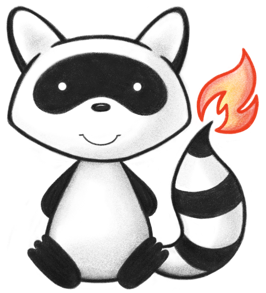
001/*- 002 * #%L 003 * HAPI FHIR - Server Framework 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.server.mail; 021 022import org.apache.commons.lang3.StringUtils; 023import org.apache.commons.lang3.builder.EqualsBuilder; 024import org.apache.commons.lang3.builder.HashCodeBuilder; 025import org.apache.commons.lang3.builder.ToStringBuilder; 026 027public class MailConfig { 028 private String mySmtpHostname; 029 private Integer mySmtpPort; 030 private String mySmtpUsername; 031 private String mySmtpPassword; 032 private boolean mySmtpUseStartTLS; 033 034 public MailConfig() {} 035 036 public String getSmtpHostname() { 037 return mySmtpHostname; 038 } 039 040 public MailConfig setSmtpHostname(String theSmtpHostname) { 041 mySmtpHostname = theSmtpHostname; 042 return this; 043 } 044 045 public Integer getSmtpPort() { 046 return mySmtpPort; 047 } 048 049 public MailConfig setSmtpPort(Integer theSmtpPort) { 050 mySmtpPort = theSmtpPort; 051 return this; 052 } 053 054 public String getSmtpUsername() { 055 return mySmtpUsername; 056 } 057 058 public MailConfig setSmtpUsername(String theSmtpUsername) { 059 // SimpleJavaMail treats empty smtp username as valid username and requires auth 060 mySmtpUsername = StringUtils.isBlank(theSmtpUsername) ? null : theSmtpUsername; 061 return this; 062 } 063 064 public String getSmtpPassword() { 065 return mySmtpPassword; 066 } 067 068 public MailConfig setSmtpPassword(String theSmtpPassword) { 069 // SimpleJavaMail treats empty smtp password as valid password and requires auth 070 mySmtpPassword = StringUtils.isBlank(theSmtpPassword) ? null : theSmtpPassword; 071 return this; 072 } 073 074 public boolean isSmtpUseStartTLS() { 075 return mySmtpUseStartTLS; 076 } 077 078 public MailConfig setSmtpUseStartTLS(boolean theSmtpUseStartTLS) { 079 mySmtpUseStartTLS = theSmtpUseStartTLS; 080 return this; 081 } 082 083 @Override 084 public boolean equals(Object object) { 085 if (this == object) { 086 return true; 087 } 088 if (object == null || getClass() != object.getClass()) { 089 return false; 090 } 091 return EqualsBuilder.reflectionEquals(this, object); 092 } 093 094 @Override 095 public int hashCode() { 096 return HashCodeBuilder.reflectionHashCode(this); 097 } 098 099 @Override 100 public String toString() { 101 return ToStringBuilder.reflectionToString(this); 102 } 103}