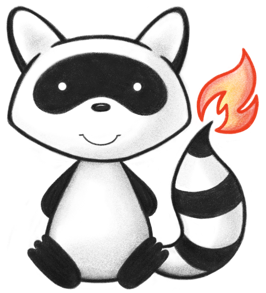
001package org.hl7.fhir.r4.model; 002 003import org.hl7.fhir.utilities.Utilities; 004 005import ca.uhn.fhir.model.api.TemporalPrecisionEnum; 006 007/* 008 Copyright (c) 2011+, HL7, Inc. 009 All rights reserved. 010 011 Redistribution and use in source and binary forms, with or without modification, 012 are permitted provided that the following conditions are met: 013 014 * Redistributions of source code must retain the above copyright notice, this 015 list of conditions and the following disclaimer. 016 * Redistributions in binary form must reproduce the above copyright notice, 017 this list of conditions and the following disclaimer in the documentation 018 and/or other materials provided with the distribution. 019 * Neither the name of HL7 nor the names of its contributors may be used to 020 endorse or promote products derived from this software without specific 021 prior written permission. 022 023 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 024 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 025 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 026 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 027 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 028 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 029 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 030 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 031 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 032 POSSIBILITY OF SUCH DAMAGE. 033 034 */ 035 036import ca.uhn.fhir.model.api.annotation.DatatypeDef; 037 038/** 039 * Represents a Time datatype, per the FHIR specification. A time is a 040 * specification of hours and minutes (and optionally milliseconds), with NO 041 * date and NO timezone information attached. It is expressed as a string in the 042 * form <code>HH:mm:ss[.SSSS]</code> 043 */ 044@DatatypeDef(name = "time") 045public class TimeType extends PrimitiveType<String> { 046 047 private static final long serialVersionUID = 3L; 048 049 /** 050 * Constructor 051 */ 052 public TimeType() { 053 // nothing 054 } 055 056 /** 057 * Constructor 058 */ 059 public TimeType(String theValue) { 060 setValue(theValue); 061 } 062 063 @Override 064 protected String parse(String theValue) { 065 return theValue; 066 } 067 068 @Override 069 protected String encode(String theValue) { 070 return theValue; 071 } 072 073 @Override 074 public TimeType copy() { 075 TimeType ret = new TimeType(getValue()); 076 copyValues(ret); 077 return ret; 078 } 079 080 public String fhirType() { 081 return "time"; 082 } 083 084 public int getHour() { 085 String v = getValue(); 086 if (v.length() < 2) { 087 return 0; 088 } 089 v = v.substring(0, 2); 090 if (!Utilities.isInteger(v)) { 091 return 0; 092 } 093 return Integer.parseInt(v); 094 } 095 096 public int getMinute() { 097 String v = getValue(); 098 if (v.length() < 5) { 099 return 0; 100 } 101 v = v.substring(3, 5); 102 if (!Utilities.isInteger(v)) { 103 return 0; 104 } 105 return Integer.parseInt(v); 106 } 107 108 public float getSecond() { 109 String v = getValue(); 110 if (v.length() < 8) { 111 return 0; 112 } 113 v = v.substring(6); 114 if (!Utilities.isDecimal(v, false, true)) { 115 return 0; 116 } 117 return Float.parseFloat(v); 118 } 119 120 public TemporalPrecisionEnum getPrecision() { 121 String v = getValue(); 122// if (v.length() == 2) { 123// return TemporalPrecisionEnum.HOUR; 124// } 125 if (v.length() == 5) { 126 return TemporalPrecisionEnum.MINUTE; 127 } 128 if (v.length() == 8) { 129 return TemporalPrecisionEnum.SECOND; 130 } 131 if (v.length() > 9) { 132 return TemporalPrecisionEnum.MILLI; 133 } 134 135 return null; 136 } 137 138 public void setPrecision(TemporalPrecisionEnum temp) { 139 if (temp == TemporalPrecisionEnum.MINUTE) { 140 setValue(getValue().substring(0, 5)); 141 } 142 if (temp == TemporalPrecisionEnum.SECOND) { 143 setValue(getValue().substring(0, 8)); 144 } 145 } 146 147 @Override 148 public String fpValue() { 149 return "@T" + primitiveValue(); 150 } 151}