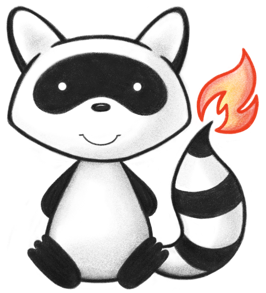
001/* 002 * #%L 003 * HAPI FHIR JPA Model 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.model.entity; 021 022import jakarta.persistence.Column; 023import jakarta.persistence.Embeddable; 024import jakarta.persistence.Entity; 025import jakarta.persistence.ForeignKey; 026import jakarta.persistence.GeneratedValue; 027import jakarta.persistence.GenerationType; 028import jakarta.persistence.Id; 029import jakarta.persistence.Index; 030import jakarta.persistence.JoinColumn; 031import jakarta.persistence.ManyToOne; 032import jakarta.persistence.SequenceGenerator; 033import jakarta.persistence.Table; 034import jakarta.persistence.UniqueConstraint; 035 036import java.io.Serializable; 037 038@Embeddable 039@Entity 040@Table( 041 name = "HFJ_HISTORY_TAG", 042 uniqueConstraints = { 043 @UniqueConstraint( 044 name = "IDX_RESHISTTAG_TAGID", 045 columnNames = {"RES_VER_PID", "TAG_ID"}), 046 }, 047 indexes = {@Index(name = "IDX_RESHISTTAG_RESID", columnList = "RES_ID")}) 048public class ResourceHistoryTag extends BaseTag implements Serializable { 049 050 private static final long serialVersionUID = 1L; 051 052 @SequenceGenerator(name = "SEQ_HISTORYTAG_ID", sequenceName = "SEQ_HISTORYTAG_ID") 053 @GeneratedValue(strategy = GenerationType.AUTO, generator = "SEQ_HISTORYTAG_ID") 054 @Id 055 @Column(name = "PID") 056 private Long myId; 057 058 @ManyToOne() 059 @JoinColumn( 060 name = "RES_VER_PID", 061 referencedColumnName = "PID", 062 nullable = false, 063 foreignKey = @ForeignKey(name = "FK_HISTORYTAG_HISTORY")) 064 private ResourceHistoryTable myResourceHistory; 065 066 @Column(name = "RES_VER_PID", insertable = false, updatable = false, nullable = false) 067 private Long myResourceHistoryPid; 068 069 @Column(name = "RES_TYPE", length = ResourceTable.RESTYPE_LEN, nullable = false) 070 private String myResourceType; 071 072 @Column(name = "RES_ID", nullable = false) 073 private Long myResourceId; 074 075 /** 076 * Constructor 077 */ 078 public ResourceHistoryTag() { 079 super(); 080 } 081 082 /** 083 * Constructor 084 */ 085 public ResourceHistoryTag( 086 ResourceHistoryTable theResourceHistoryTable, 087 TagDefinition theTag, 088 PartitionablePartitionId theRequestPartitionId) { 089 this(); 090 setTag(theTag); 091 setResource(theResourceHistoryTable); 092 setResourceId(theResourceHistoryTable.getResourceId()); 093 setResourceType(theResourceHistoryTable.getResourceType()); 094 setPartitionId(theRequestPartitionId); 095 } 096 097 public String getResourceType() { 098 return myResourceType; 099 } 100 101 public void setResourceType(String theResourceType) { 102 myResourceType = theResourceType; 103 } 104 105 public Long getResourceId() { 106 return myResourceId; 107 } 108 109 public void setResourceId(Long theResourceId) { 110 myResourceId = theResourceId; 111 } 112 113 public ResourceHistoryTable getResourceHistory() { 114 return myResourceHistory; 115 } 116 117 public void setResource(ResourceHistoryTable theResourceHistory) { 118 myResourceHistory = theResourceHistory; 119 } 120 121 public Long getId() { 122 return myId; 123 } 124}