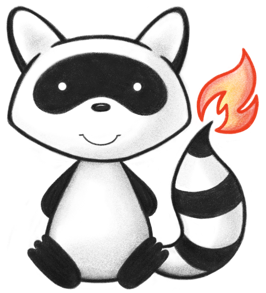
001 002 003 004 005 006 007 008 009 010 011 012 013 014 015 016 017package ca.uhn.fhir.model.dstu2.composite; 018 019import java.net.URI; 020import java.math.BigDecimal; 021import org.apache.commons.lang3.StringUtils; 022import java.util.*; 023import ca.uhn.fhir.model.api.*; 024import ca.uhn.fhir.model.primitive.*; 025import ca.uhn.fhir.model.api.annotation.*; 026import ca.uhn.fhir.model.base.composite.*; 027 028import ca.uhn.fhir.model.dstu2.valueset.AddressTypeEnum; 029import ca.uhn.fhir.model.dstu2.valueset.AddressUseEnum; 030import ca.uhn.fhir.model.dstu2.valueset.AggregationModeEnum; 031import ca.uhn.fhir.model.dstu2.valueset.BindingStrengthEnum; 032import ca.uhn.fhir.model.dstu2.composite.CodeableConceptDt; 033import ca.uhn.fhir.model.dstu2.composite.CodingDt; 034import ca.uhn.fhir.model.dstu2.valueset.ConstraintSeverityEnum; 035import ca.uhn.fhir.model.dstu2.valueset.ContactPointSystemEnum; 036import ca.uhn.fhir.model.dstu2.valueset.ContactPointUseEnum; 037import ca.uhn.fhir.model.dstu2.resource.Device; 038import ca.uhn.fhir.model.dstu2.valueset.EventTimingEnum; 039import ca.uhn.fhir.model.dstu2.valueset.IdentifierTypeCodesEnum; 040import ca.uhn.fhir.model.dstu2.valueset.IdentifierUseEnum; 041import ca.uhn.fhir.model.dstu2.valueset.NameUseEnum; 042import ca.uhn.fhir.model.dstu2.resource.Organization; 043import ca.uhn.fhir.model.dstu2.resource.Patient; 044import ca.uhn.fhir.model.dstu2.composite.PeriodDt; 045import ca.uhn.fhir.model.dstu2.resource.Practitioner; 046import ca.uhn.fhir.model.dstu2.valueset.PropertyRepresentationEnum; 047import ca.uhn.fhir.model.dstu2.valueset.QuantityComparatorEnum; 048import ca.uhn.fhir.model.dstu2.composite.QuantityDt; 049import ca.uhn.fhir.model.dstu2.composite.RangeDt; 050import ca.uhn.fhir.model.dstu2.resource.RelatedPerson; 051import ca.uhn.fhir.model.dstu2.valueset.SignatureTypeCodesEnum; 052import ca.uhn.fhir.model.dstu2.valueset.SlicingRulesEnum; 053import ca.uhn.fhir.model.api.TemporalPrecisionEnum; 054import ca.uhn.fhir.model.dstu2.valueset.TimingAbbreviationEnum; 055import ca.uhn.fhir.model.dstu2.valueset.UnitsOfTimeEnum; 056import ca.uhn.fhir.model.dstu2.resource.ValueSet; 057import ca.uhn.fhir.model.dstu2.composite.BoundCodeableConceptDt; 058import ca.uhn.fhir.model.dstu2.composite.DurationDt; 059import ca.uhn.fhir.model.dstu2.composite.ResourceReferenceDt; 060import ca.uhn.fhir.model.dstu2.composite.SimpleQuantityDt; 061import ca.uhn.fhir.model.primitive.Base64BinaryDt; 062import ca.uhn.fhir.model.primitive.BooleanDt; 063import ca.uhn.fhir.model.primitive.BoundCodeDt; 064import ca.uhn.fhir.model.primitive.CodeDt; 065import ca.uhn.fhir.model.primitive.DateTimeDt; 066import ca.uhn.fhir.model.primitive.DecimalDt; 067import ca.uhn.fhir.model.primitive.IdDt; 068import ca.uhn.fhir.model.primitive.InstantDt; 069import ca.uhn.fhir.model.primitive.IntegerDt; 070import ca.uhn.fhir.model.primitive.MarkdownDt; 071import ca.uhn.fhir.model.primitive.PositiveIntDt; 072import ca.uhn.fhir.model.primitive.StringDt; 073import ca.uhn.fhir.model.primitive.UnsignedIntDt; 074import ca.uhn.fhir.model.primitive.UriDt; 075 076/** 077 * HAPI/FHIR <b>QuantityDt</b> Datatype 078 * () 079 * 080 * <p> 081 * <b>Definition:</b> 082 * A measured amount (or an amount that can potentially be measured). Note that measured amounts include amounts that are not precisely quantified, including amounts involving arbitrary units and floating currencies 083 * </p> 084 * 085 * <p> 086 * <b>Requirements:</b> 087 * Need to able to capture all sorts of measured values, even if the measured value are not precisely quantified. Values include exact measures such as 3.51g, customary units such as 3 tablets, and currencies such as $100.32USD 088 * </p> 089 */ 090@DatatypeDef(name="Quantity") 091public class QuantityDt 092 extends BaseQuantityDt 093 implements ICompositeDatatype 094{ 095 096 /** 097 * Constructor 098 */ 099 public QuantityDt() { 100 // nothing 101 } 102 103 104 /** 105 * Constructor 106 */ 107 @SimpleSetter 108 public QuantityDt(@SimpleSetter.Parameter(name="theValue") double theValue) { 109 setValue(theValue); 110 } 111 112 /** 113 * Constructor 114 */ 115 @SimpleSetter 116 public QuantityDt(@SimpleSetter.Parameter(name="theValue") long theValue) { 117 setValue(theValue); 118 } 119 120 /** 121 * Constructor 122 */ 123 @SimpleSetter 124 public QuantityDt(@SimpleSetter.Parameter(name = "theComparator") QuantityComparatorEnum theComparator, @SimpleSetter.Parameter(name = "theValue") double theValue, 125 @SimpleSetter.Parameter(name = "theUnits") String theUnits) { 126 setValue(theValue); 127 setComparator(theComparator); 128 setUnits(theUnits); 129 } 130 131 /** 132 * Constructor 133 */ 134 @SimpleSetter 135 public QuantityDt(@SimpleSetter.Parameter(name = "theComparator") QuantityComparatorEnum theComparator, @SimpleSetter.Parameter(name = "theValue") long theValue, 136 @SimpleSetter.Parameter(name = "theUnits") String theUnits) { 137 setValue(theValue); 138 setComparator(theComparator); 139 setUnits(theUnits); 140 } 141 142 /** 143 * Constructor 144 */ 145 @SimpleSetter 146 public QuantityDt(@SimpleSetter.Parameter(name="theComparator") QuantityComparatorEnum theComparator, @SimpleSetter.Parameter(name="theValue") double theValue, @SimpleSetter.Parameter(name="theSystem") String theSystem, @SimpleSetter.Parameter(name="theUnits") String theUnits) { 147 setValue(theValue); 148 setComparator(theComparator); 149 setSystem(theSystem); 150 setUnits(theUnits); 151 } 152 153 /** 154 * Constructor 155 */ 156 @SimpleSetter 157 public QuantityDt(@SimpleSetter.Parameter(name="theComparator") QuantityComparatorEnum theComparator, @SimpleSetter.Parameter(name="theValue") long theValue, @SimpleSetter.Parameter(name="theSystem") String theSystem, @SimpleSetter.Parameter(name="theUnits") String theUnits) { 158 setValue(theValue); 159 setComparator(theComparator); 160 setSystem(theSystem); 161 setUnits(theUnits); 162 } 163 164 /** 165 * @deprecated Use {@link #setUnit(String)} instead - Quantity.units was renamed to Quantity.unit in DSTU2 166 */ 167 @Deprecated 168 @Override 169 public BaseQuantityDt setUnits(String theString) { 170 return setUnit(theString); 171 } 172 173 /** 174 * @deprecated Use {@link #getUnitElement()} - Quantity.units was renamed to Quantity.unit in DSTU2 175 */ 176 @Deprecated 177 @Override 178 public StringDt getUnitsElement() { 179 return getUnitElement(); 180 } 181 182 @Child(name="value", type=DecimalDt.class, order=0, min=0, max=1, summary=true, modifier=false) 183 @Description( 184 shortDefinition="", 185 formalDefinition="The value of the measured amount. The value includes an implicit precision in the presentation of the value" 186 ) 187 private DecimalDt myValue; 188 189 @Child(name="comparator", type=CodeDt.class, order=1, min=0, max=1, summary=true, modifier=true) 190 @Description( 191 shortDefinition="", 192 formalDefinition="How the value should be understood and represented - whether the actual value is greater or less than the stated value due to measurement issues; e.g. if the comparator is \"<\" , then the real value is < stated value" 193 ) 194 @ca.uhn.fhir.model.api.annotation.Binding(valueSet = "http://hl7.org/fhir/ValueSet/quantity-comparator") 195 private BoundCodeDt<QuantityComparatorEnum> myComparator; 196 197 @Child(name="unit", type=StringDt.class, order=2, min=0, max=1, summary=true, modifier=false) 198 @Description( 199 shortDefinition="", 200 formalDefinition="A human-readable form of the unit" 201 ) 202 private StringDt myUnit; 203 204 @Child(name="system", type=UriDt.class, order=3, min=0, max=1, summary=true, modifier=false) 205 @Description( 206 shortDefinition="", 207 formalDefinition="The identification of the system that provides the coded form of the unit" 208 ) 209 private UriDt mySystem; 210 211 @Child(name="code", type=CodeDt.class, order=4, min=0, max=1, summary=true, modifier=false) 212 @Description( 213 shortDefinition="", 214 formalDefinition="A computer processable form of the unit in some unit representation system" 215 ) 216 private CodeDt myCode; 217 218 219 @Override 220 public boolean isEmpty() { 221 return super.isBaseEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty( myValue, myComparator, myUnit, mySystem, myCode); 222 } 223 224 @Override 225 public <T extends IElement> List<T> getAllPopulatedChildElementsOfType(Class<T> theType) { 226 return ca.uhn.fhir.util.ElementUtil.allPopulatedChildElements(theType, myValue, myComparator, myUnit, mySystem, myCode); 227 } 228 229 /** 230 * Gets the value(s) for <b>value</b> (). 231 * creating it if it does 232 * not exist. Will not return <code>null</code>. 233 * 234 * <p> 235 * <b>Definition:</b> 236 * The value of the measured amount. The value includes an implicit precision in the presentation of the value 237 * </p> 238 */ 239 public DecimalDt getValueElement() { 240 if (myValue == null) { 241 myValue = new DecimalDt(); 242 } 243 return myValue; 244 } 245 246 247 /** 248 * Gets the value(s) for <b>value</b> (). 249 * creating it if it does 250 * not exist. This method may return <code>null</code>. 251 * 252 * <p> 253 * <b>Definition:</b> 254 * The value of the measured amount. The value includes an implicit precision in the presentation of the value 255 * </p> 256 */ 257 public BigDecimal getValue() { 258 return getValueElement().getValue(); 259 } 260 261 /** 262 * Sets the value(s) for <b>value</b> () 263 * 264 * <p> 265 * <b>Definition:</b> 266 * The value of the measured amount. The value includes an implicit precision in the presentation of the value 267 * </p> 268 */ 269 public QuantityDt setValue(DecimalDt theValue) { 270 myValue = theValue; 271 return this; 272 } 273 274 275 276 /** 277 * Sets the value for <b>value</b> () 278 * 279 * <p> 280 * <b>Definition:</b> 281 * The value of the measured amount. The value includes an implicit precision in the presentation of the value 282 * </p> 283 */ 284 public QuantityDt setValue( long theValue) { 285 myValue = new DecimalDt(theValue); 286 return this; 287 } 288 289 /** 290 * Sets the value for <b>value</b> () 291 * 292 * <p> 293 * <b>Definition:</b> 294 * The value of the measured amount. The value includes an implicit precision in the presentation of the value 295 * </p> 296 */ 297 public QuantityDt setValue( double theValue) { 298 myValue = new DecimalDt(theValue); 299 return this; 300 } 301 302 /** 303 * Sets the value for <b>value</b> () 304 * 305 * <p> 306 * <b>Definition:</b> 307 * The value of the measured amount. The value includes an implicit precision in the presentation of the value 308 * </p> 309 */ 310 public QuantityDt setValue( java.math.BigDecimal theValue) { 311 myValue = new DecimalDt(theValue); 312 return this; 313 } 314 315 316 /** 317 * Gets the value(s) for <b>comparator</b> (). 318 * creating it if it does 319 * not exist. Will not return <code>null</code>. 320 * 321 * <p> 322 * <b>Definition:</b> 323 * How the value should be understood and represented - whether the actual value is greater or less than the stated value due to measurement issues; e.g. if the comparator is \"<\" , then the real value is < stated value 324 * </p> 325 */ 326 public BoundCodeDt<QuantityComparatorEnum> getComparatorElement() { 327 if (myComparator == null) { 328 myComparator = new BoundCodeDt<QuantityComparatorEnum>(QuantityComparatorEnum.VALUESET_BINDER); 329 } 330 return myComparator; 331 } 332 333 334 /** 335 * Gets the value(s) for <b>comparator</b> (). 336 * creating it if it does 337 * not exist. This method may return <code>null</code>. 338 * 339 * <p> 340 * <b>Definition:</b> 341 * How the value should be understood and represented - whether the actual value is greater or less than the stated value due to measurement issues; e.g. if the comparator is \"<\" , then the real value is < stated value 342 * </p> 343 */ 344 public String getComparator() { 345 return getComparatorElement().getValue(); 346 } 347 348 /** 349 * Sets the value(s) for <b>comparator</b> () 350 * 351 * <p> 352 * <b>Definition:</b> 353 * How the value should be understood and represented - whether the actual value is greater or less than the stated value due to measurement issues; e.g. if the comparator is \"<\" , then the real value is < stated value 354 * </p> 355 */ 356 public QuantityDt setComparator(BoundCodeDt<QuantityComparatorEnum> theValue) { 357 myComparator = theValue; 358 return this; 359 } 360 361 362 363 /** 364 * Sets the value(s) for <b>comparator</b> () 365 * 366 * <p> 367 * <b>Definition:</b> 368 * How the value should be understood and represented - whether the actual value is greater or less than the stated value due to measurement issues; e.g. if the comparator is \"<\" , then the real value is < stated value 369 * </p> 370 */ 371 public QuantityDt setComparator(QuantityComparatorEnum theValue) { 372 setComparator(new BoundCodeDt<QuantityComparatorEnum>(QuantityComparatorEnum.VALUESET_BINDER, theValue)); 373 374/* 375 getComparatorElement().setValueAsEnum(theValue); 376*/ 377 return this; 378 } 379 380 381 /** 382 * Gets the value(s) for <b>unit</b> (). 383 * creating it if it does 384 * not exist. Will not return <code>null</code>. 385 * 386 * <p> 387 * <b>Definition:</b> 388 * A human-readable form of the unit 389 * </p> 390 */ 391 public StringDt getUnitElement() { 392 if (myUnit == null) { 393 myUnit = new StringDt(); 394 } 395 return myUnit; 396 } 397 398 399 /** 400 * Gets the value(s) for <b>unit</b> (). 401 * creating it if it does 402 * not exist. This method may return <code>null</code>. 403 * 404 * <p> 405 * <b>Definition:</b> 406 * A human-readable form of the unit 407 * </p> 408 */ 409 public String getUnit() { 410 return getUnitElement().getValue(); 411 } 412 413 /** 414 * Sets the value(s) for <b>unit</b> () 415 * 416 * <p> 417 * <b>Definition:</b> 418 * A human-readable form of the unit 419 * </p> 420 */ 421 public QuantityDt setUnit(StringDt theValue) { 422 myUnit = theValue; 423 return this; 424 } 425 426 427 428 /** 429 * Sets the value for <b>unit</b> () 430 * 431 * <p> 432 * <b>Definition:</b> 433 * A human-readable form of the unit 434 * </p> 435 */ 436 public QuantityDt setUnit( String theString) { 437 myUnit = new StringDt(theString); 438 return this; 439 } 440 441 442 /** 443 * Gets the value(s) for <b>system</b> (). 444 * creating it if it does 445 * not exist. Will not return <code>null</code>. 446 * 447 * <p> 448 * <b>Definition:</b> 449 * The identification of the system that provides the coded form of the unit 450 * </p> 451 */ 452 public UriDt getSystemElement() { 453 if (mySystem == null) { 454 mySystem = new UriDt(); 455 } 456 return mySystem; 457 } 458 459 460 /** 461 * Gets the value(s) for <b>system</b> (). 462 * creating it if it does 463 * not exist. This method may return <code>null</code>. 464 * 465 * <p> 466 * <b>Definition:</b> 467 * The identification of the system that provides the coded form of the unit 468 * </p> 469 */ 470 public String getSystem() { 471 return getSystemElement().getValue(); 472 } 473 474 /** 475 * Sets the value(s) for <b>system</b> () 476 * 477 * <p> 478 * <b>Definition:</b> 479 * The identification of the system that provides the coded form of the unit 480 * </p> 481 */ 482 public QuantityDt setSystem(UriDt theValue) { 483 mySystem = theValue; 484 return this; 485 } 486 487 488 489 /** 490 * Sets the value for <b>system</b> () 491 * 492 * <p> 493 * <b>Definition:</b> 494 * The identification of the system that provides the coded form of the unit 495 * </p> 496 */ 497 public QuantityDt setSystem( String theUri) { 498 mySystem = new UriDt(theUri); 499 return this; 500 } 501 502 503 /** 504 * Gets the value(s) for <b>code</b> (). 505 * creating it if it does 506 * not exist. Will not return <code>null</code>. 507 * 508 * <p> 509 * <b>Definition:</b> 510 * A computer processable form of the unit in some unit representation system 511 * </p> 512 */ 513 public CodeDt getCodeElement() { 514 if (myCode == null) { 515 myCode = new CodeDt(); 516 } 517 return myCode; 518 } 519 520 521 /** 522 * Gets the value(s) for <b>code</b> (). 523 * creating it if it does 524 * not exist. This method may return <code>null</code>. 525 * 526 * <p> 527 * <b>Definition:</b> 528 * A computer processable form of the unit in some unit representation system 529 * </p> 530 */ 531 public String getCode() { 532 return getCodeElement().getValue(); 533 } 534 535 /** 536 * Sets the value(s) for <b>code</b> () 537 * 538 * <p> 539 * <b>Definition:</b> 540 * A computer processable form of the unit in some unit representation system 541 * </p> 542 */ 543 public QuantityDt setCode(CodeDt theValue) { 544 myCode = theValue; 545 return this; 546 } 547 548 549 550 /** 551 * Sets the value for <b>code</b> () 552 * 553 * <p> 554 * <b>Definition:</b> 555 * A computer processable form of the unit in some unit representation system 556 * </p> 557 */ 558 public QuantityDt setCode( String theCode) { 559 myCode = new CodeDt(theCode); 560 return this; 561 } 562 563 564 565 566}