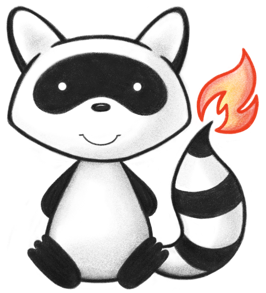
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037 038import ca.uhn.fhir.model.api.annotation.Child; 039import ca.uhn.fhir.model.api.annotation.Description; 040import ca.uhn.fhir.model.api.annotation.ResourceDef; 041import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 042 043/** 044 * Describe the undesirable effects of the medicinal product. 045 */ 046@ResourceDef(name = "MedicinalProductUndesirableEffect", profile = "http://hl7.org/fhir/StructureDefinition/MedicinalProductUndesirableEffect") 047public class MedicinalProductUndesirableEffect extends DomainResource { 048 049 /** 050 * The medication for which this is an indication. 051 */ 052 @Child(name = "subject", type = { MedicinalProduct.class, 053 Medication.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 054 @Description(shortDefinition = "The medication for which this is an indication", formalDefinition = "The medication for which this is an indication.") 055 protected List<Reference> subject; 056 /** 057 * The actual objects that are the target of the reference (The medication for 058 * which this is an indication.) 059 */ 060 protected List<Resource> subjectTarget; 061 062 /** 063 * The symptom, condition or undesirable effect. 064 */ 065 @Child(name = "symptomConditionEffect", type = { 066 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 067 @Description(shortDefinition = "The symptom, condition or undesirable effect", formalDefinition = "The symptom, condition or undesirable effect.") 068 protected CodeableConcept symptomConditionEffect; 069 070 /** 071 * Classification of the effect. 072 */ 073 @Child(name = "classification", type = { 074 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 075 @Description(shortDefinition = "Classification of the effect", formalDefinition = "Classification of the effect.") 076 protected CodeableConcept classification; 077 078 /** 079 * The frequency of occurrence of the effect. 080 */ 081 @Child(name = "frequencyOfOccurrence", type = { 082 CodeableConcept.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 083 @Description(shortDefinition = "The frequency of occurrence of the effect", formalDefinition = "The frequency of occurrence of the effect.") 084 protected CodeableConcept frequencyOfOccurrence; 085 086 /** 087 * The population group to which this applies. 088 */ 089 @Child(name = "population", type = { 090 Population.class }, order = 4, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 091 @Description(shortDefinition = "The population group to which this applies", formalDefinition = "The population group to which this applies.") 092 protected List<Population> population; 093 094 private static final long serialVersionUID = -1960253956L; 095 096 /** 097 * Constructor 098 */ 099 public MedicinalProductUndesirableEffect() { 100 super(); 101 } 102 103 /** 104 * @return {@link #subject} (The medication for which this is an indication.) 105 */ 106 public List<Reference> getSubject() { 107 if (this.subject == null) 108 this.subject = new ArrayList<Reference>(); 109 return this.subject; 110 } 111 112 /** 113 * @return Returns a reference to <code>this</code> for easy method chaining 114 */ 115 public MedicinalProductUndesirableEffect setSubject(List<Reference> theSubject) { 116 this.subject = theSubject; 117 return this; 118 } 119 120 public boolean hasSubject() { 121 if (this.subject == null) 122 return false; 123 for (Reference item : this.subject) 124 if (!item.isEmpty()) 125 return true; 126 return false; 127 } 128 129 public Reference addSubject() { // 3 130 Reference t = new Reference(); 131 if (this.subject == null) 132 this.subject = new ArrayList<Reference>(); 133 this.subject.add(t); 134 return t; 135 } 136 137 public MedicinalProductUndesirableEffect addSubject(Reference t) { // 3 138 if (t == null) 139 return this; 140 if (this.subject == null) 141 this.subject = new ArrayList<Reference>(); 142 this.subject.add(t); 143 return this; 144 } 145 146 /** 147 * @return The first repetition of repeating field {@link #subject}, creating it 148 * if it does not already exist 149 */ 150 public Reference getSubjectFirstRep() { 151 if (getSubject().isEmpty()) { 152 addSubject(); 153 } 154 return getSubject().get(0); 155 } 156 157 /** 158 * @deprecated Use Reference#setResource(IBaseResource) instead 159 */ 160 @Deprecated 161 public List<Resource> getSubjectTarget() { 162 if (this.subjectTarget == null) 163 this.subjectTarget = new ArrayList<Resource>(); 164 return this.subjectTarget; 165 } 166 167 /** 168 * @return {@link #symptomConditionEffect} (The symptom, condition or 169 * undesirable effect.) 170 */ 171 public CodeableConcept getSymptomConditionEffect() { 172 if (this.symptomConditionEffect == null) 173 if (Configuration.errorOnAutoCreate()) 174 throw new Error("Attempt to auto-create MedicinalProductUndesirableEffect.symptomConditionEffect"); 175 else if (Configuration.doAutoCreate()) 176 this.symptomConditionEffect = new CodeableConcept(); // cc 177 return this.symptomConditionEffect; 178 } 179 180 public boolean hasSymptomConditionEffect() { 181 return this.symptomConditionEffect != null && !this.symptomConditionEffect.isEmpty(); 182 } 183 184 /** 185 * @param value {@link #symptomConditionEffect} (The symptom, condition or 186 * undesirable effect.) 187 */ 188 public MedicinalProductUndesirableEffect setSymptomConditionEffect(CodeableConcept value) { 189 this.symptomConditionEffect = value; 190 return this; 191 } 192 193 /** 194 * @return {@link #classification} (Classification of the effect.) 195 */ 196 public CodeableConcept getClassification() { 197 if (this.classification == null) 198 if (Configuration.errorOnAutoCreate()) 199 throw new Error("Attempt to auto-create MedicinalProductUndesirableEffect.classification"); 200 else if (Configuration.doAutoCreate()) 201 this.classification = new CodeableConcept(); // cc 202 return this.classification; 203 } 204 205 public boolean hasClassification() { 206 return this.classification != null && !this.classification.isEmpty(); 207 } 208 209 /** 210 * @param value {@link #classification} (Classification of the effect.) 211 */ 212 public MedicinalProductUndesirableEffect setClassification(CodeableConcept value) { 213 this.classification = value; 214 return this; 215 } 216 217 /** 218 * @return {@link #frequencyOfOccurrence} (The frequency of occurrence of the 219 * effect.) 220 */ 221 public CodeableConcept getFrequencyOfOccurrence() { 222 if (this.frequencyOfOccurrence == null) 223 if (Configuration.errorOnAutoCreate()) 224 throw new Error("Attempt to auto-create MedicinalProductUndesirableEffect.frequencyOfOccurrence"); 225 else if (Configuration.doAutoCreate()) 226 this.frequencyOfOccurrence = new CodeableConcept(); // cc 227 return this.frequencyOfOccurrence; 228 } 229 230 public boolean hasFrequencyOfOccurrence() { 231 return this.frequencyOfOccurrence != null && !this.frequencyOfOccurrence.isEmpty(); 232 } 233 234 /** 235 * @param value {@link #frequencyOfOccurrence} (The frequency of occurrence of 236 * the effect.) 237 */ 238 public MedicinalProductUndesirableEffect setFrequencyOfOccurrence(CodeableConcept value) { 239 this.frequencyOfOccurrence = value; 240 return this; 241 } 242 243 /** 244 * @return {@link #population} (The population group to which this applies.) 245 */ 246 public List<Population> getPopulation() { 247 if (this.population == null) 248 this.population = new ArrayList<Population>(); 249 return this.population; 250 } 251 252 /** 253 * @return Returns a reference to <code>this</code> for easy method chaining 254 */ 255 public MedicinalProductUndesirableEffect setPopulation(List<Population> thePopulation) { 256 this.population = thePopulation; 257 return this; 258 } 259 260 public boolean hasPopulation() { 261 if (this.population == null) 262 return false; 263 for (Population item : this.population) 264 if (!item.isEmpty()) 265 return true; 266 return false; 267 } 268 269 public Population addPopulation() { // 3 270 Population t = new Population(); 271 if (this.population == null) 272 this.population = new ArrayList<Population>(); 273 this.population.add(t); 274 return t; 275 } 276 277 public MedicinalProductUndesirableEffect addPopulation(Population t) { // 3 278 if (t == null) 279 return this; 280 if (this.population == null) 281 this.population = new ArrayList<Population>(); 282 this.population.add(t); 283 return this; 284 } 285 286 /** 287 * @return The first repetition of repeating field {@link #population}, creating 288 * it if it does not already exist 289 */ 290 public Population getPopulationFirstRep() { 291 if (getPopulation().isEmpty()) { 292 addPopulation(); 293 } 294 return getPopulation().get(0); 295 } 296 297 protected void listChildren(List<Property> children) { 298 super.listChildren(children); 299 children.add(new Property("subject", "Reference(MedicinalProduct|Medication)", 300 "The medication for which this is an indication.", 0, java.lang.Integer.MAX_VALUE, subject)); 301 children.add(new Property("symptomConditionEffect", "CodeableConcept", 302 "The symptom, condition or undesirable effect.", 0, 1, symptomConditionEffect)); 303 children 304 .add(new Property("classification", "CodeableConcept", "Classification of the effect.", 0, 1, classification)); 305 children.add(new Property("frequencyOfOccurrence", "CodeableConcept", "The frequency of occurrence of the effect.", 306 0, 1, frequencyOfOccurrence)); 307 children.add(new Property("population", "Population", "The population group to which this applies.", 0, 308 java.lang.Integer.MAX_VALUE, population)); 309 } 310 311 @Override 312 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 313 switch (_hash) { 314 case -1867885268: 315 /* subject */ return new Property("subject", "Reference(MedicinalProduct|Medication)", 316 "The medication for which this is an indication.", 0, java.lang.Integer.MAX_VALUE, subject); 317 case -650549981: 318 /* symptomConditionEffect */ return new Property("symptomConditionEffect", "CodeableConcept", 319 "The symptom, condition or undesirable effect.", 0, 1, symptomConditionEffect); 320 case 382350310: 321 /* classification */ return new Property("classification", "CodeableConcept", "Classification of the effect.", 0, 322 1, classification); 323 case 791175812: 324 /* frequencyOfOccurrence */ return new Property("frequencyOfOccurrence", "CodeableConcept", 325 "The frequency of occurrence of the effect.", 0, 1, frequencyOfOccurrence); 326 case -2023558323: 327 /* population */ return new Property("population", "Population", "The population group to which this applies.", 0, 328 java.lang.Integer.MAX_VALUE, population); 329 default: 330 return super.getNamedProperty(_hash, _name, _checkValid); 331 } 332 333 } 334 335 @Override 336 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 337 switch (hash) { 338 case -1867885268: 339 /* subject */ return this.subject == null ? new Base[0] : this.subject.toArray(new Base[this.subject.size()]); // Reference 340 case -650549981: 341 /* symptomConditionEffect */ return this.symptomConditionEffect == null ? new Base[0] 342 : new Base[] { this.symptomConditionEffect }; // CodeableConcept 343 case 382350310: 344 /* classification */ return this.classification == null ? new Base[0] : new Base[] { this.classification }; // CodeableConcept 345 case 791175812: 346 /* frequencyOfOccurrence */ return this.frequencyOfOccurrence == null ? new Base[0] 347 : new Base[] { this.frequencyOfOccurrence }; // CodeableConcept 348 case -2023558323: 349 /* population */ return this.population == null ? new Base[0] 350 : this.population.toArray(new Base[this.population.size()]); // Population 351 default: 352 return super.getProperty(hash, name, checkValid); 353 } 354 355 } 356 357 @Override 358 public Base setProperty(int hash, String name, Base value) throws FHIRException { 359 switch (hash) { 360 case -1867885268: // subject 361 this.getSubject().add(castToReference(value)); // Reference 362 return value; 363 case -650549981: // symptomConditionEffect 364 this.symptomConditionEffect = castToCodeableConcept(value); // CodeableConcept 365 return value; 366 case 382350310: // classification 367 this.classification = castToCodeableConcept(value); // CodeableConcept 368 return value; 369 case 791175812: // frequencyOfOccurrence 370 this.frequencyOfOccurrence = castToCodeableConcept(value); // CodeableConcept 371 return value; 372 case -2023558323: // population 373 this.getPopulation().add(castToPopulation(value)); // Population 374 return value; 375 default: 376 return super.setProperty(hash, name, value); 377 } 378 379 } 380 381 @Override 382 public Base setProperty(String name, Base value) throws FHIRException { 383 if (name.equals("subject")) { 384 this.getSubject().add(castToReference(value)); 385 } else if (name.equals("symptomConditionEffect")) { 386 this.symptomConditionEffect = castToCodeableConcept(value); // CodeableConcept 387 } else if (name.equals("classification")) { 388 this.classification = castToCodeableConcept(value); // CodeableConcept 389 } else if (name.equals("frequencyOfOccurrence")) { 390 this.frequencyOfOccurrence = castToCodeableConcept(value); // CodeableConcept 391 } else if (name.equals("population")) { 392 this.getPopulation().add(castToPopulation(value)); 393 } else 394 return super.setProperty(name, value); 395 return value; 396 } 397 398 @Override 399 public Base makeProperty(int hash, String name) throws FHIRException { 400 switch (hash) { 401 case -1867885268: 402 return addSubject(); 403 case -650549981: 404 return getSymptomConditionEffect(); 405 case 382350310: 406 return getClassification(); 407 case 791175812: 408 return getFrequencyOfOccurrence(); 409 case -2023558323: 410 return addPopulation(); 411 default: 412 return super.makeProperty(hash, name); 413 } 414 415 } 416 417 @Override 418 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 419 switch (hash) { 420 case -1867885268: 421 /* subject */ return new String[] { "Reference" }; 422 case -650549981: 423 /* symptomConditionEffect */ return new String[] { "CodeableConcept" }; 424 case 382350310: 425 /* classification */ return new String[] { "CodeableConcept" }; 426 case 791175812: 427 /* frequencyOfOccurrence */ return new String[] { "CodeableConcept" }; 428 case -2023558323: 429 /* population */ return new String[] { "Population" }; 430 default: 431 return super.getTypesForProperty(hash, name); 432 } 433 434 } 435 436 @Override 437 public Base addChild(String name) throws FHIRException { 438 if (name.equals("subject")) { 439 return addSubject(); 440 } else if (name.equals("symptomConditionEffect")) { 441 this.symptomConditionEffect = new CodeableConcept(); 442 return this.symptomConditionEffect; 443 } else if (name.equals("classification")) { 444 this.classification = new CodeableConcept(); 445 return this.classification; 446 } else if (name.equals("frequencyOfOccurrence")) { 447 this.frequencyOfOccurrence = new CodeableConcept(); 448 return this.frequencyOfOccurrence; 449 } else if (name.equals("population")) { 450 return addPopulation(); 451 } else 452 return super.addChild(name); 453 } 454 455 public String fhirType() { 456 return "MedicinalProductUndesirableEffect"; 457 458 } 459 460 public MedicinalProductUndesirableEffect copy() { 461 MedicinalProductUndesirableEffect dst = new MedicinalProductUndesirableEffect(); 462 copyValues(dst); 463 return dst; 464 } 465 466 public void copyValues(MedicinalProductUndesirableEffect dst) { 467 super.copyValues(dst); 468 if (subject != null) { 469 dst.subject = new ArrayList<Reference>(); 470 for (Reference i : subject) 471 dst.subject.add(i.copy()); 472 } 473 ; 474 dst.symptomConditionEffect = symptomConditionEffect == null ? null : symptomConditionEffect.copy(); 475 dst.classification = classification == null ? null : classification.copy(); 476 dst.frequencyOfOccurrence = frequencyOfOccurrence == null ? null : frequencyOfOccurrence.copy(); 477 if (population != null) { 478 dst.population = new ArrayList<Population>(); 479 for (Population i : population) 480 dst.population.add(i.copy()); 481 } 482 ; 483 } 484 485 protected MedicinalProductUndesirableEffect typedCopy() { 486 return copy(); 487 } 488 489 @Override 490 public boolean equalsDeep(Base other_) { 491 if (!super.equalsDeep(other_)) 492 return false; 493 if (!(other_ instanceof MedicinalProductUndesirableEffect)) 494 return false; 495 MedicinalProductUndesirableEffect o = (MedicinalProductUndesirableEffect) other_; 496 return compareDeep(subject, o.subject, true) && compareDeep(symptomConditionEffect, o.symptomConditionEffect, true) 497 && compareDeep(classification, o.classification, true) 498 && compareDeep(frequencyOfOccurrence, o.frequencyOfOccurrence, true) 499 && compareDeep(population, o.population, true); 500 } 501 502 @Override 503 public boolean equalsShallow(Base other_) { 504 if (!super.equalsShallow(other_)) 505 return false; 506 if (!(other_ instanceof MedicinalProductUndesirableEffect)) 507 return false; 508 MedicinalProductUndesirableEffect o = (MedicinalProductUndesirableEffect) other_; 509 return true; 510 } 511 512 public boolean isEmpty() { 513 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(subject, symptomConditionEffect, classification, 514 frequencyOfOccurrence, population); 515 } 516 517 @Override 518 public ResourceType getResourceType() { 519 return ResourceType.MedicinalProductUndesirableEffect; 520 } 521 522 /** 523 * Search parameter: <b>subject</b> 524 * <p> 525 * Description: <b>The medication for which this is an undesirable 526 * effect</b><br> 527 * Type: <b>reference</b><br> 528 * Path: <b>MedicinalProductUndesirableEffect.subject</b><br> 529 * </p> 530 */ 531 @SearchParamDefinition(name = "subject", path = "MedicinalProductUndesirableEffect.subject", description = "The medication for which this is an undesirable effect", type = "reference", target = { 532 Medication.class, MedicinalProduct.class }) 533 public static final String SP_SUBJECT = "subject"; 534 /** 535 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 536 * <p> 537 * Description: <b>The medication for which this is an undesirable 538 * effect</b><br> 539 * Type: <b>reference</b><br> 540 * Path: <b>MedicinalProductUndesirableEffect.subject</b><br> 541 * </p> 542 */ 543 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 544 SP_SUBJECT); 545 546 /** 547 * Constant for fluent queries to be used to add include statements. Specifies 548 * the path value of "<b>MedicinalProductUndesirableEffect:subject</b>". 549 */ 550 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include( 551 "MedicinalProductUndesirableEffect:subject").toLocked(); 552 553}