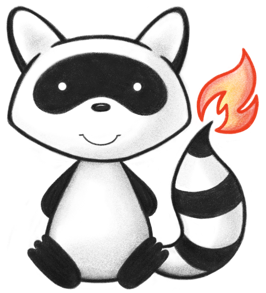
001/* 002 * #%L 003 * HAPI FHIR - Server Framework 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.server.interceptor.auth; 021 022public interface IAuthRuleBuilderRule { 023 024 /** 025 * This rule applies to <code>create</code> operations with a <code>conditional</code> 026 * URL as a part of the request. Note that this rule will allow the conditional 027 * operation to proceed, but the server is expected to determine the actual target 028 * of the conditional request and send a subsequent event to the {@link AuthorizationInterceptor} 029 * in order to authorize the actual target. 030 * <p> 031 * In other words, if the server is configured correctly, this chain will allow the 032 * client to perform a conditional update, but a different rule is required to actually 033 * authorize the target that the conditional update is determined to match. 034 * </p> 035 */ 036 IAuthRuleBuilderRuleConditional createConditional(); 037 038 /** 039 * This rule applies to the FHIR delete operation 040 */ 041 IAuthRuleBuilderRuleOpDelete delete(); 042 043 /** 044 * This rule applies to <code>create</code> operations with a <code>conditional</code> 045 * URL as a part of the request. Note that this rule will allow the conditional 046 * operation to proceed, but the server is expected to determine the actual target 047 * of the conditional request and send a subsequent event to the {@link AuthorizationInterceptor} 048 * in order to authorize the actual target. 049 * <p> 050 * In other words, if the server is configured correctly, this chain will allow the 051 * client to perform a conditional update, but a different rule is required to actually 052 * authorize the target that the conditional update is determined to match. 053 * </p> 054 */ 055 IAuthRuleBuilderRuleConditional deleteConditional(); 056 057 /** 058 * This rules applies to the metadata operation (retrieve the 059 * server's conformance statement) 060 * <p> 061 * This call completes the rule and adds the rule to the chain. 062 * </p> 063 */ 064 IAuthRuleBuilderRuleOpClassifierFinished metadata(); 065 066 /** 067 * This rule applies to a FHIR operation (e.g. <code>$validate</code>) 068 */ 069 IAuthRuleBuilderOperation operation(); 070 071 /** 072 * This rule applies to a FHIR patch operation 073 */ 074 IAuthRuleBuilderPatch patch(); 075 076 /** 077 * This rule applies to any FHIR operation involving reading, including 078 * <code>read</code>, <code>vread</code>, <code>search</code>, and 079 * <code>history</code> 080 */ 081 IAuthRuleBuilderRuleOp read(); 082 083 /** 084 * This rule applies to the FHIR transaction operation. Transaction is a special 085 * case in that it bundles other operations. This permission also allows FHIR 086 * batch to be performed. 087 */ 088 IAuthRuleBuilderRuleTransaction transaction(); 089 090 /** 091 * This rule applies to <code>update</code> operations with a <code>conditional</code> 092 * URL as a part of the request. Note that this rule will allow the conditional 093 * operation to proceed, but the server is expected to determine the actual target 094 * of the conditional request and send a subsequent event to the {@link AuthorizationInterceptor} 095 * in order to authorize the actual target. 096 * <p> 097 * In other words, if the server is configured correctly, this chain will allow the 098 * client to perform a conditional update, but a different rule is required to actually 099 * authorize the target that the conditional update is determined to match. 100 * </p> 101 */ 102 IAuthRuleBuilderRuleConditional updateConditional(); 103 104 /** 105 * This rule applies to any FHIR operation involving writing, including 106 * <code>create</code>, and <code>update</code> 107 */ 108 IAuthRuleBuilderRuleOp write(); 109 110 /** 111 * This rule specifically allows a user to perform a FHIR create, but not an update or other write operations 112 * 113 * @see #write() 114 * @since 4.1.0 115 */ 116 IAuthRuleBuilderRuleOp create(); 117 118 /** 119 * Allow a GraphQL query 120 */ 121 IAuthRuleBuilderGraphQL graphQL(); 122 123 /** 124 * This rule permits the user to initiate a FHIR bulk export 125 * 126 * @since 5.5.0 127 */ 128 IAuthRuleBuilderRuleBulkExport bulkExport(); 129 130 /** 131 * This rule specifically allows a user to perform a FHIR update on the historical version of a resource 132 * 133 * @since 6.1.0 134 */ 135 IAuthRuleBuilderUpdateHistoryRewrite updateHistoryRewrite(); 136}