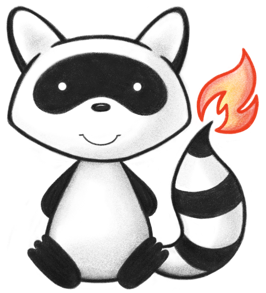
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum ClaimInformationcategory { 037 038 /** 039 * Codes conveying additional situation and condition information. 040 */ 041 INFO, 042 /** 043 * Discharge status and discharge to locations. 044 */ 045 DISCHARGE, 046 /** 047 * Period, start or end dates of aspects of the Condition. 048 */ 049 ONSET, 050 /** 051 * Nature and date of the related event e.g. Last exam, service, X-ray etc. 052 */ 053 RELATED, 054 /** 055 * Insurance policy exceptions. 056 */ 057 EXCEPTION, 058 /** 059 * Materials being forwarded, e.g. Models, molds, images, documents. 060 */ 061 MATERIAL, 062 /** 063 * Materials attached such as images, documents and resources. 064 */ 065 ATTACHMENT, 066 /** 067 * Teeth which are missing for any reason, for example: prior extraction, never 068 * developed. 069 */ 070 MISSINGTOOTH, 071 /** 072 * The type of prosthesis and date of supply if a previously supplied 073 * prosthesis. 074 */ 075 PROSTHESIS, 076 /** 077 * Other information identified by the type.system. 078 */ 079 OTHER, 080 /** 081 * An indication that the patient was hospitalized, the period if known 082 * otherwise a Yes/No (boolean). 083 */ 084 HOSPITALIZED, 085 /** 086 * An indication that the patient was unable to work, the period if known 087 * otherwise a Yes/No (boolean). 088 */ 089 EMPLOYMENTIMPACTED, 090 /** 091 * The external cause of an illness or injury. 092 */ 093 EXTERNALCAUSE, 094 /** 095 * The reason for the patient visit. 096 */ 097 PATIENTREASONFORVISIT, 098 /** 099 * added to help the parsers 100 */ 101 NULL; 102 103 public static ClaimInformationcategory fromCode(String codeString) throws FHIRException { 104 if (codeString == null || "".equals(codeString)) 105 return null; 106 if ("info".equals(codeString)) 107 return INFO; 108 if ("discharge".equals(codeString)) 109 return DISCHARGE; 110 if ("onset".equals(codeString)) 111 return ONSET; 112 if ("related".equals(codeString)) 113 return RELATED; 114 if ("exception".equals(codeString)) 115 return EXCEPTION; 116 if ("material".equals(codeString)) 117 return MATERIAL; 118 if ("attachment".equals(codeString)) 119 return ATTACHMENT; 120 if ("missingtooth".equals(codeString)) 121 return MISSINGTOOTH; 122 if ("prosthesis".equals(codeString)) 123 return PROSTHESIS; 124 if ("other".equals(codeString)) 125 return OTHER; 126 if ("hospitalized".equals(codeString)) 127 return HOSPITALIZED; 128 if ("employmentimpacted".equals(codeString)) 129 return EMPLOYMENTIMPACTED; 130 if ("externalcause".equals(codeString)) 131 return EXTERNALCAUSE; 132 if ("patientreasonforvisit".equals(codeString)) 133 return PATIENTREASONFORVISIT; 134 throw new FHIRException("Unknown ClaimInformationcategory code '" + codeString + "'"); 135 } 136 137 public String toCode() { 138 switch (this) { 139 case INFO: 140 return "info"; 141 case DISCHARGE: 142 return "discharge"; 143 case ONSET: 144 return "onset"; 145 case RELATED: 146 return "related"; 147 case EXCEPTION: 148 return "exception"; 149 case MATERIAL: 150 return "material"; 151 case ATTACHMENT: 152 return "attachment"; 153 case MISSINGTOOTH: 154 return "missingtooth"; 155 case PROSTHESIS: 156 return "prosthesis"; 157 case OTHER: 158 return "other"; 159 case HOSPITALIZED: 160 return "hospitalized"; 161 case EMPLOYMENTIMPACTED: 162 return "employmentimpacted"; 163 case EXTERNALCAUSE: 164 return "externalcause"; 165 case PATIENTREASONFORVISIT: 166 return "patientreasonforvisit"; 167 case NULL: 168 return null; 169 default: 170 return "?"; 171 } 172 } 173 174 public String getSystem() { 175 return "http://terminology.hl7.org/CodeSystem/claiminformationcategory"; 176 } 177 178 public String getDefinition() { 179 switch (this) { 180 case INFO: 181 return "Codes conveying additional situation and condition information."; 182 case DISCHARGE: 183 return "Discharge status and discharge to locations."; 184 case ONSET: 185 return "Period, start or end dates of aspects of the Condition."; 186 case RELATED: 187 return "Nature and date of the related event e.g. Last exam, service, X-ray etc."; 188 case EXCEPTION: 189 return "Insurance policy exceptions."; 190 case MATERIAL: 191 return "Materials being forwarded, e.g. Models, molds, images, documents."; 192 case ATTACHMENT: 193 return "Materials attached such as images, documents and resources."; 194 case MISSINGTOOTH: 195 return "Teeth which are missing for any reason, for example: prior extraction, never developed."; 196 case PROSTHESIS: 197 return "The type of prosthesis and date of supply if a previously supplied prosthesis."; 198 case OTHER: 199 return "Other information identified by the type.system."; 200 case HOSPITALIZED: 201 return "An indication that the patient was hospitalized, the period if known otherwise a Yes/No (boolean)."; 202 case EMPLOYMENTIMPACTED: 203 return "An indication that the patient was unable to work, the period if known otherwise a Yes/No (boolean)."; 204 case EXTERNALCAUSE: 205 return "The external cause of an illness or injury."; 206 case PATIENTREASONFORVISIT: 207 return "The reason for the patient visit."; 208 case NULL: 209 return null; 210 default: 211 return "?"; 212 } 213 } 214 215 public String getDisplay() { 216 switch (this) { 217 case INFO: 218 return "Information"; 219 case DISCHARGE: 220 return "Discharge"; 221 case ONSET: 222 return "Onset"; 223 case RELATED: 224 return "Related Services"; 225 case EXCEPTION: 226 return "Exception"; 227 case MATERIAL: 228 return "Materials Forwarded"; 229 case ATTACHMENT: 230 return "Attachment"; 231 case MISSINGTOOTH: 232 return "Missing Tooth"; 233 case PROSTHESIS: 234 return "Prosthesis"; 235 case OTHER: 236 return "Other"; 237 case HOSPITALIZED: 238 return "Hospitalized"; 239 case EMPLOYMENTIMPACTED: 240 return "EmploymentImpacted"; 241 case EXTERNALCAUSE: 242 return "External Caause"; 243 case PATIENTREASONFORVISIT: 244 return "Patient Reason for Visit"; 245 case NULL: 246 return null; 247 default: 248 return "?"; 249 } 250 } 251 252}