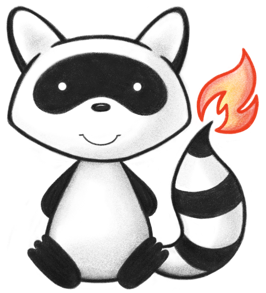
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum SubstanceCategory { 041 042 /** 043 * A substance that causes an allergic reaction. 044 */ 045 ALLERGEN, 046 /** 047 * A substance that is produced by or extracted from a biological source. 048 */ 049 BIOLOGICAL, 050 /** 051 * A substance that comes directly from a human or an animal (e.g. blood, urine, feces, tears, etc.). 052 */ 053 BODY, 054 /** 055 * Any organic or inorganic substance of a particular molecular identity, including -- (i) any combination of such substances occurring in whole or in part as a result of a chemical reaction or occurring in nature and (ii) any element or uncombined radical (http://www.epa.gov/opptintr/import-export/pubs/importguide.pdf). 056 */ 057 CHEMICAL, 058 /** 059 * A food, dietary ingredient, or dietary supplement for human or animal. 060 */ 061 FOOD, 062 /** 063 * A substance intended for use in the diagnosis, cure, mitigation, treatment, or prevention of disease in man or other animals (Federal Food Drug and Cosmetic Act). 064 */ 065 DRUG, 066 /** 067 * A finished product which is not normally ingested, absorbed or injected (e.g. steel, iron, wood, plastic and paper). 068 */ 069 MATERIAL, 070 /** 071 * added to help the parsers 072 */ 073 NULL; 074 public static SubstanceCategory fromCode(String codeString) throws FHIRException { 075 if (codeString == null || "".equals(codeString)) 076 return null; 077 if ("allergen".equals(codeString)) 078 return ALLERGEN; 079 if ("biological".equals(codeString)) 080 return BIOLOGICAL; 081 if ("body".equals(codeString)) 082 return BODY; 083 if ("chemical".equals(codeString)) 084 return CHEMICAL; 085 if ("food".equals(codeString)) 086 return FOOD; 087 if ("drug".equals(codeString)) 088 return DRUG; 089 if ("material".equals(codeString)) 090 return MATERIAL; 091 throw new FHIRException("Unknown SubstanceCategory code '"+codeString+"'"); 092 } 093 public String toCode() { 094 switch (this) { 095 case ALLERGEN: return "allergen"; 096 case BIOLOGICAL: return "biological"; 097 case BODY: return "body"; 098 case CHEMICAL: return "chemical"; 099 case FOOD: return "food"; 100 case DRUG: return "drug"; 101 case MATERIAL: return "material"; 102 case NULL: return null; 103 default: return "?"; 104 } 105 } 106 public String getSystem() { 107 return "http://hl7.org/fhir/substance-category"; 108 } 109 public String getDefinition() { 110 switch (this) { 111 case ALLERGEN: return "A substance that causes an allergic reaction."; 112 case BIOLOGICAL: return "A substance that is produced by or extracted from a biological source."; 113 case BODY: return "A substance that comes directly from a human or an animal (e.g. blood, urine, feces, tears, etc.)."; 114 case CHEMICAL: return "Any organic or inorganic substance of a particular molecular identity, including -- (i) any combination of such substances occurring in whole or in part as a result of a chemical reaction or occurring in nature and (ii) any element or uncombined radical (http://www.epa.gov/opptintr/import-export/pubs/importguide.pdf)."; 115 case FOOD: return "A food, dietary ingredient, or dietary supplement for human or animal."; 116 case DRUG: return "A substance intended for use in the diagnosis, cure, mitigation, treatment, or prevention of disease in man or other animals (Federal Food Drug and Cosmetic Act)."; 117 case MATERIAL: return "A finished product which is not normally ingested, absorbed or injected (e.g. steel, iron, wood, plastic and paper)."; 118 case NULL: return null; 119 default: return "?"; 120 } 121 } 122 public String getDisplay() { 123 switch (this) { 124 case ALLERGEN: return "Allergen"; 125 case BIOLOGICAL: return "Biological Substance"; 126 case BODY: return "Body Substance"; 127 case CHEMICAL: return "Chemical"; 128 case FOOD: return "Dietary Substance"; 129 case DRUG: return "Drug or Medicament"; 130 case MATERIAL: return "Material"; 131 case NULL: return null; 132 default: return "?"; 133 } 134 } 135 136 137}