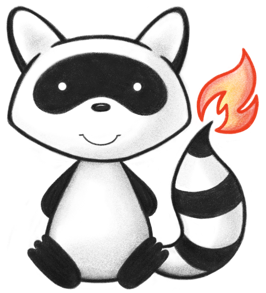
001package org.hl7.fhir.r4.model.codesystems; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Wed, Jan 30, 2019 16:19-0500 for FHIR v4.0.0 033 034import org.hl7.fhir.exceptions.FHIRException; 035 036public enum BasicResourceType { 037 038 /** 039 * An assertion of permission for an activity or set of activities to occur, 040 * possibly subject to particular limitations; e.g. surgical consent, 041 * information disclosure consent, etc. 042 */ 043 CONSENT, 044 /** 045 * A request that care of a particular type be provided to a patient. Could 046 * involve the transfer of care, a consult, etc. 047 */ 048 REFERRAL, 049 /** 050 * An undesired reaction caused by exposure to some agent (e.g. a medication, 051 * immunization, food, or environmental agent). 052 */ 053 ADVEVENT, 054 /** 055 * A request that a time be scheduled for a type of service for a specified 056 * patient, potentially subject to other constraints 057 */ 058 APTMTREQ, 059 /** 060 * The transition of a patient or set of material from one location to another 061 */ 062 TRANSFER, 063 /** 064 * The specification of a set of food and/or other nutritional material to be 065 * delivered to a patient. 066 */ 067 DIET, 068 /** 069 * An occurrence of a non-care-related event in the healthcare domain, such as 070 * approvals, reviews, etc. 071 */ 072 ADMINACT, 073 /** 074 * Record of a situation where a subject was exposed to a substance. Usually of 075 * interest to public health. 076 */ 077 EXPOSURE, 078 /** 079 * A formalized inquiry into the circumstances surrounding a particular 080 * unplanned event or potential event for the purposes of identifying possible 081 * causes and contributing factors for the event 082 */ 083 INVESTIGATION, 084 /** 085 * A financial instrument used to track costs, charges or other amounts. 086 */ 087 ACCOUNT, 088 /** 089 * A request for payment for goods and/or services. Includes the idea of a 090 * healthcare insurance claim. 091 */ 092 INVOICE, 093 /** 094 * The determination of what will be paid against a particular invoice based on 095 * coverage, plan rules, etc. 096 */ 097 ADJUDICAT, 098 /** 099 * A request for a pre-determination of the cost that would be paid under an 100 * insurance plan for a hypothetical claim for goods or services 101 */ 102 PREDETREQ, 103 /** 104 * An adjudication of what would be paid under an insurance plan for a 105 * hypothetical claim for goods or services 106 */ 107 PREDETERMINE, 108 /** 109 * An investigation to determine information about a particular therapy or 110 * product 111 */ 112 STUDY, 113 /** 114 * A set of (possibly conditional) steps to be taken to achieve some aim. 115 * Includes study protocols, treatment protocols, emergency protocols, etc. 116 */ 117 PROTOCOL, 118 /** 119 * added to help the parsers 120 */ 121 NULL; 122 123 public static BasicResourceType fromCode(String codeString) throws FHIRException { 124 if (codeString == null || "".equals(codeString)) 125 return null; 126 if ("consent".equals(codeString)) 127 return CONSENT; 128 if ("referral".equals(codeString)) 129 return REFERRAL; 130 if ("advevent".equals(codeString)) 131 return ADVEVENT; 132 if ("aptmtreq".equals(codeString)) 133 return APTMTREQ; 134 if ("transfer".equals(codeString)) 135 return TRANSFER; 136 if ("diet".equals(codeString)) 137 return DIET; 138 if ("adminact".equals(codeString)) 139 return ADMINACT; 140 if ("exposure".equals(codeString)) 141 return EXPOSURE; 142 if ("investigation".equals(codeString)) 143 return INVESTIGATION; 144 if ("account".equals(codeString)) 145 return ACCOUNT; 146 if ("invoice".equals(codeString)) 147 return INVOICE; 148 if ("adjudicat".equals(codeString)) 149 return ADJUDICAT; 150 if ("predetreq".equals(codeString)) 151 return PREDETREQ; 152 if ("predetermine".equals(codeString)) 153 return PREDETERMINE; 154 if ("study".equals(codeString)) 155 return STUDY; 156 if ("protocol".equals(codeString)) 157 return PROTOCOL; 158 throw new FHIRException("Unknown BasicResourceType code '" + codeString + "'"); 159 } 160 161 public String toCode() { 162 switch (this) { 163 case CONSENT: 164 return "consent"; 165 case REFERRAL: 166 return "referral"; 167 case ADVEVENT: 168 return "advevent"; 169 case APTMTREQ: 170 return "aptmtreq"; 171 case TRANSFER: 172 return "transfer"; 173 case DIET: 174 return "diet"; 175 case ADMINACT: 176 return "adminact"; 177 case EXPOSURE: 178 return "exposure"; 179 case INVESTIGATION: 180 return "investigation"; 181 case ACCOUNT: 182 return "account"; 183 case INVOICE: 184 return "invoice"; 185 case ADJUDICAT: 186 return "adjudicat"; 187 case PREDETREQ: 188 return "predetreq"; 189 case PREDETERMINE: 190 return "predetermine"; 191 case STUDY: 192 return "study"; 193 case PROTOCOL: 194 return "protocol"; 195 case NULL: 196 return null; 197 default: 198 return "?"; 199 } 200 } 201 202 public String getSystem() { 203 return "http://terminology.hl7.org/CodeSystem/basic-resource-type"; 204 } 205 206 public String getDefinition() { 207 switch (this) { 208 case CONSENT: 209 return "An assertion of permission for an activity or set of activities to occur, possibly subject to particular limitations; e.g. surgical consent, information disclosure consent, etc."; 210 case REFERRAL: 211 return "A request that care of a particular type be provided to a patient. Could involve the transfer of care, a consult, etc."; 212 case ADVEVENT: 213 return "An undesired reaction caused by exposure to some agent (e.g. a medication, immunization, food, or environmental agent)."; 214 case APTMTREQ: 215 return "A request that a time be scheduled for a type of service for a specified patient, potentially subject to other constraints"; 216 case TRANSFER: 217 return "The transition of a patient or set of material from one location to another"; 218 case DIET: 219 return "The specification of a set of food and/or other nutritional material to be delivered to a patient."; 220 case ADMINACT: 221 return "An occurrence of a non-care-related event in the healthcare domain, such as approvals, reviews, etc."; 222 case EXPOSURE: 223 return "Record of a situation where a subject was exposed to a substance. Usually of interest to public health."; 224 case INVESTIGATION: 225 return "A formalized inquiry into the circumstances surrounding a particular unplanned event or potential event for the purposes of identifying possible causes and contributing factors for the event"; 226 case ACCOUNT: 227 return "A financial instrument used to track costs, charges or other amounts."; 228 case INVOICE: 229 return "A request for payment for goods and/or services. Includes the idea of a healthcare insurance claim."; 230 case ADJUDICAT: 231 return "The determination of what will be paid against a particular invoice based on coverage, plan rules, etc."; 232 case PREDETREQ: 233 return "A request for a pre-determination of the cost that would be paid under an insurance plan for a hypothetical claim for goods or services"; 234 case PREDETERMINE: 235 return "An adjudication of what would be paid under an insurance plan for a hypothetical claim for goods or services"; 236 case STUDY: 237 return "An investigation to determine information about a particular therapy or product"; 238 case PROTOCOL: 239 return "A set of (possibly conditional) steps to be taken to achieve some aim. Includes study protocols, treatment protocols, emergency protocols, etc."; 240 case NULL: 241 return null; 242 default: 243 return "?"; 244 } 245 } 246 247 public String getDisplay() { 248 switch (this) { 249 case CONSENT: 250 return "Consent"; 251 case REFERRAL: 252 return "Referral"; 253 case ADVEVENT: 254 return "Adverse Event"; 255 case APTMTREQ: 256 return "Appointment Request"; 257 case TRANSFER: 258 return "Transfer"; 259 case DIET: 260 return "Diet"; 261 case ADMINACT: 262 return "Administrative Activity"; 263 case EXPOSURE: 264 return "Exposure"; 265 case INVESTIGATION: 266 return "Investigation"; 267 case ACCOUNT: 268 return "Account"; 269 case INVOICE: 270 return "Invoice"; 271 case ADJUDICAT: 272 return "Invoice Adjudication"; 273 case PREDETREQ: 274 return "Pre-determination Request"; 275 case PREDETERMINE: 276 return "Predetermination"; 277 case STUDY: 278 return "Study"; 279 case PROTOCOL: 280 return "Protocol"; 281 case NULL: 282 return null; 283 default: 284 return "?"; 285 } 286 } 287 288}