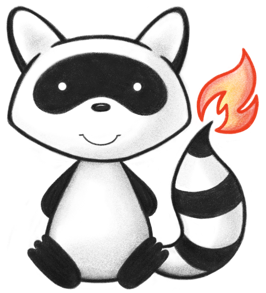
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum BenefitType { 037 038 /** 039 * Maximum benefit allowable. 040 */ 041 BENEFIT, 042 /** 043 * Cost to be incurred before benefits are applied 044 */ 045 DEDUCTIBLE, 046 /** 047 * Service visit 048 */ 049 VISIT, 050 /** 051 * Type of room 052 */ 053 ROOM, 054 /** 055 * Copayment per service 056 */ 057 COPAY, 058 /** 059 * Copayment percentage per service 060 */ 061 COPAYPERCENT, 062 /** 063 * Copayment maximum per service 064 */ 065 COPAYMAXIMUM, 066 /** 067 * Vision Exam 068 */ 069 VISIONEXAM, 070 /** 071 * Frames and lenses 072 */ 073 VISIONGLASSES, 074 /** 075 * Contact Lenses 076 */ 077 VISIONCONTACTS, 078 /** 079 * Medical Primary Health Coverage 080 */ 081 MEDICALPRIMARYCARE, 082 /** 083 * Pharmacy Dispense Coverage 084 */ 085 PHARMACYDISPENSE, 086 /** 087 * added to help the parsers 088 */ 089 NULL; 090 091 public static BenefitType fromCode(String codeString) throws FHIRException { 092 if (codeString == null || "".equals(codeString)) 093 return null; 094 if ("benefit".equals(codeString)) 095 return BENEFIT; 096 if ("deductible".equals(codeString)) 097 return DEDUCTIBLE; 098 if ("visit".equals(codeString)) 099 return VISIT; 100 if ("room".equals(codeString)) 101 return ROOM; 102 if ("copay".equals(codeString)) 103 return COPAY; 104 if ("copay-percent".equals(codeString)) 105 return COPAYPERCENT; 106 if ("copay-maximum".equals(codeString)) 107 return COPAYMAXIMUM; 108 if ("vision-exam".equals(codeString)) 109 return VISIONEXAM; 110 if ("vision-glasses".equals(codeString)) 111 return VISIONGLASSES; 112 if ("vision-contacts".equals(codeString)) 113 return VISIONCONTACTS; 114 if ("medical-primarycare".equals(codeString)) 115 return MEDICALPRIMARYCARE; 116 if ("pharmacy-dispense".equals(codeString)) 117 return PHARMACYDISPENSE; 118 throw new FHIRException("Unknown BenefitType code '" + codeString + "'"); 119 } 120 121 public String toCode() { 122 switch (this) { 123 case BENEFIT: 124 return "benefit"; 125 case DEDUCTIBLE: 126 return "deductible"; 127 case VISIT: 128 return "visit"; 129 case ROOM: 130 return "room"; 131 case COPAY: 132 return "copay"; 133 case COPAYPERCENT: 134 return "copay-percent"; 135 case COPAYMAXIMUM: 136 return "copay-maximum"; 137 case VISIONEXAM: 138 return "vision-exam"; 139 case VISIONGLASSES: 140 return "vision-glasses"; 141 case VISIONCONTACTS: 142 return "vision-contacts"; 143 case MEDICALPRIMARYCARE: 144 return "medical-primarycare"; 145 case PHARMACYDISPENSE: 146 return "pharmacy-dispense"; 147 case NULL: 148 return null; 149 default: 150 return "?"; 151 } 152 } 153 154 public String getSystem() { 155 return "http://terminology.hl7.org/CodeSystem/benefit-type"; 156 } 157 158 public String getDefinition() { 159 switch (this) { 160 case BENEFIT: 161 return "Maximum benefit allowable."; 162 case DEDUCTIBLE: 163 return "Cost to be incurred before benefits are applied"; 164 case VISIT: 165 return "Service visit"; 166 case ROOM: 167 return "Type of room"; 168 case COPAY: 169 return "Copayment per service"; 170 case COPAYPERCENT: 171 return "Copayment percentage per service"; 172 case COPAYMAXIMUM: 173 return "Copayment maximum per service"; 174 case VISIONEXAM: 175 return "Vision Exam"; 176 case VISIONGLASSES: 177 return "Frames and lenses"; 178 case VISIONCONTACTS: 179 return "Contact Lenses"; 180 case MEDICALPRIMARYCARE: 181 return "Medical Primary Health Coverage"; 182 case PHARMACYDISPENSE: 183 return "Pharmacy Dispense Coverage"; 184 case NULL: 185 return null; 186 default: 187 return "?"; 188 } 189 } 190 191 public String getDisplay() { 192 switch (this) { 193 case BENEFIT: 194 return "Benefit"; 195 case DEDUCTIBLE: 196 return "Deductible"; 197 case VISIT: 198 return "Visit"; 199 case ROOM: 200 return "Room"; 201 case COPAY: 202 return "Copayment per service"; 203 case COPAYPERCENT: 204 return "Copayment Percent per service"; 205 case COPAYMAXIMUM: 206 return "Copayment maximum per service"; 207 case VISIONEXAM: 208 return "Vision Exam"; 209 case VISIONGLASSES: 210 return "Vision Glasses"; 211 case VISIONCONTACTS: 212 return "Vision Contacts Coverage"; 213 case MEDICALPRIMARYCARE: 214 return "Medical Primary Health Coverage"; 215 case PHARMACYDISPENSE: 216 return "Pharmacy Dispense Coverage"; 217 case NULL: 218 return null; 219 default: 220 return "?"; 221 } 222 } 223 224}