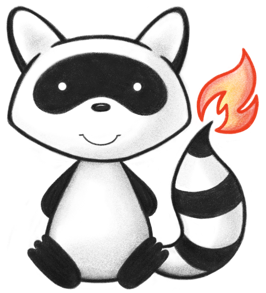
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum GoalStatusReason { 037 038 /** 039 * Goal suspended or ended because of a surgical procedure. 040 */ 041 SURGERY, 042 /** 043 * Goal suspended or ended because of a significant life event (marital change, 044 * bereavement, etc.). 045 */ 046 LIFEEVENT, 047 /** 048 * Goal has been superseded by a new goal. 049 */ 050 REPLACED, 051 /** 052 * Patient wishes the goal to be set aside, at least temporarily. 053 */ 054 PATIENTREQUEST, 055 /** 056 * Goal cannot be reached temporarily. 057 */ 058 TEMPNOTATTAINABLE, 059 /** 060 * Goal cannot be reached permanently. 061 */ 062 PERMANENTNOTATTAINABLE, 063 /** 064 * Goal cannot be reached due to financial barrier or reason. 065 */ 066 FINANCIALBARRIER, 067 /** 068 * Goal cannot be reached due to a lack of transportation. 069 */ 070 LACKOFTRANSPORTATION, 071 /** 072 * Goal cannot be reached due to a lack of social support. 073 */ 074 LACKOFSOCIALSUPPORT, 075 /** 076 * added to help the parsers 077 */ 078 NULL; 079 080 public static GoalStatusReason fromCode(String codeString) throws FHIRException { 081 if (codeString == null || "".equals(codeString)) 082 return null; 083 if ("surgery".equals(codeString)) 084 return SURGERY; 085 if ("life-event".equals(codeString)) 086 return LIFEEVENT; 087 if ("replaced".equals(codeString)) 088 return REPLACED; 089 if ("patient-request".equals(codeString)) 090 return PATIENTREQUEST; 091 if ("temp-not-attainable".equals(codeString)) 092 return TEMPNOTATTAINABLE; 093 if ("permanent-not-attainable".equals(codeString)) 094 return PERMANENTNOTATTAINABLE; 095 if ("financial-barrier".equals(codeString)) 096 return FINANCIALBARRIER; 097 if ("lack-of-transportation".equals(codeString)) 098 return LACKOFTRANSPORTATION; 099 if ("lack-of-social-support".equals(codeString)) 100 return LACKOFSOCIALSUPPORT; 101 throw new FHIRException("Unknown GoalStatusReason code '" + codeString + "'"); 102 } 103 104 public String toCode() { 105 switch (this) { 106 case SURGERY: 107 return "surgery"; 108 case LIFEEVENT: 109 return "life-event"; 110 case REPLACED: 111 return "replaced"; 112 case PATIENTREQUEST: 113 return "patient-request"; 114 case TEMPNOTATTAINABLE: 115 return "temp-not-attainable"; 116 case PERMANENTNOTATTAINABLE: 117 return "permanent-not-attainable"; 118 case FINANCIALBARRIER: 119 return "financial-barrier"; 120 case LACKOFTRANSPORTATION: 121 return "lack-of-transportation"; 122 case LACKOFSOCIALSUPPORT: 123 return "lack-of-social-support"; 124 case NULL: 125 return null; 126 default: 127 return "?"; 128 } 129 } 130 131 public String getSystem() { 132 return "http://hl7.org/fhir/goal-status-reason"; 133 } 134 135 public String getDefinition() { 136 switch (this) { 137 case SURGERY: 138 return "Goal suspended or ended because of a surgical procedure."; 139 case LIFEEVENT: 140 return "Goal suspended or ended because of a significant life event (marital change, bereavement, etc.)."; 141 case REPLACED: 142 return "Goal has been superseded by a new goal."; 143 case PATIENTREQUEST: 144 return "Patient wishes the goal to be set aside, at least temporarily."; 145 case TEMPNOTATTAINABLE: 146 return "Goal cannot be reached temporarily."; 147 case PERMANENTNOTATTAINABLE: 148 return "Goal cannot be reached permanently."; 149 case FINANCIALBARRIER: 150 return "Goal cannot be reached due to financial barrier or reason."; 151 case LACKOFTRANSPORTATION: 152 return "Goal cannot be reached due to a lack of transportation."; 153 case LACKOFSOCIALSUPPORT: 154 return "Goal cannot be reached due to a lack of social support."; 155 case NULL: 156 return null; 157 default: 158 return "?"; 159 } 160 } 161 162 public String getDisplay() { 163 switch (this) { 164 case SURGERY: 165 return "Surgery"; 166 case LIFEEVENT: 167 return "Life Event"; 168 case REPLACED: 169 return "Replaced"; 170 case PATIENTREQUEST: 171 return "Patient Request"; 172 case TEMPNOTATTAINABLE: 173 return "Goal Not Attainable Temporarily"; 174 case PERMANENTNOTATTAINABLE: 175 return "Goal Not Attainable Permanently"; 176 case FINANCIALBARRIER: 177 return "Financial Reason"; 178 case LACKOFTRANSPORTATION: 179 return "Lack Of Transportation"; 180 case LACKOFSOCIALSUPPORT: 181 return "Lack Of Social Support"; 182 case NULL: 183 return null; 184 default: 185 return "?"; 186 } 187 } 188 189}