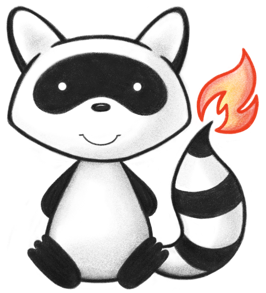
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum UsageContextType { 037 038 /** 039 * The gender of the patient. For this context type, appropriate values can be 040 * found in the http://hl7.org/fhir/ValueSet/administrative-gender value set. 041 */ 042 GENDER, 043 /** 044 * The age of the patient. For this context type, the value could be a range 045 * that specifies the applicable ages or a code from an appropriate value set 046 * such as the MeSH value set 047 * http://terminology.hl7.org/ValueSet/v3-AgeGroupObservationValue. 048 */ 049 AGE, 050 /** 051 * The clinical concept(s) addressed by the artifact. For example, disease, 052 * diagnostic test interpretation, medication ordering as in 053 * http://hl7.org/fhir/ValueSet/condition-code. 054 */ 055 FOCUS, 056 /** 057 * The clinical specialty of the context in which the patient is being treated - 058 * For example, PCP, Patient, Cardiologist, Behavioral Professional, Oral Health 059 * Professional, Prescriber, etc... taken from a specialty value set such as the 060 * NUCC Health Care provider taxonomy value set 061 * http://hl7.org/fhir/ValueSet/provider-taxonomy. 062 */ 063 USER, 064 /** 065 * The settings in which the artifact is intended for use. For example, 066 * admission, pre-op, etc. For example, the ActEncounterCode value set 067 * http://terminology.hl7.org/ValueSet/v3-ActEncounterCode. 068 */ 069 WORKFLOW, 070 /** 071 * The context for the clinical task(s) represented by this artifact. For 072 * example, this could be any task context represented by the HL7 ActTaskCode 073 * value set http://terminology.hl7.org/ValueSet/v3-ActTaskCode. General 074 * categories include: order entry, patient documentation and patient 075 * information review. 076 */ 077 TASK, 078 /** 079 * The venue in which an artifact could be used. For example, Outpatient, 080 * Inpatient, Home, Nursing home. The code value may originate from the HL7 081 * ServiceDeliveryLocationRoleType value set 082 * (http://terminology.hl7.org/ValueSet/v3-ServiceDeliveryLocationRoleType). 083 */ 084 VENUE, 085 /** 086 * The species to which an artifact applies. For example, SNOMED - 387961004 | 087 * Kingdom Animalia (organism). 088 */ 089 SPECIES, 090 /** 091 * A program/project of work for which this artifact is applicable. 092 */ 093 PROGRAM, 094 /** 095 * added to help the parsers 096 */ 097 NULL; 098 099 public static UsageContextType fromCode(String codeString) throws FHIRException { 100 if (codeString == null || "".equals(codeString)) 101 return null; 102 if ("gender".equals(codeString)) 103 return GENDER; 104 if ("age".equals(codeString)) 105 return AGE; 106 if ("focus".equals(codeString)) 107 return FOCUS; 108 if ("user".equals(codeString)) 109 return USER; 110 if ("workflow".equals(codeString)) 111 return WORKFLOW; 112 if ("task".equals(codeString)) 113 return TASK; 114 if ("venue".equals(codeString)) 115 return VENUE; 116 if ("species".equals(codeString)) 117 return SPECIES; 118 if ("program".equals(codeString)) 119 return PROGRAM; 120 throw new FHIRException("Unknown UsageContextType code '" + codeString + "'"); 121 } 122 123 public String toCode() { 124 switch (this) { 125 case GENDER: 126 return "gender"; 127 case AGE: 128 return "age"; 129 case FOCUS: 130 return "focus"; 131 case USER: 132 return "user"; 133 case WORKFLOW: 134 return "workflow"; 135 case TASK: 136 return "task"; 137 case VENUE: 138 return "venue"; 139 case SPECIES: 140 return "species"; 141 case PROGRAM: 142 return "program"; 143 case NULL: 144 return null; 145 default: 146 return "?"; 147 } 148 } 149 150 public String getSystem() { 151 return "http://terminology.hl7.org/CodeSystem/usage-context-type"; 152 } 153 154 public String getDefinition() { 155 switch (this) { 156 case GENDER: 157 return "The gender of the patient. For this context type, appropriate values can be found in the http://hl7.org/fhir/ValueSet/administrative-gender value set."; 158 case AGE: 159 return "The age of the patient. For this context type, the value could be a range that specifies the applicable ages or a code from an appropriate value set such as the MeSH value set http://terminology.hl7.org/ValueSet/v3-AgeGroupObservationValue."; 160 case FOCUS: 161 return "The clinical concept(s) addressed by the artifact. For example, disease, diagnostic test interpretation, medication ordering as in http://hl7.org/fhir/ValueSet/condition-code."; 162 case USER: 163 return "The clinical specialty of the context in which the patient is being treated - For example, PCP, Patient, Cardiologist, Behavioral Professional, Oral Health Professional, Prescriber, etc... taken from a specialty value set such as the NUCC Health Care provider taxonomy value set http://hl7.org/fhir/ValueSet/provider-taxonomy."; 164 case WORKFLOW: 165 return "The settings in which the artifact is intended for use. For example, admission, pre-op, etc. For example, the ActEncounterCode value set http://terminology.hl7.org/ValueSet/v3-ActEncounterCode."; 166 case TASK: 167 return "The context for the clinical task(s) represented by this artifact. For example, this could be any task context represented by the HL7 ActTaskCode value set http://terminology.hl7.org/ValueSet/v3-ActTaskCode. General categories include: order entry, patient documentation and patient information review."; 168 case VENUE: 169 return "The venue in which an artifact could be used. For example, Outpatient, Inpatient, Home, Nursing home. The code value may originate from the HL7 ServiceDeliveryLocationRoleType value set (http://terminology.hl7.org/ValueSet/v3-ServiceDeliveryLocationRoleType)."; 170 case SPECIES: 171 return "The species to which an artifact applies. For example, SNOMED - 387961004 | Kingdom Animalia (organism)."; 172 case PROGRAM: 173 return "A program/project of work for which this artifact is applicable."; 174 case NULL: 175 return null; 176 default: 177 return "?"; 178 } 179 } 180 181 public String getDisplay() { 182 switch (this) { 183 case GENDER: 184 return "Gender"; 185 case AGE: 186 return "Age Range"; 187 case FOCUS: 188 return "Clinical Focus"; 189 case USER: 190 return "User Type"; 191 case WORKFLOW: 192 return "Workflow Setting"; 193 case TASK: 194 return "Workflow Task"; 195 case VENUE: 196 return "Clinical Venue"; 197 case SPECIES: 198 return "Species"; 199 case PROGRAM: 200 return "Program"; 201 case NULL: 202 return null; 203 default: 204 return "?"; 205 } 206 } 207 208}