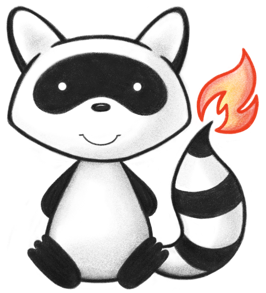
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum Tldc { 037 038 /** 039 * Design is under development (nascent). 040 */ 041 DRAFT, 042 /** 043 * Design is completed and is being reviewed. 044 */ 045 PENDING, 046 /** 047 * Design has been deemed fit for the intended purpose and is published by the 048 * governance group. 049 */ 050 ACTIVE, 051 /** 052 * Design is active, but is under review. The review may result in a change to 053 * the design. The change may necessitate a new version to be created. This in 054 * turn may result in the prior version of the template to be retired. 055 * Alternatively, the review may result in a change to the design that does not 056 * require a new version to be created, or it may result in no change to the 057 * design at all. 058 */ 059 REVIEW, 060 /** 061 * A drafted design is determined to be erroneous or not fit for intended 062 * purpose and is discontinued before ever being published in an active state. 063 */ 064 CANCELLED, 065 /** 066 * A previously drafted design is determined to be erroneous or not fit for 067 * intended purpose and is discontinued before ever being published for 068 * consideration in a pending state. 069 */ 070 REJECTED, 071 /** 072 * A previously active design is discontinued from use. It should no longer be 073 * used for future designs, but for historical purposes may be used to process 074 * data previously recorded using this design. A newer design may or may not 075 * exist. The design is published in the retired state. 076 */ 077 RETIRED, 078 /** 079 * A design is determined to be erroneous or not fit for the intended purpose 080 * and should no longer be used, even for historical purposes. No new designs 081 * can be developed for this template. The associated template no longer needs 082 * to be published, but if published, is shown in the terminated state. 083 */ 084 TERMINATED, 085 /** 086 * added to help the parsers 087 */ 088 NULL; 089 090 public static Tldc fromCode(String codeString) throws FHIRException { 091 if (codeString == null || "".equals(codeString)) 092 return null; 093 if ("draft".equals(codeString)) 094 return DRAFT; 095 if ("pending".equals(codeString)) 096 return PENDING; 097 if ("active".equals(codeString)) 098 return ACTIVE; 099 if ("review".equals(codeString)) 100 return REVIEW; 101 if ("cancelled".equals(codeString)) 102 return CANCELLED; 103 if ("rejected".equals(codeString)) 104 return REJECTED; 105 if ("retired".equals(codeString)) 106 return RETIRED; 107 if ("terminated".equals(codeString)) 108 return TERMINATED; 109 throw new FHIRException("Unknown Tldc code '" + codeString + "'"); 110 } 111 112 public String toCode() { 113 switch (this) { 114 case DRAFT: 115 return "draft"; 116 case PENDING: 117 return "pending"; 118 case ACTIVE: 119 return "active"; 120 case REVIEW: 121 return "review"; 122 case CANCELLED: 123 return "cancelled"; 124 case REJECTED: 125 return "rejected"; 126 case RETIRED: 127 return "retired"; 128 case TERMINATED: 129 return "terminated"; 130 case NULL: 131 return null; 132 default: 133 return "?"; 134 } 135 } 136 137 public String getSystem() { 138 return "urn:oid:2.16.840.1.113883.3.1937.98.5.8"; 139 } 140 141 public String getDefinition() { 142 switch (this) { 143 case DRAFT: 144 return "Design is under development (nascent)."; 145 case PENDING: 146 return "Design is completed and is being reviewed."; 147 case ACTIVE: 148 return "Design has been deemed fit for the intended purpose and is published by the governance group."; 149 case REVIEW: 150 return "Design is active, but is under review. The review may result in a change to the design. The change may necessitate a new version to be created. This in turn may result in the prior version of the template to be retired. Alternatively, the review may result in a change to the design that does not require a new version to be created, or it may result in no change to the design at all."; 151 case CANCELLED: 152 return "A drafted design is determined to be erroneous or not fit for intended purpose and is discontinued before ever being published in an active state."; 153 case REJECTED: 154 return "A previously drafted design is determined to be erroneous or not fit for intended purpose and is discontinued before ever being published for consideration in a pending state."; 155 case RETIRED: 156 return "A previously active design is discontinued from use. It should no longer be used for future designs, but for historical purposes may be used to process data previously recorded using this design. A newer design may or may not exist. The design is published in the retired state."; 157 case TERMINATED: 158 return "A design is determined to be erroneous or not fit for the intended purpose and should no longer be used, even for historical purposes. No new designs can be developed for this template. The associated template no longer needs to be published, but if published, is shown in the terminated state."; 159 case NULL: 160 return null; 161 default: 162 return "?"; 163 } 164 } 165 166 public String getDisplay() { 167 switch (this) { 168 case DRAFT: 169 return "Draft"; 170 case PENDING: 171 return "Under pre-publication review"; 172 case ACTIVE: 173 return "active"; 174 case REVIEW: 175 return "In Review"; 176 case CANCELLED: 177 return "Cancelled"; 178 case REJECTED: 179 return "Rejected"; 180 case RETIRED: 181 return "retired"; 182 case TERMINATED: 183 return "Terminated"; 184 case NULL: 185 return null; 186 default: 187 return "?"; 188 } 189 } 190 191}