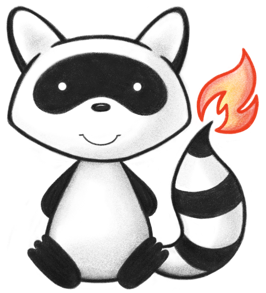
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.ICompositeType; 038import org.hl7.fhir.utilities.Utilities; 039 040import ca.uhn.fhir.model.api.annotation.Child; 041import ca.uhn.fhir.model.api.annotation.DatatypeDef; 042import ca.uhn.fhir.model.api.annotation.Description; 043 044/** 045 * Specifies contact information for a person or organization. 046 */ 047@DatatypeDef(name = "ContactDetail") 048public class ContactDetail extends Type implements ICompositeType { 049 050 /** 051 * The name of an individual to contact. 052 */ 053 @Child(name = "name", type = { StringType.class }, order = 0, min = 0, max = 1, modifier = false, summary = true) 054 @Description(shortDefinition = "Name of an individual to contact", formalDefinition = "The name of an individual to contact.") 055 protected StringType name; 056 057 /** 058 * The contact details for the individual (if a name was provided) or the 059 * organization. 060 */ 061 @Child(name = "telecom", type = { 062 ContactPoint.class }, order = 1, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 063 @Description(shortDefinition = "Contact details for individual or organization", formalDefinition = "The contact details for the individual (if a name was provided) or the organization.") 064 protected List<ContactPoint> telecom; 065 066 private static final long serialVersionUID = 816838773L; 067 068 /** 069 * Constructor 070 */ 071 public ContactDetail() { 072 super(); 073 } 074 075 /** 076 * @return {@link #name} (The name of an individual to contact.). This is the 077 * underlying object with id, value and extensions. The accessor 078 * "getName" gives direct access to the value 079 */ 080 public StringType getNameElement() { 081 if (this.name == null) 082 if (Configuration.errorOnAutoCreate()) 083 throw new Error("Attempt to auto-create ContactDetail.name"); 084 else if (Configuration.doAutoCreate()) 085 this.name = new StringType(); // bb 086 return this.name; 087 } 088 089 public boolean hasNameElement() { 090 return this.name != null && !this.name.isEmpty(); 091 } 092 093 public boolean hasName() { 094 return this.name != null && !this.name.isEmpty(); 095 } 096 097 /** 098 * @param value {@link #name} (The name of an individual to contact.). This is 099 * the underlying object with id, value and extensions. The 100 * accessor "getName" gives direct access to the value 101 */ 102 public ContactDetail setNameElement(StringType value) { 103 this.name = value; 104 return this; 105 } 106 107 /** 108 * @return The name of an individual to contact. 109 */ 110 public String getName() { 111 return this.name == null ? null : this.name.getValue(); 112 } 113 114 /** 115 * @param value The name of an individual to contact. 116 */ 117 public ContactDetail setName(String value) { 118 if (Utilities.noString(value)) 119 this.name = null; 120 else { 121 if (this.name == null) 122 this.name = new StringType(); 123 this.name.setValue(value); 124 } 125 return this; 126 } 127 128 /** 129 * @return {@link #telecom} (The contact details for the individual (if a name 130 * was provided) or the organization.) 131 */ 132 public List<ContactPoint> getTelecom() { 133 if (this.telecom == null) 134 this.telecom = new ArrayList<ContactPoint>(); 135 return this.telecom; 136 } 137 138 /** 139 * @return Returns a reference to <code>this</code> for easy method chaining 140 */ 141 public ContactDetail setTelecom(List<ContactPoint> theTelecom) { 142 this.telecom = theTelecom; 143 return this; 144 } 145 146 public boolean hasTelecom() { 147 if (this.telecom == null) 148 return false; 149 for (ContactPoint item : this.telecom) 150 if (!item.isEmpty()) 151 return true; 152 return false; 153 } 154 155 public ContactPoint addTelecom() { // 3 156 ContactPoint t = new ContactPoint(); 157 if (this.telecom == null) 158 this.telecom = new ArrayList<ContactPoint>(); 159 this.telecom.add(t); 160 return t; 161 } 162 163 public ContactDetail addTelecom(ContactPoint t) { // 3 164 if (t == null) 165 return this; 166 if (this.telecom == null) 167 this.telecom = new ArrayList<ContactPoint>(); 168 this.telecom.add(t); 169 return this; 170 } 171 172 /** 173 * @return The first repetition of repeating field {@link #telecom}, creating it 174 * if it does not already exist 175 */ 176 public ContactPoint getTelecomFirstRep() { 177 if (getTelecom().isEmpty()) { 178 addTelecom(); 179 } 180 return getTelecom().get(0); 181 } 182 183 protected void listChildren(List<Property> children) { 184 super.listChildren(children); 185 children.add(new Property("name", "string", "The name of an individual to contact.", 0, 1, name)); 186 children.add(new Property("telecom", "ContactPoint", 187 "The contact details for the individual (if a name was provided) or the organization.", 0, 188 java.lang.Integer.MAX_VALUE, telecom)); 189 } 190 191 @Override 192 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 193 switch (_hash) { 194 case 3373707: 195 /* name */ return new Property("name", "string", "The name of an individual to contact.", 0, 1, name); 196 case -1429363305: 197 /* telecom */ return new Property("telecom", "ContactPoint", 198 "The contact details for the individual (if a name was provided) or the organization.", 0, 199 java.lang.Integer.MAX_VALUE, telecom); 200 default: 201 return super.getNamedProperty(_hash, _name, _checkValid); 202 } 203 204 } 205 206 @Override 207 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 208 switch (hash) { 209 case 3373707: 210 /* name */ return this.name == null ? new Base[0] : new Base[] { this.name }; // StringType 211 case -1429363305: 212 /* telecom */ return this.telecom == null ? new Base[0] : this.telecom.toArray(new Base[this.telecom.size()]); // ContactPoint 213 default: 214 return super.getProperty(hash, name, checkValid); 215 } 216 217 } 218 219 @Override 220 public Base setProperty(int hash, String name, Base value) throws FHIRException { 221 switch (hash) { 222 case 3373707: // name 223 this.name = castToString(value); // StringType 224 return value; 225 case -1429363305: // telecom 226 this.getTelecom().add(castToContactPoint(value)); // ContactPoint 227 return value; 228 default: 229 return super.setProperty(hash, name, value); 230 } 231 232 } 233 234 @Override 235 public Base setProperty(String name, Base value) throws FHIRException { 236 if (name.equals("name")) { 237 this.name = castToString(value); // StringType 238 } else if (name.equals("telecom")) { 239 this.getTelecom().add(castToContactPoint(value)); 240 } else 241 return super.setProperty(name, value); 242 return value; 243 } 244 245 @Override 246 public Base makeProperty(int hash, String name) throws FHIRException { 247 switch (hash) { 248 case 3373707: 249 return getNameElement(); 250 case -1429363305: 251 return addTelecom(); 252 default: 253 return super.makeProperty(hash, name); 254 } 255 256 } 257 258 @Override 259 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 260 switch (hash) { 261 case 3373707: 262 /* name */ return new String[] { "string" }; 263 case -1429363305: 264 /* telecom */ return new String[] { "ContactPoint" }; 265 default: 266 return super.getTypesForProperty(hash, name); 267 } 268 269 } 270 271 @Override 272 public Base addChild(String name) throws FHIRException { 273 if (name.equals("name")) { 274 throw new FHIRException("Cannot call addChild on a singleton property ContactDetail.name"); 275 } else if (name.equals("telecom")) { 276 return addTelecom(); 277 } else 278 return super.addChild(name); 279 } 280 281 public String fhirType() { 282 return "ContactDetail"; 283 284 } 285 286 public ContactDetail copy() { 287 ContactDetail dst = new ContactDetail(); 288 copyValues(dst); 289 return dst; 290 } 291 292 public void copyValues(ContactDetail dst) { 293 super.copyValues(dst); 294 dst.name = name == null ? null : name.copy(); 295 if (telecom != null) { 296 dst.telecom = new ArrayList<ContactPoint>(); 297 for (ContactPoint i : telecom) 298 dst.telecom.add(i.copy()); 299 } 300 ; 301 } 302 303 protected ContactDetail typedCopy() { 304 return copy(); 305 } 306 307 @Override 308 public boolean equalsDeep(Base other_) { 309 if (!super.equalsDeep(other_)) 310 return false; 311 if (!(other_ instanceof ContactDetail)) 312 return false; 313 ContactDetail o = (ContactDetail) other_; 314 return compareDeep(name, o.name, true) && compareDeep(telecom, o.telecom, true); 315 } 316 317 @Override 318 public boolean equalsShallow(Base other_) { 319 if (!super.equalsShallow(other_)) 320 return false; 321 if (!(other_ instanceof ContactDetail)) 322 return false; 323 ContactDetail o = (ContactDetail) other_; 324 return compareValues(name, o.name, true); 325 } 326 327 public boolean isEmpty() { 328 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, telecom); 329 } 330 331}