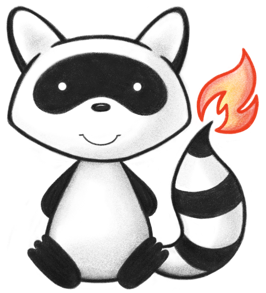
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum CodesystemContentMode { 037 038 /** 039 * None of the concepts defined by the code system are included in the code 040 * system resource. 041 */ 042 NOTPRESENT, 043 /** 044 * A few representative concepts are included in the code system resource. There 045 * is no useful intent in the subset choice and there's no process to make it 046 * workable: it's not intended to be workable. 047 */ 048 EXAMPLE, 049 /** 050 * A subset of the code system concepts are included in the code system 051 * resource. This is a curated subset released for a specific purpose under the 052 * governance of the code system steward, and that the intent, bounds and 053 * consequences of the fragmentation are clearly defined in the fragment or the 054 * code system documentation. Fragments are also known as partitions. 055 */ 056 FRAGMENT, 057 /** 058 * All the concepts defined by the code system are included in the code system 059 * resource. 060 */ 061 COMPLETE, 062 /** 063 * The resource doesn't define any new concepts; it just provides additional 064 * designations and properties to another code system. 065 */ 066 SUPPLEMENT, 067 /** 068 * added to help the parsers 069 */ 070 NULL; 071 072 public static CodesystemContentMode fromCode(String codeString) throws FHIRException { 073 if (codeString == null || "".equals(codeString)) 074 return null; 075 if ("not-present".equals(codeString)) 076 return NOTPRESENT; 077 if ("example".equals(codeString)) 078 return EXAMPLE; 079 if ("fragment".equals(codeString)) 080 return FRAGMENT; 081 if ("complete".equals(codeString)) 082 return COMPLETE; 083 if ("supplement".equals(codeString)) 084 return SUPPLEMENT; 085 throw new FHIRException("Unknown CodesystemContentMode code '" + codeString + "'"); 086 } 087 088 public String toCode() { 089 switch (this) { 090 case NOTPRESENT: 091 return "not-present"; 092 case EXAMPLE: 093 return "example"; 094 case FRAGMENT: 095 return "fragment"; 096 case COMPLETE: 097 return "complete"; 098 case SUPPLEMENT: 099 return "supplement"; 100 case NULL: 101 return null; 102 default: 103 return "?"; 104 } 105 } 106 107 public String getSystem() { 108 return "http://hl7.org/fhir/codesystem-content-mode"; 109 } 110 111 public String getDefinition() { 112 switch (this) { 113 case NOTPRESENT: 114 return "None of the concepts defined by the code system are included in the code system resource."; 115 case EXAMPLE: 116 return "A few representative concepts are included in the code system resource. There is no useful intent in the subset choice and there's no process to make it workable: it's not intended to be workable."; 117 case FRAGMENT: 118 return "A subset of the code system concepts are included in the code system resource. This is a curated subset released for a specific purpose under the governance of the code system steward, and that the intent, bounds and consequences of the fragmentation are clearly defined in the fragment or the code system documentation. Fragments are also known as partitions."; 119 case COMPLETE: 120 return "All the concepts defined by the code system are included in the code system resource."; 121 case SUPPLEMENT: 122 return "The resource doesn't define any new concepts; it just provides additional designations and properties to another code system."; 123 case NULL: 124 return null; 125 default: 126 return "?"; 127 } 128 } 129 130 public String getDisplay() { 131 switch (this) { 132 case NOTPRESENT: 133 return "Not Present"; 134 case EXAMPLE: 135 return "Example"; 136 case FRAGMENT: 137 return "Fragment"; 138 case COMPLETE: 139 return "Complete"; 140 case SUPPLEMENT: 141 return "Supplement"; 142 case NULL: 143 return null; 144 default: 145 return "?"; 146 } 147 } 148 149}