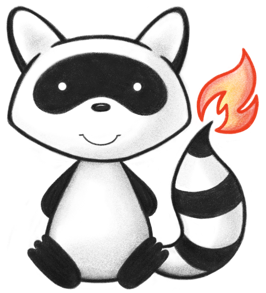
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.utilities.Utilities; 038 039import ca.uhn.fhir.model.api.annotation.Child; 040import ca.uhn.fhir.model.api.annotation.Description; 041import ca.uhn.fhir.model.api.annotation.ResourceDef; 042import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 043 044/** 045 * A container for slots of time that may be available for booking appointments. 046 */ 047@ResourceDef(name = "Schedule", profile = "http://hl7.org/fhir/StructureDefinition/Schedule") 048public class Schedule extends DomainResource { 049 050 /** 051 * External Ids for this item. 052 */ 053 @Child(name = "identifier", type = { 054 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 055 @Description(shortDefinition = "External Ids for this item", formalDefinition = "External Ids for this item.") 056 protected List<Identifier> identifier; 057 058 /** 059 * Whether this schedule record is in active use or should not be used (such as 060 * was entered in error). 061 */ 062 @Child(name = "active", type = { BooleanType.class }, order = 1, min = 0, max = 1, modifier = true, summary = true) 063 @Description(shortDefinition = "Whether this schedule is in active use", formalDefinition = "Whether this schedule record is in active use or should not be used (such as was entered in error).") 064 protected BooleanType active; 065 066 /** 067 * A broad categorization of the service that is to be performed during this 068 * appointment. 069 */ 070 @Child(name = "serviceCategory", type = { 071 CodeableConcept.class }, order = 2, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 072 @Description(shortDefinition = "High-level category", formalDefinition = "A broad categorization of the service that is to be performed during this appointment.") 073 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/service-category") 074 protected List<CodeableConcept> serviceCategory; 075 076 /** 077 * The specific service that is to be performed during this appointment. 078 */ 079 @Child(name = "serviceType", type = { 080 CodeableConcept.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 081 @Description(shortDefinition = "Specific service", formalDefinition = "The specific service that is to be performed during this appointment.") 082 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/service-type") 083 protected List<CodeableConcept> serviceType; 084 085 /** 086 * The specialty of a practitioner that would be required to perform the service 087 * requested in this appointment. 088 */ 089 @Child(name = "specialty", type = { 090 CodeableConcept.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 091 @Description(shortDefinition = "Type of specialty needed", formalDefinition = "The specialty of a practitioner that would be required to perform the service requested in this appointment.") 092 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/c80-practice-codes") 093 protected List<CodeableConcept> specialty; 094 095 /** 096 * Slots that reference this schedule resource provide the availability details 097 * to these referenced resource(s). 098 */ 099 @Child(name = "actor", type = { Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class, 100 Device.class, HealthcareService.class, 101 Location.class }, order = 5, min = 1, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 102 @Description(shortDefinition = "Resource(s) that availability information is being provided for", formalDefinition = "Slots that reference this schedule resource provide the availability details to these referenced resource(s).") 103 protected List<Reference> actor; 104 /** 105 * The actual objects that are the target of the reference (Slots that reference 106 * this schedule resource provide the availability details to these referenced 107 * resource(s).) 108 */ 109 protected List<Resource> actorTarget; 110 111 /** 112 * The period of time that the slots that reference this Schedule resource cover 113 * (even if none exist). These cover the amount of time that an organization's 114 * planning horizon; the interval for which they are currently accepting 115 * appointments. This does not define a "template" for planning outside these 116 * dates. 117 */ 118 @Child(name = "planningHorizon", type = { 119 Period.class }, order = 6, min = 0, max = 1, modifier = false, summary = true) 120 @Description(shortDefinition = "Period of time covered by schedule", formalDefinition = "The period of time that the slots that reference this Schedule resource cover (even if none exist). These cover the amount of time that an organization's planning horizon; the interval for which they are currently accepting appointments. This does not define a \"template\" for planning outside these dates.") 121 protected Period planningHorizon; 122 123 /** 124 * Comments on the availability to describe any extended information. Such as 125 * custom constraints on the slots that may be associated. 126 */ 127 @Child(name = "comment", type = { StringType.class }, order = 7, min = 0, max = 1, modifier = false, summary = false) 128 @Description(shortDefinition = "Comments on availability", formalDefinition = "Comments on the availability to describe any extended information. Such as custom constraints on the slots that may be associated.") 129 protected StringType comment; 130 131 private static final long serialVersionUID = 203182600L; 132 133 /** 134 * Constructor 135 */ 136 public Schedule() { 137 super(); 138 } 139 140 /** 141 * @return {@link #identifier} (External Ids for this item.) 142 */ 143 public List<Identifier> getIdentifier() { 144 if (this.identifier == null) 145 this.identifier = new ArrayList<Identifier>(); 146 return this.identifier; 147 } 148 149 /** 150 * @return Returns a reference to <code>this</code> for easy method chaining 151 */ 152 public Schedule setIdentifier(List<Identifier> theIdentifier) { 153 this.identifier = theIdentifier; 154 return this; 155 } 156 157 public boolean hasIdentifier() { 158 if (this.identifier == null) 159 return false; 160 for (Identifier item : this.identifier) 161 if (!item.isEmpty()) 162 return true; 163 return false; 164 } 165 166 public Identifier addIdentifier() { // 3 167 Identifier t = new Identifier(); 168 if (this.identifier == null) 169 this.identifier = new ArrayList<Identifier>(); 170 this.identifier.add(t); 171 return t; 172 } 173 174 public Schedule addIdentifier(Identifier t) { // 3 175 if (t == null) 176 return this; 177 if (this.identifier == null) 178 this.identifier = new ArrayList<Identifier>(); 179 this.identifier.add(t); 180 return this; 181 } 182 183 /** 184 * @return The first repetition of repeating field {@link #identifier}, creating 185 * it if it does not already exist 186 */ 187 public Identifier getIdentifierFirstRep() { 188 if (getIdentifier().isEmpty()) { 189 addIdentifier(); 190 } 191 return getIdentifier().get(0); 192 } 193 194 /** 195 * @return {@link #active} (Whether this schedule record is in active use or 196 * should not be used (such as was entered in error).). This is the 197 * underlying object with id, value and extensions. The accessor 198 * "getActive" gives direct access to the value 199 */ 200 public BooleanType getActiveElement() { 201 if (this.active == null) 202 if (Configuration.errorOnAutoCreate()) 203 throw new Error("Attempt to auto-create Schedule.active"); 204 else if (Configuration.doAutoCreate()) 205 this.active = new BooleanType(); // bb 206 return this.active; 207 } 208 209 public boolean hasActiveElement() { 210 return this.active != null && !this.active.isEmpty(); 211 } 212 213 public boolean hasActive() { 214 return this.active != null && !this.active.isEmpty(); 215 } 216 217 /** 218 * @param value {@link #active} (Whether this schedule record is in active use 219 * or should not be used (such as was entered in error).). This is 220 * the underlying object with id, value and extensions. The 221 * accessor "getActive" gives direct access to the value 222 */ 223 public Schedule setActiveElement(BooleanType value) { 224 this.active = value; 225 return this; 226 } 227 228 /** 229 * @return Whether this schedule record is in active use or should not be used 230 * (such as was entered in error). 231 */ 232 public boolean getActive() { 233 return this.active == null || this.active.isEmpty() ? false : this.active.getValue(); 234 } 235 236 /** 237 * @param value Whether this schedule record is in active use or should not be 238 * used (such as was entered in error). 239 */ 240 public Schedule setActive(boolean value) { 241 if (this.active == null) 242 this.active = new BooleanType(); 243 this.active.setValue(value); 244 return this; 245 } 246 247 /** 248 * @return {@link #serviceCategory} (A broad categorization of the service that 249 * is to be performed during this appointment.) 250 */ 251 public List<CodeableConcept> getServiceCategory() { 252 if (this.serviceCategory == null) 253 this.serviceCategory = new ArrayList<CodeableConcept>(); 254 return this.serviceCategory; 255 } 256 257 /** 258 * @return Returns a reference to <code>this</code> for easy method chaining 259 */ 260 public Schedule setServiceCategory(List<CodeableConcept> theServiceCategory) { 261 this.serviceCategory = theServiceCategory; 262 return this; 263 } 264 265 public boolean hasServiceCategory() { 266 if (this.serviceCategory == null) 267 return false; 268 for (CodeableConcept item : this.serviceCategory) 269 if (!item.isEmpty()) 270 return true; 271 return false; 272 } 273 274 public CodeableConcept addServiceCategory() { // 3 275 CodeableConcept t = new CodeableConcept(); 276 if (this.serviceCategory == null) 277 this.serviceCategory = new ArrayList<CodeableConcept>(); 278 this.serviceCategory.add(t); 279 return t; 280 } 281 282 public Schedule addServiceCategory(CodeableConcept t) { // 3 283 if (t == null) 284 return this; 285 if (this.serviceCategory == null) 286 this.serviceCategory = new ArrayList<CodeableConcept>(); 287 this.serviceCategory.add(t); 288 return this; 289 } 290 291 /** 292 * @return The first repetition of repeating field {@link #serviceCategory}, 293 * creating it if it does not already exist 294 */ 295 public CodeableConcept getServiceCategoryFirstRep() { 296 if (getServiceCategory().isEmpty()) { 297 addServiceCategory(); 298 } 299 return getServiceCategory().get(0); 300 } 301 302 /** 303 * @return {@link #serviceType} (The specific service that is to be performed 304 * during this appointment.) 305 */ 306 public List<CodeableConcept> getServiceType() { 307 if (this.serviceType == null) 308 this.serviceType = new ArrayList<CodeableConcept>(); 309 return this.serviceType; 310 } 311 312 /** 313 * @return Returns a reference to <code>this</code> for easy method chaining 314 */ 315 public Schedule setServiceType(List<CodeableConcept> theServiceType) { 316 this.serviceType = theServiceType; 317 return this; 318 } 319 320 public boolean hasServiceType() { 321 if (this.serviceType == null) 322 return false; 323 for (CodeableConcept item : this.serviceType) 324 if (!item.isEmpty()) 325 return true; 326 return false; 327 } 328 329 public CodeableConcept addServiceType() { // 3 330 CodeableConcept t = new CodeableConcept(); 331 if (this.serviceType == null) 332 this.serviceType = new ArrayList<CodeableConcept>(); 333 this.serviceType.add(t); 334 return t; 335 } 336 337 public Schedule addServiceType(CodeableConcept t) { // 3 338 if (t == null) 339 return this; 340 if (this.serviceType == null) 341 this.serviceType = new ArrayList<CodeableConcept>(); 342 this.serviceType.add(t); 343 return this; 344 } 345 346 /** 347 * @return The first repetition of repeating field {@link #serviceType}, 348 * creating it if it does not already exist 349 */ 350 public CodeableConcept getServiceTypeFirstRep() { 351 if (getServiceType().isEmpty()) { 352 addServiceType(); 353 } 354 return getServiceType().get(0); 355 } 356 357 /** 358 * @return {@link #specialty} (The specialty of a practitioner that would be 359 * required to perform the service requested in this appointment.) 360 */ 361 public List<CodeableConcept> getSpecialty() { 362 if (this.specialty == null) 363 this.specialty = new ArrayList<CodeableConcept>(); 364 return this.specialty; 365 } 366 367 /** 368 * @return Returns a reference to <code>this</code> for easy method chaining 369 */ 370 public Schedule setSpecialty(List<CodeableConcept> theSpecialty) { 371 this.specialty = theSpecialty; 372 return this; 373 } 374 375 public boolean hasSpecialty() { 376 if (this.specialty == null) 377 return false; 378 for (CodeableConcept item : this.specialty) 379 if (!item.isEmpty()) 380 return true; 381 return false; 382 } 383 384 public CodeableConcept addSpecialty() { // 3 385 CodeableConcept t = new CodeableConcept(); 386 if (this.specialty == null) 387 this.specialty = new ArrayList<CodeableConcept>(); 388 this.specialty.add(t); 389 return t; 390 } 391 392 public Schedule addSpecialty(CodeableConcept t) { // 3 393 if (t == null) 394 return this; 395 if (this.specialty == null) 396 this.specialty = new ArrayList<CodeableConcept>(); 397 this.specialty.add(t); 398 return this; 399 } 400 401 /** 402 * @return The first repetition of repeating field {@link #specialty}, creating 403 * it if it does not already exist 404 */ 405 public CodeableConcept getSpecialtyFirstRep() { 406 if (getSpecialty().isEmpty()) { 407 addSpecialty(); 408 } 409 return getSpecialty().get(0); 410 } 411 412 /** 413 * @return {@link #actor} (Slots that reference this schedule resource provide 414 * the availability details to these referenced resource(s).) 415 */ 416 public List<Reference> getActor() { 417 if (this.actor == null) 418 this.actor = new ArrayList<Reference>(); 419 return this.actor; 420 } 421 422 /** 423 * @return Returns a reference to <code>this</code> for easy method chaining 424 */ 425 public Schedule setActor(List<Reference> theActor) { 426 this.actor = theActor; 427 return this; 428 } 429 430 public boolean hasActor() { 431 if (this.actor == null) 432 return false; 433 for (Reference item : this.actor) 434 if (!item.isEmpty()) 435 return true; 436 return false; 437 } 438 439 public Reference addActor() { // 3 440 Reference t = new Reference(); 441 if (this.actor == null) 442 this.actor = new ArrayList<Reference>(); 443 this.actor.add(t); 444 return t; 445 } 446 447 public Schedule addActor(Reference t) { // 3 448 if (t == null) 449 return this; 450 if (this.actor == null) 451 this.actor = new ArrayList<Reference>(); 452 this.actor.add(t); 453 return this; 454 } 455 456 /** 457 * @return The first repetition of repeating field {@link #actor}, creating it 458 * if it does not already exist 459 */ 460 public Reference getActorFirstRep() { 461 if (getActor().isEmpty()) { 462 addActor(); 463 } 464 return getActor().get(0); 465 } 466 467 /** 468 * @deprecated Use Reference#setResource(IBaseResource) instead 469 */ 470 @Deprecated 471 public List<Resource> getActorTarget() { 472 if (this.actorTarget == null) 473 this.actorTarget = new ArrayList<Resource>(); 474 return this.actorTarget; 475 } 476 477 /** 478 * @return {@link #planningHorizon} (The period of time that the slots that 479 * reference this Schedule resource cover (even if none exist). These 480 * cover the amount of time that an organization's planning horizon; the 481 * interval for which they are currently accepting appointments. This 482 * does not define a "template" for planning outside these dates.) 483 */ 484 public Period getPlanningHorizon() { 485 if (this.planningHorizon == null) 486 if (Configuration.errorOnAutoCreate()) 487 throw new Error("Attempt to auto-create Schedule.planningHorizon"); 488 else if (Configuration.doAutoCreate()) 489 this.planningHorizon = new Period(); // cc 490 return this.planningHorizon; 491 } 492 493 public boolean hasPlanningHorizon() { 494 return this.planningHorizon != null && !this.planningHorizon.isEmpty(); 495 } 496 497 /** 498 * @param value {@link #planningHorizon} (The period of time that the slots that 499 * reference this Schedule resource cover (even if none exist). 500 * These cover the amount of time that an organization's planning 501 * horizon; the interval for which they are currently accepting 502 * appointments. This does not define a "template" for planning 503 * outside these dates.) 504 */ 505 public Schedule setPlanningHorizon(Period value) { 506 this.planningHorizon = value; 507 return this; 508 } 509 510 /** 511 * @return {@link #comment} (Comments on the availability to describe any 512 * extended information. Such as custom constraints on the slots that 513 * may be associated.). This is the underlying object with id, value and 514 * extensions. The accessor "getComment" gives direct access to the 515 * value 516 */ 517 public StringType getCommentElement() { 518 if (this.comment == null) 519 if (Configuration.errorOnAutoCreate()) 520 throw new Error("Attempt to auto-create Schedule.comment"); 521 else if (Configuration.doAutoCreate()) 522 this.comment = new StringType(); // bb 523 return this.comment; 524 } 525 526 public boolean hasCommentElement() { 527 return this.comment != null && !this.comment.isEmpty(); 528 } 529 530 public boolean hasComment() { 531 return this.comment != null && !this.comment.isEmpty(); 532 } 533 534 /** 535 * @param value {@link #comment} (Comments on the availability to describe any 536 * extended information. Such as custom constraints on the slots 537 * that may be associated.). This is the underlying object with id, 538 * value and extensions. The accessor "getComment" gives direct 539 * access to the value 540 */ 541 public Schedule setCommentElement(StringType value) { 542 this.comment = value; 543 return this; 544 } 545 546 /** 547 * @return Comments on the availability to describe any extended information. 548 * Such as custom constraints on the slots that may be associated. 549 */ 550 public String getComment() { 551 return this.comment == null ? null : this.comment.getValue(); 552 } 553 554 /** 555 * @param value Comments on the availability to describe any extended 556 * information. Such as custom constraints on the slots that may be 557 * associated. 558 */ 559 public Schedule setComment(String value) { 560 if (Utilities.noString(value)) 561 this.comment = null; 562 else { 563 if (this.comment == null) 564 this.comment = new StringType(); 565 this.comment.setValue(value); 566 } 567 return this; 568 } 569 570 protected void listChildren(List<Property> children) { 571 super.listChildren(children); 572 children.add(new Property("identifier", "Identifier", "External Ids for this item.", 0, java.lang.Integer.MAX_VALUE, 573 identifier)); 574 children.add(new Property("active", "boolean", 575 "Whether this schedule record is in active use or should not be used (such as was entered in error).", 0, 1, 576 active)); 577 children.add(new Property("serviceCategory", "CodeableConcept", 578 "A broad categorization of the service that is to be performed during this appointment.", 0, 579 java.lang.Integer.MAX_VALUE, serviceCategory)); 580 children.add(new Property("serviceType", "CodeableConcept", 581 "The specific service that is to be performed during this appointment.", 0, java.lang.Integer.MAX_VALUE, 582 serviceType)); 583 children.add(new Property("specialty", "CodeableConcept", 584 "The specialty of a practitioner that would be required to perform the service requested in this appointment.", 585 0, java.lang.Integer.MAX_VALUE, specialty)); 586 children.add(new Property("actor", 587 "Reference(Patient|Practitioner|PractitionerRole|RelatedPerson|Device|HealthcareService|Location)", 588 "Slots that reference this schedule resource provide the availability details to these referenced resource(s).", 589 0, java.lang.Integer.MAX_VALUE, actor)); 590 children.add(new Property("planningHorizon", "Period", 591 "The period of time that the slots that reference this Schedule resource cover (even if none exist). These cover the amount of time that an organization's planning horizon; the interval for which they are currently accepting appointments. This does not define a \"template\" for planning outside these dates.", 592 0, 1, planningHorizon)); 593 children.add(new Property("comment", "string", 594 "Comments on the availability to describe any extended information. Such as custom constraints on the slots that may be associated.", 595 0, 1, comment)); 596 } 597 598 @Override 599 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 600 switch (_hash) { 601 case -1618432855: 602 /* identifier */ return new Property("identifier", "Identifier", "External Ids for this item.", 0, 603 java.lang.Integer.MAX_VALUE, identifier); 604 case -1422950650: 605 /* active */ return new Property("active", "boolean", 606 "Whether this schedule record is in active use or should not be used (such as was entered in error).", 0, 1, 607 active); 608 case 1281188563: 609 /* serviceCategory */ return new Property("serviceCategory", "CodeableConcept", 610 "A broad categorization of the service that is to be performed during this appointment.", 0, 611 java.lang.Integer.MAX_VALUE, serviceCategory); 612 case -1928370289: 613 /* serviceType */ return new Property("serviceType", "CodeableConcept", 614 "The specific service that is to be performed during this appointment.", 0, java.lang.Integer.MAX_VALUE, 615 serviceType); 616 case -1694759682: 617 /* specialty */ return new Property("specialty", "CodeableConcept", 618 "The specialty of a practitioner that would be required to perform the service requested in this appointment.", 619 0, java.lang.Integer.MAX_VALUE, specialty); 620 case 92645877: 621 /* actor */ return new Property("actor", 622 "Reference(Patient|Practitioner|PractitionerRole|RelatedPerson|Device|HealthcareService|Location)", 623 "Slots that reference this schedule resource provide the availability details to these referenced resource(s).", 624 0, java.lang.Integer.MAX_VALUE, actor); 625 case -1718507650: 626 /* planningHorizon */ return new Property("planningHorizon", "Period", 627 "The period of time that the slots that reference this Schedule resource cover (even if none exist). These cover the amount of time that an organization's planning horizon; the interval for which they are currently accepting appointments. This does not define a \"template\" for planning outside these dates.", 628 0, 1, planningHorizon); 629 case 950398559: 630 /* comment */ return new Property("comment", "string", 631 "Comments on the availability to describe any extended information. Such as custom constraints on the slots that may be associated.", 632 0, 1, comment); 633 default: 634 return super.getNamedProperty(_hash, _name, _checkValid); 635 } 636 637 } 638 639 @Override 640 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 641 switch (hash) { 642 case -1618432855: 643 /* identifier */ return this.identifier == null ? new Base[0] 644 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 645 case -1422950650: 646 /* active */ return this.active == null ? new Base[0] : new Base[] { this.active }; // BooleanType 647 case 1281188563: 648 /* serviceCategory */ return this.serviceCategory == null ? new Base[0] 649 : this.serviceCategory.toArray(new Base[this.serviceCategory.size()]); // CodeableConcept 650 case -1928370289: 651 /* serviceType */ return this.serviceType == null ? new Base[0] 652 : this.serviceType.toArray(new Base[this.serviceType.size()]); // CodeableConcept 653 case -1694759682: 654 /* specialty */ return this.specialty == null ? new Base[0] 655 : this.specialty.toArray(new Base[this.specialty.size()]); // CodeableConcept 656 case 92645877: 657 /* actor */ return this.actor == null ? new Base[0] : this.actor.toArray(new Base[this.actor.size()]); // Reference 658 case -1718507650: 659 /* planningHorizon */ return this.planningHorizon == null ? new Base[0] : new Base[] { this.planningHorizon }; // Period 660 case 950398559: 661 /* comment */ return this.comment == null ? new Base[0] : new Base[] { this.comment }; // StringType 662 default: 663 return super.getProperty(hash, name, checkValid); 664 } 665 666 } 667 668 @Override 669 public Base setProperty(int hash, String name, Base value) throws FHIRException { 670 switch (hash) { 671 case -1618432855: // identifier 672 this.getIdentifier().add(castToIdentifier(value)); // Identifier 673 return value; 674 case -1422950650: // active 675 this.active = castToBoolean(value); // BooleanType 676 return value; 677 case 1281188563: // serviceCategory 678 this.getServiceCategory().add(castToCodeableConcept(value)); // CodeableConcept 679 return value; 680 case -1928370289: // serviceType 681 this.getServiceType().add(castToCodeableConcept(value)); // CodeableConcept 682 return value; 683 case -1694759682: // specialty 684 this.getSpecialty().add(castToCodeableConcept(value)); // CodeableConcept 685 return value; 686 case 92645877: // actor 687 this.getActor().add(castToReference(value)); // Reference 688 return value; 689 case -1718507650: // planningHorizon 690 this.planningHorizon = castToPeriod(value); // Period 691 return value; 692 case 950398559: // comment 693 this.comment = castToString(value); // StringType 694 return value; 695 default: 696 return super.setProperty(hash, name, value); 697 } 698 699 } 700 701 @Override 702 public Base setProperty(String name, Base value) throws FHIRException { 703 if (name.equals("identifier")) { 704 this.getIdentifier().add(castToIdentifier(value)); 705 } else if (name.equals("active")) { 706 this.active = castToBoolean(value); // BooleanType 707 } else if (name.equals("serviceCategory")) { 708 this.getServiceCategory().add(castToCodeableConcept(value)); 709 } else if (name.equals("serviceType")) { 710 this.getServiceType().add(castToCodeableConcept(value)); 711 } else if (name.equals("specialty")) { 712 this.getSpecialty().add(castToCodeableConcept(value)); 713 } else if (name.equals("actor")) { 714 this.getActor().add(castToReference(value)); 715 } else if (name.equals("planningHorizon")) { 716 this.planningHorizon = castToPeriod(value); // Period 717 } else if (name.equals("comment")) { 718 this.comment = castToString(value); // StringType 719 } else 720 return super.setProperty(name, value); 721 return value; 722 } 723 724 @Override 725 public Base makeProperty(int hash, String name) throws FHIRException { 726 switch (hash) { 727 case -1618432855: 728 return addIdentifier(); 729 case -1422950650: 730 return getActiveElement(); 731 case 1281188563: 732 return addServiceCategory(); 733 case -1928370289: 734 return addServiceType(); 735 case -1694759682: 736 return addSpecialty(); 737 case 92645877: 738 return addActor(); 739 case -1718507650: 740 return getPlanningHorizon(); 741 case 950398559: 742 return getCommentElement(); 743 default: 744 return super.makeProperty(hash, name); 745 } 746 747 } 748 749 @Override 750 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 751 switch (hash) { 752 case -1618432855: 753 /* identifier */ return new String[] { "Identifier" }; 754 case -1422950650: 755 /* active */ return new String[] { "boolean" }; 756 case 1281188563: 757 /* serviceCategory */ return new String[] { "CodeableConcept" }; 758 case -1928370289: 759 /* serviceType */ return new String[] { "CodeableConcept" }; 760 case -1694759682: 761 /* specialty */ return new String[] { "CodeableConcept" }; 762 case 92645877: 763 /* actor */ return new String[] { "Reference" }; 764 case -1718507650: 765 /* planningHorizon */ return new String[] { "Period" }; 766 case 950398559: 767 /* comment */ return new String[] { "string" }; 768 default: 769 return super.getTypesForProperty(hash, name); 770 } 771 772 } 773 774 @Override 775 public Base addChild(String name) throws FHIRException { 776 if (name.equals("identifier")) { 777 return addIdentifier(); 778 } else if (name.equals("active")) { 779 throw new FHIRException("Cannot call addChild on a singleton property Schedule.active"); 780 } else if (name.equals("serviceCategory")) { 781 return addServiceCategory(); 782 } else if (name.equals("serviceType")) { 783 return addServiceType(); 784 } else if (name.equals("specialty")) { 785 return addSpecialty(); 786 } else if (name.equals("actor")) { 787 return addActor(); 788 } else if (name.equals("planningHorizon")) { 789 this.planningHorizon = new Period(); 790 return this.planningHorizon; 791 } else if (name.equals("comment")) { 792 throw new FHIRException("Cannot call addChild on a singleton property Schedule.comment"); 793 } else 794 return super.addChild(name); 795 } 796 797 public String fhirType() { 798 return "Schedule"; 799 800 } 801 802 public Schedule copy() { 803 Schedule dst = new Schedule(); 804 copyValues(dst); 805 return dst; 806 } 807 808 public void copyValues(Schedule dst) { 809 super.copyValues(dst); 810 if (identifier != null) { 811 dst.identifier = new ArrayList<Identifier>(); 812 for (Identifier i : identifier) 813 dst.identifier.add(i.copy()); 814 } 815 ; 816 dst.active = active == null ? null : active.copy(); 817 if (serviceCategory != null) { 818 dst.serviceCategory = new ArrayList<CodeableConcept>(); 819 for (CodeableConcept i : serviceCategory) 820 dst.serviceCategory.add(i.copy()); 821 } 822 ; 823 if (serviceType != null) { 824 dst.serviceType = new ArrayList<CodeableConcept>(); 825 for (CodeableConcept i : serviceType) 826 dst.serviceType.add(i.copy()); 827 } 828 ; 829 if (specialty != null) { 830 dst.specialty = new ArrayList<CodeableConcept>(); 831 for (CodeableConcept i : specialty) 832 dst.specialty.add(i.copy()); 833 } 834 ; 835 if (actor != null) { 836 dst.actor = new ArrayList<Reference>(); 837 for (Reference i : actor) 838 dst.actor.add(i.copy()); 839 } 840 ; 841 dst.planningHorizon = planningHorizon == null ? null : planningHorizon.copy(); 842 dst.comment = comment == null ? null : comment.copy(); 843 } 844 845 protected Schedule typedCopy() { 846 return copy(); 847 } 848 849 @Override 850 public boolean equalsDeep(Base other_) { 851 if (!super.equalsDeep(other_)) 852 return false; 853 if (!(other_ instanceof Schedule)) 854 return false; 855 Schedule o = (Schedule) other_; 856 return compareDeep(identifier, o.identifier, true) && compareDeep(active, o.active, true) 857 && compareDeep(serviceCategory, o.serviceCategory, true) && compareDeep(serviceType, o.serviceType, true) 858 && compareDeep(specialty, o.specialty, true) && compareDeep(actor, o.actor, true) 859 && compareDeep(planningHorizon, o.planningHorizon, true) && compareDeep(comment, o.comment, true); 860 } 861 862 @Override 863 public boolean equalsShallow(Base other_) { 864 if (!super.equalsShallow(other_)) 865 return false; 866 if (!(other_ instanceof Schedule)) 867 return false; 868 Schedule o = (Schedule) other_; 869 return compareValues(active, o.active, true) && compareValues(comment, o.comment, true); 870 } 871 872 public boolean isEmpty() { 873 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, active, serviceCategory, serviceType, 874 specialty, actor, planningHorizon, comment); 875 } 876 877 @Override 878 public ResourceType getResourceType() { 879 return ResourceType.Schedule; 880 } 881 882 /** 883 * Search parameter: <b>actor</b> 884 * <p> 885 * Description: <b>The individual(HealthcareService, Practitioner, Location, 886 * ...) to find a Schedule for</b><br> 887 * Type: <b>reference</b><br> 888 * Path: <b>Schedule.actor</b><br> 889 * </p> 890 */ 891 @SearchParamDefinition(name = "actor", path = "Schedule.actor", description = "The individual(HealthcareService, Practitioner, Location, ...) to find a Schedule for", type = "reference", providesMembershipIn = { 892 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Device"), 893 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 894 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner"), 895 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { Device.class, 896 HealthcareService.class, Location.class, Patient.class, Practitioner.class, PractitionerRole.class, 897 RelatedPerson.class }) 898 public static final String SP_ACTOR = "actor"; 899 /** 900 * <b>Fluent Client</b> search parameter constant for <b>actor</b> 901 * <p> 902 * Description: <b>The individual(HealthcareService, Practitioner, Location, 903 * ...) to find a Schedule for</b><br> 904 * Type: <b>reference</b><br> 905 * Path: <b>Schedule.actor</b><br> 906 * </p> 907 */ 908 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ACTOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 909 SP_ACTOR); 910 911 /** 912 * Constant for fluent queries to be used to add include statements. Specifies 913 * the path value of "<b>Schedule:actor</b>". 914 */ 915 public static final ca.uhn.fhir.model.api.Include INCLUDE_ACTOR = new ca.uhn.fhir.model.api.Include("Schedule:actor") 916 .toLocked(); 917 918 /** 919 * Search parameter: <b>date</b> 920 * <p> 921 * Description: <b>Search for Schedule resources that have a period that 922 * contains this date specified</b><br> 923 * Type: <b>date</b><br> 924 * Path: <b>Schedule.planningHorizon</b><br> 925 * </p> 926 */ 927 @SearchParamDefinition(name = "date", path = "Schedule.planningHorizon", description = "Search for Schedule resources that have a period that contains this date specified", type = "date") 928 public static final String SP_DATE = "date"; 929 /** 930 * <b>Fluent Client</b> search parameter constant for <b>date</b> 931 * <p> 932 * Description: <b>Search for Schedule resources that have a period that 933 * contains this date specified</b><br> 934 * Type: <b>date</b><br> 935 * Path: <b>Schedule.planningHorizon</b><br> 936 * </p> 937 */ 938 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 939 SP_DATE); 940 941 /** 942 * Search parameter: <b>identifier</b> 943 * <p> 944 * Description: <b>A Schedule Identifier</b><br> 945 * Type: <b>token</b><br> 946 * Path: <b>Schedule.identifier</b><br> 947 * </p> 948 */ 949 @SearchParamDefinition(name = "identifier", path = "Schedule.identifier", description = "A Schedule Identifier", type = "token") 950 public static final String SP_IDENTIFIER = "identifier"; 951 /** 952 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 953 * <p> 954 * Description: <b>A Schedule Identifier</b><br> 955 * Type: <b>token</b><br> 956 * Path: <b>Schedule.identifier</b><br> 957 * </p> 958 */ 959 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 960 SP_IDENTIFIER); 961 962 /** 963 * Search parameter: <b>specialty</b> 964 * <p> 965 * Description: <b>Type of specialty needed</b><br> 966 * Type: <b>token</b><br> 967 * Path: <b>Schedule.specialty</b><br> 968 * </p> 969 */ 970 @SearchParamDefinition(name = "specialty", path = "Schedule.specialty", description = "Type of specialty needed", type = "token") 971 public static final String SP_SPECIALTY = "specialty"; 972 /** 973 * <b>Fluent Client</b> search parameter constant for <b>specialty</b> 974 * <p> 975 * Description: <b>Type of specialty needed</b><br> 976 * Type: <b>token</b><br> 977 * Path: <b>Schedule.specialty</b><br> 978 * </p> 979 */ 980 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SPECIALTY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 981 SP_SPECIALTY); 982 983 /** 984 * Search parameter: <b>service-category</b> 985 * <p> 986 * Description: <b>High-level category</b><br> 987 * Type: <b>token</b><br> 988 * Path: <b>Schedule.serviceCategory</b><br> 989 * </p> 990 */ 991 @SearchParamDefinition(name = "service-category", path = "Schedule.serviceCategory", description = "High-level category", type = "token") 992 public static final String SP_SERVICE_CATEGORY = "service-category"; 993 /** 994 * <b>Fluent Client</b> search parameter constant for <b>service-category</b> 995 * <p> 996 * Description: <b>High-level category</b><br> 997 * Type: <b>token</b><br> 998 * Path: <b>Schedule.serviceCategory</b><br> 999 * </p> 1000 */ 1001 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SERVICE_CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1002 SP_SERVICE_CATEGORY); 1003 1004 /** 1005 * Search parameter: <b>service-type</b> 1006 * <p> 1007 * Description: <b>The type of appointments that can be booked into associated 1008 * slot(s)</b><br> 1009 * Type: <b>token</b><br> 1010 * Path: <b>Schedule.serviceType</b><br> 1011 * </p> 1012 */ 1013 @SearchParamDefinition(name = "service-type", path = "Schedule.serviceType", description = "The type of appointments that can be booked into associated slot(s)", type = "token") 1014 public static final String SP_SERVICE_TYPE = "service-type"; 1015 /** 1016 * <b>Fluent Client</b> search parameter constant for <b>service-type</b> 1017 * <p> 1018 * Description: <b>The type of appointments that can be booked into associated 1019 * slot(s)</b><br> 1020 * Type: <b>token</b><br> 1021 * Path: <b>Schedule.serviceType</b><br> 1022 * </p> 1023 */ 1024 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SERVICE_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1025 SP_SERVICE_TYPE); 1026 1027 /** 1028 * Search parameter: <b>active</b> 1029 * <p> 1030 * Description: <b>Is the schedule in active use</b><br> 1031 * Type: <b>token</b><br> 1032 * Path: <b>Schedule.active</b><br> 1033 * </p> 1034 */ 1035 @SearchParamDefinition(name = "active", path = "Schedule.active", description = "Is the schedule in active use", type = "token") 1036 public static final String SP_ACTIVE = "active"; 1037 /** 1038 * <b>Fluent Client</b> search parameter constant for <b>active</b> 1039 * <p> 1040 * Description: <b>Is the schedule in active use</b><br> 1041 * Type: <b>token</b><br> 1042 * Path: <b>Schedule.active</b><br> 1043 * </p> 1044 */ 1045 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ACTIVE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 1046 SP_ACTIVE); 1047 1048}