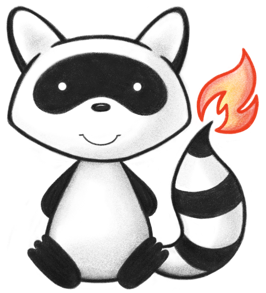
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.ICompositeType; 039import org.hl7.fhir.utilities.Utilities; 040 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.DatatypeDef; 043import ca.uhn.fhir.model.api.annotation.Description; 044 045/** 046 * A signature along with supporting context. The signature may be a digital 047 * signature that is cryptographic in nature, or some other signature acceptable 048 * to the domain. This other signature may be as simple as a graphical image 049 * representing a hand-written signature, or a signature ceremony Different 050 * signature approaches have different utilities. 051 */ 052@DatatypeDef(name = "Signature") 053public class Signature extends Type implements ICompositeType { 054 055 /** 056 * An indication of the reason that the entity signed this document. This may be 057 * explicitly included as part of the signature information and can be used when 058 * determining accountability for various actions concerning the document. 059 */ 060 @Child(name = "type", type = { 061 Coding.class }, order = 0, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 062 @Description(shortDefinition = "Indication of the reason the entity signed the object(s)", formalDefinition = "An indication of the reason that the entity signed this document. This may be explicitly included as part of the signature information and can be used when determining accountability for various actions concerning the document.") 063 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/signature-type") 064 protected List<Coding> type; 065 066 /** 067 * When the digital signature was signed. 068 */ 069 @Child(name = "when", type = { InstantType.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 070 @Description(shortDefinition = "When the signature was created", formalDefinition = "When the digital signature was signed.") 071 protected InstantType when; 072 073 /** 074 * A reference to an application-usable description of the identity that signed 075 * (e.g. the signature used their private key). 076 */ 077 @Child(name = "who", type = { Practitioner.class, PractitionerRole.class, RelatedPerson.class, Patient.class, 078 Device.class, Organization.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 079 @Description(shortDefinition = "Who signed", formalDefinition = "A reference to an application-usable description of the identity that signed (e.g. the signature used their private key).") 080 protected Reference who; 081 082 /** 083 * The actual object that is the target of the reference (A reference to an 084 * application-usable description of the identity that signed (e.g. the 085 * signature used their private key).) 086 */ 087 protected Resource whoTarget; 088 089 /** 090 * A reference to an application-usable description of the identity that is 091 * represented by the signature. 092 */ 093 @Child(name = "onBehalfOf", type = { Practitioner.class, PractitionerRole.class, RelatedPerson.class, Patient.class, 094 Device.class, Organization.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 095 @Description(shortDefinition = "The party represented", formalDefinition = "A reference to an application-usable description of the identity that is represented by the signature.") 096 protected Reference onBehalfOf; 097 098 /** 099 * The actual object that is the target of the reference (A reference to an 100 * application-usable description of the identity that is represented by the 101 * signature.) 102 */ 103 protected Resource onBehalfOfTarget; 104 105 /** 106 * A mime type that indicates the technical format of the target resources 107 * signed by the signature. 108 */ 109 @Child(name = "targetFormat", type = { 110 CodeType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 111 @Description(shortDefinition = "The technical format of the signed resources", formalDefinition = "A mime type that indicates the technical format of the target resources signed by the signature.") 112 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/mimetypes") 113 protected CodeType targetFormat; 114 115 /** 116 * A mime type that indicates the technical format of the signature. Important 117 * mime types are application/signature+xml for X ML DigSig, application/jose 118 * for JWS, and image/* for a graphical image of a signature, etc. 119 */ 120 @Child(name = "sigFormat", type = { CodeType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 121 @Description(shortDefinition = "The technical format of the signature", formalDefinition = "A mime type that indicates the technical format of the signature. Important mime types are application/signature+xml for X ML DigSig, application/jose for JWS, and image/* for a graphical image of a signature, etc.") 122 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/mimetypes") 123 protected CodeType sigFormat; 124 125 /** 126 * The base64 encoding of the Signature content. When signature is not recorded 127 * electronically this element would be empty. 128 */ 129 @Child(name = "data", type = { 130 Base64BinaryType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 131 @Description(shortDefinition = "The actual signature content (XML DigSig. JWS, picture, etc.)", formalDefinition = "The base64 encoding of the Signature content. When signature is not recorded electronically this element would be empty.") 132 protected Base64BinaryType data; 133 134 private static final long serialVersionUID = 1587325823L; 135 136 /** 137 * Constructor 138 */ 139 public Signature() { 140 super(); 141 } 142 143 /** 144 * Constructor 145 */ 146 public Signature(InstantType when, Reference who) { 147 super(); 148 this.when = when; 149 this.who = who; 150 } 151 152 /** 153 * @return {@link #type} (An indication of the reason that the entity signed 154 * this document. This may be explicitly included as part of the 155 * signature information and can be used when determining accountability 156 * for various actions concerning the document.) 157 */ 158 public List<Coding> getType() { 159 if (this.type == null) 160 this.type = new ArrayList<Coding>(); 161 return this.type; 162 } 163 164 /** 165 * @return Returns a reference to <code>this</code> for easy method chaining 166 */ 167 public Signature setType(List<Coding> theType) { 168 this.type = theType; 169 return this; 170 } 171 172 public boolean hasType() { 173 if (this.type == null) 174 return false; 175 for (Coding item : this.type) 176 if (!item.isEmpty()) 177 return true; 178 return false; 179 } 180 181 public Coding addType() { // 3 182 Coding t = new Coding(); 183 if (this.type == null) 184 this.type = new ArrayList<Coding>(); 185 this.type.add(t); 186 return t; 187 } 188 189 public Signature addType(Coding t) { // 3 190 if (t == null) 191 return this; 192 if (this.type == null) 193 this.type = new ArrayList<Coding>(); 194 this.type.add(t); 195 return this; 196 } 197 198 /** 199 * @return The first repetition of repeating field {@link #type}, creating it if 200 * it does not already exist 201 */ 202 public Coding getTypeFirstRep() { 203 if (getType().isEmpty()) { 204 addType(); 205 } 206 return getType().get(0); 207 } 208 209 /** 210 * @return {@link #when} (When the digital signature was signed.). This is the 211 * underlying object with id, value and extensions. The accessor 212 * "getWhen" gives direct access to the value 213 */ 214 public InstantType getWhenElement() { 215 if (this.when == null) 216 if (Configuration.errorOnAutoCreate()) 217 throw new Error("Attempt to auto-create Signature.when"); 218 else if (Configuration.doAutoCreate()) 219 this.when = new InstantType(); // bb 220 return this.when; 221 } 222 223 public boolean hasWhenElement() { 224 return this.when != null && !this.when.isEmpty(); 225 } 226 227 public boolean hasWhen() { 228 return this.when != null && !this.when.isEmpty(); 229 } 230 231 /** 232 * @param value {@link #when} (When the digital signature was signed.). This is 233 * the underlying object with id, value and extensions. The 234 * accessor "getWhen" gives direct access to the value 235 */ 236 public Signature setWhenElement(InstantType value) { 237 this.when = value; 238 return this; 239 } 240 241 /** 242 * @return When the digital signature was signed. 243 */ 244 public Date getWhen() { 245 return this.when == null ? null : this.when.getValue(); 246 } 247 248 /** 249 * @param value When the digital signature was signed. 250 */ 251 public Signature setWhen(Date value) { 252 if (this.when == null) 253 this.when = new InstantType(); 254 this.when.setValue(value); 255 return this; 256 } 257 258 /** 259 * @return {@link #who} (A reference to an application-usable description of the 260 * identity that signed (e.g. the signature used their private key).) 261 */ 262 public Reference getWho() { 263 if (this.who == null) 264 if (Configuration.errorOnAutoCreate()) 265 throw new Error("Attempt to auto-create Signature.who"); 266 else if (Configuration.doAutoCreate()) 267 this.who = new Reference(); // cc 268 return this.who; 269 } 270 271 public boolean hasWho() { 272 return this.who != null && !this.who.isEmpty(); 273 } 274 275 /** 276 * @param value {@link #who} (A reference to an application-usable description 277 * of the identity that signed (e.g. the signature used their 278 * private key).) 279 */ 280 public Signature setWho(Reference value) { 281 this.who = value; 282 return this; 283 } 284 285 /** 286 * @return {@link #who} The actual object that is the target of the reference. 287 * The reference library doesn't populate this, but you can use it to 288 * hold the resource if you resolve it. (A reference to an 289 * application-usable description of the identity that signed (e.g. the 290 * signature used their private key).) 291 */ 292 public Resource getWhoTarget() { 293 return this.whoTarget; 294 } 295 296 /** 297 * @param value {@link #who} The actual object that is the target of the 298 * reference. The reference library doesn't use these, but you can 299 * use it to hold the resource if you resolve it. (A reference to 300 * an application-usable description of the identity that signed 301 * (e.g. the signature used their private key).) 302 */ 303 public Signature setWhoTarget(Resource value) { 304 this.whoTarget = value; 305 return this; 306 } 307 308 /** 309 * @return {@link #onBehalfOf} (A reference to an application-usable description 310 * of the identity that is represented by the signature.) 311 */ 312 public Reference getOnBehalfOf() { 313 if (this.onBehalfOf == null) 314 if (Configuration.errorOnAutoCreate()) 315 throw new Error("Attempt to auto-create Signature.onBehalfOf"); 316 else if (Configuration.doAutoCreate()) 317 this.onBehalfOf = new Reference(); // cc 318 return this.onBehalfOf; 319 } 320 321 public boolean hasOnBehalfOf() { 322 return this.onBehalfOf != null && !this.onBehalfOf.isEmpty(); 323 } 324 325 /** 326 * @param value {@link #onBehalfOf} (A reference to an application-usable 327 * description of the identity that is represented by the 328 * signature.) 329 */ 330 public Signature setOnBehalfOf(Reference value) { 331 this.onBehalfOf = value; 332 return this; 333 } 334 335 /** 336 * @return {@link #onBehalfOf} The actual object that is the target of the 337 * reference. The reference library doesn't populate this, but you can 338 * use it to hold the resource if you resolve it. (A reference to an 339 * application-usable description of the identity that is represented by 340 * the signature.) 341 */ 342 public Resource getOnBehalfOfTarget() { 343 return this.onBehalfOfTarget; 344 } 345 346 /** 347 * @param value {@link #onBehalfOf} The actual object that is the target of the 348 * reference. The reference library doesn't use these, but you can 349 * use it to hold the resource if you resolve it. (A reference to 350 * an application-usable description of the identity that is 351 * represented by the signature.) 352 */ 353 public Signature setOnBehalfOfTarget(Resource value) { 354 this.onBehalfOfTarget = value; 355 return this; 356 } 357 358 /** 359 * @return {@link #targetFormat} (A mime type that indicates the technical 360 * format of the target resources signed by the signature.). This is the 361 * underlying object with id, value and extensions. The accessor 362 * "getTargetFormat" gives direct access to the value 363 */ 364 public CodeType getTargetFormatElement() { 365 if (this.targetFormat == null) 366 if (Configuration.errorOnAutoCreate()) 367 throw new Error("Attempt to auto-create Signature.targetFormat"); 368 else if (Configuration.doAutoCreate()) 369 this.targetFormat = new CodeType(); // bb 370 return this.targetFormat; 371 } 372 373 public boolean hasTargetFormatElement() { 374 return this.targetFormat != null && !this.targetFormat.isEmpty(); 375 } 376 377 public boolean hasTargetFormat() { 378 return this.targetFormat != null && !this.targetFormat.isEmpty(); 379 } 380 381 /** 382 * @param value {@link #targetFormat} (A mime type that indicates the technical 383 * format of the target resources signed by the signature.). This 384 * is the underlying object with id, value and extensions. The 385 * accessor "getTargetFormat" gives direct access to the value 386 */ 387 public Signature setTargetFormatElement(CodeType value) { 388 this.targetFormat = value; 389 return this; 390 } 391 392 /** 393 * @return A mime type that indicates the technical format of the target 394 * resources signed by the signature. 395 */ 396 public String getTargetFormat() { 397 return this.targetFormat == null ? null : this.targetFormat.getValue(); 398 } 399 400 /** 401 * @param value A mime type that indicates the technical format of the target 402 * resources signed by the signature. 403 */ 404 public Signature setTargetFormat(String value) { 405 if (Utilities.noString(value)) 406 this.targetFormat = null; 407 else { 408 if (this.targetFormat == null) 409 this.targetFormat = new CodeType(); 410 this.targetFormat.setValue(value); 411 } 412 return this; 413 } 414 415 /** 416 * @return {@link #sigFormat} (A mime type that indicates the technical format 417 * of the signature. Important mime types are application/signature+xml 418 * for X ML DigSig, application/jose for JWS, and image/* for a 419 * graphical image of a signature, etc.). This is the underlying object 420 * with id, value and extensions. The accessor "getSigFormat" gives 421 * direct access to the value 422 */ 423 public CodeType getSigFormatElement() { 424 if (this.sigFormat == null) 425 if (Configuration.errorOnAutoCreate()) 426 throw new Error("Attempt to auto-create Signature.sigFormat"); 427 else if (Configuration.doAutoCreate()) 428 this.sigFormat = new CodeType(); // bb 429 return this.sigFormat; 430 } 431 432 public boolean hasSigFormatElement() { 433 return this.sigFormat != null && !this.sigFormat.isEmpty(); 434 } 435 436 public boolean hasSigFormat() { 437 return this.sigFormat != null && !this.sigFormat.isEmpty(); 438 } 439 440 /** 441 * @param value {@link #sigFormat} (A mime type that indicates the technical 442 * format of the signature. Important mime types are 443 * application/signature+xml for X ML DigSig, application/jose for 444 * JWS, and image/* for a graphical image of a signature, etc.). 445 * This is the underlying object with id, value and extensions. The 446 * accessor "getSigFormat" gives direct access to the value 447 */ 448 public Signature setSigFormatElement(CodeType value) { 449 this.sigFormat = value; 450 return this; 451 } 452 453 /** 454 * @return A mime type that indicates the technical format of the signature. 455 * Important mime types are application/signature+xml for X ML DigSig, 456 * application/jose for JWS, and image/* for a graphical image of a 457 * signature, etc. 458 */ 459 public String getSigFormat() { 460 return this.sigFormat == null ? null : this.sigFormat.getValue(); 461 } 462 463 /** 464 * @param value A mime type that indicates the technical format of the 465 * signature. Important mime types are application/signature+xml 466 * for X ML DigSig, application/jose for JWS, and image/* for a 467 * graphical image of a signature, etc. 468 */ 469 public Signature setSigFormat(String value) { 470 if (Utilities.noString(value)) 471 this.sigFormat = null; 472 else { 473 if (this.sigFormat == null) 474 this.sigFormat = new CodeType(); 475 this.sigFormat.setValue(value); 476 } 477 return this; 478 } 479 480 /** 481 * @return {@link #data} (The base64 encoding of the Signature content. When 482 * signature is not recorded electronically this element would be 483 * empty.). This is the underlying object with id, value and extensions. 484 * The accessor "getData" gives direct access to the value 485 */ 486 public Base64BinaryType getDataElement() { 487 if (this.data == null) 488 if (Configuration.errorOnAutoCreate()) 489 throw new Error("Attempt to auto-create Signature.data"); 490 else if (Configuration.doAutoCreate()) 491 this.data = new Base64BinaryType(); // bb 492 return this.data; 493 } 494 495 public boolean hasDataElement() { 496 return this.data != null && !this.data.isEmpty(); 497 } 498 499 public boolean hasData() { 500 return this.data != null && !this.data.isEmpty(); 501 } 502 503 /** 504 * @param value {@link #data} (The base64 encoding of the Signature content. 505 * When signature is not recorded electronically this element would 506 * be empty.). This is the underlying object with id, value and 507 * extensions. The accessor "getData" gives direct access to the 508 * value 509 */ 510 public Signature setDataElement(Base64BinaryType value) { 511 this.data = value; 512 return this; 513 } 514 515 /** 516 * @return The base64 encoding of the Signature content. When signature is not 517 * recorded electronically this element would be empty. 518 */ 519 public byte[] getData() { 520 return this.data == null ? null : this.data.getValue(); 521 } 522 523 /** 524 * @param value The base64 encoding of the Signature content. When signature is 525 * not recorded electronically this element would be empty. 526 */ 527 public Signature setData(byte[] value) { 528 if (value == null) 529 this.data = null; 530 else { 531 if (this.data == null) 532 this.data = new Base64BinaryType(); 533 this.data.setValue(value); 534 } 535 return this; 536 } 537 538 protected void listChildren(List<Property> children) { 539 super.listChildren(children); 540 children.add(new Property("type", "Coding", 541 "An indication of the reason that the entity signed this document. This may be explicitly included as part of the signature information and can be used when determining accountability for various actions concerning the document.", 542 0, java.lang.Integer.MAX_VALUE, type)); 543 children.add(new Property("when", "instant", "When the digital signature was signed.", 0, 1, when)); 544 children.add(new Property("who", 545 "Reference(Practitioner|PractitionerRole|RelatedPerson|Patient|Device|Organization)", 546 "A reference to an application-usable description of the identity that signed (e.g. the signature used their private key).", 547 0, 1, who)); 548 children.add( 549 new Property("onBehalfOf", "Reference(Practitioner|PractitionerRole|RelatedPerson|Patient|Device|Organization)", 550 "A reference to an application-usable description of the identity that is represented by the signature.", 0, 551 1, onBehalfOf)); 552 children.add(new Property("targetFormat", "code", 553 "A mime type that indicates the technical format of the target resources signed by the signature.", 0, 1, 554 targetFormat)); 555 children.add(new Property("sigFormat", "code", 556 "A mime type that indicates the technical format of the signature. Important mime types are application/signature+xml for X ML DigSig, application/jose for JWS, and image/* for a graphical image of a signature, etc.", 557 0, 1, sigFormat)); 558 children.add(new Property("data", "base64Binary", 559 "The base64 encoding of the Signature content. When signature is not recorded electronically this element would be empty.", 560 0, 1, data)); 561 } 562 563 @Override 564 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 565 switch (_hash) { 566 case 3575610: 567 /* type */ return new Property("type", "Coding", 568 "An indication of the reason that the entity signed this document. This may be explicitly included as part of the signature information and can be used when determining accountability for various actions concerning the document.", 569 0, java.lang.Integer.MAX_VALUE, type); 570 case 3648314: 571 /* when */ return new Property("when", "instant", "When the digital signature was signed.", 0, 1, when); 572 case 117694: 573 /* who */ return new Property("who", 574 "Reference(Practitioner|PractitionerRole|RelatedPerson|Patient|Device|Organization)", 575 "A reference to an application-usable description of the identity that signed (e.g. the signature used their private key).", 576 0, 1, who); 577 case -14402964: 578 /* onBehalfOf */ return new Property("onBehalfOf", 579 "Reference(Practitioner|PractitionerRole|RelatedPerson|Patient|Device|Organization)", 580 "A reference to an application-usable description of the identity that is represented by the signature.", 0, 581 1, onBehalfOf); 582 case -917363480: 583 /* targetFormat */ return new Property("targetFormat", "code", 584 "A mime type that indicates the technical format of the target resources signed by the signature.", 0, 1, 585 targetFormat); 586 case -58720216: 587 /* sigFormat */ return new Property("sigFormat", "code", 588 "A mime type that indicates the technical format of the signature. Important mime types are application/signature+xml for X ML DigSig, application/jose for JWS, and image/* for a graphical image of a signature, etc.", 589 0, 1, sigFormat); 590 case 3076010: 591 /* data */ return new Property("data", "base64Binary", 592 "The base64 encoding of the Signature content. When signature is not recorded electronically this element would be empty.", 593 0, 1, data); 594 default: 595 return super.getNamedProperty(_hash, _name, _checkValid); 596 } 597 598 } 599 600 @Override 601 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 602 switch (hash) { 603 case 3575610: 604 /* type */ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // Coding 605 case 3648314: 606 /* when */ return this.when == null ? new Base[0] : new Base[] { this.when }; // InstantType 607 case 117694: 608 /* who */ return this.who == null ? new Base[0] : new Base[] { this.who }; // Reference 609 case -14402964: 610 /* onBehalfOf */ return this.onBehalfOf == null ? new Base[0] : new Base[] { this.onBehalfOf }; // Reference 611 case -917363480: 612 /* targetFormat */ return this.targetFormat == null ? new Base[0] : new Base[] { this.targetFormat }; // CodeType 613 case -58720216: 614 /* sigFormat */ return this.sigFormat == null ? new Base[0] : new Base[] { this.sigFormat }; // CodeType 615 case 3076010: 616 /* data */ return this.data == null ? new Base[0] : new Base[] { this.data }; // Base64BinaryType 617 default: 618 return super.getProperty(hash, name, checkValid); 619 } 620 621 } 622 623 @Override 624 public Base setProperty(int hash, String name, Base value) throws FHIRException { 625 switch (hash) { 626 case 3575610: // type 627 this.getType().add(castToCoding(value)); // Coding 628 return value; 629 case 3648314: // when 630 this.when = castToInstant(value); // InstantType 631 return value; 632 case 117694: // who 633 this.who = castToReference(value); // Reference 634 return value; 635 case -14402964: // onBehalfOf 636 this.onBehalfOf = castToReference(value); // Reference 637 return value; 638 case -917363480: // targetFormat 639 this.targetFormat = castToCode(value); // CodeType 640 return value; 641 case -58720216: // sigFormat 642 this.sigFormat = castToCode(value); // CodeType 643 return value; 644 case 3076010: // data 645 this.data = castToBase64Binary(value); // Base64BinaryType 646 return value; 647 default: 648 return super.setProperty(hash, name, value); 649 } 650 651 } 652 653 @Override 654 public Base setProperty(String name, Base value) throws FHIRException { 655 if (name.equals("type")) { 656 this.getType().add(castToCoding(value)); 657 } else if (name.equals("when")) { 658 this.when = castToInstant(value); // InstantType 659 } else if (name.equals("who")) { 660 this.who = castToReference(value); // Reference 661 } else if (name.equals("onBehalfOf")) { 662 this.onBehalfOf = castToReference(value); // Reference 663 } else if (name.equals("targetFormat")) { 664 this.targetFormat = castToCode(value); // CodeType 665 } else if (name.equals("sigFormat")) { 666 this.sigFormat = castToCode(value); // CodeType 667 } else if (name.equals("data")) { 668 this.data = castToBase64Binary(value); // Base64BinaryType 669 } else 670 return super.setProperty(name, value); 671 return value; 672 } 673 674 @Override 675 public Base makeProperty(int hash, String name) throws FHIRException { 676 switch (hash) { 677 case 3575610: 678 return addType(); 679 case 3648314: 680 return getWhenElement(); 681 case 117694: 682 return getWho(); 683 case -14402964: 684 return getOnBehalfOf(); 685 case -917363480: 686 return getTargetFormatElement(); 687 case -58720216: 688 return getSigFormatElement(); 689 case 3076010: 690 return getDataElement(); 691 default: 692 return super.makeProperty(hash, name); 693 } 694 695 } 696 697 @Override 698 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 699 switch (hash) { 700 case 3575610: 701 /* type */ return new String[] { "Coding" }; 702 case 3648314: 703 /* when */ return new String[] { "instant" }; 704 case 117694: 705 /* who */ return new String[] { "Reference" }; 706 case -14402964: 707 /* onBehalfOf */ return new String[] { "Reference" }; 708 case -917363480: 709 /* targetFormat */ return new String[] { "code" }; 710 case -58720216: 711 /* sigFormat */ return new String[] { "code" }; 712 case 3076010: 713 /* data */ return new String[] { "base64Binary" }; 714 default: 715 return super.getTypesForProperty(hash, name); 716 } 717 718 } 719 720 @Override 721 public Base addChild(String name) throws FHIRException { 722 if (name.equals("type")) { 723 return addType(); 724 } else if (name.equals("when")) { 725 throw new FHIRException("Cannot call addChild on a singleton property Signature.when"); 726 } else if (name.equals("who")) { 727 this.who = new Reference(); 728 return this.who; 729 } else if (name.equals("onBehalfOf")) { 730 this.onBehalfOf = new Reference(); 731 return this.onBehalfOf; 732 } else if (name.equals("targetFormat")) { 733 throw new FHIRException("Cannot call addChild on a singleton property Signature.targetFormat"); 734 } else if (name.equals("sigFormat")) { 735 throw new FHIRException("Cannot call addChild on a singleton property Signature.sigFormat"); 736 } else if (name.equals("data")) { 737 throw new FHIRException("Cannot call addChild on a singleton property Signature.data"); 738 } else 739 return super.addChild(name); 740 } 741 742 public String fhirType() { 743 return "Signature"; 744 745 } 746 747 public Signature copy() { 748 Signature dst = new Signature(); 749 copyValues(dst); 750 return dst; 751 } 752 753 public void copyValues(Signature dst) { 754 super.copyValues(dst); 755 if (type != null) { 756 dst.type = new ArrayList<Coding>(); 757 for (Coding i : type) 758 dst.type.add(i.copy()); 759 } 760 ; 761 dst.when = when == null ? null : when.copy(); 762 dst.who = who == null ? null : who.copy(); 763 dst.onBehalfOf = onBehalfOf == null ? null : onBehalfOf.copy(); 764 dst.targetFormat = targetFormat == null ? null : targetFormat.copy(); 765 dst.sigFormat = sigFormat == null ? null : sigFormat.copy(); 766 dst.data = data == null ? null : data.copy(); 767 } 768 769 protected Signature typedCopy() { 770 return copy(); 771 } 772 773 @Override 774 public boolean equalsDeep(Base other_) { 775 if (!super.equalsDeep(other_)) 776 return false; 777 if (!(other_ instanceof Signature)) 778 return false; 779 Signature o = (Signature) other_; 780 return compareDeep(type, o.type, true) && compareDeep(when, o.when, true) && compareDeep(who, o.who, true) 781 && compareDeep(onBehalfOf, o.onBehalfOf, true) && compareDeep(targetFormat, o.targetFormat, true) 782 && compareDeep(sigFormat, o.sigFormat, true) && compareDeep(data, o.data, true); 783 } 784 785 @Override 786 public boolean equalsShallow(Base other_) { 787 if (!super.equalsShallow(other_)) 788 return false; 789 if (!(other_ instanceof Signature)) 790 return false; 791 Signature o = (Signature) other_; 792 return compareValues(when, o.when, true) && compareValues(targetFormat, o.targetFormat, true) 793 && compareValues(sigFormat, o.sigFormat, true) && compareValues(data, o.data, true); 794 } 795 796 public boolean isEmpty() { 797 return super.isEmpty() 798 && ca.uhn.fhir.util.ElementUtil.isEmpty(type, when, who, onBehalfOf, targetFormat, sigFormat, data); 799 } 800 801}