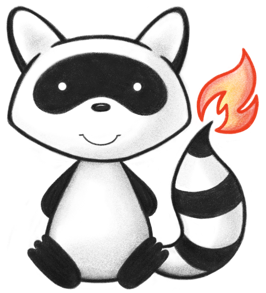
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.r4.model.Enumerations.PublicationStatus; 039import org.hl7.fhir.r4.model.Enumerations.PublicationStatusEnumFactory; 040import org.hl7.fhir.utilities.Utilities; 041 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.ChildOrder; 044import ca.uhn.fhir.model.api.annotation.Description; 045import ca.uhn.fhir.model.api.annotation.ResourceDef; 046import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 047 048/** 049 * The Evidence resource describes the conditional state (population and any 050 * exposures being compared within the population) and outcome (if specified) 051 * that the knowledge (evidence, assertion, recommendation) is about. 052 */ 053@ResourceDef(name = "Evidence", profile = "http://hl7.org/fhir/StructureDefinition/Evidence") 054@ChildOrder(names = { "url", "identifier", "version", "name", "title", "shortTitle", "subtitle", "status", "date", 055 "publisher", "contact", "description", "note", "useContext", "jurisdiction", "copyright", "approvalDate", 056 "lastReviewDate", "effectivePeriod", "topic", "author", "editor", "reviewer", "endorser", "relatedArtifact", 057 "exposureBackground", "exposureVariant", "outcome" }) 058public class Evidence extends MetadataResource { 059 060 /** 061 * A formal identifier that is used to identify this evidence when it is 062 * represented in other formats, or referenced in a specification, model, design 063 * or an instance. 064 */ 065 @Child(name = "identifier", type = { 066 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 067 @Description(shortDefinition = "Additional identifier for the evidence", formalDefinition = "A formal identifier that is used to identify this evidence when it is represented in other formats, or referenced in a specification, model, design or an instance.") 068 protected List<Identifier> identifier; 069 070 /** 071 * The short title provides an alternate title for use in informal descriptive 072 * contexts where the full, formal title is not necessary. 073 */ 074 @Child(name = "shortTitle", type = { 075 StringType.class }, order = 1, min = 0, max = 1, modifier = false, summary = false) 076 @Description(shortDefinition = "Title for use in informal contexts", formalDefinition = "The short title provides an alternate title for use in informal descriptive contexts where the full, formal title is not necessary.") 077 protected StringType shortTitle; 078 079 /** 080 * An explanatory or alternate title for the Evidence giving additional 081 * information about its content. 082 */ 083 @Child(name = "subtitle", type = { StringType.class }, order = 2, min = 0, max = 1, modifier = false, summary = false) 084 @Description(shortDefinition = "Subordinate title of the Evidence", formalDefinition = "An explanatory or alternate title for the Evidence giving additional information about its content.") 085 protected StringType subtitle; 086 087 /** 088 * A human-readable string to clarify or explain concepts about the resource. 089 */ 090 @Child(name = "note", type = { 091 Annotation.class }, order = 3, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 092 @Description(shortDefinition = "Used for footnotes or explanatory notes", formalDefinition = "A human-readable string to clarify or explain concepts about the resource.") 093 protected List<Annotation> note; 094 095 /** 096 * A copyright statement relating to the evidence and/or its contents. Copyright 097 * statements are generally legal restrictions on the use and publishing of the 098 * evidence. 099 */ 100 @Child(name = "copyright", type = { 101 MarkdownType.class }, order = 4, min = 0, max = 1, modifier = false, summary = false) 102 @Description(shortDefinition = "Use and/or publishing restrictions", formalDefinition = "A copyright statement relating to the evidence and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the evidence.") 103 protected MarkdownType copyright; 104 105 /** 106 * The date on which the resource content was approved by the publisher. 107 * Approval happens once when the content is officially approved for usage. 108 */ 109 @Child(name = "approvalDate", type = { 110 DateType.class }, order = 5, min = 0, max = 1, modifier = false, summary = false) 111 @Description(shortDefinition = "When the evidence was approved by publisher", formalDefinition = "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.") 112 protected DateType approvalDate; 113 114 /** 115 * The date on which the resource content was last reviewed. Review happens 116 * periodically after approval but does not change the original approval date. 117 */ 118 @Child(name = "lastReviewDate", type = { 119 DateType.class }, order = 6, min = 0, max = 1, modifier = false, summary = false) 120 @Description(shortDefinition = "When the evidence was last reviewed", formalDefinition = "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.") 121 protected DateType lastReviewDate; 122 123 /** 124 * The period during which the evidence content was or is planned to be in 125 * active use. 126 */ 127 @Child(name = "effectivePeriod", type = { 128 Period.class }, order = 7, min = 0, max = 1, modifier = false, summary = true) 129 @Description(shortDefinition = "When the evidence is expected to be used", formalDefinition = "The period during which the evidence content was or is planned to be in active use.") 130 protected Period effectivePeriod; 131 132 /** 133 * Descriptive topics related to the content of the Evidence. Topics provide a 134 * high-level categorization grouping types of Evidences that can be useful for 135 * filtering and searching. 136 */ 137 @Child(name = "topic", type = { 138 CodeableConcept.class }, order = 8, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 139 @Description(shortDefinition = "The category of the Evidence, such as Education, Treatment, Assessment, etc.", formalDefinition = "Descriptive topics related to the content of the Evidence. Topics provide a high-level categorization grouping types of Evidences that can be useful for filtering and searching.") 140 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/definition-topic") 141 protected List<CodeableConcept> topic; 142 143 /** 144 * An individiual or organization primarily involved in the creation and 145 * maintenance of the content. 146 */ 147 @Child(name = "author", type = { 148 ContactDetail.class }, order = 9, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 149 @Description(shortDefinition = "Who authored the content", formalDefinition = "An individiual or organization primarily involved in the creation and maintenance of the content.") 150 protected List<ContactDetail> author; 151 152 /** 153 * An individual or organization primarily responsible for internal coherence of 154 * the content. 155 */ 156 @Child(name = "editor", type = { 157 ContactDetail.class }, order = 10, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 158 @Description(shortDefinition = "Who edited the content", formalDefinition = "An individual or organization primarily responsible for internal coherence of the content.") 159 protected List<ContactDetail> editor; 160 161 /** 162 * An individual or organization primarily responsible for review of some aspect 163 * of the content. 164 */ 165 @Child(name = "reviewer", type = { 166 ContactDetail.class }, order = 11, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 167 @Description(shortDefinition = "Who reviewed the content", formalDefinition = "An individual or organization primarily responsible for review of some aspect of the content.") 168 protected List<ContactDetail> reviewer; 169 170 /** 171 * An individual or organization responsible for officially endorsing the 172 * content for use in some setting. 173 */ 174 @Child(name = "endorser", type = { 175 ContactDetail.class }, order = 12, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 176 @Description(shortDefinition = "Who endorsed the content", formalDefinition = "An individual or organization responsible for officially endorsing the content for use in some setting.") 177 protected List<ContactDetail> endorser; 178 179 /** 180 * Related artifacts such as additional documentation, justification, or 181 * bibliographic references. 182 */ 183 @Child(name = "relatedArtifact", type = { 184 RelatedArtifact.class }, order = 13, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = false) 185 @Description(shortDefinition = "Additional documentation, citations, etc.", formalDefinition = "Related artifacts such as additional documentation, justification, or bibliographic references.") 186 protected List<RelatedArtifact> relatedArtifact; 187 188 /** 189 * A reference to a EvidenceVariable resource that defines the population for 190 * the research. 191 */ 192 @Child(name = "exposureBackground", type = { 193 EvidenceVariable.class }, order = 14, min = 1, max = 1, modifier = false, summary = true) 194 @Description(shortDefinition = "What population?", formalDefinition = "A reference to a EvidenceVariable resource that defines the population for the research.") 195 protected Reference exposureBackground; 196 197 /** 198 * The actual object that is the target of the reference (A reference to a 199 * EvidenceVariable resource that defines the population for the research.) 200 */ 201 protected EvidenceVariable exposureBackgroundTarget; 202 203 /** 204 * A reference to a EvidenceVariable resource that defines the exposure for the 205 * research. 206 */ 207 @Child(name = "exposureVariant", type = { 208 EvidenceVariable.class }, order = 15, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 209 @Description(shortDefinition = "What exposure?", formalDefinition = "A reference to a EvidenceVariable resource that defines the exposure for the research.") 210 protected List<Reference> exposureVariant; 211 /** 212 * The actual objects that are the target of the reference (A reference to a 213 * EvidenceVariable resource that defines the exposure for the research.) 214 */ 215 protected List<EvidenceVariable> exposureVariantTarget; 216 217 /** 218 * A reference to a EvidenceVariable resomece that defines the outcome for the 219 * research. 220 */ 221 @Child(name = "outcome", type = { 222 EvidenceVariable.class }, order = 16, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 223 @Description(shortDefinition = "What outcome?", formalDefinition = "A reference to a EvidenceVariable resomece that defines the outcome for the research.") 224 protected List<Reference> outcome; 225 /** 226 * The actual objects that are the target of the reference (A reference to a 227 * EvidenceVariable resomece that defines the outcome for the research.) 228 */ 229 protected List<EvidenceVariable> outcomeTarget; 230 231 private static final long serialVersionUID = 137844509L; 232 233 /** 234 * Constructor 235 */ 236 public Evidence() { 237 super(); 238 } 239 240 /** 241 * Constructor 242 */ 243 public Evidence(Enumeration<PublicationStatus> status, Reference exposureBackground) { 244 super(); 245 this.status = status; 246 this.exposureBackground = exposureBackground; 247 } 248 249 /** 250 * @return {@link #url} (An absolute URI that is used to identify this evidence 251 * when it is referenced in a specification, model, design or an 252 * instance; also called its canonical identifier. This SHOULD be 253 * globally unique and SHOULD be a literal address at which at which an 254 * authoritative instance of this evidence is (or will be) published. 255 * This URL can be the target of a canonical reference. It SHALL remain 256 * the same when the evidence is stored on different servers.). This is 257 * the underlying object with id, value and extensions. The accessor 258 * "getUrl" gives direct access to the value 259 */ 260 public UriType getUrlElement() { 261 if (this.url == null) 262 if (Configuration.errorOnAutoCreate()) 263 throw new Error("Attempt to auto-create Evidence.url"); 264 else if (Configuration.doAutoCreate()) 265 this.url = new UriType(); // bb 266 return this.url; 267 } 268 269 public boolean hasUrlElement() { 270 return this.url != null && !this.url.isEmpty(); 271 } 272 273 public boolean hasUrl() { 274 return this.url != null && !this.url.isEmpty(); 275 } 276 277 /** 278 * @param value {@link #url} (An absolute URI that is used to identify this 279 * evidence when it is referenced in a specification, model, design 280 * or an instance; also called its canonical identifier. This 281 * SHOULD be globally unique and SHOULD be a literal address at 282 * which at which an authoritative instance of this evidence is (or 283 * will be) published. This URL can be the target of a canonical 284 * reference. It SHALL remain the same when the evidence is stored 285 * on different servers.). This is the underlying object with id, 286 * value and extensions. The accessor "getUrl" gives direct access 287 * to the value 288 */ 289 public Evidence setUrlElement(UriType value) { 290 this.url = value; 291 return this; 292 } 293 294 /** 295 * @return An absolute URI that is used to identify this evidence when it is 296 * referenced in a specification, model, design or an instance; also 297 * called its canonical identifier. This SHOULD be globally unique and 298 * SHOULD be a literal address at which at which an authoritative 299 * instance of this evidence is (or will be) published. This URL can be 300 * the target of a canonical reference. It SHALL remain the same when 301 * the evidence is stored on different servers. 302 */ 303 public String getUrl() { 304 return this.url == null ? null : this.url.getValue(); 305 } 306 307 /** 308 * @param value An absolute URI that is used to identify this evidence when it 309 * is referenced in a specification, model, design or an instance; 310 * also called its canonical identifier. This SHOULD be globally 311 * unique and SHOULD be a literal address at which at which an 312 * authoritative instance of this evidence is (or will be) 313 * published. This URL can be the target of a canonical reference. 314 * It SHALL remain the same when the evidence is stored on 315 * different servers. 316 */ 317 public Evidence setUrl(String value) { 318 if (Utilities.noString(value)) 319 this.url = null; 320 else { 321 if (this.url == null) 322 this.url = new UriType(); 323 this.url.setValue(value); 324 } 325 return this; 326 } 327 328 /** 329 * @return {@link #identifier} (A formal identifier that is used to identify 330 * this evidence when it is represented in other formats, or referenced 331 * in a specification, model, design or an instance.) 332 */ 333 public List<Identifier> getIdentifier() { 334 if (this.identifier == null) 335 this.identifier = new ArrayList<Identifier>(); 336 return this.identifier; 337 } 338 339 /** 340 * @return Returns a reference to <code>this</code> for easy method chaining 341 */ 342 public Evidence setIdentifier(List<Identifier> theIdentifier) { 343 this.identifier = theIdentifier; 344 return this; 345 } 346 347 public boolean hasIdentifier() { 348 if (this.identifier == null) 349 return false; 350 for (Identifier item : this.identifier) 351 if (!item.isEmpty()) 352 return true; 353 return false; 354 } 355 356 public Identifier addIdentifier() { // 3 357 Identifier t = new Identifier(); 358 if (this.identifier == null) 359 this.identifier = new ArrayList<Identifier>(); 360 this.identifier.add(t); 361 return t; 362 } 363 364 public Evidence addIdentifier(Identifier t) { // 3 365 if (t == null) 366 return this; 367 if (this.identifier == null) 368 this.identifier = new ArrayList<Identifier>(); 369 this.identifier.add(t); 370 return this; 371 } 372 373 /** 374 * @return The first repetition of repeating field {@link #identifier}, creating 375 * it if it does not already exist 376 */ 377 public Identifier getIdentifierFirstRep() { 378 if (getIdentifier().isEmpty()) { 379 addIdentifier(); 380 } 381 return getIdentifier().get(0); 382 } 383 384 /** 385 * @return {@link #version} (The identifier that is used to identify this 386 * version of the evidence when it is referenced in a specification, 387 * model, design or instance. This is an arbitrary value managed by the 388 * evidence author and is not expected to be globally unique. For 389 * example, it might be a timestamp (e.g. yyyymmdd) if a managed version 390 * is not available. There is also no expectation that versions can be 391 * placed in a lexicographical sequence. To provide a version consistent 392 * with the Decision Support Service specification, use the format 393 * Major.Minor.Revision (e.g. 1.0.0). For more information on versioning 394 * knowledge assets, refer to the Decision Support Service 395 * specification. Note that a version is required for non-experimental 396 * active artifacts.). This is the underlying object with id, value and 397 * extensions. The accessor "getVersion" gives direct access to the 398 * value 399 */ 400 public StringType getVersionElement() { 401 if (this.version == null) 402 if (Configuration.errorOnAutoCreate()) 403 throw new Error("Attempt to auto-create Evidence.version"); 404 else if (Configuration.doAutoCreate()) 405 this.version = new StringType(); // bb 406 return this.version; 407 } 408 409 public boolean hasVersionElement() { 410 return this.version != null && !this.version.isEmpty(); 411 } 412 413 public boolean hasVersion() { 414 return this.version != null && !this.version.isEmpty(); 415 } 416 417 /** 418 * @param value {@link #version} (The identifier that is used to identify this 419 * version of the evidence when it is referenced in a 420 * specification, model, design or instance. This is an arbitrary 421 * value managed by the evidence author and is not expected to be 422 * globally unique. For example, it might be a timestamp (e.g. 423 * yyyymmdd) if a managed version is not available. There is also 424 * no expectation that versions can be placed in a lexicographical 425 * sequence. To provide a version consistent with the Decision 426 * Support Service specification, use the format 427 * Major.Minor.Revision (e.g. 1.0.0). For more information on 428 * versioning knowledge assets, refer to the Decision Support 429 * Service specification. Note that a version is required for 430 * non-experimental active artifacts.). This is the underlying 431 * object with id, value and extensions. The accessor "getVersion" 432 * gives direct access to the value 433 */ 434 public Evidence setVersionElement(StringType value) { 435 this.version = value; 436 return this; 437 } 438 439 /** 440 * @return The identifier that is used to identify this version of the evidence 441 * when it is referenced in a specification, model, design or instance. 442 * This is an arbitrary value managed by the evidence author and is not 443 * expected to be globally unique. For example, it might be a timestamp 444 * (e.g. yyyymmdd) if a managed version is not available. There is also 445 * no expectation that versions can be placed in a lexicographical 446 * sequence. To provide a version consistent with the Decision Support 447 * Service specification, use the format Major.Minor.Revision (e.g. 448 * 1.0.0). For more information on versioning knowledge assets, refer to 449 * the Decision Support Service specification. Note that a version is 450 * required for non-experimental active artifacts. 451 */ 452 public String getVersion() { 453 return this.version == null ? null : this.version.getValue(); 454 } 455 456 /** 457 * @param value The identifier that is used to identify this version of the 458 * evidence when it is referenced in a specification, model, design 459 * or instance. This is an arbitrary value managed by the evidence 460 * author and is not expected to be globally unique. For example, 461 * it might be a timestamp (e.g. yyyymmdd) if a managed version is 462 * not available. There is also no expectation that versions can be 463 * placed in a lexicographical sequence. To provide a version 464 * consistent with the Decision Support Service specification, use 465 * the format Major.Minor.Revision (e.g. 1.0.0). For more 466 * information on versioning knowledge assets, refer to the 467 * Decision Support Service specification. Note that a version is 468 * required for non-experimental active artifacts. 469 */ 470 public Evidence setVersion(String value) { 471 if (Utilities.noString(value)) 472 this.version = null; 473 else { 474 if (this.version == null) 475 this.version = new StringType(); 476 this.version.setValue(value); 477 } 478 return this; 479 } 480 481 /** 482 * @return {@link #name} (A natural language name identifying the evidence. This 483 * name should be usable as an identifier for the module by machine 484 * processing applications such as code generation.). This is the 485 * underlying object with id, value and extensions. The accessor 486 * "getName" gives direct access to the value 487 */ 488 public StringType getNameElement() { 489 if (this.name == null) 490 if (Configuration.errorOnAutoCreate()) 491 throw new Error("Attempt to auto-create Evidence.name"); 492 else if (Configuration.doAutoCreate()) 493 this.name = new StringType(); // bb 494 return this.name; 495 } 496 497 public boolean hasNameElement() { 498 return this.name != null && !this.name.isEmpty(); 499 } 500 501 public boolean hasName() { 502 return this.name != null && !this.name.isEmpty(); 503 } 504 505 /** 506 * @param value {@link #name} (A natural language name identifying the evidence. 507 * This name should be usable as an identifier for the module by 508 * machine processing applications such as code generation.). This 509 * is the underlying object with id, value and extensions. The 510 * accessor "getName" gives direct access to the value 511 */ 512 public Evidence setNameElement(StringType value) { 513 this.name = value; 514 return this; 515 } 516 517 /** 518 * @return A natural language name identifying the evidence. This name should be 519 * usable as an identifier for the module by machine processing 520 * applications such as code generation. 521 */ 522 public String getName() { 523 return this.name == null ? null : this.name.getValue(); 524 } 525 526 /** 527 * @param value A natural language name identifying the evidence. This name 528 * should be usable as an identifier for the module by machine 529 * processing applications such as code generation. 530 */ 531 public Evidence setName(String value) { 532 if (Utilities.noString(value)) 533 this.name = null; 534 else { 535 if (this.name == null) 536 this.name = new StringType(); 537 this.name.setValue(value); 538 } 539 return this; 540 } 541 542 /** 543 * @return {@link #title} (A short, descriptive, user-friendly title for the 544 * evidence.). This is the underlying object with id, value and 545 * extensions. The accessor "getTitle" gives direct access to the value 546 */ 547 public StringType getTitleElement() { 548 if (this.title == null) 549 if (Configuration.errorOnAutoCreate()) 550 throw new Error("Attempt to auto-create Evidence.title"); 551 else if (Configuration.doAutoCreate()) 552 this.title = new StringType(); // bb 553 return this.title; 554 } 555 556 public boolean hasTitleElement() { 557 return this.title != null && !this.title.isEmpty(); 558 } 559 560 public boolean hasTitle() { 561 return this.title != null && !this.title.isEmpty(); 562 } 563 564 /** 565 * @param value {@link #title} (A short, descriptive, user-friendly title for 566 * the evidence.). This is the underlying object with id, value and 567 * extensions. The accessor "getTitle" gives direct access to the 568 * value 569 */ 570 public Evidence setTitleElement(StringType value) { 571 this.title = value; 572 return this; 573 } 574 575 /** 576 * @return A short, descriptive, user-friendly title for the evidence. 577 */ 578 public String getTitle() { 579 return this.title == null ? null : this.title.getValue(); 580 } 581 582 /** 583 * @param value A short, descriptive, user-friendly title for the evidence. 584 */ 585 public Evidence setTitle(String value) { 586 if (Utilities.noString(value)) 587 this.title = null; 588 else { 589 if (this.title == null) 590 this.title = new StringType(); 591 this.title.setValue(value); 592 } 593 return this; 594 } 595 596 /** 597 * @return {@link #shortTitle} (The short title provides an alternate title for 598 * use in informal descriptive contexts where the full, formal title is 599 * not necessary.). This is the underlying object with id, value and 600 * extensions. The accessor "getShortTitle" gives direct access to the 601 * value 602 */ 603 public StringType getShortTitleElement() { 604 if (this.shortTitle == null) 605 if (Configuration.errorOnAutoCreate()) 606 throw new Error("Attempt to auto-create Evidence.shortTitle"); 607 else if (Configuration.doAutoCreate()) 608 this.shortTitle = new StringType(); // bb 609 return this.shortTitle; 610 } 611 612 public boolean hasShortTitleElement() { 613 return this.shortTitle != null && !this.shortTitle.isEmpty(); 614 } 615 616 public boolean hasShortTitle() { 617 return this.shortTitle != null && !this.shortTitle.isEmpty(); 618 } 619 620 /** 621 * @param value {@link #shortTitle} (The short title provides an alternate title 622 * for use in informal descriptive contexts where the full, formal 623 * title is not necessary.). This is the underlying object with id, 624 * value and extensions. The accessor "getShortTitle" gives direct 625 * access to the value 626 */ 627 public Evidence setShortTitleElement(StringType value) { 628 this.shortTitle = value; 629 return this; 630 } 631 632 /** 633 * @return The short title provides an alternate title for use in informal 634 * descriptive contexts where the full, formal title is not necessary. 635 */ 636 public String getShortTitle() { 637 return this.shortTitle == null ? null : this.shortTitle.getValue(); 638 } 639 640 /** 641 * @param value The short title provides an alternate title for use in informal 642 * descriptive contexts where the full, formal title is not 643 * necessary. 644 */ 645 public Evidence setShortTitle(String value) { 646 if (Utilities.noString(value)) 647 this.shortTitle = null; 648 else { 649 if (this.shortTitle == null) 650 this.shortTitle = new StringType(); 651 this.shortTitle.setValue(value); 652 } 653 return this; 654 } 655 656 /** 657 * @return {@link #subtitle} (An explanatory or alternate title for the Evidence 658 * giving additional information about its content.). This is the 659 * underlying object with id, value and extensions. The accessor 660 * "getSubtitle" gives direct access to the value 661 */ 662 public StringType getSubtitleElement() { 663 if (this.subtitle == null) 664 if (Configuration.errorOnAutoCreate()) 665 throw new Error("Attempt to auto-create Evidence.subtitle"); 666 else if (Configuration.doAutoCreate()) 667 this.subtitle = new StringType(); // bb 668 return this.subtitle; 669 } 670 671 public boolean hasSubtitleElement() { 672 return this.subtitle != null && !this.subtitle.isEmpty(); 673 } 674 675 public boolean hasSubtitle() { 676 return this.subtitle != null && !this.subtitle.isEmpty(); 677 } 678 679 /** 680 * @param value {@link #subtitle} (An explanatory or alternate title for the 681 * Evidence giving additional information about its content.). This 682 * is the underlying object with id, value and extensions. The 683 * accessor "getSubtitle" gives direct access to the value 684 */ 685 public Evidence setSubtitleElement(StringType value) { 686 this.subtitle = value; 687 return this; 688 } 689 690 /** 691 * @return An explanatory or alternate title for the Evidence giving additional 692 * information about its content. 693 */ 694 public String getSubtitle() { 695 return this.subtitle == null ? null : this.subtitle.getValue(); 696 } 697 698 /** 699 * @param value An explanatory or alternate title for the Evidence giving 700 * additional information about its content. 701 */ 702 public Evidence setSubtitle(String value) { 703 if (Utilities.noString(value)) 704 this.subtitle = null; 705 else { 706 if (this.subtitle == null) 707 this.subtitle = new StringType(); 708 this.subtitle.setValue(value); 709 } 710 return this; 711 } 712 713 /** 714 * @return {@link #status} (The status of this evidence. Enables tracking the 715 * life-cycle of the content.). This is the underlying object with id, 716 * value and extensions. The accessor "getStatus" gives direct access to 717 * the value 718 */ 719 public Enumeration<PublicationStatus> getStatusElement() { 720 if (this.status == null) 721 if (Configuration.errorOnAutoCreate()) 722 throw new Error("Attempt to auto-create Evidence.status"); 723 else if (Configuration.doAutoCreate()) 724 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 725 return this.status; 726 } 727 728 public boolean hasStatusElement() { 729 return this.status != null && !this.status.isEmpty(); 730 } 731 732 public boolean hasStatus() { 733 return this.status != null && !this.status.isEmpty(); 734 } 735 736 /** 737 * @param value {@link #status} (The status of this evidence. Enables tracking 738 * the life-cycle of the content.). This is the underlying object 739 * with id, value and extensions. The accessor "getStatus" gives 740 * direct access to the value 741 */ 742 public Evidence setStatusElement(Enumeration<PublicationStatus> value) { 743 this.status = value; 744 return this; 745 } 746 747 /** 748 * @return The status of this evidence. Enables tracking the life-cycle of the 749 * content. 750 */ 751 public PublicationStatus getStatus() { 752 return this.status == null ? null : this.status.getValue(); 753 } 754 755 /** 756 * @param value The status of this evidence. Enables tracking the life-cycle of 757 * the content. 758 */ 759 public Evidence setStatus(PublicationStatus value) { 760 if (this.status == null) 761 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 762 this.status.setValue(value); 763 return this; 764 } 765 766 /** 767 * @return {@link #date} (The date (and optionally time) when the evidence was 768 * published. The date must change when the business version changes and 769 * it must change if the status code changes. In addition, it should 770 * change when the substantive content of the evidence changes.). This 771 * is the underlying object with id, value and extensions. The accessor 772 * "getDate" gives direct access to the value 773 */ 774 public DateTimeType getDateElement() { 775 if (this.date == null) 776 if (Configuration.errorOnAutoCreate()) 777 throw new Error("Attempt to auto-create Evidence.date"); 778 else if (Configuration.doAutoCreate()) 779 this.date = new DateTimeType(); // bb 780 return this.date; 781 } 782 783 public boolean hasDateElement() { 784 return this.date != null && !this.date.isEmpty(); 785 } 786 787 public boolean hasDate() { 788 return this.date != null && !this.date.isEmpty(); 789 } 790 791 /** 792 * @param value {@link #date} (The date (and optionally time) when the evidence 793 * was published. The date must change when the business version 794 * changes and it must change if the status code changes. In 795 * addition, it should change when the substantive content of the 796 * evidence changes.). This is the underlying object with id, value 797 * and extensions. The accessor "getDate" gives direct access to 798 * the value 799 */ 800 public Evidence setDateElement(DateTimeType value) { 801 this.date = value; 802 return this; 803 } 804 805 /** 806 * @return The date (and optionally time) when the evidence was published. The 807 * date must change when the business version changes and it must change 808 * if the status code changes. In addition, it should change when the 809 * substantive content of the evidence changes. 810 */ 811 public Date getDate() { 812 return this.date == null ? null : this.date.getValue(); 813 } 814 815 /** 816 * @param value The date (and optionally time) when the evidence was published. 817 * The date must change when the business version changes and it 818 * must change if the status code changes. In addition, it should 819 * change when the substantive content of the evidence changes. 820 */ 821 public Evidence setDate(Date value) { 822 if (value == null) 823 this.date = null; 824 else { 825 if (this.date == null) 826 this.date = new DateTimeType(); 827 this.date.setValue(value); 828 } 829 return this; 830 } 831 832 /** 833 * @return {@link #publisher} (The name of the organization or individual that 834 * published the evidence.). This is the underlying object with id, 835 * value and extensions. The accessor "getPublisher" gives direct access 836 * to the value 837 */ 838 public StringType getPublisherElement() { 839 if (this.publisher == null) 840 if (Configuration.errorOnAutoCreate()) 841 throw new Error("Attempt to auto-create Evidence.publisher"); 842 else if (Configuration.doAutoCreate()) 843 this.publisher = new StringType(); // bb 844 return this.publisher; 845 } 846 847 public boolean hasPublisherElement() { 848 return this.publisher != null && !this.publisher.isEmpty(); 849 } 850 851 public boolean hasPublisher() { 852 return this.publisher != null && !this.publisher.isEmpty(); 853 } 854 855 /** 856 * @param value {@link #publisher} (The name of the organization or individual 857 * that published the evidence.). This is the underlying object 858 * with id, value and extensions. The accessor "getPublisher" gives 859 * direct access to the value 860 */ 861 public Evidence setPublisherElement(StringType value) { 862 this.publisher = value; 863 return this; 864 } 865 866 /** 867 * @return The name of the organization or individual that published the 868 * evidence. 869 */ 870 public String getPublisher() { 871 return this.publisher == null ? null : this.publisher.getValue(); 872 } 873 874 /** 875 * @param value The name of the organization or individual that published the 876 * evidence. 877 */ 878 public Evidence setPublisher(String value) { 879 if (Utilities.noString(value)) 880 this.publisher = null; 881 else { 882 if (this.publisher == null) 883 this.publisher = new StringType(); 884 this.publisher.setValue(value); 885 } 886 return this; 887 } 888 889 /** 890 * @return {@link #contact} (Contact details to assist a user in finding and 891 * communicating with the publisher.) 892 */ 893 public List<ContactDetail> getContact() { 894 if (this.contact == null) 895 this.contact = new ArrayList<ContactDetail>(); 896 return this.contact; 897 } 898 899 /** 900 * @return Returns a reference to <code>this</code> for easy method chaining 901 */ 902 public Evidence setContact(List<ContactDetail> theContact) { 903 this.contact = theContact; 904 return this; 905 } 906 907 public boolean hasContact() { 908 if (this.contact == null) 909 return false; 910 for (ContactDetail item : this.contact) 911 if (!item.isEmpty()) 912 return true; 913 return false; 914 } 915 916 public ContactDetail addContact() { // 3 917 ContactDetail t = new ContactDetail(); 918 if (this.contact == null) 919 this.contact = new ArrayList<ContactDetail>(); 920 this.contact.add(t); 921 return t; 922 } 923 924 public Evidence addContact(ContactDetail t) { // 3 925 if (t == null) 926 return this; 927 if (this.contact == null) 928 this.contact = new ArrayList<ContactDetail>(); 929 this.contact.add(t); 930 return this; 931 } 932 933 /** 934 * @return The first repetition of repeating field {@link #contact}, creating it 935 * if it does not already exist 936 */ 937 public ContactDetail getContactFirstRep() { 938 if (getContact().isEmpty()) { 939 addContact(); 940 } 941 return getContact().get(0); 942 } 943 944 /** 945 * @return {@link #description} (A free text natural language description of the 946 * evidence from a consumer's perspective.). This is the underlying 947 * object with id, value and extensions. The accessor "getDescription" 948 * gives direct access to the value 949 */ 950 public MarkdownType getDescriptionElement() { 951 if (this.description == null) 952 if (Configuration.errorOnAutoCreate()) 953 throw new Error("Attempt to auto-create Evidence.description"); 954 else if (Configuration.doAutoCreate()) 955 this.description = new MarkdownType(); // bb 956 return this.description; 957 } 958 959 public boolean hasDescriptionElement() { 960 return this.description != null && !this.description.isEmpty(); 961 } 962 963 public boolean hasDescription() { 964 return this.description != null && !this.description.isEmpty(); 965 } 966 967 /** 968 * @param value {@link #description} (A free text natural language description 969 * of the evidence from a consumer's perspective.). This is the 970 * underlying object with id, value and extensions. The accessor 971 * "getDescription" gives direct access to the value 972 */ 973 public Evidence setDescriptionElement(MarkdownType value) { 974 this.description = value; 975 return this; 976 } 977 978 /** 979 * @return A free text natural language description of the evidence from a 980 * consumer's perspective. 981 */ 982 public String getDescription() { 983 return this.description == null ? null : this.description.getValue(); 984 } 985 986 /** 987 * @param value A free text natural language description of the evidence from a 988 * consumer's perspective. 989 */ 990 public Evidence setDescription(String value) { 991 if (value == null) 992 this.description = null; 993 else { 994 if (this.description == null) 995 this.description = new MarkdownType(); 996 this.description.setValue(value); 997 } 998 return this; 999 } 1000 1001 /** 1002 * @return {@link #note} (A human-readable string to clarify or explain concepts 1003 * about the resource.) 1004 */ 1005 public List<Annotation> getNote() { 1006 if (this.note == null) 1007 this.note = new ArrayList<Annotation>(); 1008 return this.note; 1009 } 1010 1011 /** 1012 * @return Returns a reference to <code>this</code> for easy method chaining 1013 */ 1014 public Evidence setNote(List<Annotation> theNote) { 1015 this.note = theNote; 1016 return this; 1017 } 1018 1019 public boolean hasNote() { 1020 if (this.note == null) 1021 return false; 1022 for (Annotation item : this.note) 1023 if (!item.isEmpty()) 1024 return true; 1025 return false; 1026 } 1027 1028 public Annotation addNote() { // 3 1029 Annotation t = new Annotation(); 1030 if (this.note == null) 1031 this.note = new ArrayList<Annotation>(); 1032 this.note.add(t); 1033 return t; 1034 } 1035 1036 public Evidence addNote(Annotation t) { // 3 1037 if (t == null) 1038 return this; 1039 if (this.note == null) 1040 this.note = new ArrayList<Annotation>(); 1041 this.note.add(t); 1042 return this; 1043 } 1044 1045 /** 1046 * @return The first repetition of repeating field {@link #note}, creating it if 1047 * it does not already exist 1048 */ 1049 public Annotation getNoteFirstRep() { 1050 if (getNote().isEmpty()) { 1051 addNote(); 1052 } 1053 return getNote().get(0); 1054 } 1055 1056 /** 1057 * @return {@link #useContext} (The content was developed with a focus and 1058 * intent of supporting the contexts that are listed. These contexts may 1059 * be general categories (gender, age, ...) or may be references to 1060 * specific programs (insurance plans, studies, ...) and may be used to 1061 * assist with indexing and searching for appropriate evidence 1062 * instances.) 1063 */ 1064 public List<UsageContext> getUseContext() { 1065 if (this.useContext == null) 1066 this.useContext = new ArrayList<UsageContext>(); 1067 return this.useContext; 1068 } 1069 1070 /** 1071 * @return Returns a reference to <code>this</code> for easy method chaining 1072 */ 1073 public Evidence setUseContext(List<UsageContext> theUseContext) { 1074 this.useContext = theUseContext; 1075 return this; 1076 } 1077 1078 public boolean hasUseContext() { 1079 if (this.useContext == null) 1080 return false; 1081 for (UsageContext item : this.useContext) 1082 if (!item.isEmpty()) 1083 return true; 1084 return false; 1085 } 1086 1087 public UsageContext addUseContext() { // 3 1088 UsageContext t = new UsageContext(); 1089 if (this.useContext == null) 1090 this.useContext = new ArrayList<UsageContext>(); 1091 this.useContext.add(t); 1092 return t; 1093 } 1094 1095 public Evidence addUseContext(UsageContext t) { // 3 1096 if (t == null) 1097 return this; 1098 if (this.useContext == null) 1099 this.useContext = new ArrayList<UsageContext>(); 1100 this.useContext.add(t); 1101 return this; 1102 } 1103 1104 /** 1105 * @return The first repetition of repeating field {@link #useContext}, creating 1106 * it if it does not already exist 1107 */ 1108 public UsageContext getUseContextFirstRep() { 1109 if (getUseContext().isEmpty()) { 1110 addUseContext(); 1111 } 1112 return getUseContext().get(0); 1113 } 1114 1115 /** 1116 * @return {@link #jurisdiction} (A legal or geographic region in which the 1117 * evidence is intended to be used.) 1118 */ 1119 public List<CodeableConcept> getJurisdiction() { 1120 if (this.jurisdiction == null) 1121 this.jurisdiction = new ArrayList<CodeableConcept>(); 1122 return this.jurisdiction; 1123 } 1124 1125 /** 1126 * @return Returns a reference to <code>this</code> for easy method chaining 1127 */ 1128 public Evidence setJurisdiction(List<CodeableConcept> theJurisdiction) { 1129 this.jurisdiction = theJurisdiction; 1130 return this; 1131 } 1132 1133 public boolean hasJurisdiction() { 1134 if (this.jurisdiction == null) 1135 return false; 1136 for (CodeableConcept item : this.jurisdiction) 1137 if (!item.isEmpty()) 1138 return true; 1139 return false; 1140 } 1141 1142 public CodeableConcept addJurisdiction() { // 3 1143 CodeableConcept t = new CodeableConcept(); 1144 if (this.jurisdiction == null) 1145 this.jurisdiction = new ArrayList<CodeableConcept>(); 1146 this.jurisdiction.add(t); 1147 return t; 1148 } 1149 1150 public Evidence addJurisdiction(CodeableConcept t) { // 3 1151 if (t == null) 1152 return this; 1153 if (this.jurisdiction == null) 1154 this.jurisdiction = new ArrayList<CodeableConcept>(); 1155 this.jurisdiction.add(t); 1156 return this; 1157 } 1158 1159 /** 1160 * @return The first repetition of repeating field {@link #jurisdiction}, 1161 * creating it if it does not already exist 1162 */ 1163 public CodeableConcept getJurisdictionFirstRep() { 1164 if (getJurisdiction().isEmpty()) { 1165 addJurisdiction(); 1166 } 1167 return getJurisdiction().get(0); 1168 } 1169 1170 /** 1171 * @return {@link #copyright} (A copyright statement relating to the evidence 1172 * and/or its contents. Copyright statements are generally legal 1173 * restrictions on the use and publishing of the evidence.). This is the 1174 * underlying object with id, value and extensions. The accessor 1175 * "getCopyright" gives direct access to the value 1176 */ 1177 public MarkdownType getCopyrightElement() { 1178 if (this.copyright == null) 1179 if (Configuration.errorOnAutoCreate()) 1180 throw new Error("Attempt to auto-create Evidence.copyright"); 1181 else if (Configuration.doAutoCreate()) 1182 this.copyright = new MarkdownType(); // bb 1183 return this.copyright; 1184 } 1185 1186 public boolean hasCopyrightElement() { 1187 return this.copyright != null && !this.copyright.isEmpty(); 1188 } 1189 1190 public boolean hasCopyright() { 1191 return this.copyright != null && !this.copyright.isEmpty(); 1192 } 1193 1194 /** 1195 * @param value {@link #copyright} (A copyright statement relating to the 1196 * evidence and/or its contents. Copyright statements are generally 1197 * legal restrictions on the use and publishing of the evidence.). 1198 * This is the underlying object with id, value and extensions. The 1199 * accessor "getCopyright" gives direct access to the value 1200 */ 1201 public Evidence setCopyrightElement(MarkdownType value) { 1202 this.copyright = value; 1203 return this; 1204 } 1205 1206 /** 1207 * @return A copyright statement relating to the evidence and/or its contents. 1208 * Copyright statements are generally legal restrictions on the use and 1209 * publishing of the evidence. 1210 */ 1211 public String getCopyright() { 1212 return this.copyright == null ? null : this.copyright.getValue(); 1213 } 1214 1215 /** 1216 * @param value A copyright statement relating to the evidence and/or its 1217 * contents. Copyright statements are generally legal restrictions 1218 * on the use and publishing of the evidence. 1219 */ 1220 public Evidence setCopyright(String value) { 1221 if (value == null) 1222 this.copyright = null; 1223 else { 1224 if (this.copyright == null) 1225 this.copyright = new MarkdownType(); 1226 this.copyright.setValue(value); 1227 } 1228 return this; 1229 } 1230 1231 /** 1232 * @return {@link #approvalDate} (The date on which the resource content was 1233 * approved by the publisher. Approval happens once when the content is 1234 * officially approved for usage.). This is the underlying object with 1235 * id, value and extensions. The accessor "getApprovalDate" gives direct 1236 * access to the value 1237 */ 1238 public DateType getApprovalDateElement() { 1239 if (this.approvalDate == null) 1240 if (Configuration.errorOnAutoCreate()) 1241 throw new Error("Attempt to auto-create Evidence.approvalDate"); 1242 else if (Configuration.doAutoCreate()) 1243 this.approvalDate = new DateType(); // bb 1244 return this.approvalDate; 1245 } 1246 1247 public boolean hasApprovalDateElement() { 1248 return this.approvalDate != null && !this.approvalDate.isEmpty(); 1249 } 1250 1251 public boolean hasApprovalDate() { 1252 return this.approvalDate != null && !this.approvalDate.isEmpty(); 1253 } 1254 1255 /** 1256 * @param value {@link #approvalDate} (The date on which the resource content 1257 * was approved by the publisher. Approval happens once when the 1258 * content is officially approved for usage.). This is the 1259 * underlying object with id, value and extensions. The accessor 1260 * "getApprovalDate" gives direct access to the value 1261 */ 1262 public Evidence setApprovalDateElement(DateType value) { 1263 this.approvalDate = value; 1264 return this; 1265 } 1266 1267 /** 1268 * @return The date on which the resource content was approved by the publisher. 1269 * Approval happens once when the content is officially approved for 1270 * usage. 1271 */ 1272 public Date getApprovalDate() { 1273 return this.approvalDate == null ? null : this.approvalDate.getValue(); 1274 } 1275 1276 /** 1277 * @param value The date on which the resource content was approved by the 1278 * publisher. Approval happens once when the content is officially 1279 * approved for usage. 1280 */ 1281 public Evidence setApprovalDate(Date value) { 1282 if (value == null) 1283 this.approvalDate = null; 1284 else { 1285 if (this.approvalDate == null) 1286 this.approvalDate = new DateType(); 1287 this.approvalDate.setValue(value); 1288 } 1289 return this; 1290 } 1291 1292 /** 1293 * @return {@link #lastReviewDate} (The date on which the resource content was 1294 * last reviewed. Review happens periodically after approval but does 1295 * not change the original approval date.). This is the underlying 1296 * object with id, value and extensions. The accessor 1297 * "getLastReviewDate" gives direct access to the value 1298 */ 1299 public DateType getLastReviewDateElement() { 1300 if (this.lastReviewDate == null) 1301 if (Configuration.errorOnAutoCreate()) 1302 throw new Error("Attempt to auto-create Evidence.lastReviewDate"); 1303 else if (Configuration.doAutoCreate()) 1304 this.lastReviewDate = new DateType(); // bb 1305 return this.lastReviewDate; 1306 } 1307 1308 public boolean hasLastReviewDateElement() { 1309 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 1310 } 1311 1312 public boolean hasLastReviewDate() { 1313 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 1314 } 1315 1316 /** 1317 * @param value {@link #lastReviewDate} (The date on which the resource content 1318 * was last reviewed. Review happens periodically after approval 1319 * but does not change the original approval date.). This is the 1320 * underlying object with id, value and extensions. The accessor 1321 * "getLastReviewDate" gives direct access to the value 1322 */ 1323 public Evidence setLastReviewDateElement(DateType value) { 1324 this.lastReviewDate = value; 1325 return this; 1326 } 1327 1328 /** 1329 * @return The date on which the resource content was last reviewed. Review 1330 * happens periodically after approval but does not change the original 1331 * approval date. 1332 */ 1333 public Date getLastReviewDate() { 1334 return this.lastReviewDate == null ? null : this.lastReviewDate.getValue(); 1335 } 1336 1337 /** 1338 * @param value The date on which the resource content was last reviewed. Review 1339 * happens periodically after approval but does not change the 1340 * original approval date. 1341 */ 1342 public Evidence setLastReviewDate(Date value) { 1343 if (value == null) 1344 this.lastReviewDate = null; 1345 else { 1346 if (this.lastReviewDate == null) 1347 this.lastReviewDate = new DateType(); 1348 this.lastReviewDate.setValue(value); 1349 } 1350 return this; 1351 } 1352 1353 /** 1354 * @return {@link #effectivePeriod} (The period during which the evidence 1355 * content was or is planned to be in active use.) 1356 */ 1357 public Period getEffectivePeriod() { 1358 if (this.effectivePeriod == null) 1359 if (Configuration.errorOnAutoCreate()) 1360 throw new Error("Attempt to auto-create Evidence.effectivePeriod"); 1361 else if (Configuration.doAutoCreate()) 1362 this.effectivePeriod = new Period(); // cc 1363 return this.effectivePeriod; 1364 } 1365 1366 public boolean hasEffectivePeriod() { 1367 return this.effectivePeriod != null && !this.effectivePeriod.isEmpty(); 1368 } 1369 1370 /** 1371 * @param value {@link #effectivePeriod} (The period during which the evidence 1372 * content was or is planned to be in active use.) 1373 */ 1374 public Evidence setEffectivePeriod(Period value) { 1375 this.effectivePeriod = value; 1376 return this; 1377 } 1378 1379 /** 1380 * @return {@link #topic} (Descriptive topics related to the content of the 1381 * Evidence. Topics provide a high-level categorization grouping types 1382 * of Evidences that can be useful for filtering and searching.) 1383 */ 1384 public List<CodeableConcept> getTopic() { 1385 if (this.topic == null) 1386 this.topic = new ArrayList<CodeableConcept>(); 1387 return this.topic; 1388 } 1389 1390 /** 1391 * @return Returns a reference to <code>this</code> for easy method chaining 1392 */ 1393 public Evidence setTopic(List<CodeableConcept> theTopic) { 1394 this.topic = theTopic; 1395 return this; 1396 } 1397 1398 public boolean hasTopic() { 1399 if (this.topic == null) 1400 return false; 1401 for (CodeableConcept item : this.topic) 1402 if (!item.isEmpty()) 1403 return true; 1404 return false; 1405 } 1406 1407 public CodeableConcept addTopic() { // 3 1408 CodeableConcept t = new CodeableConcept(); 1409 if (this.topic == null) 1410 this.topic = new ArrayList<CodeableConcept>(); 1411 this.topic.add(t); 1412 return t; 1413 } 1414 1415 public Evidence addTopic(CodeableConcept t) { // 3 1416 if (t == null) 1417 return this; 1418 if (this.topic == null) 1419 this.topic = new ArrayList<CodeableConcept>(); 1420 this.topic.add(t); 1421 return this; 1422 } 1423 1424 /** 1425 * @return The first repetition of repeating field {@link #topic}, creating it 1426 * if it does not already exist 1427 */ 1428 public CodeableConcept getTopicFirstRep() { 1429 if (getTopic().isEmpty()) { 1430 addTopic(); 1431 } 1432 return getTopic().get(0); 1433 } 1434 1435 /** 1436 * @return {@link #author} (An individiual or organization primarily involved in 1437 * the creation and maintenance of the content.) 1438 */ 1439 public List<ContactDetail> getAuthor() { 1440 if (this.author == null) 1441 this.author = new ArrayList<ContactDetail>(); 1442 return this.author; 1443 } 1444 1445 /** 1446 * @return Returns a reference to <code>this</code> for easy method chaining 1447 */ 1448 public Evidence setAuthor(List<ContactDetail> theAuthor) { 1449 this.author = theAuthor; 1450 return this; 1451 } 1452 1453 public boolean hasAuthor() { 1454 if (this.author == null) 1455 return false; 1456 for (ContactDetail item : this.author) 1457 if (!item.isEmpty()) 1458 return true; 1459 return false; 1460 } 1461 1462 public ContactDetail addAuthor() { // 3 1463 ContactDetail t = new ContactDetail(); 1464 if (this.author == null) 1465 this.author = new ArrayList<ContactDetail>(); 1466 this.author.add(t); 1467 return t; 1468 } 1469 1470 public Evidence addAuthor(ContactDetail t) { // 3 1471 if (t == null) 1472 return this; 1473 if (this.author == null) 1474 this.author = new ArrayList<ContactDetail>(); 1475 this.author.add(t); 1476 return this; 1477 } 1478 1479 /** 1480 * @return The first repetition of repeating field {@link #author}, creating it 1481 * if it does not already exist 1482 */ 1483 public ContactDetail getAuthorFirstRep() { 1484 if (getAuthor().isEmpty()) { 1485 addAuthor(); 1486 } 1487 return getAuthor().get(0); 1488 } 1489 1490 /** 1491 * @return {@link #editor} (An individual or organization primarily responsible 1492 * for internal coherence of the content.) 1493 */ 1494 public List<ContactDetail> getEditor() { 1495 if (this.editor == null) 1496 this.editor = new ArrayList<ContactDetail>(); 1497 return this.editor; 1498 } 1499 1500 /** 1501 * @return Returns a reference to <code>this</code> for easy method chaining 1502 */ 1503 public Evidence setEditor(List<ContactDetail> theEditor) { 1504 this.editor = theEditor; 1505 return this; 1506 } 1507 1508 public boolean hasEditor() { 1509 if (this.editor == null) 1510 return false; 1511 for (ContactDetail item : this.editor) 1512 if (!item.isEmpty()) 1513 return true; 1514 return false; 1515 } 1516 1517 public ContactDetail addEditor() { // 3 1518 ContactDetail t = new ContactDetail(); 1519 if (this.editor == null) 1520 this.editor = new ArrayList<ContactDetail>(); 1521 this.editor.add(t); 1522 return t; 1523 } 1524 1525 public Evidence addEditor(ContactDetail t) { // 3 1526 if (t == null) 1527 return this; 1528 if (this.editor == null) 1529 this.editor = new ArrayList<ContactDetail>(); 1530 this.editor.add(t); 1531 return this; 1532 } 1533 1534 /** 1535 * @return The first repetition of repeating field {@link #editor}, creating it 1536 * if it does not already exist 1537 */ 1538 public ContactDetail getEditorFirstRep() { 1539 if (getEditor().isEmpty()) { 1540 addEditor(); 1541 } 1542 return getEditor().get(0); 1543 } 1544 1545 /** 1546 * @return {@link #reviewer} (An individual or organization primarily 1547 * responsible for review of some aspect of the content.) 1548 */ 1549 public List<ContactDetail> getReviewer() { 1550 if (this.reviewer == null) 1551 this.reviewer = new ArrayList<ContactDetail>(); 1552 return this.reviewer; 1553 } 1554 1555 /** 1556 * @return Returns a reference to <code>this</code> for easy method chaining 1557 */ 1558 public Evidence setReviewer(List<ContactDetail> theReviewer) { 1559 this.reviewer = theReviewer; 1560 return this; 1561 } 1562 1563 public boolean hasReviewer() { 1564 if (this.reviewer == null) 1565 return false; 1566 for (ContactDetail item : this.reviewer) 1567 if (!item.isEmpty()) 1568 return true; 1569 return false; 1570 } 1571 1572 public ContactDetail addReviewer() { // 3 1573 ContactDetail t = new ContactDetail(); 1574 if (this.reviewer == null) 1575 this.reviewer = new ArrayList<ContactDetail>(); 1576 this.reviewer.add(t); 1577 return t; 1578 } 1579 1580 public Evidence addReviewer(ContactDetail t) { // 3 1581 if (t == null) 1582 return this; 1583 if (this.reviewer == null) 1584 this.reviewer = new ArrayList<ContactDetail>(); 1585 this.reviewer.add(t); 1586 return this; 1587 } 1588 1589 /** 1590 * @return The first repetition of repeating field {@link #reviewer}, creating 1591 * it if it does not already exist 1592 */ 1593 public ContactDetail getReviewerFirstRep() { 1594 if (getReviewer().isEmpty()) { 1595 addReviewer(); 1596 } 1597 return getReviewer().get(0); 1598 } 1599 1600 /** 1601 * @return {@link #endorser} (An individual or organization responsible for 1602 * officially endorsing the content for use in some setting.) 1603 */ 1604 public List<ContactDetail> getEndorser() { 1605 if (this.endorser == null) 1606 this.endorser = new ArrayList<ContactDetail>(); 1607 return this.endorser; 1608 } 1609 1610 /** 1611 * @return Returns a reference to <code>this</code> for easy method chaining 1612 */ 1613 public Evidence setEndorser(List<ContactDetail> theEndorser) { 1614 this.endorser = theEndorser; 1615 return this; 1616 } 1617 1618 public boolean hasEndorser() { 1619 if (this.endorser == null) 1620 return false; 1621 for (ContactDetail item : this.endorser) 1622 if (!item.isEmpty()) 1623 return true; 1624 return false; 1625 } 1626 1627 public ContactDetail addEndorser() { // 3 1628 ContactDetail t = new ContactDetail(); 1629 if (this.endorser == null) 1630 this.endorser = new ArrayList<ContactDetail>(); 1631 this.endorser.add(t); 1632 return t; 1633 } 1634 1635 public Evidence addEndorser(ContactDetail t) { // 3 1636 if (t == null) 1637 return this; 1638 if (this.endorser == null) 1639 this.endorser = new ArrayList<ContactDetail>(); 1640 this.endorser.add(t); 1641 return this; 1642 } 1643 1644 /** 1645 * @return The first repetition of repeating field {@link #endorser}, creating 1646 * it if it does not already exist 1647 */ 1648 public ContactDetail getEndorserFirstRep() { 1649 if (getEndorser().isEmpty()) { 1650 addEndorser(); 1651 } 1652 return getEndorser().get(0); 1653 } 1654 1655 /** 1656 * @return {@link #relatedArtifact} (Related artifacts such as additional 1657 * documentation, justification, or bibliographic references.) 1658 */ 1659 public List<RelatedArtifact> getRelatedArtifact() { 1660 if (this.relatedArtifact == null) 1661 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 1662 return this.relatedArtifact; 1663 } 1664 1665 /** 1666 * @return Returns a reference to <code>this</code> for easy method chaining 1667 */ 1668 public Evidence setRelatedArtifact(List<RelatedArtifact> theRelatedArtifact) { 1669 this.relatedArtifact = theRelatedArtifact; 1670 return this; 1671 } 1672 1673 public boolean hasRelatedArtifact() { 1674 if (this.relatedArtifact == null) 1675 return false; 1676 for (RelatedArtifact item : this.relatedArtifact) 1677 if (!item.isEmpty()) 1678 return true; 1679 return false; 1680 } 1681 1682 public RelatedArtifact addRelatedArtifact() { // 3 1683 RelatedArtifact t = new RelatedArtifact(); 1684 if (this.relatedArtifact == null) 1685 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 1686 this.relatedArtifact.add(t); 1687 return t; 1688 } 1689 1690 public Evidence addRelatedArtifact(RelatedArtifact t) { // 3 1691 if (t == null) 1692 return this; 1693 if (this.relatedArtifact == null) 1694 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 1695 this.relatedArtifact.add(t); 1696 return this; 1697 } 1698 1699 /** 1700 * @return The first repetition of repeating field {@link #relatedArtifact}, 1701 * creating it if it does not already exist 1702 */ 1703 public RelatedArtifact getRelatedArtifactFirstRep() { 1704 if (getRelatedArtifact().isEmpty()) { 1705 addRelatedArtifact(); 1706 } 1707 return getRelatedArtifact().get(0); 1708 } 1709 1710 /** 1711 * @return {@link #exposureBackground} (A reference to a EvidenceVariable 1712 * resource that defines the population for the research.) 1713 */ 1714 public Reference getExposureBackground() { 1715 if (this.exposureBackground == null) 1716 if (Configuration.errorOnAutoCreate()) 1717 throw new Error("Attempt to auto-create Evidence.exposureBackground"); 1718 else if (Configuration.doAutoCreate()) 1719 this.exposureBackground = new Reference(); // cc 1720 return this.exposureBackground; 1721 } 1722 1723 public boolean hasExposureBackground() { 1724 return this.exposureBackground != null && !this.exposureBackground.isEmpty(); 1725 } 1726 1727 /** 1728 * @param value {@link #exposureBackground} (A reference to a EvidenceVariable 1729 * resource that defines the population for the research.) 1730 */ 1731 public Evidence setExposureBackground(Reference value) { 1732 this.exposureBackground = value; 1733 return this; 1734 } 1735 1736 /** 1737 * @return {@link #exposureBackground} The actual object that is the target of 1738 * the reference. The reference library doesn't populate this, but you 1739 * can use it to hold the resource if you resolve it. (A reference to a 1740 * EvidenceVariable resource that defines the population for the 1741 * research.) 1742 */ 1743 public EvidenceVariable getExposureBackgroundTarget() { 1744 if (this.exposureBackgroundTarget == null) 1745 if (Configuration.errorOnAutoCreate()) 1746 throw new Error("Attempt to auto-create Evidence.exposureBackground"); 1747 else if (Configuration.doAutoCreate()) 1748 this.exposureBackgroundTarget = new EvidenceVariable(); // aa 1749 return this.exposureBackgroundTarget; 1750 } 1751 1752 /** 1753 * @param value {@link #exposureBackground} The actual object that is the target 1754 * of the reference. The reference library doesn't use these, but 1755 * you can use it to hold the resource if you resolve it. (A 1756 * reference to a EvidenceVariable resource that defines the 1757 * population for the research.) 1758 */ 1759 public Evidence setExposureBackgroundTarget(EvidenceVariable value) { 1760 this.exposureBackgroundTarget = value; 1761 return this; 1762 } 1763 1764 /** 1765 * @return {@link #exposureVariant} (A reference to a EvidenceVariable resource 1766 * that defines the exposure for the research.) 1767 */ 1768 public List<Reference> getExposureVariant() { 1769 if (this.exposureVariant == null) 1770 this.exposureVariant = new ArrayList<Reference>(); 1771 return this.exposureVariant; 1772 } 1773 1774 /** 1775 * @return Returns a reference to <code>this</code> for easy method chaining 1776 */ 1777 public Evidence setExposureVariant(List<Reference> theExposureVariant) { 1778 this.exposureVariant = theExposureVariant; 1779 return this; 1780 } 1781 1782 public boolean hasExposureVariant() { 1783 if (this.exposureVariant == null) 1784 return false; 1785 for (Reference item : this.exposureVariant) 1786 if (!item.isEmpty()) 1787 return true; 1788 return false; 1789 } 1790 1791 public Reference addExposureVariant() { // 3 1792 Reference t = new Reference(); 1793 if (this.exposureVariant == null) 1794 this.exposureVariant = new ArrayList<Reference>(); 1795 this.exposureVariant.add(t); 1796 return t; 1797 } 1798 1799 public Evidence addExposureVariant(Reference t) { // 3 1800 if (t == null) 1801 return this; 1802 if (this.exposureVariant == null) 1803 this.exposureVariant = new ArrayList<Reference>(); 1804 this.exposureVariant.add(t); 1805 return this; 1806 } 1807 1808 /** 1809 * @return The first repetition of repeating field {@link #exposureVariant}, 1810 * creating it if it does not already exist 1811 */ 1812 public Reference getExposureVariantFirstRep() { 1813 if (getExposureVariant().isEmpty()) { 1814 addExposureVariant(); 1815 } 1816 return getExposureVariant().get(0); 1817 } 1818 1819 /** 1820 * @deprecated Use Reference#setResource(IBaseResource) instead 1821 */ 1822 @Deprecated 1823 public List<EvidenceVariable> getExposureVariantTarget() { 1824 if (this.exposureVariantTarget == null) 1825 this.exposureVariantTarget = new ArrayList<EvidenceVariable>(); 1826 return this.exposureVariantTarget; 1827 } 1828 1829 /** 1830 * @deprecated Use Reference#setResource(IBaseResource) instead 1831 */ 1832 @Deprecated 1833 public EvidenceVariable addExposureVariantTarget() { 1834 EvidenceVariable r = new EvidenceVariable(); 1835 if (this.exposureVariantTarget == null) 1836 this.exposureVariantTarget = new ArrayList<EvidenceVariable>(); 1837 this.exposureVariantTarget.add(r); 1838 return r; 1839 } 1840 1841 /** 1842 * @return {@link #outcome} (A reference to a EvidenceVariable resomece that 1843 * defines the outcome for the research.) 1844 */ 1845 public List<Reference> getOutcome() { 1846 if (this.outcome == null) 1847 this.outcome = new ArrayList<Reference>(); 1848 return this.outcome; 1849 } 1850 1851 /** 1852 * @return Returns a reference to <code>this</code> for easy method chaining 1853 */ 1854 public Evidence setOutcome(List<Reference> theOutcome) { 1855 this.outcome = theOutcome; 1856 return this; 1857 } 1858 1859 public boolean hasOutcome() { 1860 if (this.outcome == null) 1861 return false; 1862 for (Reference item : this.outcome) 1863 if (!item.isEmpty()) 1864 return true; 1865 return false; 1866 } 1867 1868 public Reference addOutcome() { // 3 1869 Reference t = new Reference(); 1870 if (this.outcome == null) 1871 this.outcome = new ArrayList<Reference>(); 1872 this.outcome.add(t); 1873 return t; 1874 } 1875 1876 public Evidence addOutcome(Reference t) { // 3 1877 if (t == null) 1878 return this; 1879 if (this.outcome == null) 1880 this.outcome = new ArrayList<Reference>(); 1881 this.outcome.add(t); 1882 return this; 1883 } 1884 1885 /** 1886 * @return The first repetition of repeating field {@link #outcome}, creating it 1887 * if it does not already exist 1888 */ 1889 public Reference getOutcomeFirstRep() { 1890 if (getOutcome().isEmpty()) { 1891 addOutcome(); 1892 } 1893 return getOutcome().get(0); 1894 } 1895 1896 /** 1897 * @deprecated Use Reference#setResource(IBaseResource) instead 1898 */ 1899 @Deprecated 1900 public List<EvidenceVariable> getOutcomeTarget() { 1901 if (this.outcomeTarget == null) 1902 this.outcomeTarget = new ArrayList<EvidenceVariable>(); 1903 return this.outcomeTarget; 1904 } 1905 1906 /** 1907 * @deprecated Use Reference#setResource(IBaseResource) instead 1908 */ 1909 @Deprecated 1910 public EvidenceVariable addOutcomeTarget() { 1911 EvidenceVariable r = new EvidenceVariable(); 1912 if (this.outcomeTarget == null) 1913 this.outcomeTarget = new ArrayList<EvidenceVariable>(); 1914 this.outcomeTarget.add(r); 1915 return r; 1916 } 1917 1918 protected void listChildren(List<Property> children) { 1919 super.listChildren(children); 1920 children.add(new Property("url", "uri", 1921 "An absolute URI that is used to identify this evidence when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this evidence is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the evidence is stored on different servers.", 1922 0, 1, url)); 1923 children.add(new Property("identifier", "Identifier", 1924 "A formal identifier that is used to identify this evidence when it is represented in other formats, or referenced in a specification, model, design or an instance.", 1925 0, java.lang.Integer.MAX_VALUE, identifier)); 1926 children.add(new Property("version", "string", 1927 "The identifier that is used to identify this version of the evidence when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the evidence author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts.", 1928 0, 1, version)); 1929 children.add(new Property("name", "string", 1930 "A natural language name identifying the evidence. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 1931 0, 1, name)); 1932 children.add( 1933 new Property("title", "string", "A short, descriptive, user-friendly title for the evidence.", 0, 1, title)); 1934 children.add(new Property("shortTitle", "string", 1935 "The short title provides an alternate title for use in informal descriptive contexts where the full, formal title is not necessary.", 1936 0, 1, shortTitle)); 1937 children.add(new Property("subtitle", "string", 1938 "An explanatory or alternate title for the Evidence giving additional information about its content.", 0, 1, 1939 subtitle)); 1940 children.add(new Property("status", "code", 1941 "The status of this evidence. Enables tracking the life-cycle of the content.", 0, 1, status)); 1942 children.add(new Property("date", "dateTime", 1943 "The date (and optionally time) when the evidence was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the evidence changes.", 1944 0, 1, date)); 1945 children.add(new Property("publisher", "string", 1946 "The name of the organization or individual that published the evidence.", 0, 1, publisher)); 1947 children.add(new Property("contact", "ContactDetail", 1948 "Contact details to assist a user in finding and communicating with the publisher.", 0, 1949 java.lang.Integer.MAX_VALUE, contact)); 1950 children.add(new Property("description", "markdown", 1951 "A free text natural language description of the evidence from a consumer's perspective.", 0, 1, description)); 1952 children.add( 1953 new Property("note", "Annotation", "A human-readable string to clarify or explain concepts about the resource.", 1954 0, java.lang.Integer.MAX_VALUE, note)); 1955 children.add(new Property("useContext", "UsageContext", 1956 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate evidence instances.", 1957 0, java.lang.Integer.MAX_VALUE, useContext)); 1958 children.add(new Property("jurisdiction", "CodeableConcept", 1959 "A legal or geographic region in which the evidence is intended to be used.", 0, java.lang.Integer.MAX_VALUE, 1960 jurisdiction)); 1961 children.add(new Property("copyright", "markdown", 1962 "A copyright statement relating to the evidence and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the evidence.", 1963 0, 1, copyright)); 1964 children.add(new Property("approvalDate", "date", 1965 "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 1966 0, 1, approvalDate)); 1967 children.add(new Property("lastReviewDate", "date", 1968 "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 1969 0, 1, lastReviewDate)); 1970 children.add(new Property("effectivePeriod", "Period", 1971 "The period during which the evidence content was or is planned to be in active use.", 0, 1, effectivePeriod)); 1972 children.add(new Property("topic", "CodeableConcept", 1973 "Descriptive topics related to the content of the Evidence. Topics provide a high-level categorization grouping types of Evidences that can be useful for filtering and searching.", 1974 0, java.lang.Integer.MAX_VALUE, topic)); 1975 children.add(new Property("author", "ContactDetail", 1976 "An individiual or organization primarily involved in the creation and maintenance of the content.", 0, 1977 java.lang.Integer.MAX_VALUE, author)); 1978 children.add(new Property("editor", "ContactDetail", 1979 "An individual or organization primarily responsible for internal coherence of the content.", 0, 1980 java.lang.Integer.MAX_VALUE, editor)); 1981 children.add(new Property("reviewer", "ContactDetail", 1982 "An individual or organization primarily responsible for review of some aspect of the content.", 0, 1983 java.lang.Integer.MAX_VALUE, reviewer)); 1984 children.add(new Property("endorser", "ContactDetail", 1985 "An individual or organization responsible for officially endorsing the content for use in some setting.", 0, 1986 java.lang.Integer.MAX_VALUE, endorser)); 1987 children.add(new Property("relatedArtifact", "RelatedArtifact", 1988 "Related artifacts such as additional documentation, justification, or bibliographic references.", 0, 1989 java.lang.Integer.MAX_VALUE, relatedArtifact)); 1990 children.add(new Property("exposureBackground", "Reference(EvidenceVariable)", 1991 "A reference to a EvidenceVariable resource that defines the population for the research.", 0, 1, 1992 exposureBackground)); 1993 children.add(new Property("exposureVariant", "Reference(EvidenceVariable)", 1994 "A reference to a EvidenceVariable resource that defines the exposure for the research.", 0, 1995 java.lang.Integer.MAX_VALUE, exposureVariant)); 1996 children.add(new Property("outcome", "Reference(EvidenceVariable)", 1997 "A reference to a EvidenceVariable resomece that defines the outcome for the research.", 0, 1998 java.lang.Integer.MAX_VALUE, outcome)); 1999 } 2000 2001 @Override 2002 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2003 switch (_hash) { 2004 case 116079: 2005 /* url */ return new Property("url", "uri", 2006 "An absolute URI that is used to identify this evidence when it is referenced in a specification, model, design or an instance; also called its canonical identifier. This SHOULD be globally unique and SHOULD be a literal address at which at which an authoritative instance of this evidence is (or will be) published. This URL can be the target of a canonical reference. It SHALL remain the same when the evidence is stored on different servers.", 2007 0, 1, url); 2008 case -1618432855: 2009 /* identifier */ return new Property("identifier", "Identifier", 2010 "A formal identifier that is used to identify this evidence when it is represented in other formats, or referenced in a specification, model, design or an instance.", 2011 0, java.lang.Integer.MAX_VALUE, identifier); 2012 case 351608024: 2013 /* version */ return new Property("version", "string", 2014 "The identifier that is used to identify this version of the evidence when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the evidence author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts.", 2015 0, 1, version); 2016 case 3373707: 2017 /* name */ return new Property("name", "string", 2018 "A natural language name identifying the evidence. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 2019 0, 1, name); 2020 case 110371416: 2021 /* title */ return new Property("title", "string", "A short, descriptive, user-friendly title for the evidence.", 2022 0, 1, title); 2023 case 1555503932: 2024 /* shortTitle */ return new Property("shortTitle", "string", 2025 "The short title provides an alternate title for use in informal descriptive contexts where the full, formal title is not necessary.", 2026 0, 1, shortTitle); 2027 case -2060497896: 2028 /* subtitle */ return new Property("subtitle", "string", 2029 "An explanatory or alternate title for the Evidence giving additional information about its content.", 0, 1, 2030 subtitle); 2031 case -892481550: 2032 /* status */ return new Property("status", "code", 2033 "The status of this evidence. Enables tracking the life-cycle of the content.", 0, 1, status); 2034 case 3076014: 2035 /* date */ return new Property("date", "dateTime", 2036 "The date (and optionally time) when the evidence was published. The date must change when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the evidence changes.", 2037 0, 1, date); 2038 case 1447404028: 2039 /* publisher */ return new Property("publisher", "string", 2040 "The name of the organization or individual that published the evidence.", 0, 1, publisher); 2041 case 951526432: 2042 /* contact */ return new Property("contact", "ContactDetail", 2043 "Contact details to assist a user in finding and communicating with the publisher.", 0, 2044 java.lang.Integer.MAX_VALUE, contact); 2045 case -1724546052: 2046 /* description */ return new Property("description", "markdown", 2047 "A free text natural language description of the evidence from a consumer's perspective.", 0, 1, description); 2048 case 3387378: 2049 /* note */ return new Property("note", "Annotation", 2050 "A human-readable string to clarify or explain concepts about the resource.", 0, java.lang.Integer.MAX_VALUE, 2051 note); 2052 case -669707736: 2053 /* useContext */ return new Property("useContext", "UsageContext", 2054 "The content was developed with a focus and intent of supporting the contexts that are listed. These contexts may be general categories (gender, age, ...) or may be references to specific programs (insurance plans, studies, ...) and may be used to assist with indexing and searching for appropriate evidence instances.", 2055 0, java.lang.Integer.MAX_VALUE, useContext); 2056 case -507075711: 2057 /* jurisdiction */ return new Property("jurisdiction", "CodeableConcept", 2058 "A legal or geographic region in which the evidence is intended to be used.", 0, java.lang.Integer.MAX_VALUE, 2059 jurisdiction); 2060 case 1522889671: 2061 /* copyright */ return new Property("copyright", "markdown", 2062 "A copyright statement relating to the evidence and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the evidence.", 2063 0, 1, copyright); 2064 case 223539345: 2065 /* approvalDate */ return new Property("approvalDate", "date", 2066 "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 2067 0, 1, approvalDate); 2068 case -1687512484: 2069 /* lastReviewDate */ return new Property("lastReviewDate", "date", 2070 "The date on which the resource content was last reviewed. Review happens periodically after approval but does not change the original approval date.", 2071 0, 1, lastReviewDate); 2072 case -403934648: 2073 /* effectivePeriod */ return new Property("effectivePeriod", "Period", 2074 "The period during which the evidence content was or is planned to be in active use.", 0, 1, effectivePeriod); 2075 case 110546223: 2076 /* topic */ return new Property("topic", "CodeableConcept", 2077 "Descriptive topics related to the content of the Evidence. Topics provide a high-level categorization grouping types of Evidences that can be useful for filtering and searching.", 2078 0, java.lang.Integer.MAX_VALUE, topic); 2079 case -1406328437: 2080 /* author */ return new Property("author", "ContactDetail", 2081 "An individiual or organization primarily involved in the creation and maintenance of the content.", 0, 2082 java.lang.Integer.MAX_VALUE, author); 2083 case -1307827859: 2084 /* editor */ return new Property("editor", "ContactDetail", 2085 "An individual or organization primarily responsible for internal coherence of the content.", 0, 2086 java.lang.Integer.MAX_VALUE, editor); 2087 case -261190139: 2088 /* reviewer */ return new Property("reviewer", "ContactDetail", 2089 "An individual or organization primarily responsible for review of some aspect of the content.", 0, 2090 java.lang.Integer.MAX_VALUE, reviewer); 2091 case 1740277666: 2092 /* endorser */ return new Property("endorser", "ContactDetail", 2093 "An individual or organization responsible for officially endorsing the content for use in some setting.", 0, 2094 java.lang.Integer.MAX_VALUE, endorser); 2095 case 666807069: 2096 /* relatedArtifact */ return new Property("relatedArtifact", "RelatedArtifact", 2097 "Related artifacts such as additional documentation, justification, or bibliographic references.", 0, 2098 java.lang.Integer.MAX_VALUE, relatedArtifact); 2099 case -832155243: 2100 /* exposureBackground */ return new Property("exposureBackground", "Reference(EvidenceVariable)", 2101 "A reference to a EvidenceVariable resource that defines the population for the research.", 0, 1, 2102 exposureBackground); 2103 case -19675778: 2104 /* exposureVariant */ return new Property("exposureVariant", "Reference(EvidenceVariable)", 2105 "A reference to a EvidenceVariable resource that defines the exposure for the research.", 0, 2106 java.lang.Integer.MAX_VALUE, exposureVariant); 2107 case -1106507950: 2108 /* outcome */ return new Property("outcome", "Reference(EvidenceVariable)", 2109 "A reference to a EvidenceVariable resomece that defines the outcome for the research.", 0, 2110 java.lang.Integer.MAX_VALUE, outcome); 2111 default: 2112 return super.getNamedProperty(_hash, _name, _checkValid); 2113 } 2114 2115 } 2116 2117 @Override 2118 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2119 switch (hash) { 2120 case 116079: 2121 /* url */ return this.url == null ? new Base[0] : new Base[] { this.url }; // UriType 2122 case -1618432855: 2123 /* identifier */ return this.identifier == null ? new Base[0] 2124 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2125 case 351608024: 2126 /* version */ return this.version == null ? new Base[0] : new Base[] { this.version }; // StringType 2127 case 3373707: 2128 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 2129 case 110371416: 2130 /* title */ return this.title == null ? new Base[0] : new Base[] { this.title }; // StringType 2131 case 1555503932: 2132 /* shortTitle */ return this.shortTitle == null ? new Base[0] : new Base[] { this.shortTitle }; // StringType 2133 case -2060497896: 2134 /* subtitle */ return this.subtitle == null ? new Base[0] : new Base[] { this.subtitle }; // StringType 2135 case -892481550: 2136 /* status */ return this.status == null ? new Base[0] : new Base[] { this.status }; // Enumeration<PublicationStatus> 2137 case 3076014: 2138 /* date */ return this.date == null ? new Base[0] : new Base[] { this.date }; // DateTimeType 2139 case 1447404028: 2140 /* publisher */ return this.publisher == null ? new Base[0] : new Base[] { this.publisher }; // StringType 2141 case 951526432: 2142 /* contact */ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 2143 case -1724546052: 2144 /* description */ return this.description == null ? new Base[0] : new Base[] { this.description }; // MarkdownType 2145 case 3387378: 2146 /* note */ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 2147 case -669707736: 2148 /* useContext */ return this.useContext == null ? new Base[0] 2149 : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 2150 case -507075711: 2151 /* jurisdiction */ return this.jurisdiction == null ? new Base[0] 2152 : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 2153 case 1522889671: 2154 /* copyright */ return this.copyright == null ? new Base[0] : new Base[] { this.copyright }; // MarkdownType 2155 case 223539345: 2156 /* approvalDate */ return this.approvalDate == null ? new Base[0] : new Base[] { this.approvalDate }; // DateType 2157 case -1687512484: 2158 /* lastReviewDate */ return this.lastReviewDate == null ? new Base[0] : new Base[] { this.lastReviewDate }; // DateType 2159 case -403934648: 2160 /* effectivePeriod */ return this.effectivePeriod == null ? new Base[0] : new Base[] { this.effectivePeriod }; // Period 2161 case 110546223: 2162 /* topic */ return this.topic == null ? new Base[0] : this.topic.toArray(new Base[this.topic.size()]); // CodeableConcept 2163 case -1406328437: 2164 /* author */ return this.author == null ? new Base[0] : this.author.toArray(new Base[this.author.size()]); // ContactDetail 2165 case -1307827859: 2166 /* editor */ return this.editor == null ? new Base[0] : this.editor.toArray(new Base[this.editor.size()]); // ContactDetail 2167 case -261190139: 2168 /* reviewer */ return this.reviewer == null ? new Base[0] : this.reviewer.toArray(new Base[this.reviewer.size()]); // ContactDetail 2169 case 1740277666: 2170 /* endorser */ return this.endorser == null ? new Base[0] : this.endorser.toArray(new Base[this.endorser.size()]); // ContactDetail 2171 case 666807069: 2172 /* relatedArtifact */ return this.relatedArtifact == null ? new Base[0] 2173 : this.relatedArtifact.toArray(new Base[this.relatedArtifact.size()]); // RelatedArtifact 2174 case -832155243: 2175 /* exposureBackground */ return this.exposureBackground == null ? new Base[0] 2176 : new Base[] { this.exposureBackground }; // Reference 2177 case -19675778: 2178 /* exposureVariant */ return this.exposureVariant == null ? new Base[0] 2179 : this.exposureVariant.toArray(new Base[this.exposureVariant.size()]); // Reference 2180 case -1106507950: 2181 /* outcome */ return this.outcome == null ? new Base[0] : this.outcome.toArray(new Base[this.outcome.size()]); // Reference 2182 default: 2183 return super.getProperty(hash, name, checkValid); 2184 } 2185 2186 } 2187 2188 @Override 2189 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2190 switch (hash) { 2191 case 116079: // url 2192 this.url = castToUri(value); // UriType 2193 return value; 2194 case -1618432855: // identifier 2195 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2196 return value; 2197 case 351608024: // version 2198 this.version = castToString(value); // StringType 2199 return value; 2200 case 3373707: // name 2201 this.name = castToString(value); // StringType 2202 return value; 2203 case 110371416: // title 2204 this.title = castToString(value); // StringType 2205 return value; 2206 case 1555503932: // shortTitle 2207 this.shortTitle = castToString(value); // StringType 2208 return value; 2209 case -2060497896: // subtitle 2210 this.subtitle = castToString(value); // StringType 2211 return value; 2212 case -892481550: // status 2213 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 2214 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 2215 return value; 2216 case 3076014: // date 2217 this.date = castToDateTime(value); // DateTimeType 2218 return value; 2219 case 1447404028: // publisher 2220 this.publisher = castToString(value); // StringType 2221 return value; 2222 case 951526432: // contact 2223 this.getContact().add(castToContactDetail(value)); // ContactDetail 2224 return value; 2225 case -1724546052: // description 2226 this.description = castToMarkdown(value); // MarkdownType 2227 return value; 2228 case 3387378: // note 2229 this.getNote().add(castToAnnotation(value)); // Annotation 2230 return value; 2231 case -669707736: // useContext 2232 this.getUseContext().add(castToUsageContext(value)); // UsageContext 2233 return value; 2234 case -507075711: // jurisdiction 2235 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 2236 return value; 2237 case 1522889671: // copyright 2238 this.copyright = castToMarkdown(value); // MarkdownType 2239 return value; 2240 case 223539345: // approvalDate 2241 this.approvalDate = castToDate(value); // DateType 2242 return value; 2243 case -1687512484: // lastReviewDate 2244 this.lastReviewDate = castToDate(value); // DateType 2245 return value; 2246 case -403934648: // effectivePeriod 2247 this.effectivePeriod = castToPeriod(value); // Period 2248 return value; 2249 case 110546223: // topic 2250 this.getTopic().add(castToCodeableConcept(value)); // CodeableConcept 2251 return value; 2252 case -1406328437: // author 2253 this.getAuthor().add(castToContactDetail(value)); // ContactDetail 2254 return value; 2255 case -1307827859: // editor 2256 this.getEditor().add(castToContactDetail(value)); // ContactDetail 2257 return value; 2258 case -261190139: // reviewer 2259 this.getReviewer().add(castToContactDetail(value)); // ContactDetail 2260 return value; 2261 case 1740277666: // endorser 2262 this.getEndorser().add(castToContactDetail(value)); // ContactDetail 2263 return value; 2264 case 666807069: // relatedArtifact 2265 this.getRelatedArtifact().add(castToRelatedArtifact(value)); // RelatedArtifact 2266 return value; 2267 case -832155243: // exposureBackground 2268 this.exposureBackground = castToReference(value); // Reference 2269 return value; 2270 case -19675778: // exposureVariant 2271 this.getExposureVariant().add(castToReference(value)); // Reference 2272 return value; 2273 case -1106507950: // outcome 2274 this.getOutcome().add(castToReference(value)); // Reference 2275 return value; 2276 default: 2277 return super.setProperty(hash, name, value); 2278 } 2279 2280 } 2281 2282 @Override 2283 public Base setProperty(String name, Base value) throws FHIRException { 2284 if (name.equals("url")) { 2285 this.url = castToUri(value); // UriType 2286 } else if (name.equals("identifier")) { 2287 this.getIdentifier().add(castToIdentifier(value)); 2288 } else if (name.equals("version")) { 2289 this.version = castToString(value); // StringType 2290 } else if (name.equals("name")) { 2291 this.name = castToString(value); // StringType 2292 } else if (name.equals("title")) { 2293 this.title = castToString(value); // StringType 2294 } else if (name.equals("shortTitle")) { 2295 this.shortTitle = castToString(value); // StringType 2296 } else if (name.equals("subtitle")) { 2297 this.subtitle = castToString(value); // StringType 2298 } else if (name.equals("status")) { 2299 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 2300 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 2301 } else if (name.equals("date")) { 2302 this.date = castToDateTime(value); // DateTimeType 2303 } else if (name.equals("publisher")) { 2304 this.publisher = castToString(value); // StringType 2305 } else if (name.equals("contact")) { 2306 this.getContact().add(castToContactDetail(value)); 2307 } else if (name.equals("description")) { 2308 this.description = castToMarkdown(value); // MarkdownType 2309 } else if (name.equals("note")) { 2310 this.getNote().add(castToAnnotation(value)); 2311 } else if (name.equals("useContext")) { 2312 this.getUseContext().add(castToUsageContext(value)); 2313 } else if (name.equals("jurisdiction")) { 2314 this.getJurisdiction().add(castToCodeableConcept(value)); 2315 } else if (name.equals("copyright")) { 2316 this.copyright = castToMarkdown(value); // MarkdownType 2317 } else if (name.equals("approvalDate")) { 2318 this.approvalDate = castToDate(value); // DateType 2319 } else if (name.equals("lastReviewDate")) { 2320 this.lastReviewDate = castToDate(value); // DateType 2321 } else if (name.equals("effectivePeriod")) { 2322 this.effectivePeriod = castToPeriod(value); // Period 2323 } else if (name.equals("topic")) { 2324 this.getTopic().add(castToCodeableConcept(value)); 2325 } else if (name.equals("author")) { 2326 this.getAuthor().add(castToContactDetail(value)); 2327 } else if (name.equals("editor")) { 2328 this.getEditor().add(castToContactDetail(value)); 2329 } else if (name.equals("reviewer")) { 2330 this.getReviewer().add(castToContactDetail(value)); 2331 } else if (name.equals("endorser")) { 2332 this.getEndorser().add(castToContactDetail(value)); 2333 } else if (name.equals("relatedArtifact")) { 2334 this.getRelatedArtifact().add(castToRelatedArtifact(value)); 2335 } else if (name.equals("exposureBackground")) { 2336 this.exposureBackground = castToReference(value); // Reference 2337 } else if (name.equals("exposureVariant")) { 2338 this.getExposureVariant().add(castToReference(value)); 2339 } else if (name.equals("outcome")) { 2340 this.getOutcome().add(castToReference(value)); 2341 } else 2342 return super.setProperty(name, value); 2343 return value; 2344 } 2345 2346 @Override 2347 public Base makeProperty(int hash, String name) throws FHIRException { 2348 switch (hash) { 2349 case 116079: 2350 return getUrlElement(); 2351 case -1618432855: 2352 return addIdentifier(); 2353 case 351608024: 2354 return getVersionElement(); 2355 case 3373707: 2356 return getNameElement(); 2357 case 110371416: 2358 return getTitleElement(); 2359 case 1555503932: 2360 return getShortTitleElement(); 2361 case -2060497896: 2362 return getSubtitleElement(); 2363 case -892481550: 2364 return getStatusElement(); 2365 case 3076014: 2366 return getDateElement(); 2367 case 1447404028: 2368 return getPublisherElement(); 2369 case 951526432: 2370 return addContact(); 2371 case -1724546052: 2372 return getDescriptionElement(); 2373 case 3387378: 2374 return addNote(); 2375 case -669707736: 2376 return addUseContext(); 2377 case -507075711: 2378 return addJurisdiction(); 2379 case 1522889671: 2380 return getCopyrightElement(); 2381 case 223539345: 2382 return getApprovalDateElement(); 2383 case -1687512484: 2384 return getLastReviewDateElement(); 2385 case -403934648: 2386 return getEffectivePeriod(); 2387 case 110546223: 2388 return addTopic(); 2389 case -1406328437: 2390 return addAuthor(); 2391 case -1307827859: 2392 return addEditor(); 2393 case -261190139: 2394 return addReviewer(); 2395 case 1740277666: 2396 return addEndorser(); 2397 case 666807069: 2398 return addRelatedArtifact(); 2399 case -832155243: 2400 return getExposureBackground(); 2401 case -19675778: 2402 return addExposureVariant(); 2403 case -1106507950: 2404 return addOutcome(); 2405 default: 2406 return super.makeProperty(hash, name); 2407 } 2408 2409 } 2410 2411 @Override 2412 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2413 switch (hash) { 2414 case 116079: 2415 /* url */ return new String[] { "uri" }; 2416 case -1618432855: 2417 /* identifier */ return new String[] { "Identifier" }; 2418 case 351608024: 2419 /* version */ return new String[] { "string" }; 2420 case 3373707: 2421 /* name */ return new String[] { "string" }; 2422 case 110371416: 2423 /* title */ return new String[] { "string" }; 2424 case 1555503932: 2425 /* shortTitle */ return new String[] { "string" }; 2426 case -2060497896: 2427 /* subtitle */ return new String[] { "string" }; 2428 case -892481550: 2429 /* status */ return new String[] { "code" }; 2430 case 3076014: 2431 /* date */ return new String[] { "dateTime" }; 2432 case 1447404028: 2433 /* publisher */ return new String[] { "string" }; 2434 case 951526432: 2435 /* contact */ return new String[] { "ContactDetail" }; 2436 case -1724546052: 2437 /* description */ return new String[] { "markdown" }; 2438 case 3387378: 2439 /* note */ return new String[] { "Annotation" }; 2440 case -669707736: 2441 /* useContext */ return new String[] { "UsageContext" }; 2442 case -507075711: 2443 /* jurisdiction */ return new String[] { "CodeableConcept" }; 2444 case 1522889671: 2445 /* copyright */ return new String[] { "markdown" }; 2446 case 223539345: 2447 /* approvalDate */ return new String[] { "date" }; 2448 case -1687512484: 2449 /* lastReviewDate */ return new String[] { "date" }; 2450 case -403934648: 2451 /* effectivePeriod */ return new String[] { "Period" }; 2452 case 110546223: 2453 /* topic */ return new String[] { "CodeableConcept" }; 2454 case -1406328437: 2455 /* author */ return new String[] { "ContactDetail" }; 2456 case -1307827859: 2457 /* editor */ return new String[] { "ContactDetail" }; 2458 case -261190139: 2459 /* reviewer */ return new String[] { "ContactDetail" }; 2460 case 1740277666: 2461 /* endorser */ return new String[] { "ContactDetail" }; 2462 case 666807069: 2463 /* relatedArtifact */ return new String[] { "RelatedArtifact" }; 2464 case -832155243: 2465 /* exposureBackground */ return new String[] { "Reference" }; 2466 case -19675778: 2467 /* exposureVariant */ return new String[] { "Reference" }; 2468 case -1106507950: 2469 /* outcome */ return new String[] { "Reference" }; 2470 default: 2471 return super.getTypesForProperty(hash, name); 2472 } 2473 2474 } 2475 2476 @Override 2477 public Base addChild(String name) throws FHIRException { 2478 if (name.equals("url")) { 2479 throw new FHIRException("Cannot call addChild on a singleton property Evidence.url"); 2480 } else if (name.equals("identifier")) { 2481 return addIdentifier(); 2482 } else if (name.equals("version")) { 2483 throw new FHIRException("Cannot call addChild on a singleton property Evidence.version"); 2484 } else if (name.equals("name")) { 2485 throw new FHIRException("Cannot call addChild on a singleton property Evidence.name"); 2486 } else if (name.equals("title")) { 2487 throw new FHIRException("Cannot call addChild on a singleton property Evidence.title"); 2488 } else if (name.equals("shortTitle")) { 2489 throw new FHIRException("Cannot call addChild on a singleton property Evidence.shortTitle"); 2490 } else if (name.equals("subtitle")) { 2491 throw new FHIRException("Cannot call addChild on a singleton property Evidence.subtitle"); 2492 } else if (name.equals("status")) { 2493 throw new FHIRException("Cannot call addChild on a singleton property Evidence.status"); 2494 } else if (name.equals("date")) { 2495 throw new FHIRException("Cannot call addChild on a singleton property Evidence.date"); 2496 } else if (name.equals("publisher")) { 2497 throw new FHIRException("Cannot call addChild on a singleton property Evidence.publisher"); 2498 } else if (name.equals("contact")) { 2499 return addContact(); 2500 } else if (name.equals("description")) { 2501 throw new FHIRException("Cannot call addChild on a singleton property Evidence.description"); 2502 } else if (name.equals("note")) { 2503 return addNote(); 2504 } else if (name.equals("useContext")) { 2505 return addUseContext(); 2506 } else if (name.equals("jurisdiction")) { 2507 return addJurisdiction(); 2508 } else if (name.equals("copyright")) { 2509 throw new FHIRException("Cannot call addChild on a singleton property Evidence.copyright"); 2510 } else if (name.equals("approvalDate")) { 2511 throw new FHIRException("Cannot call addChild on a singleton property Evidence.approvalDate"); 2512 } else if (name.equals("lastReviewDate")) { 2513 throw new FHIRException("Cannot call addChild on a singleton property Evidence.lastReviewDate"); 2514 } else if (name.equals("effectivePeriod")) { 2515 this.effectivePeriod = new Period(); 2516 return this.effectivePeriod; 2517 } else if (name.equals("topic")) { 2518 return addTopic(); 2519 } else if (name.equals("author")) { 2520 return addAuthor(); 2521 } else if (name.equals("editor")) { 2522 return addEditor(); 2523 } else if (name.equals("reviewer")) { 2524 return addReviewer(); 2525 } else if (name.equals("endorser")) { 2526 return addEndorser(); 2527 } else if (name.equals("relatedArtifact")) { 2528 return addRelatedArtifact(); 2529 } else if (name.equals("exposureBackground")) { 2530 this.exposureBackground = new Reference(); 2531 return this.exposureBackground; 2532 } else if (name.equals("exposureVariant")) { 2533 return addExposureVariant(); 2534 } else if (name.equals("outcome")) { 2535 return addOutcome(); 2536 } else 2537 return super.addChild(name); 2538 } 2539 2540 public String fhirType() { 2541 return "Evidence"; 2542 2543 } 2544 2545 public Evidence copy() { 2546 Evidence dst = new Evidence(); 2547 copyValues(dst); 2548 return dst; 2549 } 2550 2551 public void copyValues(Evidence dst) { 2552 super.copyValues(dst); 2553 dst.url = url == null ? null : url.copy(); 2554 if (identifier != null) { 2555 dst.identifier = new ArrayList<Identifier>(); 2556 for (Identifier i : identifier) 2557 dst.identifier.add(i.copy()); 2558 } 2559 ; 2560 dst.version = version == null ? null : version.copy(); 2561 dst.name = name == null ? null : name.copy(); 2562 dst.title = title == null ? null : title.copy(); 2563 dst.shortTitle = shortTitle == null ? null : shortTitle.copy(); 2564 dst.subtitle = subtitle == null ? null : subtitle.copy(); 2565 dst.status = status == null ? null : status.copy(); 2566 dst.date = date == null ? null : date.copy(); 2567 dst.publisher = publisher == null ? null : publisher.copy(); 2568 if (contact != null) { 2569 dst.contact = new ArrayList<ContactDetail>(); 2570 for (ContactDetail i : contact) 2571 dst.contact.add(i.copy()); 2572 } 2573 ; 2574 dst.description = description == null ? null : description.copy(); 2575 if (note != null) { 2576 dst.note = new ArrayList<Annotation>(); 2577 for (Annotation i : note) 2578 dst.note.add(i.copy()); 2579 } 2580 ; 2581 if (useContext != null) { 2582 dst.useContext = new ArrayList<UsageContext>(); 2583 for (UsageContext i : useContext) 2584 dst.useContext.add(i.copy()); 2585 } 2586 ; 2587 if (jurisdiction != null) { 2588 dst.jurisdiction = new ArrayList<CodeableConcept>(); 2589 for (CodeableConcept i : jurisdiction) 2590 dst.jurisdiction.add(i.copy()); 2591 } 2592 ; 2593 dst.copyright = copyright == null ? null : copyright.copy(); 2594 dst.approvalDate = approvalDate == null ? null : approvalDate.copy(); 2595 dst.lastReviewDate = lastReviewDate == null ? null : lastReviewDate.copy(); 2596 dst.effectivePeriod = effectivePeriod == null ? null : effectivePeriod.copy(); 2597 if (topic != null) { 2598 dst.topic = new ArrayList<CodeableConcept>(); 2599 for (CodeableConcept i : topic) 2600 dst.topic.add(i.copy()); 2601 } 2602 ; 2603 if (author != null) { 2604 dst.author = new ArrayList<ContactDetail>(); 2605 for (ContactDetail i : author) 2606 dst.author.add(i.copy()); 2607 } 2608 ; 2609 if (editor != null) { 2610 dst.editor = new ArrayList<ContactDetail>(); 2611 for (ContactDetail i : editor) 2612 dst.editor.add(i.copy()); 2613 } 2614 ; 2615 if (reviewer != null) { 2616 dst.reviewer = new ArrayList<ContactDetail>(); 2617 for (ContactDetail i : reviewer) 2618 dst.reviewer.add(i.copy()); 2619 } 2620 ; 2621 if (endorser != null) { 2622 dst.endorser = new ArrayList<ContactDetail>(); 2623 for (ContactDetail i : endorser) 2624 dst.endorser.add(i.copy()); 2625 } 2626 ; 2627 if (relatedArtifact != null) { 2628 dst.relatedArtifact = new ArrayList<RelatedArtifact>(); 2629 for (RelatedArtifact i : relatedArtifact) 2630 dst.relatedArtifact.add(i.copy()); 2631 } 2632 ; 2633 dst.exposureBackground = exposureBackground == null ? null : exposureBackground.copy(); 2634 if (exposureVariant != null) { 2635 dst.exposureVariant = new ArrayList<Reference>(); 2636 for (Reference i : exposureVariant) 2637 dst.exposureVariant.add(i.copy()); 2638 } 2639 ; 2640 if (outcome != null) { 2641 dst.outcome = new ArrayList<Reference>(); 2642 for (Reference i : outcome) 2643 dst.outcome.add(i.copy()); 2644 } 2645 ; 2646 } 2647 2648 protected Evidence typedCopy() { 2649 return copy(); 2650 } 2651 2652 @Override 2653 public boolean equalsDeep(Base other_) { 2654 if (!super.equalsDeep(other_)) 2655 return false; 2656 if (!(other_ instanceof Evidence)) 2657 return false; 2658 Evidence o = (Evidence) other_; 2659 return compareDeep(identifier, o.identifier, true) && compareDeep(shortTitle, o.shortTitle, true) 2660 && compareDeep(subtitle, o.subtitle, true) && compareDeep(note, o.note, true) 2661 && compareDeep(copyright, o.copyright, true) && compareDeep(approvalDate, o.approvalDate, true) 2662 && compareDeep(lastReviewDate, o.lastReviewDate, true) && compareDeep(effectivePeriod, o.effectivePeriod, true) 2663 && compareDeep(topic, o.topic, true) && compareDeep(author, o.author, true) 2664 && compareDeep(editor, o.editor, true) && compareDeep(reviewer, o.reviewer, true) 2665 && compareDeep(endorser, o.endorser, true) && compareDeep(relatedArtifact, o.relatedArtifact, true) 2666 && compareDeep(exposureBackground, o.exposureBackground, true) 2667 && compareDeep(exposureVariant, o.exposureVariant, true) && compareDeep(outcome, o.outcome, true); 2668 } 2669 2670 @Override 2671 public boolean equalsShallow(Base other_) { 2672 if (!super.equalsShallow(other_)) 2673 return false; 2674 if (!(other_ instanceof Evidence)) 2675 return false; 2676 Evidence o = (Evidence) other_; 2677 return compareValues(shortTitle, o.shortTitle, true) && compareValues(subtitle, o.subtitle, true) 2678 && compareValues(copyright, o.copyright, true) && compareValues(approvalDate, o.approvalDate, true) 2679 && compareValues(lastReviewDate, o.lastReviewDate, true); 2680 } 2681 2682 public boolean isEmpty() { 2683 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, shortTitle, subtitle, note, copyright, 2684 approvalDate, lastReviewDate, effectivePeriod, topic, author, editor, reviewer, endorser, relatedArtifact, 2685 exposureBackground, exposureVariant, outcome); 2686 } 2687 2688 @Override 2689 public ResourceType getResourceType() { 2690 return ResourceType.Evidence; 2691 } 2692 2693 /** 2694 * Search parameter: <b>date</b> 2695 * <p> 2696 * Description: <b>The evidence publication date</b><br> 2697 * Type: <b>date</b><br> 2698 * Path: <b>Evidence.date</b><br> 2699 * </p> 2700 */ 2701 @SearchParamDefinition(name = "date", path = "Evidence.date", description = "The evidence publication date", type = "date") 2702 public static final String SP_DATE = "date"; 2703 /** 2704 * <b>Fluent Client</b> search parameter constant for <b>date</b> 2705 * <p> 2706 * Description: <b>The evidence publication date</b><br> 2707 * Type: <b>date</b><br> 2708 * Path: <b>Evidence.date</b><br> 2709 * </p> 2710 */ 2711 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam( 2712 SP_DATE); 2713 2714 /** 2715 * Search parameter: <b>identifier</b> 2716 * <p> 2717 * Description: <b>External identifier for the evidence</b><br> 2718 * Type: <b>token</b><br> 2719 * Path: <b>Evidence.identifier</b><br> 2720 * </p> 2721 */ 2722 @SearchParamDefinition(name = "identifier", path = "Evidence.identifier", description = "External identifier for the evidence", type = "token") 2723 public static final String SP_IDENTIFIER = "identifier"; 2724 /** 2725 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2726 * <p> 2727 * Description: <b>External identifier for the evidence</b><br> 2728 * Type: <b>token</b><br> 2729 * Path: <b>Evidence.identifier</b><br> 2730 * </p> 2731 */ 2732 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2733 SP_IDENTIFIER); 2734 2735 /** 2736 * Search parameter: <b>successor</b> 2737 * <p> 2738 * Description: <b>What resource is being referenced</b><br> 2739 * Type: <b>reference</b><br> 2740 * Path: <b>Evidence.relatedArtifact.resource</b><br> 2741 * </p> 2742 */ 2743 @SearchParamDefinition(name = "successor", path = "Evidence.relatedArtifact.where(type='successor').resource", description = "What resource is being referenced", type = "reference") 2744 public static final String SP_SUCCESSOR = "successor"; 2745 /** 2746 * <b>Fluent Client</b> search parameter constant for <b>successor</b> 2747 * <p> 2748 * Description: <b>What resource is being referenced</b><br> 2749 * Type: <b>reference</b><br> 2750 * Path: <b>Evidence.relatedArtifact.resource</b><br> 2751 * </p> 2752 */ 2753 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUCCESSOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2754 SP_SUCCESSOR); 2755 2756 /** 2757 * Constant for fluent queries to be used to add include statements. Specifies 2758 * the path value of "<b>Evidence:successor</b>". 2759 */ 2760 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUCCESSOR = new ca.uhn.fhir.model.api.Include( 2761 "Evidence:successor").toLocked(); 2762 2763 /** 2764 * Search parameter: <b>context-type-value</b> 2765 * <p> 2766 * Description: <b>A use context type and value assigned to the evidence</b><br> 2767 * Type: <b>composite</b><br> 2768 * Path: <b></b><br> 2769 * </p> 2770 */ 2771 @SearchParamDefinition(name = "context-type-value", path = "Evidence.useContext", description = "A use context type and value assigned to the evidence", type = "composite", compositeOf = { 2772 "context-type", "context" }) 2773 public static final String SP_CONTEXT_TYPE_VALUE = "context-type-value"; 2774 /** 2775 * <b>Fluent Client</b> search parameter constant for <b>context-type-value</b> 2776 * <p> 2777 * Description: <b>A use context type and value assigned to the evidence</b><br> 2778 * Type: <b>composite</b><br> 2779 * Path: <b></b><br> 2780 * </p> 2781 */ 2782 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CONTEXT_TYPE_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>( 2783 SP_CONTEXT_TYPE_VALUE); 2784 2785 /** 2786 * Search parameter: <b>jurisdiction</b> 2787 * <p> 2788 * Description: <b>Intended jurisdiction for the evidence</b><br> 2789 * Type: <b>token</b><br> 2790 * Path: <b>Evidence.jurisdiction</b><br> 2791 * </p> 2792 */ 2793 @SearchParamDefinition(name = "jurisdiction", path = "Evidence.jurisdiction", description = "Intended jurisdiction for the evidence", type = "token") 2794 public static final String SP_JURISDICTION = "jurisdiction"; 2795 /** 2796 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 2797 * <p> 2798 * Description: <b>Intended jurisdiction for the evidence</b><br> 2799 * Type: <b>token</b><br> 2800 * Path: <b>Evidence.jurisdiction</b><br> 2801 * </p> 2802 */ 2803 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2804 SP_JURISDICTION); 2805 2806 /** 2807 * Search parameter: <b>description</b> 2808 * <p> 2809 * Description: <b>The description of the evidence</b><br> 2810 * Type: <b>string</b><br> 2811 * Path: <b>Evidence.description</b><br> 2812 * </p> 2813 */ 2814 @SearchParamDefinition(name = "description", path = "Evidence.description", description = "The description of the evidence", type = "string") 2815 public static final String SP_DESCRIPTION = "description"; 2816 /** 2817 * <b>Fluent Client</b> search parameter constant for <b>description</b> 2818 * <p> 2819 * Description: <b>The description of the evidence</b><br> 2820 * Type: <b>string</b><br> 2821 * Path: <b>Evidence.description</b><br> 2822 * </p> 2823 */ 2824 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam( 2825 SP_DESCRIPTION); 2826 2827 /** 2828 * Search parameter: <b>derived-from</b> 2829 * <p> 2830 * Description: <b>What resource is being referenced</b><br> 2831 * Type: <b>reference</b><br> 2832 * Path: <b>Evidence.relatedArtifact.resource</b><br> 2833 * </p> 2834 */ 2835 @SearchParamDefinition(name = "derived-from", path = "Evidence.relatedArtifact.where(type='derived-from').resource", description = "What resource is being referenced", type = "reference") 2836 public static final String SP_DERIVED_FROM = "derived-from"; 2837 /** 2838 * <b>Fluent Client</b> search parameter constant for <b>derived-from</b> 2839 * <p> 2840 * Description: <b>What resource is being referenced</b><br> 2841 * Type: <b>reference</b><br> 2842 * Path: <b>Evidence.relatedArtifact.resource</b><br> 2843 * </p> 2844 */ 2845 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DERIVED_FROM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2846 SP_DERIVED_FROM); 2847 2848 /** 2849 * Constant for fluent queries to be used to add include statements. Specifies 2850 * the path value of "<b>Evidence:derived-from</b>". 2851 */ 2852 public static final ca.uhn.fhir.model.api.Include INCLUDE_DERIVED_FROM = new ca.uhn.fhir.model.api.Include( 2853 "Evidence:derived-from").toLocked(); 2854 2855 /** 2856 * Search parameter: <b>context-type</b> 2857 * <p> 2858 * Description: <b>A type of use context assigned to the evidence</b><br> 2859 * Type: <b>token</b><br> 2860 * Path: <b>Evidence.useContext.code</b><br> 2861 * </p> 2862 */ 2863 @SearchParamDefinition(name = "context-type", path = "Evidence.useContext.code", description = "A type of use context assigned to the evidence", type = "token") 2864 public static final String SP_CONTEXT_TYPE = "context-type"; 2865 /** 2866 * <b>Fluent Client</b> search parameter constant for <b>context-type</b> 2867 * <p> 2868 * Description: <b>A type of use context assigned to the evidence</b><br> 2869 * Type: <b>token</b><br> 2870 * Path: <b>Evidence.useContext.code</b><br> 2871 * </p> 2872 */ 2873 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2874 SP_CONTEXT_TYPE); 2875 2876 /** 2877 * Search parameter: <b>predecessor</b> 2878 * <p> 2879 * Description: <b>What resource is being referenced</b><br> 2880 * Type: <b>reference</b><br> 2881 * Path: <b>Evidence.relatedArtifact.resource</b><br> 2882 * </p> 2883 */ 2884 @SearchParamDefinition(name = "predecessor", path = "Evidence.relatedArtifact.where(type='predecessor').resource", description = "What resource is being referenced", type = "reference") 2885 public static final String SP_PREDECESSOR = "predecessor"; 2886 /** 2887 * <b>Fluent Client</b> search parameter constant for <b>predecessor</b> 2888 * <p> 2889 * Description: <b>What resource is being referenced</b><br> 2890 * Type: <b>reference</b><br> 2891 * Path: <b>Evidence.relatedArtifact.resource</b><br> 2892 * </p> 2893 */ 2894 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PREDECESSOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2895 SP_PREDECESSOR); 2896 2897 /** 2898 * Constant for fluent queries to be used to add include statements. Specifies 2899 * the path value of "<b>Evidence:predecessor</b>". 2900 */ 2901 public static final ca.uhn.fhir.model.api.Include INCLUDE_PREDECESSOR = new ca.uhn.fhir.model.api.Include( 2902 "Evidence:predecessor").toLocked(); 2903 2904 /** 2905 * Search parameter: <b>title</b> 2906 * <p> 2907 * Description: <b>The human-friendly name of the evidence</b><br> 2908 * Type: <b>string</b><br> 2909 * Path: <b>Evidence.title</b><br> 2910 * </p> 2911 */ 2912 @SearchParamDefinition(name = "title", path = "Evidence.title", description = "The human-friendly name of the evidence", type = "string") 2913 public static final String SP_TITLE = "title"; 2914 /** 2915 * <b>Fluent Client</b> search parameter constant for <b>title</b> 2916 * <p> 2917 * Description: <b>The human-friendly name of the evidence</b><br> 2918 * Type: <b>string</b><br> 2919 * Path: <b>Evidence.title</b><br> 2920 * </p> 2921 */ 2922 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam( 2923 SP_TITLE); 2924 2925 /** 2926 * Search parameter: <b>composed-of</b> 2927 * <p> 2928 * Description: <b>What resource is being referenced</b><br> 2929 * Type: <b>reference</b><br> 2930 * Path: <b>Evidence.relatedArtifact.resource</b><br> 2931 * </p> 2932 */ 2933 @SearchParamDefinition(name = "composed-of", path = "Evidence.relatedArtifact.where(type='composed-of').resource", description = "What resource is being referenced", type = "reference") 2934 public static final String SP_COMPOSED_OF = "composed-of"; 2935 /** 2936 * <b>Fluent Client</b> search parameter constant for <b>composed-of</b> 2937 * <p> 2938 * Description: <b>What resource is being referenced</b><br> 2939 * Type: <b>reference</b><br> 2940 * Path: <b>Evidence.relatedArtifact.resource</b><br> 2941 * </p> 2942 */ 2943 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam COMPOSED_OF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 2944 SP_COMPOSED_OF); 2945 2946 /** 2947 * Constant for fluent queries to be used to add include statements. Specifies 2948 * the path value of "<b>Evidence:composed-of</b>". 2949 */ 2950 public static final ca.uhn.fhir.model.api.Include INCLUDE_COMPOSED_OF = new ca.uhn.fhir.model.api.Include( 2951 "Evidence:composed-of").toLocked(); 2952 2953 /** 2954 * Search parameter: <b>version</b> 2955 * <p> 2956 * Description: <b>The business version of the evidence</b><br> 2957 * Type: <b>token</b><br> 2958 * Path: <b>Evidence.version</b><br> 2959 * </p> 2960 */ 2961 @SearchParamDefinition(name = "version", path = "Evidence.version", description = "The business version of the evidence", type = "token") 2962 public static final String SP_VERSION = "version"; 2963 /** 2964 * <b>Fluent Client</b> search parameter constant for <b>version</b> 2965 * <p> 2966 * Description: <b>The business version of the evidence</b><br> 2967 * Type: <b>token</b><br> 2968 * Path: <b>Evidence.version</b><br> 2969 * </p> 2970 */ 2971 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam( 2972 SP_VERSION); 2973 2974 /** 2975 * Search parameter: <b>url</b> 2976 * <p> 2977 * Description: <b>The uri that identifies the evidence</b><br> 2978 * Type: <b>uri</b><br> 2979 * Path: <b>Evidence.url</b><br> 2980 * </p> 2981 */ 2982 @SearchParamDefinition(name = "url", path = "Evidence.url", description = "The uri that identifies the evidence", type = "uri") 2983 public static final String SP_URL = "url"; 2984 /** 2985 * <b>Fluent Client</b> search parameter constant for <b>url</b> 2986 * <p> 2987 * Description: <b>The uri that identifies the evidence</b><br> 2988 * Type: <b>uri</b><br> 2989 * Path: <b>Evidence.url</b><br> 2990 * </p> 2991 */ 2992 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 2993 2994 /** 2995 * Search parameter: <b>context-quantity</b> 2996 * <p> 2997 * Description: <b>A quantity- or range-valued use context assigned to the 2998 * evidence</b><br> 2999 * Type: <b>quantity</b><br> 3000 * Path: <b>Evidence.useContext.valueQuantity, 3001 * Evidence.useContext.valueRange</b><br> 3002 * </p> 3003 */ 3004 @SearchParamDefinition(name = "context-quantity", path = "(Evidence.useContext.value as Quantity) | (Evidence.useContext.value as Range)", description = "A quantity- or range-valued use context assigned to the evidence", type = "quantity") 3005 public static final String SP_CONTEXT_QUANTITY = "context-quantity"; 3006 /** 3007 * <b>Fluent Client</b> search parameter constant for <b>context-quantity</b> 3008 * <p> 3009 * Description: <b>A quantity- or range-valued use context assigned to the 3010 * evidence</b><br> 3011 * Type: <b>quantity</b><br> 3012 * Path: <b>Evidence.useContext.valueQuantity, 3013 * Evidence.useContext.valueRange</b><br> 3014 * </p> 3015 */ 3016 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam CONTEXT_QUANTITY = new ca.uhn.fhir.rest.gclient.QuantityClientParam( 3017 SP_CONTEXT_QUANTITY); 3018 3019 /** 3020 * Search parameter: <b>effective</b> 3021 * <p> 3022 * Description: <b>The time during which the evidence is intended to be in 3023 * use</b><br> 3024 * Type: <b>date</b><br> 3025 * Path: <b>Evidence.effectivePeriod</b><br> 3026 * </p> 3027 */ 3028 @SearchParamDefinition(name = "effective", path = "Evidence.effectivePeriod", description = "The time during which the evidence is intended to be in use", type = "date") 3029 public static final String SP_EFFECTIVE = "effective"; 3030 /** 3031 * <b>Fluent Client</b> search parameter constant for <b>effective</b> 3032 * <p> 3033 * Description: <b>The time during which the evidence is intended to be in 3034 * use</b><br> 3035 * Type: <b>date</b><br> 3036 * Path: <b>Evidence.effectivePeriod</b><br> 3037 * </p> 3038 */ 3039 public static final ca.uhn.fhir.rest.gclient.DateClientParam EFFECTIVE = new ca.uhn.fhir.rest.gclient.DateClientParam( 3040 SP_EFFECTIVE); 3041 3042 /** 3043 * Search parameter: <b>depends-on</b> 3044 * <p> 3045 * Description: <b>What resource is being referenced</b><br> 3046 * Type: <b>reference</b><br> 3047 * Path: <b>Evidence.relatedArtifact.resource</b><br> 3048 * </p> 3049 */ 3050 @SearchParamDefinition(name = "depends-on", path = "Evidence.relatedArtifact.where(type='depends-on').resource", description = "What resource is being referenced", type = "reference") 3051 public static final String SP_DEPENDS_ON = "depends-on"; 3052 /** 3053 * <b>Fluent Client</b> search parameter constant for <b>depends-on</b> 3054 * <p> 3055 * Description: <b>What resource is being referenced</b><br> 3056 * Type: <b>reference</b><br> 3057 * Path: <b>Evidence.relatedArtifact.resource</b><br> 3058 * </p> 3059 */ 3060 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEPENDS_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 3061 SP_DEPENDS_ON); 3062 3063 /** 3064 * Constant for fluent queries to be used to add include statements. Specifies 3065 * the path value of "<b>Evidence:depends-on</b>". 3066 */ 3067 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEPENDS_ON = new ca.uhn.fhir.model.api.Include( 3068 "Evidence:depends-on").toLocked(); 3069 3070 /** 3071 * Search parameter: <b>name</b> 3072 * <p> 3073 * Description: <b>Computationally friendly name of the evidence</b><br> 3074 * Type: <b>string</b><br> 3075 * Path: <b>Evidence.name</b><br> 3076 * </p> 3077 */ 3078 @SearchParamDefinition(name = "name", path = "Evidence.name", description = "Computationally friendly name of the evidence", type = "string") 3079 public static final String SP_NAME = "name"; 3080 /** 3081 * <b>Fluent Client</b> search parameter constant for <b>name</b> 3082 * <p> 3083 * Description: <b>Computationally friendly name of the evidence</b><br> 3084 * Type: <b>string</b><br> 3085 * Path: <b>Evidence.name</b><br> 3086 * </p> 3087 */ 3088 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam( 3089 SP_NAME); 3090 3091 /** 3092 * Search parameter: <b>context</b> 3093 * <p> 3094 * Description: <b>A use context assigned to the evidence</b><br> 3095 * Type: <b>token</b><br> 3096 * Path: <b>Evidence.useContext.valueCodeableConcept</b><br> 3097 * </p> 3098 */ 3099 @SearchParamDefinition(name = "context", path = "(Evidence.useContext.value as CodeableConcept)", description = "A use context assigned to the evidence", type = "token") 3100 public static final String SP_CONTEXT = "context"; 3101 /** 3102 * <b>Fluent Client</b> search parameter constant for <b>context</b> 3103 * <p> 3104 * Description: <b>A use context assigned to the evidence</b><br> 3105 * Type: <b>token</b><br> 3106 * Path: <b>Evidence.useContext.valueCodeableConcept</b><br> 3107 * </p> 3108 */ 3109 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3110 SP_CONTEXT); 3111 3112 /** 3113 * Search parameter: <b>publisher</b> 3114 * <p> 3115 * Description: <b>Name of the publisher of the evidence</b><br> 3116 * Type: <b>string</b><br> 3117 * Path: <b>Evidence.publisher</b><br> 3118 * </p> 3119 */ 3120 @SearchParamDefinition(name = "publisher", path = "Evidence.publisher", description = "Name of the publisher of the evidence", type = "string") 3121 public static final String SP_PUBLISHER = "publisher"; 3122 /** 3123 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 3124 * <p> 3125 * Description: <b>Name of the publisher of the evidence</b><br> 3126 * Type: <b>string</b><br> 3127 * Path: <b>Evidence.publisher</b><br> 3128 * </p> 3129 */ 3130 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam( 3131 SP_PUBLISHER); 3132 3133 /** 3134 * Search parameter: <b>topic</b> 3135 * <p> 3136 * Description: <b>Topics associated with the Evidence</b><br> 3137 * Type: <b>token</b><br> 3138 * Path: <b>Evidence.topic</b><br> 3139 * </p> 3140 */ 3141 @SearchParamDefinition(name = "topic", path = "Evidence.topic", description = "Topics associated with the Evidence", type = "token") 3142 public static final String SP_TOPIC = "topic"; 3143 /** 3144 * <b>Fluent Client</b> search parameter constant for <b>topic</b> 3145 * <p> 3146 * Description: <b>Topics associated with the Evidence</b><br> 3147 * Type: <b>token</b><br> 3148 * Path: <b>Evidence.topic</b><br> 3149 * </p> 3150 */ 3151 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TOPIC = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3152 SP_TOPIC); 3153 3154 /** 3155 * Search parameter: <b>context-type-quantity</b> 3156 * <p> 3157 * Description: <b>A use context type and quantity- or range-based value 3158 * assigned to the evidence</b><br> 3159 * Type: <b>composite</b><br> 3160 * Path: <b></b><br> 3161 * </p> 3162 */ 3163 @SearchParamDefinition(name = "context-type-quantity", path = "Evidence.useContext", description = "A use context type and quantity- or range-based value assigned to the evidence", type = "composite", compositeOf = { 3164 "context-type", "context-quantity" }) 3165 public static final String SP_CONTEXT_TYPE_QUANTITY = "context-type-quantity"; 3166 /** 3167 * <b>Fluent Client</b> search parameter constant for 3168 * <b>context-type-quantity</b> 3169 * <p> 3170 * Description: <b>A use context type and quantity- or range-based value 3171 * assigned to the evidence</b><br> 3172 * Type: <b>composite</b><br> 3173 * Path: <b></b><br> 3174 * </p> 3175 */ 3176 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam> CONTEXT_TYPE_QUANTITY = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.QuantityClientParam>( 3177 SP_CONTEXT_TYPE_QUANTITY); 3178 3179 /** 3180 * Search parameter: <b>status</b> 3181 * <p> 3182 * Description: <b>The current status of the evidence</b><br> 3183 * Type: <b>token</b><br> 3184 * Path: <b>Evidence.status</b><br> 3185 * </p> 3186 */ 3187 @SearchParamDefinition(name = "status", path = "Evidence.status", description = "The current status of the evidence", type = "token") 3188 public static final String SP_STATUS = "status"; 3189 /** 3190 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3191 * <p> 3192 * Description: <b>The current status of the evidence</b><br> 3193 * Type: <b>token</b><br> 3194 * Path: <b>Evidence.status</b><br> 3195 * </p> 3196 */ 3197 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam( 3198 SP_STATUS); 3199 3200}