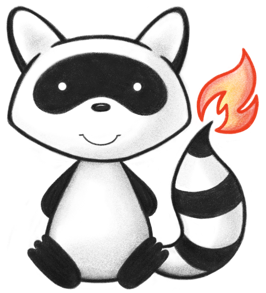
Interface IServerInterceptor
- All Known Subinterfaces:
IServerOperationInterceptor
- All Known Implementing Classes:
BanUnsupportedHttpMethodsInterceptor
,CorsInterceptor
,InterceptorAdapter
,ServerOperationInterceptorAdapter
,VerboseLoggingInterceptor
InterceptorAdapter
in order to not need to implement every method.
See: See the server interceptor documentation for more information on how to use this class.
Note that unless otherwise stated, it is possible to throw any subclass ofBaseServerResponseException
from any interceptor method.-
Method Summary
Modifier and TypeMethodDescriptionboolean
handleException
(RequestDetails theRequestDetails, ca.uhn.fhir.rest.server.exceptions.BaseServerResponseException theException, jakarta.servlet.http.HttpServletRequest theServletRequest, jakarta.servlet.http.HttpServletResponse theServletResponse) This method is called upon any exception being thrown within the server's request processing code.boolean
incomingRequestPostProcessed
(RequestDetails theRequestDetails, jakarta.servlet.http.HttpServletRequest theRequest, jakarta.servlet.http.HttpServletResponse theResponse) This method is called just before the actual implementing server method is invoked.void
incomingRequestPreHandled
(ca.uhn.fhir.rest.api.RestOperationTypeEnum theOperation, RequestDetails theProcessedRequest) Invoked before an incoming request is processed.boolean
incomingRequestPreProcessed
(jakarta.servlet.http.HttpServletRequest theRequest, jakarta.servlet.http.HttpServletResponse theResponse) This method is called before any other processing takes place for each incoming request.boolean
outgoingResponse
(RequestDetails theRequestDetails) Deprecated.As of HAPI FHIR 3.2.0, this method is deprecated and will be removed in a future version of HAPI FHIR.boolean
outgoingResponse
(RequestDetails theRequestDetails, ca.uhn.fhir.model.api.TagList theResponseObject) Deprecated.As of HAPI FHIR 3.2.0, this method is deprecated and will be removed in a future version of HAPI FHIR.boolean
outgoingResponse
(RequestDetails theRequestDetails, ca.uhn.fhir.model.api.TagList theResponseObject, jakarta.servlet.http.HttpServletRequest theServletRequest, jakarta.servlet.http.HttpServletResponse theServletResponse) Deprecated.As of HAPI FHIR 3.2.0, this method is deprecated and will be removed in a future version of HAPI FHIR.boolean
outgoingResponse
(RequestDetails theRequestDetails, ResponseDetails theResponseDetails, jakarta.servlet.http.HttpServletRequest theServletRequest, jakarta.servlet.http.HttpServletResponse theServletResponse) This method is called after the server implementation method has been called, but before any attempt to stream the response back to the client.boolean
outgoingResponse
(RequestDetails theRequestDetails, jakarta.servlet.http.HttpServletRequest theServletRequest, jakarta.servlet.http.HttpServletResponse theServletResponse) Deprecated.As of HAPI FHIR 3.2.0, this method is deprecated and will be removed in a future version of HAPI FHIR.boolean
outgoingResponse
(RequestDetails theRequestDetails, org.hl7.fhir.instance.model.api.IBaseResource theResponseObject) Deprecated.As of HAPI FHIR 3.2.0, this method is deprecated and will be removed in a future version of HAPI FHIR.boolean
outgoingResponse
(RequestDetails theRequestDetails, org.hl7.fhir.instance.model.api.IBaseResource theResponseObject, jakarta.servlet.http.HttpServletRequest theServletRequest, jakarta.servlet.http.HttpServletResponse theServletResponse) Deprecated.As of HAPI FHIR 3.3.0, this method has been deprecated in favour ofoutgoingResponse(RequestDetails, ResponseDetails, HttpServletRequest, HttpServletResponse)
and will be removed in a future version of HAPI FHIR.ca.uhn.fhir.rest.server.exceptions.BaseServerResponseException
preProcessOutgoingException
(RequestDetails theRequestDetails, Throwable theException, jakarta.servlet.http.HttpServletRequest theServletRequest) This method is called upon any exception being thrown within the server's request processing code.void
processingCompletedNormally
(ServletRequestDetails theRequestDetails) This method is called after all processing is completed for a request, but only if the request completes normally (i.e.
-
Method Details
-
handleException
boolean handleException(RequestDetails theRequestDetails, ca.uhn.fhir.rest.server.exceptions.BaseServerResponseException theException, jakarta.servlet.http.HttpServletRequest theServletRequest, jakarta.servlet.http.HttpServletResponse theServletResponse) throws jakarta.servlet.ServletException, IOException This method is called upon any exception being thrown within the server's request processing code. This includes any exceptions thrown within resource provider methods (e.g.Search
andRead
methods) as well as any runtime exceptions thrown by the server itself. This also includes anyAuthenticationException
s thrown.Implementations of this method may choose to ignore/log/count/etc exceptions, and return
true
. In this case, processing will continue, and the server will automatically generate anOperationOutcome
. Implementations may also choose to provide their own response to the client. In this case, they should returnfalse
, to indicate that they have handled the request and processing should stop.- Parameters:
theRequestDetails
- A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of theservlet request
. Note that the bean properties are not all guaranteed to be populated, depending on how early during processing the exception occurred.theServletRequest
- The incoming requesttheServletResponse
- The response. Note that interceptors may choose to provide a response (i.e. by callingServletResponse.getWriter()
) but in that case it is important to returnfalse
to indicate that the server itself should not also provide a response.- Returns:
- Return
true
if processing should continue normally. This is generally the right thing to do. If your interceptor is providing a response rather than letting HAPI handle the response normally, you must returnfalse
. In this case, no further processing will occur and no further interceptors will be called. - Throws:
jakarta.servlet.ServletException
- If this exception is thrown, it will be re-thrown up to the container for handling.IOException
- If this exception is thrown, it will be re-thrown up to the container for handling.
-
incomingRequestPostProcessed
boolean incomingRequestPostProcessed(RequestDetails theRequestDetails, jakarta.servlet.http.HttpServletRequest theRequest, jakarta.servlet.http.HttpServletResponse theResponse) throws ca.uhn.fhir.rest.server.exceptions.AuthenticationException This method is called just before the actual implementing server method is invoked.- Parameters:
theRequestDetails
- A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of theservlet request
.theRequest
- The incoming requesttheResponse
- The response. Note that interceptors may choose to provide a response (i.e. by callingServletResponse.getWriter()
) but in that case it is important to returnfalse
to indicate that the server itself should not also provide a response.- Returns:
- Return
true
if processing should continue normally. This is generally the right thing to do. If your interceptor is providing a response rather than letting HAPI handle the response normally, you must returnfalse
. In this case, no further processing will occur and no further interceptors will be called. - Throws:
ca.uhn.fhir.rest.server.exceptions.AuthenticationException
- This exception may be thrown to indicate that the interceptor has detected an unauthorized access attempt. If thrown, processing will stop and an HTTP 401 will be returned to the client.
-
incomingRequestPreHandled
void incomingRequestPreHandled(ca.uhn.fhir.rest.api.RestOperationTypeEnum theOperation, RequestDetails theProcessedRequest) Invoked before an incoming request is processed. Note that this method is called after the server has begin preparing the response to the incoming client request. As such, it is not able to supply a response to the incoming request in the way thatincomingRequestPostProcessed(RequestDetails, HttpServletRequest, HttpServletResponse)
are.This method may however throw a subclass of
BaseServerResponseException
, and processing will be aborted with an appropriate error returned to the client.- Parameters:
theOperation
- The type of operation that the FHIR server has determined that the client is trying to invoketheProcessedRequest
- An object which will be populated with the details which were extracted from the raw request by the server, e.g. the FHIR operation type and the parsed resource body (if any).
-
incomingRequestPreProcessed
boolean incomingRequestPreProcessed(jakarta.servlet.http.HttpServletRequest theRequest, jakarta.servlet.http.HttpServletResponse theResponse) This method is called before any other processing takes place for each incoming request. It may be used to provide alternate handling for some requests, or to screen requests before they are handled, etc.Note that any exceptions thrown by this method will not be trapped by HAPI (they will be passed up to the server)
- Parameters:
theRequest
- The incoming requesttheResponse
- The response. Note that interceptors may choose to provide a response (i.e. by callingServletResponse.getWriter()
) but in that case it is important to returnfalse
to indicate that the server itself should not also provide a response.- Returns:
- Return
true
if processing should continue normally. This is generally the right thing to do. If your interceptor is providing a response rather than letting HAPI handle the response normally, you must returnfalse
. In this case, no further processing will occur and no further interceptors will be called.
-
outgoingResponse
Deprecated.As of HAPI FHIR 3.2.0, this method is deprecated and will be removed in a future version of HAPI FHIR. -
outgoingResponse
@Deprecated boolean outgoingResponse(RequestDetails theRequestDetails, jakarta.servlet.http.HttpServletRequest theServletRequest, jakarta.servlet.http.HttpServletResponse theServletResponse) throws ca.uhn.fhir.rest.server.exceptions.AuthenticationException Deprecated.As of HAPI FHIR 3.2.0, this method is deprecated and will be removed in a future version of HAPI FHIR.UseoutgoingResponse(RequestDetails, IBaseResource, HttpServletRequest, HttpServletResponse)
instead- Throws:
ca.uhn.fhir.rest.server.exceptions.AuthenticationException
-
outgoingResponse
@Deprecated boolean outgoingResponse(RequestDetails theRequestDetails, org.hl7.fhir.instance.model.api.IBaseResource theResponseObject) Deprecated.As of HAPI FHIR 3.2.0, this method is deprecated and will be removed in a future version of HAPI FHIR. -
outgoingResponse
@Deprecated boolean outgoingResponse(RequestDetails theRequestDetails, org.hl7.fhir.instance.model.api.IBaseResource theResponseObject, jakarta.servlet.http.HttpServletRequest theServletRequest, jakarta.servlet.http.HttpServletResponse theServletResponse) throws ca.uhn.fhir.rest.server.exceptions.AuthenticationException Deprecated.As of HAPI FHIR 3.3.0, this method has been deprecated in favour ofoutgoingResponse(RequestDetails, ResponseDetails, HttpServletRequest, HttpServletResponse)
and will be removed in a future version of HAPI FHIR.This method is called after the server implementation method has been called, but before any attempt to stream the response back to the client.- Parameters:
theRequestDetails
- A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of theservlet request
.theResponseObject
- The actual object which is being streamed to the client as a response. This may benull
if the response does not include a resource.theServletRequest
- The incoming requesttheServletResponse
- The response. Note that interceptors may choose to provide a response (i.e. by callingServletResponse.getWriter()
) but in that case it is important to returnfalse
to indicate that the server itself should not also provide a response.- Returns:
- Return
true
if processing should continue normally. This is generally the right thing to do. If your interceptor is providing a response rather than letting HAPI handle the response normally, you must returnfalse
. In this case, no further processing will occur and no further interceptors will be called. - Throws:
ca.uhn.fhir.rest.server.exceptions.AuthenticationException
- This exception may be thrown to indicate that the interceptor has detected an unauthorized access attempt. If thrown, processing will stop and an HTTP 401 will be returned to the client.
-
outgoingResponse
boolean outgoingResponse(RequestDetails theRequestDetails, ResponseDetails theResponseDetails, jakarta.servlet.http.HttpServletRequest theServletRequest, jakarta.servlet.http.HttpServletResponse theServletResponse) throws ca.uhn.fhir.rest.server.exceptions.AuthenticationException This method is called after the server implementation method has been called, but before any attempt to stream the response back to the client.- Parameters:
theRequestDetails
- A bean containing details about the request that is about to be processed, including details such as the resource type and logical ID (if any) and other FHIR-specific aspects of the request which have been pulled out of theservlet request
.theResponseDetails
- This object contains details about the response, including the actual payload that will be returnedtheServletRequest
- The incoming requesttheServletResponse
- The response. Note that interceptors may choose to provide a response (i.e. by callingServletResponse.getWriter()
) but in that case it is important to returnfalse
to indicate that the server itself should not also provide a response.- Returns:
- Return
true
if processing should continue normally. This is generally the right thing to do. If your interceptor is providing a response rather than letting HAPI handle the response normally, you must returnfalse
. In this case, no further processing will occur and no further interceptors will be called. - Throws:
ca.uhn.fhir.rest.server.exceptions.AuthenticationException
- This exception may be thrown to indicate that the interceptor has detected an unauthorized access attempt. If thrown, processing will stop and an HTTP 401 will be returned to the client.
-
outgoingResponse
@Deprecated boolean outgoingResponse(RequestDetails theRequestDetails, ca.uhn.fhir.model.api.TagList theResponseObject) Deprecated.As of HAPI FHIR 3.2.0, this method is deprecated and will be removed in a future version of HAPI FHIR. -
outgoingResponse
@Deprecated boolean outgoingResponse(RequestDetails theRequestDetails, ca.uhn.fhir.model.api.TagList theResponseObject, jakarta.servlet.http.HttpServletRequest theServletRequest, jakarta.servlet.http.HttpServletResponse theServletResponse) throws ca.uhn.fhir.rest.server.exceptions.AuthenticationException Deprecated.As of HAPI FHIR 3.2.0, this method is deprecated and will be removed in a future version of HAPI FHIR.UseoutgoingResponse(RequestDetails, IBaseResource, HttpServletRequest, HttpServletResponse)
instead- Throws:
ca.uhn.fhir.rest.server.exceptions.AuthenticationException
-
preProcessOutgoingException
ca.uhn.fhir.rest.server.exceptions.BaseServerResponseException preProcessOutgoingException(RequestDetails theRequestDetails, Throwable theException, jakarta.servlet.http.HttpServletRequest theServletRequest) throws jakarta.servlet.ServletException This method is called upon any exception being thrown within the server's request processing code. This includes any exceptions thrown within resource provider methods (e.g.Search
andRead
methods) as well as any runtime exceptions thrown by the server itself. This method is invoked for each interceptor (until one of them returns a non-null
response or the end of the list is reached), after whichhandleException(RequestDetails, BaseServerResponseException, HttpServletRequest, HttpServletResponse)
is called for each interceptor.This may be used to add an OperationOutcome to a response, or to convert between exception types for any reason.
Implementations of this method may choose to ignore/log/count/etc exceptions, and return
null
. In this case, processing will continue, and the server will automatically generate anOperationOutcome
. Implementations may also choose to provide their own response to the client. In this case, they should return a non-null
, to indicate that they have handled the request and processing should stop.- Returns:
- Returns the new exception to use for processing, or
null
if this interceptor is not trying to modify the exception. For example, if this interceptor has nothing to do with exception processing, it should always returnnull
. If this interceptor adds an OperationOutcome to the exception, it should return an exception. - Throws:
jakarta.servlet.ServletException
-
processingCompletedNormally
This method is called after all processing is completed for a request, but only if the request completes normally (i.e. no exception is thrown).This method should not throw any exceptions. Any exception that is thrown by this method will be logged, but otherwise not acted upon.
Note that this individual interceptors will have this method called in the reverse order from the order in which the interceptors were registered with the server.
- Parameters:
theRequestDetails
- The request itself
-