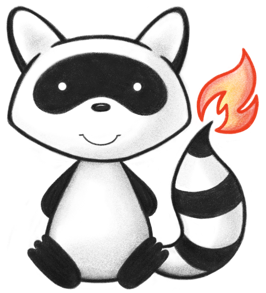
001 002 003 004 005 006 007 008 009 010 011 012 013 014 015 016 017package ca.uhn.fhir.model.dstu2.composite; 018 019import java.net.URI; 020import java.math.BigDecimal; 021import org.apache.commons.lang3.StringUtils; 022import java.util.*; 023import ca.uhn.fhir.model.api.*; 024import ca.uhn.fhir.model.primitive.*; 025import ca.uhn.fhir.model.api.annotation.*; 026import ca.uhn.fhir.model.base.composite.*; 027 028import ca.uhn.fhir.model.dstu2.valueset.AddressTypeEnum; 029import ca.uhn.fhir.model.dstu2.valueset.AddressUseEnum; 030import ca.uhn.fhir.model.dstu2.valueset.AggregationModeEnum; 031import ca.uhn.fhir.model.dstu2.valueset.BindingStrengthEnum; 032import ca.uhn.fhir.model.dstu2.composite.CodeableConceptDt; 033import ca.uhn.fhir.model.dstu2.composite.CodingDt; 034import ca.uhn.fhir.model.dstu2.valueset.ConstraintSeverityEnum; 035import ca.uhn.fhir.model.dstu2.valueset.ContactPointSystemEnum; 036import ca.uhn.fhir.model.dstu2.valueset.ContactPointUseEnum; 037import ca.uhn.fhir.model.dstu2.resource.Device; 038import ca.uhn.fhir.model.dstu2.valueset.EventTimingEnum; 039import ca.uhn.fhir.model.dstu2.valueset.IdentifierTypeCodesEnum; 040import ca.uhn.fhir.model.dstu2.valueset.IdentifierUseEnum; 041import ca.uhn.fhir.model.dstu2.valueset.NameUseEnum; 042import ca.uhn.fhir.model.dstu2.resource.Organization; 043import ca.uhn.fhir.model.dstu2.resource.Patient; 044import ca.uhn.fhir.model.dstu2.composite.PeriodDt; 045import ca.uhn.fhir.model.dstu2.resource.Practitioner; 046import ca.uhn.fhir.model.dstu2.valueset.PropertyRepresentationEnum; 047import ca.uhn.fhir.model.dstu2.valueset.QuantityComparatorEnum; 048import ca.uhn.fhir.model.dstu2.composite.QuantityDt; 049import ca.uhn.fhir.model.dstu2.composite.RangeDt; 050import ca.uhn.fhir.model.dstu2.resource.RelatedPerson; 051import ca.uhn.fhir.model.dstu2.valueset.SignatureTypeCodesEnum; 052import ca.uhn.fhir.model.dstu2.valueset.SlicingRulesEnum; 053import ca.uhn.fhir.model.api.TemporalPrecisionEnum; 054import ca.uhn.fhir.model.dstu2.valueset.TimingAbbreviationEnum; 055import ca.uhn.fhir.model.dstu2.valueset.UnitsOfTimeEnum; 056import ca.uhn.fhir.model.dstu2.resource.ValueSet; 057import ca.uhn.fhir.model.dstu2.composite.BoundCodeableConceptDt; 058import ca.uhn.fhir.model.dstu2.composite.DurationDt; 059import ca.uhn.fhir.model.dstu2.composite.ResourceReferenceDt; 060import ca.uhn.fhir.model.dstu2.composite.SimpleQuantityDt; 061import ca.uhn.fhir.model.primitive.Base64BinaryDt; 062import ca.uhn.fhir.model.primitive.BooleanDt; 063import ca.uhn.fhir.model.primitive.BoundCodeDt; 064import ca.uhn.fhir.model.primitive.CodeDt; 065import ca.uhn.fhir.model.primitive.DateTimeDt; 066import ca.uhn.fhir.model.primitive.DecimalDt; 067import ca.uhn.fhir.model.primitive.IdDt; 068import ca.uhn.fhir.model.primitive.InstantDt; 069import ca.uhn.fhir.model.primitive.IntegerDt; 070import ca.uhn.fhir.model.primitive.MarkdownDt; 071import ca.uhn.fhir.model.primitive.PositiveIntDt; 072import ca.uhn.fhir.model.primitive.StringDt; 073import ca.uhn.fhir.model.primitive.UnsignedIntDt; 074import ca.uhn.fhir.model.primitive.UriDt; 075 076/** 077 * HAPI/FHIR <b>SignatureDt</b> Datatype 078 * () 079 * 080 * <p> 081 * <b>Definition:</b> 082 * A digital signature along with supporting context. The signature may be electronic/cryptographic in nature, or a graphical image representing a hand-written signature, or a signature process. Different Signature approaches have different utilities 083 * </p> 084 * 085 * <p> 086 * <b>Requirements:</b> 087 * There are a number of places where content must be signed in healthcare 088 * </p> 089 */ 090@DatatypeDef(name="Signature") 091public class SignatureDt 092 extends BaseIdentifiableElement 093 implements ICompositeDatatype 094{ 095 096 /** 097 * Constructor 098 */ 099 public SignatureDt() { 100 // nothing 101 } 102 103 104 @Child(name="type", type=CodingDt.class, order=0, min=1, max=Child.MAX_UNLIMITED, summary=true, modifier=false) 105 @Description( 106 shortDefinition="", 107 formalDefinition="An indication of the reason that the entity signed this document. This may be explicitly included as part of the signature information and can be used when determining accountability for various actions concerning the document." 108 ) 109 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/valueset-signature-type") 110 private java.util.List<CodingDt> myType; 111 112 @Child(name="when", type=InstantDt.class, order=1, min=1, max=1, summary=true, modifier=false) 113 @Description( 114 shortDefinition="", 115 formalDefinition="When the digital signature was signed." 116 ) 117 private InstantDt myWhen; 118 119 @Child(name="who", order=2, min=1, max=1, summary=true, modifier=false, type={ 120 UriDt.class, 121 Practitioner.class, 122 RelatedPerson.class, 123 Patient.class, 124 Device.class, 125 Organization.class 126 }) 127 @Description( 128 shortDefinition="", 129 formalDefinition="A reference to an application-usable description of the person that signed the certificate (e.g. the signature used their private key)" 130 ) 131 private IDatatype myWho; 132 133 @Child(name="contentType", type=CodeDt.class, order=3, min=1, max=1, summary=true, modifier=false) 134 @Description( 135 shortDefinition="", 136 formalDefinition="A mime type that indicates the technical format of the signature. Important mime types are application/signature+xml for X ML DigSig, application/jwt for JWT, and image/* for a graphical image of a signature" 137 ) 138 private CodeDt myContentType; 139 140 @Child(name="blob", type=Base64BinaryDt.class, order=4, min=1, max=1, summary=true, modifier=false) 141 @Description( 142 shortDefinition="", 143 formalDefinition="The base64 encoding of the Signature content" 144 ) 145 private Base64BinaryDt myBlob; 146 147 148 @Override 149 public boolean isEmpty() { 150 return super.isBaseEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty( myType, myWhen, myWho, myContentType, myBlob); 151 } 152 153 @Override 154 public <T extends IElement> List<T> getAllPopulatedChildElementsOfType(Class<T> theType) { 155 return ca.uhn.fhir.util.ElementUtil.allPopulatedChildElements(theType, myType, myWhen, myWho, myContentType, myBlob); 156 } 157 158 /** 159 * Gets the value(s) for <b>type</b> (). 160 * creating it if it does 161 * not exist. Will not return <code>null</code>. 162 * 163 * <p> 164 * <b>Definition:</b> 165 * An indication of the reason that the entity signed this document. This may be explicitly included as part of the signature information and can be used when determining accountability for various actions concerning the document. 166 * </p> 167 */ 168 public java.util.List<CodingDt> getType() { 169 if (myType == null) { 170 myType = new java.util.ArrayList<CodingDt>(); 171 } 172 return myType; 173 } 174 175 /** 176 * Sets the value(s) for <b>type</b> () 177 * 178 * <p> 179 * <b>Definition:</b> 180 * An indication of the reason that the entity signed this document. This may be explicitly included as part of the signature information and can be used when determining accountability for various actions concerning the document. 181 * </p> 182 */ 183 public SignatureDt setType(java.util.List<CodingDt> theValue) { 184 myType = theValue; 185 return this; 186 } 187 188 189 190 /** 191 * Adds and returns a new value for <b>type</b> () 192 * 193 * <p> 194 * <b>Definition:</b> 195 * An indication of the reason that the entity signed this document. This may be explicitly included as part of the signature information and can be used when determining accountability for various actions concerning the document. 196 * </p> 197 */ 198 public CodingDt addType() { 199 CodingDt newType = new CodingDt(); 200 getType().add(newType); 201 return newType; 202 } 203 204 /** 205 * Adds a given new value for <b>type</b> () 206 * 207 * <p> 208 * <b>Definition:</b> 209 * An indication of the reason that the entity signed this document. This may be explicitly included as part of the signature information and can be used when determining accountability for various actions concerning the document. 210 * </p> 211 * @param theValue The type to add (must not be <code>null</code>) 212 */ 213 public SignatureDt addType(CodingDt theValue) { 214 if (theValue == null) { 215 throw new NullPointerException("theValue must not be null"); 216 } 217 getType().add(theValue); 218 return this; 219 } 220 221 /** 222 * Gets the first repetition for <b>type</b> (), 223 * creating it if it does not already exist. 224 * 225 * <p> 226 * <b>Definition:</b> 227 * An indication of the reason that the entity signed this document. This may be explicitly included as part of the signature information and can be used when determining accountability for various actions concerning the document. 228 * </p> 229 */ 230 public CodingDt getTypeFirstRep() { 231 if (getType().isEmpty()) { 232 return addType(); 233 } 234 return getType().get(0); 235 } 236 237 /** 238 * Gets the value(s) for <b>when</b> (). 239 * creating it if it does 240 * not exist. Will not return <code>null</code>. 241 * 242 * <p> 243 * <b>Definition:</b> 244 * When the digital signature was signed. 245 * </p> 246 */ 247 public InstantDt getWhenElement() { 248 if (myWhen == null) { 249 myWhen = new InstantDt(); 250 } 251 return myWhen; 252 } 253 254 255 /** 256 * Gets the value(s) for <b>when</b> (). 257 * creating it if it does 258 * not exist. This method may return <code>null</code>. 259 * 260 * <p> 261 * <b>Definition:</b> 262 * When the digital signature was signed. 263 * </p> 264 */ 265 public Date getWhen() { 266 return getWhenElement().getValue(); 267 } 268 269 /** 270 * Sets the value(s) for <b>when</b> () 271 * 272 * <p> 273 * <b>Definition:</b> 274 * When the digital signature was signed. 275 * </p> 276 */ 277 public SignatureDt setWhen(InstantDt theValue) { 278 myWhen = theValue; 279 return this; 280 } 281 282 283 284 /** 285 * Sets the value for <b>when</b> () 286 * 287 * <p> 288 * <b>Definition:</b> 289 * When the digital signature was signed. 290 * </p> 291 */ 292 public SignatureDt setWhenWithMillisPrecision( Date theDate) { 293 myWhen = new InstantDt(theDate); 294 return this; 295 } 296 297 /** 298 * Sets the value for <b>when</b> () 299 * 300 * <p> 301 * <b>Definition:</b> 302 * When the digital signature was signed. 303 * </p> 304 */ 305 public SignatureDt setWhen( Date theDate, TemporalPrecisionEnum thePrecision) { 306 myWhen = new InstantDt(theDate, thePrecision); 307 return this; 308 } 309 310 311 /** 312 * Gets the value(s) for <b>who[x]</b> (). 313 * creating it if it does 314 * not exist. Will not return <code>null</code>. 315 * 316 * <p> 317 * <b>Definition:</b> 318 * A reference to an application-usable description of the person that signed the certificate (e.g. the signature used their private key) 319 * </p> 320 */ 321 public IDatatype getWho() { 322 return myWho; 323 } 324 325 /** 326 * Sets the value(s) for <b>who[x]</b> () 327 * 328 * <p> 329 * <b>Definition:</b> 330 * A reference to an application-usable description of the person that signed the certificate (e.g. the signature used their private key) 331 * </p> 332 */ 333 public SignatureDt setWho(IDatatype theValue) { 334 myWho = theValue; 335 return this; 336 } 337 338 339 340 341 /** 342 * Gets the value(s) for <b>contentType</b> (). 343 * creating it if it does 344 * not exist. Will not return <code>null</code>. 345 * 346 * <p> 347 * <b>Definition:</b> 348 * A mime type that indicates the technical format of the signature. Important mime types are application/signature+xml for X ML DigSig, application/jwt for JWT, and image/* for a graphical image of a signature 349 * </p> 350 */ 351 public CodeDt getContentTypeElement() { 352 if (myContentType == null) { 353 myContentType = new CodeDt(); 354 } 355 return myContentType; 356 } 357 358 359 /** 360 * Gets the value(s) for <b>contentType</b> (). 361 * creating it if it does 362 * not exist. This method may return <code>null</code>. 363 * 364 * <p> 365 * <b>Definition:</b> 366 * A mime type that indicates the technical format of the signature. Important mime types are application/signature+xml for X ML DigSig, application/jwt for JWT, and image/* for a graphical image of a signature 367 * </p> 368 */ 369 public String getContentType() { 370 return getContentTypeElement().getValue(); 371 } 372 373 /** 374 * Sets the value(s) for <b>contentType</b> () 375 * 376 * <p> 377 * <b>Definition:</b> 378 * A mime type that indicates the technical format of the signature. Important mime types are application/signature+xml for X ML DigSig, application/jwt for JWT, and image/* for a graphical image of a signature 379 * </p> 380 */ 381 public SignatureDt setContentType(CodeDt theValue) { 382 myContentType = theValue; 383 return this; 384 } 385 386 387 388 /** 389 * Sets the value for <b>contentType</b> () 390 * 391 * <p> 392 * <b>Definition:</b> 393 * A mime type that indicates the technical format of the signature. Important mime types are application/signature+xml for X ML DigSig, application/jwt for JWT, and image/* for a graphical image of a signature 394 * </p> 395 */ 396 public SignatureDt setContentType( String theCode) { 397 myContentType = new CodeDt(theCode); 398 return this; 399 } 400 401 402 /** 403 * Gets the value(s) for <b>blob</b> (). 404 * creating it if it does 405 * not exist. Will not return <code>null</code>. 406 * 407 * <p> 408 * <b>Definition:</b> 409 * The base64 encoding of the Signature content 410 * </p> 411 */ 412 public Base64BinaryDt getBlobElement() { 413 if (myBlob == null) { 414 myBlob = new Base64BinaryDt(); 415 } 416 return myBlob; 417 } 418 419 420 /** 421 * Gets the value(s) for <b>blob</b> (). 422 * creating it if it does 423 * not exist. This method may return <code>null</code>. 424 * 425 * <p> 426 * <b>Definition:</b> 427 * The base64 encoding of the Signature content 428 * </p> 429 */ 430 public byte[] getBlob() { 431 return getBlobElement().getValue(); 432 } 433 434 /** 435 * Sets the value(s) for <b>blob</b> () 436 * 437 * <p> 438 * <b>Definition:</b> 439 * The base64 encoding of the Signature content 440 * </p> 441 */ 442 public SignatureDt setBlob(Base64BinaryDt theValue) { 443 myBlob = theValue; 444 return this; 445 } 446 447 448 449 /** 450 * Sets the value for <b>blob</b> () 451 * 452 * <p> 453 * <b>Definition:</b> 454 * The base64 encoding of the Signature content 455 * </p> 456 */ 457 public SignatureDt setBlob( byte[] theBytes) { 458 myBlob = new Base64BinaryDt(theBytes); 459 return this; 460 } 461 462 463 464 465}