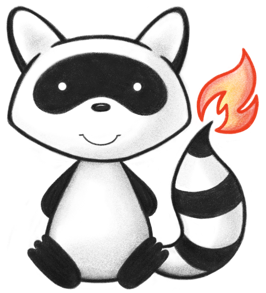
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum SmartCapabilities { 037 038 /** 039 * support for SMART?s EHR Launch mode. 040 */ 041 LAUNCHEHR, 042 /** 043 * support for SMART?s Standalone Launch mode. 044 */ 045 LAUNCHSTANDALONE, 046 /** 047 * support for SMART?s public client profile (no client authentication). 048 */ 049 CLIENTPUBLIC, 050 /** 051 * support for SMART?s confidential client profile (symmetric client secret 052 * authentication). 053 */ 054 CLIENTCONFIDENTIALSYMMETRIC, 055 /** 056 * support for SMART?s OpenID Connect profile. 057 */ 058 SSOOPENIDCONNECT, 059 /** 060 * support for ?need patient banner? launch context (conveyed via 061 * need_patient_banner token parameter). 062 */ 063 CONTEXTPASSTHROUGHBANNER, 064 /** 065 * support for ?SMART style URL? launch context (conveyed via smart_style_url 066 * token parameter). 067 */ 068 CONTEXTPASSTHROUGHSTYLE, 069 /** 070 * support for patient-level launch context (requested by launch/patient scope, 071 * conveyed via patient token parameter). 072 */ 073 CONTEXTEHRPATIENT, 074 /** 075 * support for encounter-level launch context (requested by launch/encounter 076 * scope, conveyed via encounter token parameter). 077 */ 078 CONTEXTEHRENCOUNTER, 079 /** 080 * support for patient-level launch context (requested by launch/patient scope, 081 * conveyed via patient token parameter). 082 */ 083 CONTEXTSTANDALONEPATIENT, 084 /** 085 * support for encounter-level launch context (requested by launch/encounter 086 * scope, conveyed via encounter token parameter). 087 */ 088 CONTEXTSTANDALONEENCOUNTER, 089 /** 090 * support for refresh tokens (requested by offline_access scope). 091 */ 092 PERMISSIONOFFLINE, 093 /** 094 * support for patient-level scopes (e.g. patient/Observation.read). 095 */ 096 PERMISSIONPATIENT, 097 /** 098 * support for user-level scopes (e.g. user/Appointment.read). 099 */ 100 PERMISSIONUSER, 101 /** 102 * added to help the parsers 103 */ 104 NULL; 105 106 public static SmartCapabilities fromCode(String codeString) throws FHIRException { 107 if (codeString == null || "".equals(codeString)) 108 return null; 109 if ("launch-ehr".equals(codeString)) 110 return LAUNCHEHR; 111 if ("launch-standalone".equals(codeString)) 112 return LAUNCHSTANDALONE; 113 if ("client-public".equals(codeString)) 114 return CLIENTPUBLIC; 115 if ("client-confidential-symmetric".equals(codeString)) 116 return CLIENTCONFIDENTIALSYMMETRIC; 117 if ("sso-openid-connect".equals(codeString)) 118 return SSOOPENIDCONNECT; 119 if ("context-passthrough-banner".equals(codeString)) 120 return CONTEXTPASSTHROUGHBANNER; 121 if ("context-passthrough-style".equals(codeString)) 122 return CONTEXTPASSTHROUGHSTYLE; 123 if ("context-ehr-patient".equals(codeString)) 124 return CONTEXTEHRPATIENT; 125 if ("context-ehr-encounter".equals(codeString)) 126 return CONTEXTEHRENCOUNTER; 127 if ("context-standalone-patient".equals(codeString)) 128 return CONTEXTSTANDALONEPATIENT; 129 if ("context-standalone-encounter".equals(codeString)) 130 return CONTEXTSTANDALONEENCOUNTER; 131 if ("permission-offline".equals(codeString)) 132 return PERMISSIONOFFLINE; 133 if ("permission-patient".equals(codeString)) 134 return PERMISSIONPATIENT; 135 if ("permission-user".equals(codeString)) 136 return PERMISSIONUSER; 137 throw new FHIRException("Unknown SmartCapabilities code '" + codeString + "'"); 138 } 139 140 public String toCode() { 141 switch (this) { 142 case LAUNCHEHR: 143 return "launch-ehr"; 144 case LAUNCHSTANDALONE: 145 return "launch-standalone"; 146 case CLIENTPUBLIC: 147 return "client-public"; 148 case CLIENTCONFIDENTIALSYMMETRIC: 149 return "client-confidential-symmetric"; 150 case SSOOPENIDCONNECT: 151 return "sso-openid-connect"; 152 case CONTEXTPASSTHROUGHBANNER: 153 return "context-passthrough-banner"; 154 case CONTEXTPASSTHROUGHSTYLE: 155 return "context-passthrough-style"; 156 case CONTEXTEHRPATIENT: 157 return "context-ehr-patient"; 158 case CONTEXTEHRENCOUNTER: 159 return "context-ehr-encounter"; 160 case CONTEXTSTANDALONEPATIENT: 161 return "context-standalone-patient"; 162 case CONTEXTSTANDALONEENCOUNTER: 163 return "context-standalone-encounter"; 164 case PERMISSIONOFFLINE: 165 return "permission-offline"; 166 case PERMISSIONPATIENT: 167 return "permission-patient"; 168 case PERMISSIONUSER: 169 return "permission-user"; 170 case NULL: 171 return null; 172 default: 173 return "?"; 174 } 175 } 176 177 public String getSystem() { 178 return "http://terminology.hl7.org/CodeSystem/smart-capabilities"; 179 } 180 181 public String getDefinition() { 182 switch (this) { 183 case LAUNCHEHR: 184 return "support for SMART?s EHR Launch mode."; 185 case LAUNCHSTANDALONE: 186 return "support for SMART?s Standalone Launch mode."; 187 case CLIENTPUBLIC: 188 return "support for SMART?s public client profile (no client authentication)."; 189 case CLIENTCONFIDENTIALSYMMETRIC: 190 return "support for SMART?s confidential client profile (symmetric client secret authentication)."; 191 case SSOOPENIDCONNECT: 192 return "support for SMART?s OpenID Connect profile."; 193 case CONTEXTPASSTHROUGHBANNER: 194 return "support for ?need patient banner? launch context (conveyed via need_patient_banner token parameter)."; 195 case CONTEXTPASSTHROUGHSTYLE: 196 return "support for ?SMART style URL? launch context (conveyed via smart_style_url token parameter)."; 197 case CONTEXTEHRPATIENT: 198 return "support for patient-level launch context (requested by launch/patient scope, conveyed via patient token parameter)."; 199 case CONTEXTEHRENCOUNTER: 200 return "support for encounter-level launch context (requested by launch/encounter scope, conveyed via encounter token parameter)."; 201 case CONTEXTSTANDALONEPATIENT: 202 return "support for patient-level launch context (requested by launch/patient scope, conveyed via patient token parameter)."; 203 case CONTEXTSTANDALONEENCOUNTER: 204 return "support for encounter-level launch context (requested by launch/encounter scope, conveyed via encounter token parameter)."; 205 case PERMISSIONOFFLINE: 206 return "support for refresh tokens (requested by offline_access scope)."; 207 case PERMISSIONPATIENT: 208 return "support for patient-level scopes (e.g. patient/Observation.read)."; 209 case PERMISSIONUSER: 210 return "support for user-level scopes (e.g. user/Appointment.read)."; 211 case NULL: 212 return null; 213 default: 214 return "?"; 215 } 216 } 217 218 public String getDisplay() { 219 switch (this) { 220 case LAUNCHEHR: 221 return "EHR Launch Mode"; 222 case LAUNCHSTANDALONE: 223 return "Standalone Launch Mode"; 224 case CLIENTPUBLIC: 225 return "Public Client Profile"; 226 case CLIENTCONFIDENTIALSYMMETRIC: 227 return "Confidential Client Profile"; 228 case SSOOPENIDCONNECT: 229 return "Supports OpenID Connect"; 230 case CONTEXTPASSTHROUGHBANNER: 231 return "Allows \"Need Patient Banner\""; 232 case CONTEXTPASSTHROUGHSTYLE: 233 return "Allows \"Smart Style Style\""; 234 case CONTEXTEHRPATIENT: 235 return "Allows \"Patient Level Launch Context (EHR)\""; 236 case CONTEXTEHRENCOUNTER: 237 return "Allows \"Encounter Level Launch Context (EHR)\""; 238 case CONTEXTSTANDALONEPATIENT: 239 return "Allows \"Patient Level Launch Context (STANDALONE)\""; 240 case CONTEXTSTANDALONEENCOUNTER: 241 return "Allows \"Encounter Level Launch Context (STANDALONE)\""; 242 case PERMISSIONOFFLINE: 243 return "Supports Refresh Token"; 244 case PERMISSIONPATIENT: 245 return "Supports Patient Level Scopes"; 246 case PERMISSIONUSER: 247 return "Supports User Level Scopes"; 248 case NULL: 249 return null; 250 default: 251 return "?"; 252 } 253 } 254 255}