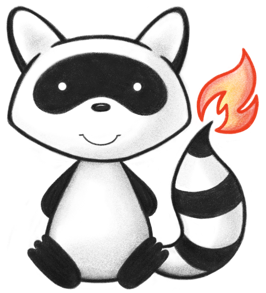
001/*- 002 * #%L 003 * HAPI FHIR Subscription Server 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.topic; 021 022import org.hl7.fhir.r5.model.SubscriptionTopic; 023 024import java.util.Collection; 025import java.util.HashSet; 026import java.util.Map; 027import java.util.Set; 028import java.util.concurrent.ConcurrentHashMap; 029 030public class ActiveSubscriptionTopicCache { 031 // We canonicalize on R5 SubscriptionTopic and convert back to R4B when necessary 032 private final Map<String, SubscriptionTopic> myCache = new ConcurrentHashMap<>(); 033 034 public int size() { 035 return myCache.size(); 036 } 037 038 /** 039 * @return true if the subscription topic was added, false if it was already present 040 */ 041 public boolean add(SubscriptionTopic theSubscriptionTopic) { 042 String key = theSubscriptionTopic.getIdElement().getIdPart(); 043 SubscriptionTopic previousValue = myCache.put(key, theSubscriptionTopic); 044 return previousValue == null; 045 } 046 047 /** 048 * @return the number of entries removed 049 */ 050 public int removeIdsNotInCollection(Set<String> theIdsToRetain) { 051 int retval = 0; 052 HashSet<String> safeCopy = new HashSet<>(myCache.keySet()); 053 054 for (String next : safeCopy) { 055 if (!theIdsToRetain.contains(next)) { 056 myCache.remove(next); 057 ++retval; 058 } 059 } 060 return retval; 061 } 062 063 public Collection<SubscriptionTopic> getAll() { 064 return myCache.values(); 065 } 066 067 public void remove(String theSubscriptionTopicId) { 068 myCache.remove(theSubscriptionTopicId); 069 } 070}