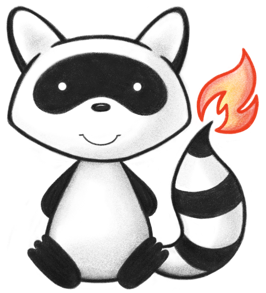
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum KnowledgeResourceTypes { 037 038 /** 039 * The definition of a specific activity to be taken, independent of any 040 * particular patient or context. 041 */ 042 ACTIVITYDEFINITION, 043 /** 044 * A set of codes drawn from one or more code systems. 045 */ 046 CODESYSTEM, 047 /** 048 * A map from one set of concepts to one or more other concepts. 049 */ 050 CONCEPTMAP, 051 /** 052 * Represents a library of quality improvement components. 053 */ 054 LIBRARY, 055 /** 056 * A quality measure definition. 057 */ 058 MEASURE, 059 /** 060 * The definition of a plan for a series of actions, independent of any specific 061 * patient or context. 062 */ 063 PLANDEFINITION, 064 /** 065 * Structural Definition. 066 */ 067 STRUCTUREDEFINITION, 068 /** 069 * A Map of relationships between 2 structures that can be used to transform 070 * data. 071 */ 072 STRUCTUREMAP, 073 /** 074 * A set of codes drawn from one or more code systems. 075 */ 076 VALUESET, 077 /** 078 * added to help the parsers 079 */ 080 NULL; 081 082 public static KnowledgeResourceTypes fromCode(String codeString) throws FHIRException { 083 if (codeString == null || "".equals(codeString)) 084 return null; 085 if ("ActivityDefinition".equals(codeString)) 086 return ACTIVITYDEFINITION; 087 if ("CodeSystem".equals(codeString)) 088 return CODESYSTEM; 089 if ("ConceptMap".equals(codeString)) 090 return CONCEPTMAP; 091 if ("Library".equals(codeString)) 092 return LIBRARY; 093 if ("Measure".equals(codeString)) 094 return MEASURE; 095 if ("PlanDefinition".equals(codeString)) 096 return PLANDEFINITION; 097 if ("StructureDefinition".equals(codeString)) 098 return STRUCTUREDEFINITION; 099 if ("StructureMap".equals(codeString)) 100 return STRUCTUREMAP; 101 if ("ValueSet".equals(codeString)) 102 return VALUESET; 103 throw new FHIRException("Unknown KnowledgeResourceTypes code '" + codeString + "'"); 104 } 105 106 public String toCode() { 107 switch (this) { 108 case ACTIVITYDEFINITION: 109 return "ActivityDefinition"; 110 case CODESYSTEM: 111 return "CodeSystem"; 112 case CONCEPTMAP: 113 return "ConceptMap"; 114 case LIBRARY: 115 return "Library"; 116 case MEASURE: 117 return "Measure"; 118 case PLANDEFINITION: 119 return "PlanDefinition"; 120 case STRUCTUREDEFINITION: 121 return "StructureDefinition"; 122 case STRUCTUREMAP: 123 return "StructureMap"; 124 case VALUESET: 125 return "ValueSet"; 126 case NULL: 127 return null; 128 default: 129 return "?"; 130 } 131 } 132 133 public String getSystem() { 134 return "http://hl7.org/fhir/knowledge-resource-types"; 135 } 136 137 public String getDefinition() { 138 switch (this) { 139 case ACTIVITYDEFINITION: 140 return "The definition of a specific activity to be taken, independent of any particular patient or context."; 141 case CODESYSTEM: 142 return "A set of codes drawn from one or more code systems."; 143 case CONCEPTMAP: 144 return "A map from one set of concepts to one or more other concepts."; 145 case LIBRARY: 146 return "Represents a library of quality improvement components."; 147 case MEASURE: 148 return "A quality measure definition."; 149 case PLANDEFINITION: 150 return "The definition of a plan for a series of actions, independent of any specific patient or context."; 151 case STRUCTUREDEFINITION: 152 return "Structural Definition."; 153 case STRUCTUREMAP: 154 return "A Map of relationships between 2 structures that can be used to transform data."; 155 case VALUESET: 156 return "A set of codes drawn from one or more code systems."; 157 case NULL: 158 return null; 159 default: 160 return "?"; 161 } 162 } 163 164 public String getDisplay() { 165 switch (this) { 166 case ACTIVITYDEFINITION: 167 return "ActivityDefinition"; 168 case CODESYSTEM: 169 return "CodeSystem"; 170 case CONCEPTMAP: 171 return "ConceptMap"; 172 case LIBRARY: 173 return "Library"; 174 case MEASURE: 175 return "Measure"; 176 case PLANDEFINITION: 177 return "PlanDefinition"; 178 case STRUCTUREDEFINITION: 179 return "StructureDefinition"; 180 case STRUCTUREMAP: 181 return "StructureMap"; 182 case VALUESET: 183 return "ValueSet"; 184 case NULL: 185 return null; 186 default: 187 return "?"; 188 } 189 } 190 191}