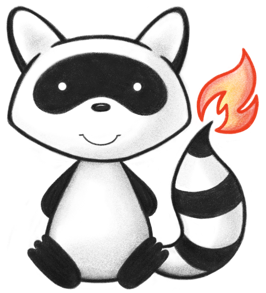
001package ca.uhn.fhir.jpa.model.entity; 002 003/*- 004 * #%L 005 * HAPI FHIR JPA Model 006 * %% 007 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 008 * %% 009 * Licensed under the Apache License, Version 2.0 (the "License"); 010 * you may not use this file except in compliance with the License. 011 * You may obtain a copy of the License at 012 * 013 * http://www.apache.org/licenses/LICENSE-2.0 014 * 015 * Unless required by applicable law or agreed to in writing, software 016 * distributed under the License is distributed on an "AS IS" BASIS, 017 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 018 * See the License for the specific language governing permissions and 019 * limitations under the License. 020 * #L% 021 */ 022 023import jakarta.persistence.Column; 024import jakarta.persistence.EmbeddedId; 025import jakarta.persistence.Entity; 026import jakarta.persistence.Table; 027import jakarta.persistence.Temporal; 028import jakarta.persistence.TemporalType; 029 030import java.io.Serializable; 031import java.util.Date; 032 033/** 034 * This class describes how a resourceModifiedMessage is stored for later processing in the event where 035 * submission to the subscription processing pipeline would fail. The persisted message does not include a 036 * payload (resource) as an in-memory version of the same message would. Instead, it points to a payload 037 * through the entity primary key {@link PersistedResourceModifiedMessageEntityPK} which is composed 038 * of the resource Pid and current version. 039 */ 040@Entity 041@Table(name = "HFJ_RESOURCE_MODIFIED") 042public class ResourceModifiedEntity implements IPersistedResourceModifiedMessage, Serializable { 043 044 public static final int MESSAGE_LENGTH = 4000; 045 046 @EmbeddedId 047 private PersistedResourceModifiedMessageEntityPK myResourceModifiedEntityPK; 048 049 @Column(name = "SUMMARY_MESSAGE", length = MESSAGE_LENGTH, nullable = false) 050 private String mySummaryResourceModifiedMessage; 051 052 @Column(name = "CREATED_TIME", nullable = false) 053 @Temporal(TemporalType.TIMESTAMP) 054 private Date myCreatedTime; 055 056 @Column(name = "RESOURCE_TYPE", length = ResourceTable.RESTYPE_LEN, nullable = false) 057 private String myResourceType; 058 059 public PersistedResourceModifiedMessageEntityPK getResourceModifiedEntityPK() { 060 return myResourceModifiedEntityPK; 061 } 062 063 public ResourceModifiedEntity setResourceModifiedEntityPK( 064 PersistedResourceModifiedMessageEntityPK theResourceModifiedEntityPK) { 065 myResourceModifiedEntityPK = theResourceModifiedEntityPK; 066 return this; 067 } 068 069 @Override 070 public String getResourceType() { 071 return myResourceType; 072 } 073 074 public ResourceModifiedEntity setResourceType(String theResourceType) { 075 myResourceType = theResourceType; 076 return this; 077 } 078 079 public Date getCreatedTime() { 080 return myCreatedTime; 081 } 082 083 public void setCreatedTime(Date theCreatedTime) { 084 myCreatedTime = theCreatedTime; 085 } 086 087 public String getSummaryResourceModifiedMessage() { 088 return mySummaryResourceModifiedMessage; 089 } 090 091 public ResourceModifiedEntity setSummaryResourceModifiedMessage(String theSummaryResourceModifiedMessage) { 092 mySummaryResourceModifiedMessage = theSummaryResourceModifiedMessage; 093 return this; 094 } 095 096 @Override 097 public IPersistedResourceModifiedMessagePK getPersistedResourceModifiedMessagePk() { 098 return myResourceModifiedEntityPK; 099 } 100}