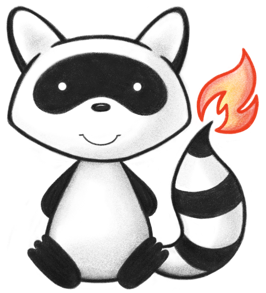
001package org.hl7.fhir.r4.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030*/ 031 032// Generated on Mon, Mar 5, 2018 18:26+1100 for FHIR v3.2.0 033import java.util.ArrayList; 034import java.util.List; 035 036import org.hl7.fhir.exceptions.FHIRException; 037import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 038 039import ca.uhn.fhir.model.api.annotation.Child; 040import ca.uhn.fhir.model.api.annotation.DatatypeDef; 041import ca.uhn.fhir.model.api.annotation.Description; 042 043/** 044 * Base definition for all elements that are defined inside a resource - but not 045 * those in a data type. 046 */ 047@DatatypeDef(name = "BackboneElement") 048public abstract class BackboneType extends Type implements IBaseBackboneElement { 049 050 /** 051 * May be used to represent additional information that is not part of the basic 052 * definition of the element, and that modifies the understanding of the element 053 * that contains it. Usually modifier elements provide negation or 054 * qualification. In order to make the use of extensions safe and manageable, 055 * there is a strict set of governance applied to the definition and use of 056 * extensions. Though any implementer is allowed to define an extension, there 057 * is a set of requirements that SHALL be met as part of the definition of the 058 * extension. Applications processing a resource are required to check for 059 * modifier extensions. 060 */ 061 @Child(name = "modifierExtension", type = { 062 Extension.class }, order = 0, min = 0, max = Child.MAX_UNLIMITED, modifier = true, summary = true) 063 @Description(shortDefinition = "Extensions that cannot be ignored", formalDefinition = "May be used to represent additional information that is not part of the basic definition of the element, and that modifies the understanding of the element that contains it. Usually modifier elements provide negation or qualification. In order to make the use of extensions safe and manageable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer is allowed to define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension. Applications processing a resource are required to check for modifier extensions.") 064 protected List<Extension> modifierExtension; 065 066 private static final long serialVersionUID = -1431673179L; 067 068 /** 069 * Constructor 070 */ 071 public BackboneType() { 072 super(); 073 } 074 075 /** 076 * @return {@link #modifierExtension} (May be used to represent additional 077 * information that is not part of the basic definition of the element, 078 * and that modifies the understanding of the element that contains it. 079 * Usually modifier elements provide negation or qualification. In order 080 * to make the use of extensions safe and manageable, there is a strict 081 * set of governance applied to the definition and use of extensions. 082 * Though any implementer is allowed to define an extension, there is a 083 * set of requirements that SHALL be met as part of the definition of 084 * the extension. Applications processing a resource are required to 085 * check for modifier extensions.) 086 */ 087 public List<Extension> getModifierExtension() { 088 if (this.modifierExtension == null) 089 this.modifierExtension = new ArrayList<Extension>(); 090 return this.modifierExtension; 091 } 092 093 /** 094 * @return Returns a reference to <code>this</code> for easy method chaining 095 */ 096 public BackboneType setModifierExtension(List<Extension> theModifierExtension) { 097 this.modifierExtension = theModifierExtension; 098 return this; 099 } 100 101 public boolean hasModifierExtension() { 102 if (this.modifierExtension == null) 103 return false; 104 for (Extension item : this.modifierExtension) 105 if (!item.isEmpty()) 106 return true; 107 return false; 108 } 109 110 public Extension addModifierExtension() { // 3 111 Extension t = new Extension(); 112 if (this.modifierExtension == null) 113 this.modifierExtension = new ArrayList<Extension>(); 114 this.modifierExtension.add(t); 115 return t; 116 } 117 118 public BackboneType addModifierExtension(Extension t) { // 3 119 if (t == null) 120 return this; 121 if (this.modifierExtension == null) 122 this.modifierExtension = new ArrayList<Extension>(); 123 this.modifierExtension.add(t); 124 return this; 125 } 126 127 /** 128 * @return The first repetition of repeating field {@link #modifierExtension}, 129 * creating it if it does not already exist 130 */ 131 public Extension getModifierExtensionFirstRep() { 132 if (getModifierExtension().isEmpty()) { 133 addModifierExtension(); 134 } 135 return getModifierExtension().get(0); 136 } 137 138 protected void listChildren(List<Property> children) { 139 children.add(new Property("modifierExtension", "Extension", 140 "May be used to represent additional information that is not part of the basic definition of the element, and that modifies the understanding of the element that contains it. Usually modifier elements provide negation or qualification. In order to make the use of extensions safe and manageable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer is allowed to define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension. Applications processing a resource are required to check for modifier extensions.", 141 0, java.lang.Integer.MAX_VALUE, modifierExtension)); 142 } 143 144 @Override 145 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 146 switch (_hash) { 147 case -298878168: 148 /* modifierExtension */ return new Property("modifierExtension", "Extension", 149 "May be used to represent additional information that is not part of the basic definition of the element, and that modifies the understanding of the element that contains it. Usually modifier elements provide negation or qualification. In order to make the use of extensions safe and manageable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer is allowed to define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension. Applications processing a resource are required to check for modifier extensions.", 150 0, java.lang.Integer.MAX_VALUE, modifierExtension); 151 default: 152 return super.getNamedProperty(_hash, _name, _checkValid); 153 } 154 155 } 156 157 @Override 158 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 159 switch (hash) { 160 case -298878168: 161 /* modifierExtension */ return this.modifierExtension == null ? new Base[0] 162 : this.modifierExtension.toArray(new Base[this.modifierExtension.size()]); // Extension 163 default: 164 return super.getProperty(hash, name, checkValid); 165 } 166 167 } 168 169 @Override 170 public Base setProperty(int hash, String name, Base value) throws FHIRException { 171 switch (hash) { 172 case -298878168: // modifierExtension 173 this.getModifierExtension().add(castToExtension(value)); // Extension 174 return value; 175 default: 176 return super.setProperty(hash, name, value); 177 } 178 179 } 180 181 @Override 182 public Base setProperty(String name, Base value) throws FHIRException { 183 if (name.equals("modifierExtension")) { 184 this.getModifierExtension().add(castToExtension(value)); 185 } else 186 return super.setProperty(name, value); 187 return value; 188 } 189 190 @Override 191 public Base makeProperty(int hash, String name) throws FHIRException { 192 switch (hash) { 193 case -298878168: 194 return addModifierExtension(); 195 default: 196 return super.makeProperty(hash, name); 197 } 198 199 } 200 201 @Override 202 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 203 switch (hash) { 204 case -298878168: 205 /* modifierExtension */ return new String[] { "Extension" }; 206 default: 207 return super.getTypesForProperty(hash, name); 208 } 209 210 } 211 212 @Override 213 public Base addChild(String name) throws FHIRException { 214 if (name.equals("modifierExtension")) { 215 return addModifierExtension(); 216 } else 217 return super.addChild(name); 218 } 219 220 public String fhirType() { 221 return "BackboneElement"; 222 223 } 224 225 public abstract BackboneType copy(); 226 227 public void copyValues(BackboneType dst) { 228 super.copyValues(dst); 229 if (modifierExtension != null) { 230 dst.modifierExtension = new ArrayList<Extension>(); 231 for (Extension i : modifierExtension) 232 dst.modifierExtension.add(i.copy()); 233 } 234 ; 235 } 236 237 @Override 238 public boolean equalsDeep(Base other_) { 239 if (!super.equalsDeep(other_)) 240 return false; 241 if (!(other_ instanceof BackboneType)) 242 return false; 243 BackboneType o = (BackboneType) other_; 244 return compareDeep(modifierExtension, o.modifierExtension, true); 245 } 246 247 @Override 248 public boolean equalsShallow(Base other_) { 249 if (!super.equalsShallow(other_)) 250 return false; 251 if (!(other_ instanceof BackboneType)) 252 return false; 253 BackboneType o = (BackboneType) other_; 254 return true; 255 } 256 257 public boolean isEmpty() { 258 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(modifierExtension); 259 } 260 261// added from java-adornments.txt: 262 263 public void checkNoModifiers(String noun, String verb) throws FHIRException { 264 if (hasModifierExtension()) { 265 throw new FHIRException("Found unknown Modifier Exceptions on " + noun + " doing " + verb); 266 } 267 268 } 269 270// end addition 271 272}