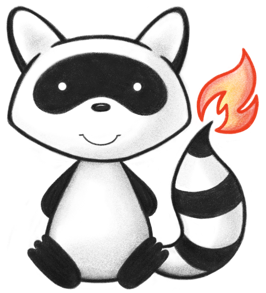
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum MetricColor { 037 038 /** 039 * Color for representation - black. 040 */ 041 BLACK, 042 /** 043 * Color for representation - red. 044 */ 045 RED, 046 /** 047 * Color for representation - green. 048 */ 049 GREEN, 050 /** 051 * Color for representation - yellow. 052 */ 053 YELLOW, 054 /** 055 * Color for representation - blue. 056 */ 057 BLUE, 058 /** 059 * Color for representation - magenta. 060 */ 061 MAGENTA, 062 /** 063 * Color for representation - cyan. 064 */ 065 CYAN, 066 /** 067 * Color for representation - white. 068 */ 069 WHITE, 070 /** 071 * added to help the parsers 072 */ 073 NULL; 074 075 public static MetricColor fromCode(String codeString) throws FHIRException { 076 if (codeString == null || "".equals(codeString)) 077 return null; 078 if ("black".equals(codeString)) 079 return BLACK; 080 if ("red".equals(codeString)) 081 return RED; 082 if ("green".equals(codeString)) 083 return GREEN; 084 if ("yellow".equals(codeString)) 085 return YELLOW; 086 if ("blue".equals(codeString)) 087 return BLUE; 088 if ("magenta".equals(codeString)) 089 return MAGENTA; 090 if ("cyan".equals(codeString)) 091 return CYAN; 092 if ("white".equals(codeString)) 093 return WHITE; 094 throw new FHIRException("Unknown MetricColor code '" + codeString + "'"); 095 } 096 097 public String toCode() { 098 switch (this) { 099 case BLACK: 100 return "black"; 101 case RED: 102 return "red"; 103 case GREEN: 104 return "green"; 105 case YELLOW: 106 return "yellow"; 107 case BLUE: 108 return "blue"; 109 case MAGENTA: 110 return "magenta"; 111 case CYAN: 112 return "cyan"; 113 case WHITE: 114 return "white"; 115 case NULL: 116 return null; 117 default: 118 return "?"; 119 } 120 } 121 122 public String getSystem() { 123 return "http://hl7.org/fhir/metric-color"; 124 } 125 126 public String getDefinition() { 127 switch (this) { 128 case BLACK: 129 return "Color for representation - black."; 130 case RED: 131 return "Color for representation - red."; 132 case GREEN: 133 return "Color for representation - green."; 134 case YELLOW: 135 return "Color for representation - yellow."; 136 case BLUE: 137 return "Color for representation - blue."; 138 case MAGENTA: 139 return "Color for representation - magenta."; 140 case CYAN: 141 return "Color for representation - cyan."; 142 case WHITE: 143 return "Color for representation - white."; 144 case NULL: 145 return null; 146 default: 147 return "?"; 148 } 149 } 150 151 public String getDisplay() { 152 switch (this) { 153 case BLACK: 154 return "Color Black"; 155 case RED: 156 return "Color Red"; 157 case GREEN: 158 return "Color Green"; 159 case YELLOW: 160 return "Color Yellow"; 161 case BLUE: 162 return "Color Blue"; 163 case MAGENTA: 164 return "Color Magenta"; 165 case CYAN: 166 return "Color Cyan"; 167 case WHITE: 168 return "Color White"; 169 case NULL: 170 return null; 171 default: 172 return "?"; 173 } 174 } 175 176}