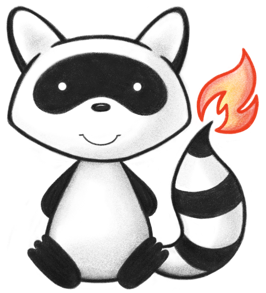
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum V3AddressPartType { 037 038 /** 039 * This can be a unit designator, such as apartment number, suite number, or 040 * floor. There may be several unit designators in an address (e.g., "3rd floor, 041 * Appt. 342"). This can also be a designator pointing away from the location, 042 * rather than specifying a smaller location within some larger one (e.g., Dutch 043 * "t.o." means "opposite to" for house boats located across the street facing 044 * houses). 045 */ 046 ADL, 047 /** 048 * Description: An address line is for either an additional locator, a delivery 049 * address or a street address. 050 */ 051 AL, 052 /** 053 * A delivery address line is frequently used instead of breaking out delivery 054 * mode, delivery installation, etc. An address generally has only a delivery 055 * address line or a street address line, but not both. 056 */ 057 DAL, 058 /** 059 * Description: A street address line is frequently used instead of breaking out 060 * build number, street name, street type, etc. An address generally has only a 061 * delivery address line or a street address line, but not both. 062 */ 063 SAL, 064 /** 065 * The numeric portion of a building number 066 */ 067 BNN, 068 /** 069 * The number of a building, house or lot alongside the street. Also known as 070 * "primary street number". This does not number the street but rather the 071 * building. 072 */ 073 BNR, 074 /** 075 * Any alphabetic character, fraction or other text that may appear after the 076 * numeric portion of a building number 077 */ 078 BNS, 079 /** 080 * The name of the party who will take receipt at the specified address, and 081 * will take on responsibility for ensuring delivery to the target recipient 082 */ 083 CAR, 084 /** 085 * A geographic sub-unit delineated for demographic purposes. 086 */ 087 CEN, 088 /** 089 * Country 090 */ 091 CNT, 092 /** 093 * A sub-unit of a state or province. (49 of the United States of America use 094 * the term "county;" Louisiana uses the term "parish".) 095 */ 096 CPA, 097 /** 098 * The name of the city, town, village, or other community or delivery center 099 */ 100 CTY, 101 /** 102 * Delimiters are printed without framing white space. If no value component is 103 * provided, the delimiter appears as a line break. 104 */ 105 DEL, 106 /** 107 * Indicates the type of delivery installation (the facility to which the mail 108 * will be delivered prior to final shipping via the delivery mode.) Example: 109 * post office, letter carrier depot, community mail center, station, etc. 110 */ 111 DINST, 112 /** 113 * The location of the delivery installation, usually a town or city, and is 114 * only required if the area is different from the municipality. Area to which 115 * mail delivery service is provided from any postal facility or service such as 116 * an individual letter carrier, rural route, or postal route. 117 */ 118 DINSTA, 119 /** 120 * A number, letter or name identifying a delivery installation. E.g., for 121 * Station A, the delivery installation qualifier would be 'A'. 122 */ 123 DINSTQ, 124 /** 125 * Direction (e.g., N, S, W, E) 126 */ 127 DIR, 128 /** 129 * Indicates the type of service offered, method of delivery. For example: post 130 * office box, rural route, general delivery, etc. 131 */ 132 DMOD, 133 /** 134 * Represents the routing information such as a letter carrier route number. It 135 * is the identifying number of the designator (the box number or rural route 136 * number). 137 */ 138 DMODID, 139 /** 140 * A value that uniquely identifies the postal address. 141 */ 142 DPID, 143 /** 144 * Description:An intersection denotes that the actual address is located AT or 145 * CLOSE TO the intersection OF two or more streets. 146 */ 147 INT, 148 /** 149 * A numbered box located in a post station. 150 */ 151 POB, 152 /** 153 * A subsection of a municipality 154 */ 155 PRE, 156 /** 157 * A sub-unit of a country with limited sovereignty in a federally organized 158 * country. 159 */ 160 STA, 161 /** 162 * The base name of a roadway or artery recognized by a municipality (excluding 163 * street type and direction) 164 */ 165 STB, 166 /** 167 * street name 168 */ 169 STR, 170 /** 171 * The designation given to the street. (e.g. Street, Avenue, Crescent, etc.) 172 */ 173 STTYP, 174 /** 175 * The number or name of a specific unit contained within a building or complex, 176 * as assigned by that building or complex. 177 */ 178 UNID, 179 /** 180 * Indicates the type of specific unit contained within a building or complex. 181 * E.g. Appartment, Floor 182 */ 183 UNIT, 184 /** 185 * A postal code designating a region defined by the postal service. 186 */ 187 ZIP, 188 /** 189 * added to help the parsers 190 */ 191 NULL; 192 193 public static V3AddressPartType fromCode(String codeString) throws FHIRException { 194 if (codeString == null || "".equals(codeString)) 195 return null; 196 if ("ADL".equals(codeString)) 197 return ADL; 198 if ("AL".equals(codeString)) 199 return AL; 200 if ("DAL".equals(codeString)) 201 return DAL; 202 if ("SAL".equals(codeString)) 203 return SAL; 204 if ("BNN".equals(codeString)) 205 return BNN; 206 if ("BNR".equals(codeString)) 207 return BNR; 208 if ("BNS".equals(codeString)) 209 return BNS; 210 if ("CAR".equals(codeString)) 211 return CAR; 212 if ("CEN".equals(codeString)) 213 return CEN; 214 if ("CNT".equals(codeString)) 215 return CNT; 216 if ("CPA".equals(codeString)) 217 return CPA; 218 if ("CTY".equals(codeString)) 219 return CTY; 220 if ("DEL".equals(codeString)) 221 return DEL; 222 if ("DINST".equals(codeString)) 223 return DINST; 224 if ("DINSTA".equals(codeString)) 225 return DINSTA; 226 if ("DINSTQ".equals(codeString)) 227 return DINSTQ; 228 if ("DIR".equals(codeString)) 229 return DIR; 230 if ("DMOD".equals(codeString)) 231 return DMOD; 232 if ("DMODID".equals(codeString)) 233 return DMODID; 234 if ("DPID".equals(codeString)) 235 return DPID; 236 if ("INT".equals(codeString)) 237 return INT; 238 if ("POB".equals(codeString)) 239 return POB; 240 if ("PRE".equals(codeString)) 241 return PRE; 242 if ("STA".equals(codeString)) 243 return STA; 244 if ("STB".equals(codeString)) 245 return STB; 246 if ("STR".equals(codeString)) 247 return STR; 248 if ("STTYP".equals(codeString)) 249 return STTYP; 250 if ("UNID".equals(codeString)) 251 return UNID; 252 if ("UNIT".equals(codeString)) 253 return UNIT; 254 if ("ZIP".equals(codeString)) 255 return ZIP; 256 throw new FHIRException("Unknown V3AddressPartType code '" + codeString + "'"); 257 } 258 259 public String toCode() { 260 switch (this) { 261 case ADL: 262 return "ADL"; 263 case AL: 264 return "AL"; 265 case DAL: 266 return "DAL"; 267 case SAL: 268 return "SAL"; 269 case BNN: 270 return "BNN"; 271 case BNR: 272 return "BNR"; 273 case BNS: 274 return "BNS"; 275 case CAR: 276 return "CAR"; 277 case CEN: 278 return "CEN"; 279 case CNT: 280 return "CNT"; 281 case CPA: 282 return "CPA"; 283 case CTY: 284 return "CTY"; 285 case DEL: 286 return "DEL"; 287 case DINST: 288 return "DINST"; 289 case DINSTA: 290 return "DINSTA"; 291 case DINSTQ: 292 return "DINSTQ"; 293 case DIR: 294 return "DIR"; 295 case DMOD: 296 return "DMOD"; 297 case DMODID: 298 return "DMODID"; 299 case DPID: 300 return "DPID"; 301 case INT: 302 return "INT"; 303 case POB: 304 return "POB"; 305 case PRE: 306 return "PRE"; 307 case STA: 308 return "STA"; 309 case STB: 310 return "STB"; 311 case STR: 312 return "STR"; 313 case STTYP: 314 return "STTYP"; 315 case UNID: 316 return "UNID"; 317 case UNIT: 318 return "UNIT"; 319 case ZIP: 320 return "ZIP"; 321 case NULL: 322 return null; 323 default: 324 return "?"; 325 } 326 } 327 328 public String getSystem() { 329 return "http://terminology.hl7.org/CodeSystem/v3-AddressPartType"; 330 } 331 332 public String getDefinition() { 333 switch (this) { 334 case ADL: 335 return "This can be a unit designator, such as apartment number, suite number, or floor. There may be several unit designators in an address (e.g., \"3rd floor, Appt. 342\"). This can also be a designator pointing away from the location, rather than specifying a smaller location within some larger one (e.g., Dutch \"t.o.\" means \"opposite to\" for house boats located across the street facing houses)."; 336 case AL: 337 return "Description: An address line is for either an additional locator, a delivery address or a street address."; 338 case DAL: 339 return "A delivery address line is frequently used instead of breaking out delivery mode, delivery installation, etc. An address generally has only a delivery address line or a street address line, but not both."; 340 case SAL: 341 return "Description: A street address line is frequently used instead of breaking out build number, street name, street type, etc. An address generally has only a delivery address line or a street address line, but not both."; 342 case BNN: 343 return "The numeric portion of a building number"; 344 case BNR: 345 return "The number of a building, house or lot alongside the street. Also known as \"primary street number\". This does not number the street but rather the building."; 346 case BNS: 347 return "Any alphabetic character, fraction or other text that may appear after the numeric portion of a building number"; 348 case CAR: 349 return "The name of the party who will take receipt at the specified address, and will take on responsibility for ensuring delivery to the target recipient"; 350 case CEN: 351 return "A geographic sub-unit delineated for demographic purposes."; 352 case CNT: 353 return "Country"; 354 case CPA: 355 return "A sub-unit of a state or province. (49 of the United States of America use the term \"county;\" Louisiana uses the term \"parish\".)"; 356 case CTY: 357 return "The name of the city, town, village, or other community or delivery center"; 358 case DEL: 359 return "Delimiters are printed without framing white space. If no value component is provided, the delimiter appears as a line break."; 360 case DINST: 361 return "Indicates the type of delivery installation (the facility to which the mail will be delivered prior to final shipping via the delivery mode.) Example: post office, letter carrier depot, community mail center, station, etc."; 362 case DINSTA: 363 return "The location of the delivery installation, usually a town or city, and is only required if the area is different from the municipality. Area to which mail delivery service is provided from any postal facility or service such as an individual letter carrier, rural route, or postal route."; 364 case DINSTQ: 365 return "A number, letter or name identifying a delivery installation. E.g., for Station A, the delivery installation qualifier would be 'A'."; 366 case DIR: 367 return "Direction (e.g., N, S, W, E)"; 368 case DMOD: 369 return "Indicates the type of service offered, method of delivery. For example: post office box, rural route, general delivery, etc."; 370 case DMODID: 371 return "Represents the routing information such as a letter carrier route number. It is the identifying number of the designator (the box number or rural route number)."; 372 case DPID: 373 return "A value that uniquely identifies the postal address."; 374 case INT: 375 return "Description:An intersection denotes that the actual address is located AT or CLOSE TO the intersection OF two or more streets."; 376 case POB: 377 return "A numbered box located in a post station."; 378 case PRE: 379 return "A subsection of a municipality"; 380 case STA: 381 return "A sub-unit of a country with limited sovereignty in a federally organized country."; 382 case STB: 383 return "The base name of a roadway or artery recognized by a municipality (excluding street type and direction)"; 384 case STR: 385 return "street name"; 386 case STTYP: 387 return "The designation given to the street. (e.g. Street, Avenue, Crescent, etc.)"; 388 case UNID: 389 return "The number or name of a specific unit contained within a building or complex, as assigned by that building or complex."; 390 case UNIT: 391 return "Indicates the type of specific unit contained within a building or complex. E.g. Appartment, Floor"; 392 case ZIP: 393 return "A postal code designating a region defined by the postal service."; 394 case NULL: 395 return null; 396 default: 397 return "?"; 398 } 399 } 400 401 public String getDisplay() { 402 switch (this) { 403 case ADL: 404 return "additional locator"; 405 case AL: 406 return "address line"; 407 case DAL: 408 return "delivery address line"; 409 case SAL: 410 return "street address line"; 411 case BNN: 412 return "building number numeric"; 413 case BNR: 414 return "building number"; 415 case BNS: 416 return "building number suffix"; 417 case CAR: 418 return "care of"; 419 case CEN: 420 return "census tract"; 421 case CNT: 422 return "country"; 423 case CPA: 424 return "county or parish"; 425 case CTY: 426 return "municipality"; 427 case DEL: 428 return "delimiter"; 429 case DINST: 430 return "delivery installation type"; 431 case DINSTA: 432 return "delivery installation area"; 433 case DINSTQ: 434 return "delivery installation qualifier"; 435 case DIR: 436 return "direction"; 437 case DMOD: 438 return "delivery mode"; 439 case DMODID: 440 return "delivery mode identifier"; 441 case DPID: 442 return "delivery point identifier"; 443 case INT: 444 return "intersection"; 445 case POB: 446 return "post box"; 447 case PRE: 448 return "precinct"; 449 case STA: 450 return "state or province"; 451 case STB: 452 return "street name base"; 453 case STR: 454 return "street name"; 455 case STTYP: 456 return "street type"; 457 case UNID: 458 return "unit identifier"; 459 case UNIT: 460 return "unit designator"; 461 case ZIP: 462 return "postal code"; 463 case NULL: 464 return null; 465 default: 466 return "?"; 467 } 468 } 469 470}