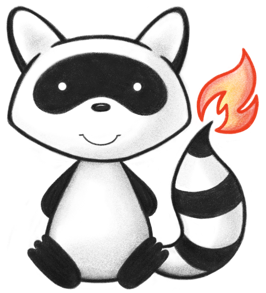
001package org.hl7.fhir.dstu3.model.codesystems; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Sat, Mar 25, 2017 21:03-0400 for FHIR v3.0.0 036 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040public enum BenefitType { 041 042 /** 043 * Maximum benefit allowable. 044 */ 045 BENEFIT, 046 /** 047 * Cost to be incurred before benefits are applied 048 */ 049 DEDUCTABLE, 050 /** 051 * Service visit 052 */ 053 VISIT, 054 /** 055 * Type of room 056 */ 057 ROOM, 058 /** 059 * Copayment per service 060 */ 061 COPAY, 062 /** 063 * Copayment percentage per service 064 */ 065 COPAYPERCENT, 066 /** 067 * Copayment maximum per service 068 */ 069 COPAYMAXIMUM, 070 /** 071 * Vision Exam 072 */ 073 VISIONEXAM, 074 /** 075 * Frames and lenses 076 */ 077 VISIONGLASSES, 078 /** 079 * Contact Lenses 080 */ 081 VISIONCONTACTS, 082 /** 083 * Medical Primary Health Coverage 084 */ 085 MEDICALPRIMARYCARE, 086 /** 087 * Pharmacy Dispense Coverage 088 */ 089 PHARMACYDISPENSE, 090 /** 091 * added to help the parsers 092 */ 093 NULL; 094 public static BenefitType fromCode(String codeString) throws FHIRException { 095 if (codeString == null || "".equals(codeString)) 096 return null; 097 if ("benefit".equals(codeString)) 098 return BENEFIT; 099 if ("deductable".equals(codeString)) 100 return DEDUCTABLE; 101 if ("visit".equals(codeString)) 102 return VISIT; 103 if ("room".equals(codeString)) 104 return ROOM; 105 if ("copay".equals(codeString)) 106 return COPAY; 107 if ("copay-percent".equals(codeString)) 108 return COPAYPERCENT; 109 if ("copay-maximum".equals(codeString)) 110 return COPAYMAXIMUM; 111 if ("vision-exam".equals(codeString)) 112 return VISIONEXAM; 113 if ("vision-glasses".equals(codeString)) 114 return VISIONGLASSES; 115 if ("vision-contacts".equals(codeString)) 116 return VISIONCONTACTS; 117 if ("medical-primarycare".equals(codeString)) 118 return MEDICALPRIMARYCARE; 119 if ("pharmacy-dispense".equals(codeString)) 120 return PHARMACYDISPENSE; 121 throw new FHIRException("Unknown BenefitType code '"+codeString+"'"); 122 } 123 public String toCode() { 124 switch (this) { 125 case BENEFIT: return "benefit"; 126 case DEDUCTABLE: return "deductable"; 127 case VISIT: return "visit"; 128 case ROOM: return "room"; 129 case COPAY: return "copay"; 130 case COPAYPERCENT: return "copay-percent"; 131 case COPAYMAXIMUM: return "copay-maximum"; 132 case VISIONEXAM: return "vision-exam"; 133 case VISIONGLASSES: return "vision-glasses"; 134 case VISIONCONTACTS: return "vision-contacts"; 135 case MEDICALPRIMARYCARE: return "medical-primarycare"; 136 case PHARMACYDISPENSE: return "pharmacy-dispense"; 137 case NULL: return null; 138 default: return "?"; 139 } 140 } 141 public String getSystem() { 142 return "http://hl7.org/fhir/benefit-type"; 143 } 144 public String getDefinition() { 145 switch (this) { 146 case BENEFIT: return "Maximum benefit allowable."; 147 case DEDUCTABLE: return "Cost to be incurred before benefits are applied"; 148 case VISIT: return "Service visit"; 149 case ROOM: return "Type of room"; 150 case COPAY: return "Copayment per service"; 151 case COPAYPERCENT: return "Copayment percentage per service"; 152 case COPAYMAXIMUM: return "Copayment maximum per service"; 153 case VISIONEXAM: return "Vision Exam"; 154 case VISIONGLASSES: return "Frames and lenses"; 155 case VISIONCONTACTS: return "Contact Lenses"; 156 case MEDICALPRIMARYCARE: return "Medical Primary Health Coverage"; 157 case PHARMACYDISPENSE: return "Pharmacy Dispense Coverage"; 158 case NULL: return null; 159 default: return "?"; 160 } 161 } 162 public String getDisplay() { 163 switch (this) { 164 case BENEFIT: return "Benefit"; 165 case DEDUCTABLE: return "Deductable"; 166 case VISIT: return "Visit"; 167 case ROOM: return "Room"; 168 case COPAY: return "Copayment per service"; 169 case COPAYPERCENT: return "Copayment Percent per service"; 170 case COPAYMAXIMUM: return "Copayment maximum per service"; 171 case VISIONEXAM: return "Vision Exam"; 172 case VISIONGLASSES: return "Vision Glasses"; 173 case VISIONCONTACTS: return "Vision Contacts Coverage"; 174 case MEDICALPRIMARYCARE: return "Medical Primary Health Coverage"; 175 case PHARMACYDISPENSE: return "Pharmacy Dispense Coverage"; 176 case NULL: return null; 177 default: return "?"; 178 } 179 } 180 181 182}