APPEND".equals(codeString)) 186 return APPEND; 187 if ("MODIFYSTATUS".equals(codeString)) 188 return MODIFYSTATUS; 189 if ("ABORT".equals(codeString)) 190 return ABORT; 191 if ("ACTIVATE".equals(codeString)) 192 return ACTIVATE; 193 if ("CANCEL".equals(codeString)) 194 return CANCEL; 195 if ("COMPLETE".equals(codeString)) 196 return COMPLETE; 197 if ("HOLD".equals(codeString)) 198 return HOLD; 199 if ("JUMP".equals(codeString)) 200 return JUMP; 201 if ("NULLIFY".equals(codeString)) 202 return NULLIFY; 203 if ("OBSOLETE".equals(codeString)) 204 return OBSOLETE; 205 if ("REACTIVATE".equals(codeString)) 206 return REACTIVATE; 207 if ("RELEASE".equals(codeString)) 208 return RELEASE; 209 if ("RESUME".equals(codeString)) 210 return RESUME; 211 if ("SUSPEND".equals(codeString)) 212 return SUSPEND; 213 throw new FHIRException("Unknown V3DataOperation code '" + codeString + "'"); 214 } 215 216 public String toCode() { 217 switch (this) { 218 case OPERATE: 219 return "OPERATE"; 220 case CREATE: 221 return "CREATE"; 222 case DELETE: 223 return "DELETE"; 224 case EXECUTE: 225 return "EXECUTE"; 226 case READ: 227 return "READ"; 228 case UPDATE: 229 return "UPDATE"; 230 case APPEND: 231 return "APPEND"; 232 case MODIFYSTATUS: 233 return "MODIFYSTATUS"; 234 case ABORT: 235 return "ABORT"; 236 case ACTIVATE: 237 return "ACTIVATE"; 238 case CANCEL: 239 return "CANCEL"; 240 case COMPLETE: 241 return "COMPLETE"; 242 case HOLD: 243 return "HOLD"; 244 case JUMP: 245 return "JUMP"; 246 case NULLIFY: 247 return "NULLIFY"; 248 case OBSOLETE: 249 return "OBSOLETE"; 250 case REACTIVATE: 251 return "REACTIVATE"; 252 case RELEASE: 253 return "RELEASE"; 254 case RESUME: 255 return "RESUME"; 256 case SUSPEND: 257 return "SUSPEND"; 258 case NULL: 259 return null; 260 default: 261 return "?"; 262 } 263 } 264 265 public String getSystem() { 266 return "http://terminology.hl7.org/CodeSystem/v3-DataOperation"; 267 } 268 269 public String getDefinition() { 270 switch (this) { 271 case OPERATE: 272 return "Description:Act on an object or objects."; 273 case CREATE: 274 return "Description:Fundamental operation in an Information System (IS) that results only in the act of bringing an object into existence. Note: The preceding definition is taken from the HL7 RBAC specification. There is no restriction on how the operation is invoked, e.g., via a user interface. For an HL7 Act, the state transitions per the HL7 Reference Information Model."; 275 case DELETE: 276 return "Description:Fundamental operation in an Information System (IS) that results only in the removal of information about an object from memory or storage. Note: The preceding definition is taken from the HL7 RBAC specification. There is no restriction on how the operation is invoked, e.g., via a user interface."; 277 case EXECUTE: 278 return "Description:Fundamental operation in an IS that results only in initiating performance of a single or set of programs (i.e., software objects). Note: The preceding definition is taken from the HL7 RBAC specification. There is no restriction on how the operation is invoked, e.g., via a user interface."; 279 case READ: 280 return "Description:Fundamental operation in an Information System (IS) that results only in the flow of information about an object to a subject. Note: The preceding definition is taken from the HL7 RBAC specification. There is no restriction on how the operation is invoked, e.g., via a user interface."; 281 case UPDATE: 282 return "Definition:Fundamental operation in an Information System (IS) that results only in the revision or alteration of an object. Note: The preceding definition is taken from the HL7 RBAC specification. There is no restriction on how the operation is invoked, e.g., via a user interface."; 283 case APPEND: 284 return "Description:Fundamental operation in an Information System (IS) that results only in the addition of information to an object already in existence. Note: The preceding definition is taken from the HL7 RBAC specification. There is no restriction on how the operation is invoked, e.g., via a user interface."; 285 case MODIFYSTATUS: 286 return "Description:Change the status of an object representing an Act."; 287 case ABORT: 288 return "Description:Change the status of an object representing an Act to \"aborted\", i.e., terminated prior to the originally intended completion. For an HL7 Act, the state transitions per the HL7 Reference Information Model."; 289 case ACTIVATE: 290 return "Description:Change the status of an object representing an Act to \"active\", i.e., so it can be performed or is being performed, for the first time. (Contrast with REACTIVATE.) For an HL7 Act, the state transitions per the HL7 Reference Information Model."; 291 case CANCEL: 292 return "Description:Change the status of an object representing an Act to \"cancelled\", i.e., abandoned before activation. For an HL7 Act, the state transitions per the HL7 Reference Information Model."; 293 case COMPLETE: 294 return "Description:Change the status of an object representing an Act to \"completed\", i.e., terminated normally after all of its constituents have been performed. For an HL7 Act, the state transitions per the HL7 Reference Information Model."; 295 case HOLD: 296 return "Description:Change the status of an object representing an Act to \"held\", i.e., put aside an Act that is still in preparatory stages. No action can occur until the Act is released. For an HL7 Act, the state transitions per the HL7 Reference Information Model."; 297 case JUMP: 298 return "Description:Change the status of an object representing an Act to a normal state. For an HL7 Act, the state transitions per the HL7 Reference Information Model."; 299 case NULLIFY: 300 return "Description:Change the status of an object representing an Act to \"nullified\", i.e., treat as though it never existed. For an HL7 Act, the state transitions per the HL7 Reference Information Model."; 301 case OBSOLETE: 302 return "Description:Change the status of an object representing an Act to \"obsolete\" when it has been replaced by a new instance. For an HL7 Act, the state transitions per the HL7 Reference Information Model."; 303 case REACTIVATE: 304 return "Description:Change the status of a formerly active object representing an Act to \"active\", i.e., so it can again be performed or is being performed. (Contrast with ACTIVATE.) For an HL7 Act, the state transitions per the HL7 Reference Information Model."; 305 case RELEASE: 306 return "Description:Change the status of an object representing an Act so it is no longer \"held\", i.e., allow action to occur. For an HL7 Act, the state transitions per the HL7 Reference Information Model."; 307 case RESUME: 308 return "Description:Change the status of a suspended object representing an Act to \"active\", i.e., so it can be performed or is being performed. For an HL7 Act, the state transitions per the HL7 Reference Information Model."; 309 case SUSPEND: 310 return "Definition:Change the status of an object representing an Act to suspended, i.e., so it is temporarily not in service."; 311 case NULL: 312 return null; 313 default: 314 return "?"; 315 } 316 } 317 318 public String getDisplay() { 319 switch (this) { 320 case OPERATE: 321 return "operate"; 322 case CREATE: 323 return "create"; 324 case DELETE: 325 return "delete"; 326 case EXECUTE: 327 return "execute"; 328 case READ: 329 return "read"; 330 case UPDATE: 331 return "revise"; 332 case APPEND: 333 return "append"; 334 case MODIFYSTATUS: 335 return "modify status"; 336 case ABORT: 337 return "abort"; 338 case ACTIVATE: 339 return "activate"; 340 case CANCEL: 341 return "cancel"; 342 case COMPLETE: 343 return "complete"; 344 case HOLD: 345 return "hold"; 346 case JUMP: 347 return "jump"; 348 case NULLIFY: 349 return "nullify"; 350 case OBSOLETE: 351 return "obsolete"; 352 case REACTIVATE: 353 return "reactivate"; 354 case RELEASE: 355 return "release"; 356 case RESUME: 357 return "resume"; 358 case SUSPEND: 359 return "suspend"; 360 case NULL: 361 return null; 362 default: 363 return "?"; 364 } 365 } 366 367}