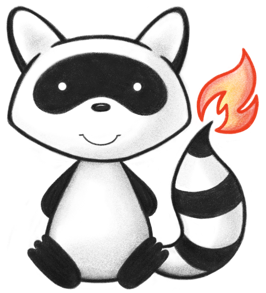
001/*- 002 * #%L 003 * HAPI FHIR JPA Model 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.model.entity; 021 022import jakarta.persistence.Column; 023import jakarta.persistence.Entity; 024import jakarta.persistence.Id; 025import jakarta.persistence.Index; 026import jakarta.persistence.Table; 027import jakarta.persistence.Temporal; 028import jakarta.persistence.TemporalType; 029 030import java.util.Date; 031 032/** 033 * This entity is used to enforce uniqueness on a given search URL being 034 * used as a conditional operation URL, e.g. a conditional create or a 035 * conditional update. When we perform a conditional operation that is 036 * creating a new resource, we store an entity with the conditional URL 037 * in this table. The URL is the PK of the table, so the database 038 * ensures that two concurrent threads don't accidentally create two 039 * resources with the same conditional URL. 040 */ 041@Entity 042@Table( 043 name = "HFJ_RES_SEARCH_URL", 044 indexes = { 045 @Index(name = "IDX_RESSEARCHURL_RES", columnList = "RES_ID"), 046 @Index(name = "IDX_RESSEARCHURL_TIME", columnList = "CREATED_TIME") 047 }) 048public class ResourceSearchUrlEntity { 049 050 public static final String RES_SEARCH_URL_COLUMN_NAME = "RES_SEARCH_URL"; 051 052 public static final int RES_SEARCH_URL_LENGTH = 768; 053 054 @Id 055 @Column(name = RES_SEARCH_URL_COLUMN_NAME, length = RES_SEARCH_URL_LENGTH, nullable = false) 056 private String mySearchUrl; 057 058 @Column(name = "RES_ID", updatable = false, nullable = false) 059 private Long myResourcePid; 060 061 @Column(name = "CREATED_TIME", nullable = false) 062 @Temporal(TemporalType.TIMESTAMP) 063 private Date myCreatedTime; 064 065 public static ResourceSearchUrlEntity from(String theUrl, Long theId) { 066 return new ResourceSearchUrlEntity() 067 .setResourcePid(theId) 068 .setSearchUrl(theUrl) 069 .setCreatedTime(new Date()); 070 } 071 072 public Long getResourcePid() { 073 return myResourcePid; 074 } 075 076 public ResourceSearchUrlEntity setResourcePid(Long theResourcePid) { 077 myResourcePid = theResourcePid; 078 return this; 079 } 080 081 public Date getCreatedTime() { 082 return myCreatedTime; 083 } 084 085 public ResourceSearchUrlEntity setCreatedTime(Date theCreatedTime) { 086 myCreatedTime = theCreatedTime; 087 return this; 088 } 089 090 public String getSearchUrl() { 091 return mySearchUrl; 092 } 093 094 public ResourceSearchUrlEntity setSearchUrl(String theSearchUrl) { 095 mySearchUrl = theSearchUrl; 096 return this; 097 } 098}