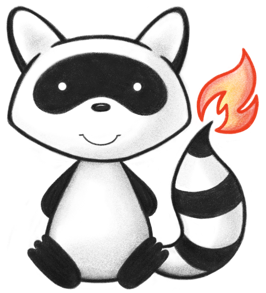
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum ReferencerangeMeaning { 037 038 /** 039 * General types of reference range. 040 */ 041 TYPE, 042 /** 043 * Values expected for a normal member of the relevant control population being 044 * measured. Typically each results producer such as a laboratory has specific 045 * normal ranges and they are usually defined as within two standard deviations 046 * from the mean and account for 95.45% of this population. 047 */ 048 NORMAL, 049 /** 050 * The range that is recommended by a relevant professional body. 051 */ 052 RECOMMENDED, 053 /** 054 * The range at which treatment would/should be considered. 055 */ 056 TREATMENT, 057 /** 058 * The optimal range for best therapeutic outcomes. 059 */ 060 THERAPEUTIC, 061 /** 062 * The optimal range for best therapeutic outcomes for a specimen taken 063 * immediately before administration. 064 */ 065 PRE, 066 /** 067 * The optimal range for best therapeutic outcomes for a specimen taken 068 * immediately after administration. 069 */ 070 POST, 071 /** 072 * Endocrine related states that change the expected value. 073 */ 074 ENDOCRINE, 075 /** 076 * An expected range in an individual prior to puberty. 077 */ 078 PREPUBERTY, 079 /** 080 * An expected range in an individual during the follicular stage of the cycle. 081 */ 082 FOLLICULAR, 083 /** 084 * An expected range in an individual during the midcycle stage of the cycle. 085 */ 086 MIDCYCLE, 087 /** 088 * An expected range in an individual during the luteal stage of the cycle. 089 */ 090 LUTEAL, 091 /** 092 * An expected range in an individual post-menopause. 093 */ 094 POSTMENOPAUSAL, 095 /** 096 * added to help the parsers 097 */ 098 NULL; 099 100 public static ReferencerangeMeaning fromCode(String codeString) throws FHIRException { 101 if (codeString == null || "".equals(codeString)) 102 return null; 103 if ("type".equals(codeString)) 104 return TYPE; 105 if ("normal".equals(codeString)) 106 return NORMAL; 107 if ("recommended".equals(codeString)) 108 return RECOMMENDED; 109 if ("treatment".equals(codeString)) 110 return TREATMENT; 111 if ("therapeutic".equals(codeString)) 112 return THERAPEUTIC; 113 if ("pre".equals(codeString)) 114 return PRE; 115 if ("post".equals(codeString)) 116 return POST; 117 if ("endocrine".equals(codeString)) 118 return ENDOCRINE; 119 if ("pre-puberty".equals(codeString)) 120 return PREPUBERTY; 121 if ("follicular".equals(codeString)) 122 return FOLLICULAR; 123 if ("midcycle".equals(codeString)) 124 return MIDCYCLE; 125 if ("luteal".equals(codeString)) 126 return LUTEAL; 127 if ("postmenopausal".equals(codeString)) 128 return POSTMENOPAUSAL; 129 throw new FHIRException("Unknown ReferencerangeMeaning code '" + codeString + "'"); 130 } 131 132 public String toCode() { 133 switch (this) { 134 case TYPE: 135 return "type"; 136 case NORMAL: 137 return "normal"; 138 case RECOMMENDED: 139 return "recommended"; 140 case TREATMENT: 141 return "treatment"; 142 case THERAPEUTIC: 143 return "therapeutic"; 144 case PRE: 145 return "pre"; 146 case POST: 147 return "post"; 148 case ENDOCRINE: 149 return "endocrine"; 150 case PREPUBERTY: 151 return "pre-puberty"; 152 case FOLLICULAR: 153 return "follicular"; 154 case MIDCYCLE: 155 return "midcycle"; 156 case LUTEAL: 157 return "luteal"; 158 case POSTMENOPAUSAL: 159 return "postmenopausal"; 160 case NULL: 161 return null; 162 default: 163 return "?"; 164 } 165 } 166 167 public String getSystem() { 168 return "http://terminology.hl7.org/CodeSystem/referencerange-meaning"; 169 } 170 171 public String getDefinition() { 172 switch (this) { 173 case TYPE: 174 return "General types of reference range."; 175 case NORMAL: 176 return "Values expected for a normal member of the relevant control population being measured. Typically each results producer such as a laboratory has specific normal ranges and they are usually defined as within two standard deviations from the mean and account for 95.45% of this population."; 177 case RECOMMENDED: 178 return "The range that is recommended by a relevant professional body."; 179 case TREATMENT: 180 return "The range at which treatment would/should be considered."; 181 case THERAPEUTIC: 182 return "The optimal range for best therapeutic outcomes."; 183 case PRE: 184 return "The optimal range for best therapeutic outcomes for a specimen taken immediately before administration."; 185 case POST: 186 return "The optimal range for best therapeutic outcomes for a specimen taken immediately after administration."; 187 case ENDOCRINE: 188 return "Endocrine related states that change the expected value."; 189 case PREPUBERTY: 190 return "An expected range in an individual prior to puberty."; 191 case FOLLICULAR: 192 return "An expected range in an individual during the follicular stage of the cycle."; 193 case MIDCYCLE: 194 return "An expected range in an individual during the midcycle stage of the cycle."; 195 case LUTEAL: 196 return "An expected range in an individual during the luteal stage of the cycle."; 197 case POSTMENOPAUSAL: 198 return "An expected range in an individual post-menopause."; 199 case NULL: 200 return null; 201 default: 202 return "?"; 203 } 204 } 205 206 public String getDisplay() { 207 switch (this) { 208 case TYPE: 209 return "Type"; 210 case NORMAL: 211 return "Normal Range"; 212 case RECOMMENDED: 213 return "Recommended Range"; 214 case TREATMENT: 215 return "Treatment Range"; 216 case THERAPEUTIC: 217 return "Therapeutic Desired Level"; 218 case PRE: 219 return "Pre Therapeutic Desired Level"; 220 case POST: 221 return "Post Therapeutic Desired Level"; 222 case ENDOCRINE: 223 return "Endocrine"; 224 case PREPUBERTY: 225 return "Pre-Puberty"; 226 case FOLLICULAR: 227 return "Follicular Stage"; 228 case MIDCYCLE: 229 return "MidCycle"; 230 case LUTEAL: 231 return "Luteal"; 232 case POSTMENOPAUSAL: 233 return "Post-Menopause"; 234 case NULL: 235 return null; 236 default: 237 return "?"; 238 } 239 } 240 241}