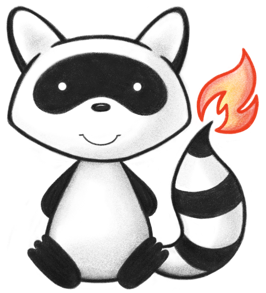
001/*- 002 * #%L 003 * HAPI FHIR Subscription Server 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.topic.filter; 021 022import ca.uhn.fhir.jpa.subscription.model.CanonicalTopicSubscription; 023import ca.uhn.fhir.jpa.subscription.model.CanonicalTopicSubscriptionFilter; 024import ca.uhn.fhir.util.Logs; 025import jakarta.annotation.Nonnull; 026import org.hl7.fhir.instance.model.api.IBaseResource; 027import org.slf4j.Logger; 028 029public final class SubscriptionTopicFilterUtil { 030 private static final Logger ourLog = Logs.getSubscriptionTopicLog(); 031 032 private SubscriptionTopicFilterUtil() {} 033 034 public static boolean matchFilters( 035 @Nonnull IBaseResource theResource, 036 @Nonnull String theResourceType, 037 @Nonnull ISubscriptionTopicFilterMatcher theSubscriptionTopicFilterMatcher, 038 @Nonnull CanonicalTopicSubscription topicSubscription) { 039 boolean match = true; 040 for (CanonicalTopicSubscriptionFilter filter : topicSubscription.getFilters()) { 041 if (filter.getResourceType() == null 042 || "Resource".equals(filter.getResourceType()) 043 || !filter.getResourceType().equals(theResourceType)) { 044 continue; 045 } 046 if (!theSubscriptionTopicFilterMatcher.match(filter, theResource).matched()) { 047 match = false; 048 ourLog.debug( 049 "Resource {} did not match filter {}. Skipping remaining filters.", 050 theResource.getIdElement().toUnqualifiedVersionless().getValue(), 051 filter.asCriteriaString()); 052 break; 053 } 054 ourLog.debug( 055 "Resource {} matches filter {}", 056 theResource.getIdElement().toUnqualifiedVersionless().getValue(), 057 filter.asCriteriaString()); 058 } 059 return match; 060 } 061}