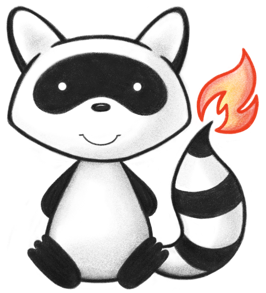
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Tue, May 12, 2020 07:26+1000 for FHIR v4.0.1 033import java.util.ArrayList; 034import java.util.Date; 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038 039import ca.uhn.fhir.model.api.annotation.Child; 040import ca.uhn.fhir.model.api.annotation.Description; 041import ca.uhn.fhir.model.api.annotation.ResourceDef; 042import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 043 044/** 045 * Basic is used for handling concepts not yet defined in FHIR, narrative-only 046 * resources that don't map to an existing resource, and custom resources not 047 * appropriate for inclusion in the FHIR specification. 048 */ 049@ResourceDef(name = "Basic", profile = "http://hl7.org/fhir/StructureDefinition/Basic") 050public class Basic extends DomainResource { 051 052 /** 053 * Identifier assigned to the resource for business purposes, outside the 054 * context of FHIR. 055 */ 056 @Child(name = "identifier", type = { 057 Identifier.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = false, summary = true) 058 @Description(shortDefinition = "Business identifier", formalDefinition = "Identifier assigned to the resource for business purposes, outside the context of FHIR.") 059 protected List<Identifier> identifier; 060 061 /** 062 * Identifies the 'type' of resource - equivalent to the resource name for other 063 * resources. 064 */ 065 @Child(name = "code", type = { CodeableConcept.class }, order = 1, min = 1, max = 1, modifier = true, summary = true) 066 @Description(shortDefinition = "Kind of Resource", formalDefinition = "Identifies the 'type' of resource - equivalent to the resource name for other resources.") 067 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/basic-resource-type") 068 protected CodeableConcept code; 069 070 /** 071 * Identifies the patient, practitioner, device or any other resource that is 072 * the "focus" of this resource. 073 */ 074 @Child(name = "subject", type = { Reference.class }, order = 2, min = 0, max = 1, modifier = false, summary = true) 075 @Description(shortDefinition = "Identifies the focus of this resource", formalDefinition = "Identifies the patient, practitioner, device or any other resource that is the \"focus\" of this resource.") 076 protected Reference subject; 077 078 /** 079 * The actual object that is the target of the reference (Identifies the 080 * patient, practitioner, device or any other resource that is the "focus" of 081 * this resource.) 082 */ 083 protected Resource subjectTarget; 084 085 /** 086 * Identifies when the resource was first created. 087 */ 088 @Child(name = "created", type = { DateType.class }, order = 3, min = 0, max = 1, modifier = false, summary = true) 089 @Description(shortDefinition = "When created", formalDefinition = "Identifies when the resource was first created.") 090 protected DateType created; 091 092 /** 093 * Indicates who was responsible for creating the resource instance. 094 */ 095 @Child(name = "author", type = { Practitioner.class, PractitionerRole.class, Patient.class, RelatedPerson.class, 096 Organization.class }, order = 4, min = 0, max = 1, modifier = false, summary = true) 097 @Description(shortDefinition = "Who created", formalDefinition = "Indicates who was responsible for creating the resource instance.") 098 protected Reference author; 099 100 /** 101 * The actual object that is the target of the reference (Indicates who was 102 * responsible for creating the resource instance.) 103 */ 104 protected Resource authorTarget; 105 106 private static final long serialVersionUID = 650756402L; 107 108 /** 109 * Constructor 110 */ 111 public Basic() { 112 super(); 113 } 114 115 /** 116 * Constructor 117 */ 118 public Basic(CodeableConcept code) { 119 super(); 120 this.code = code; 121 } 122 123 /** 124 * @return {@link #identifier} (Identifier assigned to the resource for business 125 * purposes, outside the context of FHIR.) 126 */ 127 public List<Identifier> getIdentifier() { 128 if (this.identifier == null) 129 this.identifier = new ArrayList<Identifier>(); 130 return this.identifier; 131 } 132 133 /** 134 * @return Returns a reference to <code>this</code> for easy method chaining 135 */ 136 public Basic setIdentifier(List<Identifier> theIdentifier) { 137 this.identifier = theIdentifier; 138 return this; 139 } 140 141 public boolean hasIdentifier() { 142 if (this.identifier == null) 143 return false; 144 for (Identifier item : this.identifier) 145 if (!item.isEmpty()) 146 return true; 147 return false; 148 } 149 150 public Identifier addIdentifier() { // 3 151 Identifier t = new Identifier(); 152 if (this.identifier == null) 153 this.identifier = new ArrayList<Identifier>(); 154 this.identifier.add(t); 155 return t; 156 } 157 158 public Basic addIdentifier(Identifier t) { // 3 159 if (t == null) 160 return this; 161 if (this.identifier == null) 162 this.identifier = new ArrayList<Identifier>(); 163 this.identifier.add(t); 164 return this; 165 } 166 167 /** 168 * @return The first repetition of repeating field {@link #identifier}, creating 169 * it if it does not already exist 170 */ 171 public Identifier getIdentifierFirstRep() { 172 if (getIdentifier().isEmpty()) { 173 addIdentifier(); 174 } 175 return getIdentifier().get(0); 176 } 177 178 /** 179 * @return {@link #code} (Identifies the 'type' of resource - equivalent to the 180 * resource name for other resources.) 181 */ 182 public CodeableConcept getCode() { 183 if (this.code == null) 184 if (Configuration.errorOnAutoCreate()) 185 throw new Error("Attempt to auto-create Basic.code"); 186 else if (Configuration.doAutoCreate()) 187 this.code = new CodeableConcept(); // cc 188 return this.code; 189 } 190 191 public boolean hasCode() { 192 return this.code != null && !this.code.isEmpty(); 193 } 194 195 /** 196 * @param value {@link #code} (Identifies the 'type' of resource - equivalent to 197 * the resource name for other resources.) 198 */ 199 public Basic setCode(CodeableConcept value) { 200 this.code = value; 201 return this; 202 } 203 204 /** 205 * @return {@link #subject} (Identifies the patient, practitioner, device or any 206 * other resource that is the "focus" of this resource.) 207 */ 208 public Reference getSubject() { 209 if (this.subject == null) 210 if (Configuration.errorOnAutoCreate()) 211 throw new Error("Attempt to auto-create Basic.subject"); 212 else if (Configuration.doAutoCreate()) 213 this.subject = new Reference(); // cc 214 return this.subject; 215 } 216 217 public boolean hasSubject() { 218 return this.subject != null && !this.subject.isEmpty(); 219 } 220 221 /** 222 * @param value {@link #subject} (Identifies the patient, practitioner, device 223 * or any other resource that is the "focus" of this resource.) 224 */ 225 public Basic setSubject(Reference value) { 226 this.subject = value; 227 return this; 228 } 229 230 /** 231 * @return {@link #subject} The actual object that is the target of the 232 * reference. The reference library doesn't populate this, but you can 233 * use it to hold the resource if you resolve it. (Identifies the 234 * patient, practitioner, device or any other resource that is the 235 * "focus" of this resource.) 236 */ 237 public Resource getSubjectTarget() { 238 return this.subjectTarget; 239 } 240 241 /** 242 * @param value {@link #subject} The actual object that is the target of the 243 * reference. The reference library doesn't use these, but you can 244 * use it to hold the resource if you resolve it. (Identifies the 245 * patient, practitioner, device or any other resource that is the 246 * "focus" of this resource.) 247 */ 248 public Basic setSubjectTarget(Resource value) { 249 this.subjectTarget = value; 250 return this; 251 } 252 253 /** 254 * @return {@link #created} (Identifies when the resource was first created.). 255 * This is the underlying object with id, value and extensions. The 256 * accessor "getCreated" gives direct access to the value 257 */ 258 public DateType getCreatedElement() { 259 if (this.created == null) 260 if (Configuration.errorOnAutoCreate()) 261 throw new Error("Attempt to auto-create Basic.created"); 262 else if (Configuration.doAutoCreate()) 263 this.created = new DateType(); // bb 264 return this.created; 265 } 266 267 public boolean hasCreatedElement() { 268 return this.created != null && !this.created.isEmpty(); 269 } 270 271 public boolean hasCreated() { 272 return this.created != null && !this.created.isEmpty(); 273 } 274 275 /** 276 * @param value {@link #created} (Identifies when the resource was first 277 * created.). This is the underlying object with id, value and 278 * extensions. The accessor "getCreated" gives direct access to the 279 * value 280 */ 281 public Basic setCreatedElement(DateType value) { 282 this.created = value; 283 return this; 284 } 285 286 /** 287 * @return Identifies when the resource was first created. 288 */ 289 public Date getCreated() { 290 return this.created == null ? null : this.created.getValue(); 291 } 292 293 /** 294 * @param value Identifies when the resource was first created. 295 */ 296 public Basic setCreated(Date value) { 297 if (value == null) 298 this.created = null; 299 else { 300 if (this.created == null) 301 this.created = new DateType(); 302 this.created.setValue(value); 303 } 304 return this; 305 } 306 307 /** 308 * @return {@link #author} (Indicates who was responsible for creating the 309 * resource instance.) 310 */ 311 public Reference getAuthor() { 312 if (this.author == null) 313 if (Configuration.errorOnAutoCreate()) 314 throw new Error("Attempt to auto-create Basic.author"); 315 else if (Configuration.doAutoCreate()) 316 this.author = new Reference(); // cc 317 return this.author; 318 } 319 320 public boolean hasAuthor() { 321 return this.author != null && !this.author.isEmpty(); 322 } 323 324 /** 325 * @param value {@link #author} (Indicates who was responsible for creating the 326 * resource instance.) 327 */ 328 public Basic setAuthor(Reference value) { 329 this.author = value; 330 return this; 331 } 332 333 /** 334 * @return {@link #author} The actual object that is the target of the 335 * reference. The reference library doesn't populate this, but you can 336 * use it to hold the resource if you resolve it. (Indicates who was 337 * responsible for creating the resource instance.) 338 */ 339 public Resource getAuthorTarget() { 340 return this.authorTarget; 341 } 342 343 /** 344 * @param value {@link #author} The actual object that is the target of the 345 * reference. The reference library doesn't use these, but you can 346 * use it to hold the resource if you resolve it. (Indicates who 347 * was responsible for creating the resource instance.) 348 */ 349 public Basic setAuthorTarget(Resource value) { 350 this.authorTarget = value; 351 return this; 352 } 353 354 protected void listChildren(List<Property> children) { 355 super.listChildren(children); 356 children.add(new Property("identifier", "Identifier", 357 "Identifier assigned to the resource for business purposes, outside the context of FHIR.", 0, 358 java.lang.Integer.MAX_VALUE, identifier)); 359 children.add(new Property("code", "CodeableConcept", 360 "Identifies the 'type' of resource - equivalent to the resource name for other resources.", 0, 1, code)); 361 children.add(new Property("subject", "Reference(Any)", 362 "Identifies the patient, practitioner, device or any other resource that is the \"focus\" of this resource.", 0, 363 1, subject)); 364 children.add(new Property("created", "date", "Identifies when the resource was first created.", 0, 1, created)); 365 children.add(new Property("author", "Reference(Practitioner|PractitionerRole|Patient|RelatedPerson|Organization)", 366 "Indicates who was responsible for creating the resource instance.", 0, 1, author)); 367 } 368 369 @Override 370 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 371 switch (_hash) { 372 case -1618432855: 373 /* identifier */ return new Property("identifier", "Identifier", 374 "Identifier assigned to the resource for business purposes, outside the context of FHIR.", 0, 375 java.lang.Integer.MAX_VALUE, identifier); 376 case 3059181: 377 /* code */ return new Property("code", "CodeableConcept", 378 "Identifies the 'type' of resource - equivalent to the resource name for other resources.", 0, 1, code); 379 case -1867885268: 380 /* subject */ return new Property("subject", "Reference(Any)", 381 "Identifies the patient, practitioner, device or any other resource that is the \"focus\" of this resource.", 382 0, 1, subject); 383 case 1028554472: 384 /* created */ return new Property("created", "date", "Identifies when the resource was first created.", 0, 1, 385 created); 386 case -1406328437: 387 /* author */ return new Property("author", 388 "Reference(Practitioner|PractitionerRole|Patient|RelatedPerson|Organization)", 389 "Indicates who was responsible for creating the resource instance.", 0, 1, author); 390 default: 391 return super.getNamedProperty(_hash, _name, _checkValid); 392 } 393 394 } 395 396 @Override 397 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 398 switch (hash) { 399 case -1618432855: 400 /* identifier */ return this.identifier == null ? new Base[0] 401 : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 402 case 3059181: 403 /* code */ return this.code == null ? new Base[0] : new Base[] { this.code }; // CodeableConcept 404 case -1867885268: 405 /* subject */ return this.subject == null ? new Base[0] : new Base[] { this.subject }; // Reference 406 case 1028554472: 407 /* created */ return this.created == null ? new Base[0] : new Base[] { this.created }; // DateType 408 case -1406328437: 409 /* author */ return this.author == null ? new Base[0] : new Base[] { this.author }; // Reference 410 default: 411 return super.getProperty(hash, name, checkValid); 412 } 413 414 } 415 416 @Override 417 public Base setProperty(int hash, String name, Base value) throws FHIRException { 418 switch (hash) { 419 case -1618432855: // identifier 420 this.getIdentifier().add(castToIdentifier(value)); // Identifier 421 return value; 422 case 3059181: // code 423 this.code = castToCodeableConcept(value); // CodeableConcept 424 return value; 425 case -1867885268: // subject 426 this.subject = castToReference(value); // Reference 427 return value; 428 case 1028554472: // created 429 this.created = castToDate(value); // DateType 430 return value; 431 case -1406328437: // author 432 this.author = castToReference(value); // Reference 433 return value; 434 default: 435 return super.setProperty(hash, name, value); 436 } 437 438 } 439 440 @Override 441 public Base setProperty(String name, Base value) throws FHIRException { 442 if (name.equals("identifier")) { 443 this.getIdentifier().add(castToIdentifier(value)); 444 } else if (name.equals("code")) { 445 this.code = castToCodeableConcept(value); // CodeableConcept 446 } else if (name.equals("subject")) { 447 this.subject = castToReference(value); // Reference 448 } else if (name.equals("created")) { 449 this.created = castToDate(value); // DateType 450 } else if (name.equals("author")) { 451 this.author = castToReference(value); // Reference 452 } else 453 return super.setProperty(name, value); 454 return value; 455 } 456 457 @Override 458 public Base makeProperty(int hash, String name) throws FHIRException { 459 switch (hash) { 460 case -1618432855: 461 return addIdentifier(); 462 case 3059181: 463 return getCode(); 464 case -1867885268: 465 return getSubject(); 466 case 1028554472: 467 return getCreatedElement(); 468 case -1406328437: 469 return getAuthor(); 470 default: 471 return super.makeProperty(hash, name); 472 } 473 474 } 475 476 @Override 477 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 478 switch (hash) { 479 case -1618432855: 480 /* identifier */ return new String[] { "Identifier" }; 481 case 3059181: 482 /* code */ return new String[] { "CodeableConcept" }; 483 case -1867885268: 484 /* subject */ return new String[] { "Reference" }; 485 case 1028554472: 486 /* created */ return new String[] { "date" }; 487 case -1406328437: 488 /* author */ return new String[] { "Reference" }; 489 default: 490 return super.getTypesForProperty(hash, name); 491 } 492 493 } 494 495 @Override 496 public Base addChild(String name) throws FHIRException { 497 if (name.equals("identifier")) { 498 return addIdentifier(); 499 } else if (name.equals("code")) { 500 this.code = new CodeableConcept(); 501 return this.code; 502 } else if (name.equals("subject")) { 503 this.subject = new Reference(); 504 return this.subject; 505 } else if (name.equals("created")) { 506 throw new FHIRException("Cannot call addChild on a singleton property Basic.created"); 507 } else if (name.equals("author")) { 508 this.author = new Reference(); 509 return this.author; 510 } else 511 return super.addChild(name); 512 } 513 514 public String fhirType() { 515 return "Basic"; 516 517 } 518 519 public Basic copy() { 520 Basic dst = new Basic(); 521 copyValues(dst); 522 return dst; 523 } 524 525 public void copyValues(Basic dst) { 526 super.copyValues(dst); 527 if (identifier != null) { 528 dst.identifier = new ArrayList<Identifier>(); 529 for (Identifier i : identifier) 530 dst.identifier.add(i.copy()); 531 } 532 ; 533 dst.code = code == null ? null : code.copy(); 534 dst.subject = subject == null ? null : subject.copy(); 535 dst.created = created == null ? null : created.copy(); 536 dst.author = author == null ? null : author.copy(); 537 } 538 539 protected Basic typedCopy() { 540 return copy(); 541 } 542 543 @Override 544 public boolean equalsDeep(Base other_) { 545 if (!super.equalsDeep(other_)) 546 return false; 547 if (!(other_ instanceof Basic)) 548 return false; 549 Basic o = (Basic) other_; 550 return compareDeep(identifier, o.identifier, true) && compareDeep(code, o.code, true) 551 && compareDeep(subject, o.subject, true) && compareDeep(created, o.created, true) 552 && compareDeep(author, o.author, true); 553 } 554 555 @Override 556 public boolean equalsShallow(Base other_) { 557 if (!super.equalsShallow(other_)) 558 return false; 559 if (!(other_ instanceof Basic)) 560 return false; 561 Basic o = (Basic) other_; 562 return compareValues(created, o.created, true); 563 } 564 565 public boolean isEmpty() { 566 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, code, subject, created, author); 567 } 568 569 @Override 570 public ResourceType getResourceType() { 571 return ResourceType.Basic; 572 } 573 574 /** 575 * Search parameter: <b>identifier</b> 576 * <p> 577 * Description: <b>Business identifier</b><br> 578 * Type: <b>token</b><br> 579 * Path: <b>Basic.identifier</b><br> 580 * </p> 581 */ 582 @SearchParamDefinition(name = "identifier", path = "Basic.identifier", description = "Business identifier", type = "token") 583 public static final String SP_IDENTIFIER = "identifier"; 584 /** 585 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 586 * <p> 587 * Description: <b>Business identifier</b><br> 588 * Type: <b>token</b><br> 589 * Path: <b>Basic.identifier</b><br> 590 * </p> 591 */ 592 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam( 593 SP_IDENTIFIER); 594 595 /** 596 * Search parameter: <b>code</b> 597 * <p> 598 * Description: <b>Kind of Resource</b><br> 599 * Type: <b>token</b><br> 600 * Path: <b>Basic.code</b><br> 601 * </p> 602 */ 603 @SearchParamDefinition(name = "code", path = "Basic.code", description = "Kind of Resource", type = "token") 604 public static final String SP_CODE = "code"; 605 /** 606 * <b>Fluent Client</b> search parameter constant for <b>code</b> 607 * <p> 608 * Description: <b>Kind of Resource</b><br> 609 * Type: <b>token</b><br> 610 * Path: <b>Basic.code</b><br> 611 * </p> 612 */ 613 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam( 614 SP_CODE); 615 616 /** 617 * Search parameter: <b>subject</b> 618 * <p> 619 * Description: <b>Identifies the focus of this resource</b><br> 620 * Type: <b>reference</b><br> 621 * Path: <b>Basic.subject</b><br> 622 * </p> 623 */ 624 @SearchParamDefinition(name = "subject", path = "Basic.subject", description = "Identifies the focus of this resource", type = "reference") 625 public static final String SP_SUBJECT = "subject"; 626 /** 627 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 628 * <p> 629 * Description: <b>Identifies the focus of this resource</b><br> 630 * Type: <b>reference</b><br> 631 * Path: <b>Basic.subject</b><br> 632 * </p> 633 */ 634 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 635 SP_SUBJECT); 636 637 /** 638 * Constant for fluent queries to be used to add include statements. Specifies 639 * the path value of "<b>Basic:subject</b>". 640 */ 641 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("Basic:subject") 642 .toLocked(); 643 644 /** 645 * Search parameter: <b>created</b> 646 * <p> 647 * Description: <b>When created</b><br> 648 * Type: <b>date</b><br> 649 * Path: <b>Basic.created</b><br> 650 * </p> 651 */ 652 @SearchParamDefinition(name = "created", path = "Basic.created", description = "When created", type = "date") 653 public static final String SP_CREATED = "created"; 654 /** 655 * <b>Fluent Client</b> search parameter constant for <b>created</b> 656 * <p> 657 * Description: <b>When created</b><br> 658 * Type: <b>date</b><br> 659 * Path: <b>Basic.created</b><br> 660 * </p> 661 */ 662 public static final ca.uhn.fhir.rest.gclient.DateClientParam CREATED = new ca.uhn.fhir.rest.gclient.DateClientParam( 663 SP_CREATED); 664 665 /** 666 * Search parameter: <b>patient</b> 667 * <p> 668 * Description: <b>Identifies the focus of this resource</b><br> 669 * Type: <b>reference</b><br> 670 * Path: <b>Basic.subject</b><br> 671 * </p> 672 */ 673 @SearchParamDefinition(name = "patient", path = "Basic.subject.where(resolve() is Patient)", description = "Identifies the focus of this resource", type = "reference", providesMembershipIn = { 674 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient") }, target = { Patient.class }) 675 public static final String SP_PATIENT = "patient"; 676 /** 677 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 678 * <p> 679 * Description: <b>Identifies the focus of this resource</b><br> 680 * Type: <b>reference</b><br> 681 * Path: <b>Basic.subject</b><br> 682 * </p> 683 */ 684 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 685 SP_PATIENT); 686 687 /** 688 * Constant for fluent queries to be used to add include statements. Specifies 689 * the path value of "<b>Basic:patient</b>". 690 */ 691 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("Basic:patient") 692 .toLocked(); 693 694 /** 695 * Search parameter: <b>author</b> 696 * <p> 697 * Description: <b>Who created</b><br> 698 * Type: <b>reference</b><br> 699 * Path: <b>Basic.author</b><br> 700 * </p> 701 */ 702 @SearchParamDefinition(name = "author", path = "Basic.author", description = "Who created", type = "reference", providesMembershipIn = { 703 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Patient"), 704 @ca.uhn.fhir.model.api.annotation.Compartment(name = "Practitioner"), 705 @ca.uhn.fhir.model.api.annotation.Compartment(name = "RelatedPerson") }, target = { Organization.class, 706 Patient.class, Practitioner.class, PractitionerRole.class, RelatedPerson.class }) 707 public static final String SP_AUTHOR = "author"; 708 /** 709 * <b>Fluent Client</b> search parameter constant for <b>author</b> 710 * <p> 711 * Description: <b>Who created</b><br> 712 * Type: <b>reference</b><br> 713 * Path: <b>Basic.author</b><br> 714 * </p> 715 */ 716 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam AUTHOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam( 717 SP_AUTHOR); 718 719 /** 720 * Constant for fluent queries to be used to add include statements. Specifies 721 * the path value of "<b>Basic:author</b>". 722 */ 723 public static final ca.uhn.fhir.model.api.Include INCLUDE_AUTHOR = new ca.uhn.fhir.model.api.Include("Basic:author") 724 .toLocked(); 725 726}