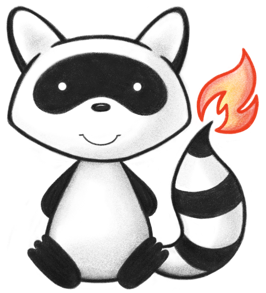
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 038 039import ca.uhn.fhir.model.api.annotation.Block; 040import ca.uhn.fhir.model.api.annotation.Child; 041import ca.uhn.fhir.model.api.annotation.Description; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044 045/** 046 * Indication for the Medicinal Product. 047 */ 048@ResourceDef(name = "MedicinalProductIndication", profile = "http://hl7.org/fhir/StructureDefinition/MedicinalProductIndication") 049public class MedicinalProductIndication extends DomainResource { 050 051 @Block() 052 public static class MedicinalProductIndicationOtherTherapyComponent extends BackboneElement 053 implements IBaseBackboneElement { 054 /** 055 * The type of relationship between the medicinal product indication or 056 * contraindication and another therapy. 057 */ 058 @Child(name = "therapyRelationshipType", type = { 059 CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = false, summary = true) 060 @Description(shortDefinition = "The type of relationship between the medicinal product indication or contraindication and another therapy", formalDefinition = "The type of relationship between the medicinal product indication or contraindication and another therapy.") 061 protected CodeableConcept therapyRelationshipType; 062 063 /** 064 * Reference to a specific medication (active substance, medicinal product or 065 * class of products) as part of an indication or contraindication. 066 */ 067 @Child(name = "medication", type = { CodeableConcept.class, MedicinalProduct.class, Medication.class, 068 Substance.class, SubstanceSpecification.class }, order = 2, min = 1, max = 1, modifier = false, summary = true) 069 @Description(shortDefinition = "Reference to a specific medication (active substance, medicinal product or class of products) as part of an indication or contraindication", formalDefinition = "Reference to a specific medication (active substance, medicinal product or class of products) as part of an indication or contraindication.") 070 protected Type medication; 071 072 private static final long serialVersionUID = 1438478115L; 073 074 /** 075 * Constructor 076 */ 077 public MedicinalProductIndicationOtherTherapyComponent() { 078 super(); 079 } 080 081 /** 082 * Constructor 083 */ 084 public MedicinalProductIndicationOtherTherapyComponent(CodeableConcept therapyRelationshipType, Type medication) { 085 super(); 086 this.therapyRelationshipType = therapyRelationshipType; 087 this.medication = medication; 088 } 089 090 /** 091 * @return {@link #therapyRelationshipType} (The type of relationship between 092 * the medicinal product indication or contraindication and another 093 * therapy.) 094 */ 095 public CodeableConcept getTherapyRelationshipType() { 096 if (this.therapyRelationshipType == null) 097 if (Configuration.errorOnAutoCreate()) 098 throw new Error( 099 "Attempt to auto-create MedicinalProductIndicationOtherTherapyComponent.therapyRelationshipType"); 100 else if (Configuration.doAutoCreate()) 101 this.therapyRelationshipType = new CodeableConcept(); // cc 102 return this.therapyRelationshipType; 103 } 104 105 public boolean hasTherapyRelationshipType() { 106 return this.therapyRelationshipType != null && !this.therapyRelationshipType.isEmpty(); 107 } 108 109 /** 110 * @param value {@link #therapyRelationshipType} (The type of relationship 111 * between the medicinal product indication or contraindication and 112 * another therapy.) 113 */ 114 public MedicinalProductIndicationOtherTherapyComponent setTherapyRelationshipType(CodeableConcept value) { 115 this.therapyRelationshipType = value; 116 return this; 117 } 118 119 /** 120 * @return {@link #medication} (Reference to a specific medication (active 121 * substance, medicinal product or class of products) as part of an 122 * indication or contraindication.) 123 */ 124 public Type getMedication() { 125 return this.medication; 126 } 127 128 /** 129 * @return {@link #medication} (Reference to a specific medication (active 130 * substance, medicinal product or class of products) as part of an 131 * indication or contraindication.) 132 */ 133 public CodeableConcept getMedicationCodeableConcept() throws FHIRException { 134 if (this.medication == null) 135 this.medication = new CodeableConcept(); 136 if (!(this.medication instanceof CodeableConcept)) 137 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but " 138 + this.medication.getClass().getName() + " was encountered"); 139 return (CodeableConcept) this.medication; 140 } 141 142 public boolean hasMedicationCodeableConcept() { 143 return this != null && this.medication instanceof CodeableConcept; 144 } 145 146 /** 147 * @return {@link #medication} (Reference to a specific medication (active 148 * substance, medicinal product or class of products) as part of an 149 * indication or contraindication.) 150 */ 151 public Reference getMedicationReference() throws FHIRException { 152 if (this.medication == null) 153 this.medication = new Reference(); 154 if (!(this.medication instanceof Reference)) 155 throw new FHIRException("Type mismatch: the type Reference was expected, but " 156 + this.medication.getClass().getName() + " was encountered"); 157 return (Reference) this.medication; 158 } 159 160 public boolean hasMedicationReference() { 161 return this != null && this.medication instanceof Reference; 162 } 163 164 public boolean hasMedication() { 165 return this.medication != null && !this.medication.isEmpty(); 166 } 167 168 /** 169 * @param value {@link #medication} (Reference to a specific medication (active 170 * substance, medicinal product or class of products) as part of an 171 * indication or contraindication.) 172 */ 173 public MedicinalProductIndicationOtherTherapyComponent setMedication(Type value) { 174 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 175 throw new Error( 176 "Not the right type for MedicinalProductIndication.otherTherapy.medication[x]: " + value.fhirType()); 177 this.medication = value; 178 return this; 179 } 180 181 protected void listChildren(List<Property> children) { 182 super.listChildren(children); 183 children.add(new Property("therapyRelationshipType", "CodeableConcept", 184 "The type of relationship between the medicinal product indication or contraindication and another therapy.", 185 0, 1, therapyRelationshipType)); 186 children.add(new Property("medication[x]", 187 "CodeableConcept|Reference(MedicinalProduct|Medication|Substance|SubstanceSpecification)", 188 "Reference to a specific medication (active substance, medicinal product or class of products) as part of an indication or contraindication.", 189 0, 1, medication)); 190 } 191 192 @Override 193 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 194 switch (_hash) { 195 case -551658469: 196 /* therapyRelationshipType */ return new Property("therapyRelationshipType", "CodeableConcept", 197 "The type of relationship between the medicinal product indication or contraindication and another therapy.", 198 0, 1, therapyRelationshipType); 199 case 1458402129: 200 /* medication[x] */ return new Property("medication[x]", 201 "CodeableConcept|Reference(MedicinalProduct|Medication|Substance|SubstanceSpecification)", 202 "Reference to a specific medication (active substance, medicinal product or class of products) as part of an indication or contraindication.", 203 0, 1, medication); 204 case 1998965455: 205 /* medication */ return new Property("medication[x]", 206 "CodeableConcept|Reference(MedicinalProduct|Medication|Substance|SubstanceSpecification)", 207 "Reference to a specific medication (active substance, medicinal product or class of products) as part of an indication or contraindication.", 208 0, 1, medication); 209 case -209845038: 210 /* medicationCodeableConcept */ return new Property("medication[x]", 211 "CodeableConcept|Reference(MedicinalProduct|Medication|Substance|SubstanceSpecification)", 212 "Reference to a specific medication (active substance, medicinal product or class of products) as part of an indication or contraindication.", 213 0, 1, medication); 214 case 2104315196: 215 /* medicationReference */ return new Property("medication[x]", 216 "CodeableConcept|Reference(MedicinalProduct|Medication|Substance|SubstanceSpecification)", 217 "Reference to a specific medication (active substance, medicinal product or class of products) as part of an indication or contraindication.", 218 0, 1, medication); 219 default: 220 return super.getNamedProperty(_hash, _name, _checkValid); 221 } 222 223 } 224 225 @Override 226 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 227 switch (hash) { 228 case -551658469: 229 /* therapyRelationshipType */ return this.therapyRelationshipType == null ? new Base[0] 230 : new Base[] { this.therapyRelationshipType }; // CodeableConcept 231 case 1998965455: 232 /* medication */ return this.medication == null ? new Base[0] : new Base[] { this.medication }; // Type 233 default: 234 return super.getProperty(hash, name, checkValid); 235 } 236 237 } 238 239 @Override 240 public Base setProperty(int hash, String name, Base value) throws FHIRException { 241 switch (hash) { 242 case -551658469: // therapyRelationshipType 243 this.therapyRelationshipType = castToCodeableConcept(value); // CodeableConcept 244 return value; 245 case 1998965455: // medication 246 this.medication = castToType(value); // Type 247 return value; 248 default: 249 return super.setProperty(hash, name, value); 250 } 251 252 } 253 254 @Override 255 public Base setProperty(String name, Base value) throws FHIRException { 256 if (name.equals("therapyRelationshipType")) { 257 this.therapyRelationshipType = castToCodeableConcept(value); // CodeableConcept 258 } else if (name.equals("medication[x]")) { 259 this.medication = castToType(value); // Type 260 } else 261 return super.setProperty(name, value); 262 return value; 263 } 264 265 @Override 266 public Base makeProperty(int hash, String name) throws FHIRException { 267 switch (hash) { 268 case -551658469: 269 return getTherapyRelationshipType(); 270 case 1458402129: 271 return getMedication(); 272 case 1998965455: 273 return getMedication(); 274 default: 275 return super.makeProperty(hash, name); 276 } 277 278 } 279 280 @Override 281 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 282 switch (hash) { 283 case -551658469: 284 /* therapyRelationshipType */ return new String[] { "CodeableConcept" }; 285 case 1998965455: 286 /* medication */ return new String[] { "CodeableConcept", "Reference" }; 287 default: 288 return super.getTypesForProperty(hash, name); 289 } 290 291 } 292 293 @Override 294 public Base addChild(String name) throws FHIRException { 295 if (name.equals("therapyRelationshipType")) { 296 this.therapyRelationshipType = new CodeableConcept(); 297 return this.therapyRelationshipType; 298 } else if (name.equals("medicationCodeableConcept")) { 299 this.medication = new CodeableConcept(); 300 return this.medication; 301 } else if (name.equals("medicationReference")) { 302 this.medication = new Reference(); 303 return this.medication; 304 } else 305 return super.addChild(name); 306 } 307 308 public MedicinalProductIndicationOtherTherapyComponent copy() { 309 MedicinalProductIndicationOtherTherapyComponent dst = new MedicinalProductIndicationOtherTherapyComponent(); 310 copyValues(dst); 311 return dst; 312 } 313 314 public void copyValues(MedicinalProductIndicationOtherTherapyComponent dst) { 315 super.copyValues(dst); 316 dst.therapyRelationshipType = therapyRelationshipType == null ? null : therapyRelationshipType.copy(); 317 dst.medication = medication == null ? null : medication.copy(); 318 } 319 320 @Override 321 public boolean equalsDeep(Base other_) { 322 if (!super.equalsDeep(other_)) 323 return false; 324 if (!(other_ instanceof MedicinalProductIndicationOtherTherapyComponent)) 325 return false; 326 MedicinalProductIndicationOtherTherapyComponent o = (MedicinalProductIndicationOtherTherapyComponent) other_; 327 return compareDeep(therapyRelationshipType, o.therapyRelationshipType, true) 328 && compareDeep(medication, o.medication, true); 329 } 330 331 @Override 332 public boolean equalsShallow(Base other_) { 333 if (!super.equalsShallow(other_)) 334 return false; 335 if (!(other_ instanceof MedicinalProductIndicationOtherTherapyComponent)) 336 return false; 337 MedicinalProductIndicationOtherTherapyComponent o = (MedicinalProductIndicationOtherTherapyComponent) other_; 338 return true; 339 } 340 341 public boolean isEmpty() { 342 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(therapyRelationshipType, medication); 343 } 344 345 public String fhirType() { 346 return "MedicinalProductIndication.otherTherapy"; 347 348 } 349 350 } 351 352 /** 353 * The medication for which this is an indication. 354 */ 355 @Child(name = "subject", type = { MedicinalProduct.class, 356 Medication.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 357 @Description(shortDefinition = "The medication for which this is an indication", formalDefinition = "The medication for which this is an indication.") 358 protected List<Reference> subject; 359 /** 360 * The actual objects that are the target of the reference (The medication for 361 * which this is an indication.) 362 */ 363 protected List<Resource> subjectTarget; 364 365 /** 366 * The disease, symptom or procedure that is the indication for treatment. 367 */ 368 @Child(name = "diseaseSymptomProcedure", type = { 369 CodeableConcept.class }, order = 1, min = 0, max = 1, modifier = false, summary = true) 370 @Description(shortDefinition = "The disease, symptom or procedure that is the indication for treatment", formalDefinition = "The disease, symptom or procedure that is the indication for treatment.") 371 protected CodeableConcept diseaseSymptomProcedure; 372 373 /** 374 * The status of the disease or symptom for which the indication applies. 375 */ 376 @Child(name = "diseaseStatus", type = { 377 CodeableConcept.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 378 @Description(shortDefinition = "The status of the disease or symptom for which the indication applies", formalDefinition = "The status of the disease or symptom for which the indication applies.") 379 protected CodeableConcept diseaseStatus; 380 381 /** 382 * Comorbidity (concurrent condition) or co-infection as part of the indication. 383 */ 384 @Child(name = "comorbidity", type = { 385 CodeableConcept.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 386 @Description(shortDefinition = "Comorbidity (concurrent condition) or co-infection as part of the indication", formalDefinition = "Comorbidity (concurrent condition) or co-infection as part of the indication.") 387 protected List<CodeableConcept> comorbidity; 388 389 /** 390 * The intended effect, aim or strategy to be achieved by the indication. 391 */ 392 @Child(name = "intendedEffect", type = { 393 CodeableConcept.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 394 @Description(shortDefinition = "The intended effect, aim or strategy to be achieved by the indication", formalDefinition = "The intended effect, aim or strategy to be achieved by the indication.") 395 protected CodeableConcept intendedEffect; 396 397 /** 398 * Timing or duration information as part of the indication. 399 */ 400 @Child(name = "duration", type = { Quantity.class }, order = 5, min = 0, max = 1, modifier = false, summary = true) 401 @Description(shortDefinition = "Timing or duration information as part of the indication", formalDefinition = "Timing or duration information as part of the indication.") 402 protected Quantity duration; 403 404 /** 405 * Information about the use of the medicinal product in relation to other 406 * therapies described as part of the indication. 407 */ 408 @Child(name = "otherTherapy", type = {}, order = 6, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 409 @Description(shortDefinition = "Information about the use of the medicinal product in relation to other therapies described as part of the indication", formalDefinition = "Information about the use of the medicinal product in relation to other therapies described as part of the indication.") 410 protected List<MedicinalProductIndicationOtherTherapyComponent> otherTherapy; 411 412 /** 413 * Describe the undesirable effects of the medicinal product. 414 */ 415 @Child(name = "undesirableEffect", type = { 416 MedicinalProductUndesirableEffect.class }, order = 7, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 417 @Description(shortDefinition = "Describe the undesirable effects of the medicinal product", formalDefinition = "Describe the undesirable effects of the medicinal product.") 418 protected List<Reference> undesirableEffect; 419 /** 420 * The actual objects that are the target of the reference (Describe the 421 * undesirable effects of the medicinal product.) 422 */ 423 protected List<MedicinalProductUndesirableEffect> undesirableEffectTarget; 424 425 /** 426 * The population group to which this applies. 427 */ 428 @Child(name = "population", type = { 429 Population.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 430 @Description(shortDefinition = "The population group to which this applies", formalDefinition = "The population group to which this applies.") 431 protected List<Population> population; 432 433 private static final long serialVersionUID = 1205519664L; 434 435 /** 436 * Constructor 437 */ 438 public MedicinalProductIndication() { 439 super(); 440 } 441 442 /** 443 * @return {@link #subject} (The medication for which this is an indication.) 444 */ 445 public List<Reference> getSubject() { 446 if (this.subject == null) 447 this.subject = new ArrayList<Reference>(); 448 return this.subject; 449 } 450 451 /** 452 * @return Returns a reference to <code>this</code> for easy method chaining 453 */ 454 public MedicinalProductIndication setSubject(List<Reference> theSubject) { 455 this.subject = theSubject; 456 return this; 457 } 458 459 public boolean hasSubject() { 460 if (this.subject == null) 461 return false; 462 for (Reference item : this.subject) 463 if (!item.isEmpty()) 464 return true; 465 return false; 466 } 467 468 public Reference addSubject() { // 3 469 Reference t = new Reference(); 470 if (this.subject == null) 471 this.subject = new ArrayList<Reference>(); 472 this.subject.add(t); 473 return t; 474 } 475 476 public MedicinalProductIndication addSubject(Reference t) { // 3 477 if (t == null) 478 return this; 479 if (this.subject == null) 480 this.subject = new ArrayList<Reference>(); 481 this.subject.add(t); 482 return this; 483 } 484 485 /** 486 * @return The first repetition of repeating field {@link #subject}, creating it 487 * if it does not already exist 488 */ 489 public Reference getSubjectFirstRep() { 490 if (getSubject().isEmpty()) { 491 addSubject(); 492 } 493 return getSubject().get(0); 494 } 495 496 /** 497 * @deprecated Use Reference#setResource(IBaseResource) instead 498 */ 499 @Deprecated 500 public List<Resource> getSubjectTarget() { 501 if (this.subjectTarget == null) 502 this.subjectTarget = new ArrayList<Resource>(); 503 return this.subjectTarget; 504 } 505 506 /** 507 * @return {@link #diseaseSymptomProcedure} (The disease, symptom or procedure 508 * that is the indication for treatment.) 509 */ 510 public CodeableConcept getDiseaseSymptomProcedure() { 511 if (this.diseaseSymptomProcedure == null) 512 if (Configuration.errorOnAutoCreate()) 513 throw new Error("Attempt to auto-create MedicinalProductIndication.diseaseSymptomProcedure"); 514 else if (Configuration.doAutoCreate()) 515 this.diseaseSymptomProcedure = new CodeableConcept(); // cc 516 return this.diseaseSymptomProcedure; 517 } 518 519 public boolean hasDiseaseSymptomProcedure() { 520 return this.diseaseSymptomProcedure != null && !this.diseaseSymptomProcedure.isEmpty(); 521 } 522 523 /** 524 * @param value {@link #diseaseSymptomProcedure} (The disease, symptom or 525 * procedure that is the indication for treatment.) 526 */ 527 public MedicinalProductIndication setDiseaseSymptomProcedure(CodeableConcept value) { 528 this.diseaseSymptomProcedure = value; 529 return this; 530 } 531 532 /** 533 * @return {@link #diseaseStatus} (The status of the disease or symptom for 534 * which the indication applies.) 535 */ 536 public CodeableConcept getDiseaseStatus() { 537 if (this.diseaseStatus == null) 538 if (Configuration.errorOnAutoCreate()) 539 throw new Error("Attempt to auto-create MedicinalProductIndication.diseaseStatus"); 540 else if (Configuration.doAutoCreate()) 541 this.diseaseStatus = new CodeableConcept(); // cc 542 return this.diseaseStatus; 543 } 544 545 public boolean hasDiseaseStatus() { 546 return this.diseaseStatus != null && !this.diseaseStatus.isEmpty(); 547 } 548 549 /** 550 * @param value {@link #diseaseStatus} (The status of the disease or symptom for 551 * which the indication applies.) 552 */ 553 public MedicinalProductIndication setDiseaseStatus(CodeableConcept value) { 554 this.diseaseStatus = value; 555 return this; 556 } 557 558 /** 559 * @return {@link #comorbidity} (Comorbidity (concurrent condition) or 560 * co-infection as part of the indication.) 561 */ 562 public List<CodeableConcept> getComorbidity() { 563 if (this.comorbidity == null) 564 this.comorbidity = new ArrayList<CodeableConcept>(); 565 return this.comorbidity; 566 } 567 568 /** 569 * @return Returns a reference to <code>this</code> for easy method chaining 570 */ 571 public MedicinalProductIndication setComorbidity(List<CodeableConcept> theComorbidity) { 572 this.comorbidity = theComorbidity; 573 return this; 574 } 575 576 public boolean hasComorbidity() { 577 if (this.comorbidity == null) 578 return false; 579 for (CodeableConcept item : this.comorbidity) 580 if (!item.isEmpty()) 581 return true; 582 return false; 583 } 584 585 public CodeableConcept addComorbidity() { // 3 586 CodeableConcept t = new CodeableConcept(); 587 if (this.comorbidity == null) 588 this.comorbidity = new ArrayList<CodeableConcept>(); 589 this.comorbidity.add(t); 590 return t; 591 } 592 593 public MedicinalProductIndication addComorbidity(CodeableConcept t) { // 3 594 if (t == null) 595 return this; 596 if (this.comorbidity == null) 597 this.comorbidity = new ArrayList<CodeableConcept>(); 598 this.comorbidity.add(t); 599 return this; 600 } 601 602 /** 603 * @return The first repetition of repeating field {@link #comorbidity}, 604 * creating it if it does not already exist 605 */ 606 public CodeableConcept getComorbidityFirstRep() { 607 if (getComorbidity().isEmpty()) { 608 addComorbidity(); 609 } 610 return getComorbidity().get(0); 611 } 612 613 /** 614 * @return {@link #intendedEffect} (The intended effect, aim or strategy to be 615 * achieved by the indication.) 616 */ 617 public CodeableConcept getIntendedEffect() { 618 if (this.intendedEffect == null) 619 if (Configuration.errorOnAutoCreate()) 620 throw new Error("Attempt to auto-create MedicinalProductIndication.intendedEffect"); 621 else if (Configuration.doAutoCreate()) 622 this.intendedEffect = new CodeableConcept(); // cc 623 return this.intendedEffect; 624 } 625 626 public boolean hasIntendedEffect() { 627 return this.intendedEffect != null && !this.intendedEffect.isEmpty(); 628 } 629 630 /** 631 * @param value {@link #intendedEffect} (The intended effect, aim or strategy to 632 * be achieved by the indication.) 633 */ 634 public MedicinalProductIndication setIntendedEffect(CodeableConcept value) { 635 this.intendedEffect = value; 636 return this; 637 } 638 639 /** 640 * @return {@link #duration} (Timing or duration information as part of the 641 * indication.) 642 */ 643 public Quantity getDuration() { 644 if (this.duration == null) 645 if (Configuration.errorOnAutoCreate()) 646 throw new Error("Attempt to auto-create MedicinalProductIndication.duration"); 647 else if (Configuration.doAutoCreate()) 648 this.duration = new Quantity(); // cc 649 return this.duration; 650 } 651 652 public boolean hasDuration() { 653 return this.duration != null && !this.duration.isEmpty(); 654 } 655 656 /** 657 * @param value {@link #duration} (Timing or duration information as part of the 658 * indication.) 659 */ 660 public MedicinalProductIndication setDuration(Quantity value) { 661 this.duration = value; 662 return this; 663 } 664 665 /** 666 * @return {@link #otherTherapy} (Information about the use of the medicinal 667 * product in relation to other therapies described as part of the 668 * indication.) 669 */ 670 public List<MedicinalProductIndicationOtherTherapyComponent> getOtherTherapy() { 671 if (this.otherTherapy == null) 672 this.otherTherapy = new ArrayList<MedicinalProductIndicationOtherTherapyComponent>(); 673 return this.otherTherapy; 674 } 675 676 /** 677 * @return Returns a reference to <code>this</code> for easy method chaining 678 */ 679 public MedicinalProductIndication setOtherTherapy( 680 List<MedicinalProductIndicationOtherTherapyComponent> theOtherTherapy) { 681 this.otherTherapy = theOtherTherapy; 682 return this; 683 } 684 685 public boolean hasOtherTherapy() { 686 if (this.otherTherapy == null) 687 return false; 688 for (MedicinalProductIndicationOtherTherapyComponent item : this.otherTherapy) 689 if (!item.isEmpty()) 690 return true; 691 return false; 692 } 693 694 public MedicinalProductIndicationOtherTherapyComponent addOtherTherapy() { // 3 695 MedicinalProductIndicationOtherTherapyComponent t = new MedicinalProductIndicationOtherTherapyComponent(); 696 if (this.otherTherapy == null) 697 this.otherTherapy = new ArrayList<MedicinalProductIndicationOtherTherapyComponent>(); 698 this.otherTherapy.add(t); 699 return t; 700 } 701 702 public MedicinalProductIndication addOtherTherapy(MedicinalProductIndicationOtherTherapyComponent t) { // 3 703 if (t == null) 704 return this; 705 if (this.otherTherapy == null) 706 this.otherTherapy = new ArrayList<MedicinalProductIndicationOtherTherapyComponent>(); 707 this.otherTherapy.add(t); 708 return this; 709 } 710 711 /** 712 * @return The first repetition of repeating field {@link #otherTherapy}, 713 * creating it if it does not already exist 714 */ 715 public MedicinalProductIndicationOtherTherapyComponent getOtherTherapyFirstRep() { 716 if (getOtherTherapy().isEmpty()) { 717 addOtherTherapy(); 718 } 719 return getOtherTherapy().get(0); 720 } 721 722 /** 723 * @return {@link #undesirableEffect} (Describe the undesirable effects of the 724 * medicinal product.) 725 */ 726 public List<Reference> getUndesirableEffect() { 727 if (this.undesirableEffect == null) 728 this.undesirableEffect = new ArrayList<Reference>(); 729 return this.undesirableEffect; 730 } 731 732 /** 733 * @return Returns a reference to <code>this</code> for easy method chaining 734 */ 735 public MedicinalProductIndication setUndesirableEffect(List<Reference> theUndesirableEffect) { 736 this.undesirableEffect = theUndesirableEffect; 737 return this; 738 } 739 740 public boolean hasUndesirableEffect() { 741 if (this.undesirableEffect == null) 742 return false; 743 for (Reference item : this.undesirableEffect) 744 if (!item.isEmpty()) 745 return true; 746 return false; 747 } 748 749 public Reference addUndesirableEffect() { // 3 750 Reference t = new Reference(); 751 if (this.undesirableEffect == null) 752 this.undesirableEffect = new ArrayList<Reference>(); 753 this.undesirableEffect.add(t); 754 return t; 755 } 756 757 public MedicinalProductIndication addUndesirableEffect(Reference t) { // 3 758 if (t == null) 759 return this; 760 if (this.undesirableEffect == null) 761 this.undesirableEffect = new ArrayList<Reference>(); 762 this.undesirableEffect.add(t); 763 return this; 764 } 765 766 /** 767 * @return The first repetition of repeating field {@link #undesirableEffect}, 768 * creating it if it does not already exist 769 */ 770 public Reference getUndesirableEffectFirstRep() { 771 if (getUndesirableEffect().isEmpty()) { 772 addUndesirableEffect(); 773 } 774 return getUndesirableEffect().get(0); 775 } 776 777 /** 778 * @deprecated Use Reference#setResource(IBaseResource) instead 779 */ 780 @Deprecated 781 public List<MedicinalProductUndesirableEffect> getUndesirableEffectTarget() { 782 if (this.undesirableEffectTarget == null) 783 this.undesirableEffectTarget = new ArrayList<MedicinalProductUndesirableEffect>(); 784 return this.undesirableEffectTarget; 785 } 786 787 /** 788 * @deprecated Use Reference#setResource(IBaseResource) instead 789 */ 790 @Deprecated 791 public MedicinalProductUndesirableEffect addUndesirableEffectTarget() { 792 MedicinalProductUndesirableEffect r = new MedicinalProductUndesirableEffect(); 793 if (this.undesirableEffectTarget == null) 794 this.undesirableEffectTarget = new ArrayList<MedicinalProductUndesirableEffect>(); 795 this.undesirableEffectTarget.add(r); 796 return r; 797 } 798 799 /** 800 * @return {@link #population} (The population group to which this applies.) 801 */ 802 public List<Population> getPopulation() { 803 if (this.population == null) 804 this.population = new ArrayList<Population>(); 805 return this.population; 806 } 807 808 /** 809 * @return Returns a reference to <code>this</code> for easy method chaining 810 */ 811 public MedicinalProductIndication setPopulation(List<Population> thePopulation) { 812 this.population = thePopulation; 813 return this; 814 } 815 816 public boolean hasPopulation() { 817 if (this.population == null) 818 return false; 819 for (Population item : this.population) 820 if (!item.isEmpty()) 821 return true; 822 return false; 823 } 824 825 public Population addPopulation() { // 3 826 Population t = new Population(); 827 if (this.population == null) 828 this.population = new ArrayList<Population>(); 829 this.population.add(t); 830 return t; 831 } 832 833 public MedicinalProductIndication addPopulation(Population t) { // 3 834 if (t == null) 835 return this; 836 if (this.population == null) 837 this.population = new ArrayList<Population>(); 838 this.population.add(t); 839 return this; 840 } 841 842 /** 843 * @return The first repetition of repeating field {@link #population}, creating 844 * it if it does not already exist 845 */ 846 public Population getPopulationFirstRep() { 847 if (getPopulation().isEmpty()) { 848 addPopulation(); 849 } 850 return getPopulation().get(0); 851 } 852 853 protected void listChildren(List<Property> children) { 854 super.listChildren(children); 855 children.add(new Property("subject", "Reference(MedicinalProduct|Medication)", 856 "The medication for which this is an indication.", 0, java.lang.Integer.MAX_VALUE, subject)); 857 children.add(new Property("diseaseSymptomProcedure", "CodeableConcept", 858 "The disease, symptom or procedure that is the indication for treatment.", 0, 1, diseaseSymptomProcedure)); 859 children.add(new Property("diseaseStatus", "CodeableConcept", 860 "The status of the disease or symptom for which the indication applies.", 0, 1, diseaseStatus)); 861 children.add(new Property("comorbidity", "CodeableConcept", 862 "Comorbidity (concurrent condition) or co-infection as part of the indication.", 0, java.lang.Integer.MAX_VALUE, 863 comorbidity)); 864 children.add(new Property("intendedEffect", "CodeableConcept", 865 "The intended effect, aim or strategy to be achieved by the indication.", 0, 1, intendedEffect)); 866 children.add(new Property("duration", "Quantity", "Timing or duration information as part of the indication.", 0, 1, 867 duration)); 868 children.add(new Property("otherTherapy", "", 869 "Information about the use of the medicinal product in relation to other therapies described as part of the indication.", 870 0, java.lang.Integer.MAX_VALUE, otherTherapy)); 871 children.add(new Property("undesirableEffect", "Reference(MedicinalProductUndesirableEffect)", 872 "Describe the undesirable effects of the medicinal product.", 0, java.lang.Integer.MAX_VALUE, 873 undesirableEffect)); 874 children.add(new Property("population", "Population", "The population group to which this applies.", 0, 875 java.lang.Integer.MAX_VALUE, population)); 876 } 877 878 @Override 879 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 880 switch (_hash) { 881 case -1867885268: 882 /* subject */ return new Property("subject", "Reference(MedicinalProduct|Medication)", 883 "The medication for which this is an indication.", 0, java.lang.Integer.MAX_VALUE, subject); 884 case -1497395130: 885 /* diseaseSymptomProcedure */ return new Property("diseaseSymptomProcedure", "CodeableConcept", 886 "The disease, symptom or procedure that is the indication for treatment.", 0, 1, diseaseSymptomProcedure); 887 case -505503602: 888 /* diseaseStatus */ return new Property("diseaseStatus", "CodeableConcept", 889 "The status of the disease or symptom for which the indication applies.", 0, 1, diseaseStatus); 890 case -406395211: 891 /* comorbidity */ return new Property("comorbidity", "CodeableConcept", 892 "Comorbidity (concurrent condition) or co-infection as part of the indication.", 0, 893 java.lang.Integer.MAX_VALUE, comorbidity); 894 case 1587112348: 895 /* intendedEffect */ return new Property("intendedEffect", "CodeableConcept", 896 "The intended effect, aim or strategy to be achieved by the indication.", 0, 1, intendedEffect); 897 case -1992012396: 898 /* duration */ return new Property("duration", "Quantity", 899 "Timing or duration information as part of the indication.", 0, 1, duration); 900 case -544509127: 901 /* otherTherapy */ return new Property("otherTherapy", "", 902 "Information about the use of the medicinal product in relation to other therapies described as part of the indication.", 903 0, java.lang.Integer.MAX_VALUE, otherTherapy); 904 case 444367565: 905 /* undesirableEffect */ return new Property("undesirableEffect", "Reference(MedicinalProductUndesirableEffect)", 906 "Describe the undesirable effects of the medicinal product.", 0, java.lang.Integer.MAX_VALUE, 907 undesirableEffect); 908 case -2023558323: 909 /* population */ return new Property("population", "Population", "The population group to which this applies.", 0, 910 java.lang.Integer.MAX_VALUE, population); 911 default: 912 return super.getNamedProperty(_hash, _name, _checkValid); 913 } 914 915 } 916 917 @Override 918 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 919 switch (hash) { 920 case -1867885268: 921 /* subject */ return this.subject == null ? new Base[0] : this.subject.toArray(new Base[this.subject.size()]); // Reference 922 case -1497395130: 923 /* diseaseSymptomProcedure */ return this.diseaseSymptomProcedure == null ? new Base[0] 924 : new Base[] { this.diseaseSymptomProcedure }; // CodeableConcept 925 case -505503602: 926 /* diseaseStatus */ return this.diseaseStatus == null ? new Base[0] : new Base[] { this.diseaseStatus }; // CodeableConcept 927 case -406395211: 928 /* comorbidity */ return this.comorbidity == null ? new Base[0] 929 : this.comorbidity.toArray(new Base[this.comorbidity.size()]); // CodeableConcept 930 case 1587112348: 931 /* intendedEffect */ return this.intendedEffect == null ? new Base[0] : new Base[] { this.intendedEffect }; // CodeableConcept 932 case -1992012396: 933 /* duration */ return this.duration == null ? new Base[0] : new Base[] { this.duration }; // Quantity 934 case -544509127: 935 /* otherTherapy */ return this.otherTherapy == null ? new Base[0] 936 : this.otherTherapy.toArray(new Base[this.otherTherapy.size()]); // MedicinalProductIndicationOtherTherapyComponent 937 case 444367565: 938 /* undesirableEffect */ return this.undesirableEffect == null ? new Base[0] 939 : this.undesirableEffect.toArray(new Base[this.undesirableEffect.size()]); // Reference 940 case -2023558323: 941 /* population */ return this.population == null ? new Base[0] 942 : this.population.toArray(new Base[this.population.size()]); // Population 943 default: 944 return super.getProperty(hash, name, checkValid); 945 } 946 947 } 948 949 @Override 950 public Base setProperty(int hash, String name, Base value) throws FHIRException { 951 switch (hash) { 952 case -1867885268: // subject 953 this.getSubject().add(castToReference(value)); // Reference 954 return value; 955 case -1497395130: // diseaseSymptomProcedure 956 this.diseaseSymptomProcedure = castToCodeableConcept(value); // CodeableConcept 957 return value; 958 case -505503602: // diseaseStatus 959 this.diseaseStatus = castToCodeableConcept(value); // CodeableConcept 960 return value; 961 case -406395211: // comorbidity 962 this.getComorbidity().add(castToCodeableConcept(value)); // CodeableConcept 963 return value; 964 case 1587112348: // intendedEffect 965 this.intendedEffect = castToCodeableConcept(value); // CodeableConcept 966 return value; 967 case -1992012396: // duration 968 this.duration = castToQuantity(value); // Quantity 969 return value; 970 case -544509127: // otherTherapy 971 this.getOtherTherapy().add((MedicinalProductIndicationOtherTherapyComponent) value); // MedicinalProductIndicationOtherTherapyComponent 972 return value; 973 case 444367565: // undesirableEffect 974 this.getUndesirableEffect().add(castToReference(value)); // Reference 975 return value; 976 case -2023558323: // population 977 this.getPopulation().add(castToPopulation(value)); // Population 978 return value; 979 default: 980 return super.setProperty(hash, name, value); 981 } 982 983 } 984 985 @Override 986 public Base setProperty(String name, Base value) throws FHIRException { 987 if (name.equals("subject")) { 988 this.getSubject().add(castToReference(value)); 989 } else if (name.equals("diseaseSymptomProcedure")) { 990 this.diseaseSymptomProcedure = castToCodeableConcept(value); // CodeableConcept 991 } else if (name.equals("diseaseStatus")) { 992 this.diseaseStatus = castToCodeableConcept(value); // CodeableConcept 993 } else if (name.equals("comorbidity")) { 994 this.getComorbidity().add(castToCodeableConcept(value)); 995 } else if (name.equals("intendedEffect")) { 996 this.intendedEffect = castToCodeableConcept(value); // CodeableConcept 997 } else if (name.equals("duration")) { 998 this.duration = castToQuantity(value); // Quantity 999 } else if (name.equals("otherTherapy")) { 1000 this.getOtherTherapy().add((MedicinalProductIndicationOtherTherapyComponent) value); 1001 } else if (name.equals("undesirableEffect")) { 1002 this.getUndesirableEffect().add(castToReference(value)); 1003 } else if (name.equals("population")) { 1004 this.getPopulation().add(castToPopulation(value)); 1005 } else 1006 return super.setProperty(name, value); 1007 return value; 1008 } 1009 1010 @Override 1011 public Base makeProperty(int hash, String name) throws FHIRException { 1012 switch (hash) { 1013 case -1867885268: 1014 return addSubject(); 1015 case -1497395130: 1016 return getDiseaseSymptomProcedure(); 1017 case -505503602: 1018 return getDiseaseStatus(); 1019 case -406395211: 1020 return addComorbidity(); 1021 case 1587112348: 1022 return getIntendedEffect(); 1023 case -1992012396: 1024 return getDuration(); 1025 case -544509127: 1026 return addOtherTherapy(); 1027 case 444367565: 1028 return addUndesirableEffect(); 1029 case -2023558323: 1030 return addPopulation(); 1031 default: 1032 return super.makeProperty(hash, name); 1033 } 1034 1035 } 1036 1037 @Override 1038 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1039 switch (hash) { 1040 case -1867885268: 1041 /* subject */ return new String[] { "Reference" }; 1042 case -1497395130: 1043 /* diseaseSymptomProcedure */ return new String[] { "CodeableConcept" }; 1044 case -505503602: 1045 /* diseaseStatus */ return new String[] { "CodeableConcept" }; 1046 case -406395211: 1047 /* comorbidity */ return new String[] { "CodeableConcept" }; 1048 case 1587112348: 1049 /* intendedEffect */ return new String[] { "CodeableConcept" }; 1050 case -1992012396: 1051 /* duration */ return new String[] { "Quantity" }; 1052 case -544509127: 1053 /* otherTherapy */ return new String[] {}; 1054 case 444367565: 1055 /* undesirableEffect */ return new String[] { "Reference" }; 1056 case -2023558323: 1057 /* population */ return new String[] { "Population" }; 1058 default: 1059 return super.getTypesForProperty(hash, name); 1060 } 1061 1062 } 1063 1064 @Override 1065 public Base addChild(String name) throws FHIRException { 1066 if (name.equals("subject")) { 1067 return addSubject(); 1068 } else if (name.equals("diseaseSymptomProcedure")) { 1069 this.diseaseSymptomProcedure = new CodeableConcept(); 1070 return this.diseaseSymptomProcedure; 1071 } else if (name.equals("diseaseStatus")) { 1072 this.diseaseStatus = new CodeableConcept(); 1073 return this.diseaseStatus; 1074 } else if (name.equals("comorbidity")) { 1075 return addComorbidity(); 1076 } else if (name.equals("intendedEffect")) { 1077 this.intendedEffect = new CodeableConcept(); 1078 return this.intendedEffect; 1079 } else if (name.equals("duration")) { 1080 this.duration = new Quantity(); 1081 return this.duration; 1082 } else if (name.equals("otherTherapy")) { 1083 return addOtherTherapy(); 1084 } else if (name.equals("undesirableEffect")) { 1085 return addUndesirableEffect(); 1086 } else if (name.equals("population")) { 1087 return addPopulation(); 1088 } else 1089 return super.addChild(name); 1090 } 1091 1092 public String fhirType() { 1093 return "MedicinalProductIndication"; 1094 1095 } 1096 1097 public MedicinalProductIndication copy() { 1098 MedicinalProductIndication dst = new MedicinalProductIndication(); 1099 copyValues(dst); 1100 return dst; 1101 } 1102 1103 public void copyValues(MedicinalProductIndication dst) { 1104 super.copyValues(dst); 1105 if (subject != null) { 1106 dst.subject = new ArrayList<Reference>(); 1107 for (Reference i : subject) 1108 dst.subject.add(i.copy()); 1109 } 1110 ; 1111 dst.diseaseSymptomProcedure = diseaseSymptomProcedure == null ? null : diseaseSymptomProcedure.copy(); 1112 dst.diseaseStatus = diseaseStatus == null ? null : diseaseStatus.copy(); 1113 if (comorbidity != null) { 1114 dst.comorbidity = new ArrayList<CodeableConcept>(); 1115 for (CodeableConcept i : comorbidity) 1116 dst.comorbidity.add(i.copy()); 1117 } 1118 ; 1119 dst.intendedEffect = intendedEffect == null ? null : intendedEffect.copy(); 1120 dst.duration = duration == null ? null : duration.copy(); 1121 if (otherTherapy != null) { 1122 dst.otherTherapy = new ArrayList<MedicinalProductIndicationOtherTherapyComponent>(); 1123 for (MedicinalProductIndicationOtherTherapyComponent i : otherTherapy) 1124 dst.otherTherapy.add(i.copy()); 1125 } 1126 ; 1127 if (undesirableEffect != null) { 1128 dst.undesirableEffect = new ArrayList<Reference>(); 1129 for (Reference i : undesirableEffect) 1130 dst.undesirableEffect.add(i.copy()); 1131 } 1132 ; 1133 if (population != null) { 1134 dst.population = new ArrayList<Population>(); 1135 for (Population i : population) 1136 dst.population.add(i.copy()); 1137 } 1138 ; 1139 } 1140 1141 protected MedicinalProductIndication typedCopy() { 1142 return copy(); 1143 } 1144 1145 @Override 1146 public boolean equalsDeep(Base other_) { 1147 if (!super.equalsDeep(other_)) 1148 return false; 1149 if (!(other_ instanceof MedicinalProductIndication)) 1150 return false; 1151 MedicinalProductIndication o = (MedicinalProductIndication) other_; 1152 return compareDeep(subject, o.subject, true) 1153 && compareDeep(diseaseSymptomProcedure, o.diseaseSymptomProcedure, true) 1154 && compareDeep(diseaseStatus, o.diseaseStatus, true) && compareDeep(comorbidity, o.comorbidity, true) 1155 && compareDeep(intendedEffect, o.intendedEffect, true) && compareDeep(duration, o.duration, true) 1156 && compareDeep(otherTherapy, o.otherTherapy, true) && compareDeep(undesirableEffect, o.undesirableEffect, true) 1157 && compareDeep(population, o.population, true); 1158 } 1159 1160 @Override 1161 public boolean equalsShallow(Base other_) { 1162 if (!super.equalsShallow(other_)) 1163 return false; 1164 if (!(other_ instanceof MedicinalProductIndication)) 1165 return false; 1166 MedicinalProductIndication o = (MedicinalProductIndication) other_; 1167 return true; 1168 } 1169 1170 public boolean isEmpty() { 1171 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(subject, diseaseSymptomProcedure, diseaseStatus, 1172 comorbidity, intendedEffect, duration, otherTherapy, undesirableEffect, population); 1173 } 1174 1175 @Override 1176 public ResourceType getResourceType() { 1177 return ResourceType.MedicinalProductIndication; 1178 } 1179 1180 /** 1181 * Search parameter: <b>subject</b> 1182 * <p> 1183 * Description: <b>The medication for which this is an indication</b><br> 1184 * Type: <b>reference</b><br> 1185 * Path: <b>MedicinalProductIndication.subject</b><br> 1186 * </p> 1187 */ 1188 @SearchParamDefinition(name = "subject", path = "MedicinalProductIndication.subject", description = "The medication for which this is an indication", type = "reference", target = { 1189 Medication.class, MedicinalProduct.class }) 1190 public static final String SP_SUBJECT = "subject"; 1191 /** 1192 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 1193 * <p> 1194 * Description: <b>The medication for which this is an indication</b><br> 1195 * Type: <b>reference</b><br> 1196 * Path: <b>MedicinalProductIndication.subject</b><br> 1197 * </p> 1198 */ 1199 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 1200 SP_SUBJECT); 1201 1202 /** 1203 * Constant for fluent queries to be used to add include statements. Specifies 1204 * the path value of "<b>MedicinalProductIndication:subject</b>". 1205 */ 1206 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include( 1207 "MedicinalProductIndication:subject").toLocked(); 1208 1209}