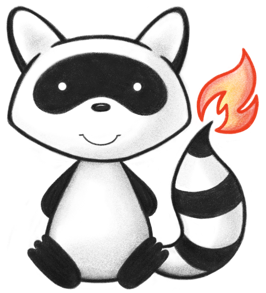
001package ca.uhn.fhir.jpa.model.entity; 002 003/*- 004 * #%L 005 * HAPI FHIR JPA Model 006 * %% 007 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 008 * %% 009 * Licensed under the Apache License, Version 2.0 (the "License"); 010 * you may not use this file except in compliance with the License. 011 * You may obtain a copy of the License at 012 * 013 * http://www.apache.org/licenses/LICENSE-2.0 014 * 015 * Unless required by applicable law or agreed to in writing, software 016 * distributed under the License is distributed on an "AS IS" BASIS, 017 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 018 * See the License for the specific language governing permissions and 019 * limitations under the License. 020 * #L% 021 */ 022 023import jakarta.persistence.Column; 024import jakarta.persistence.Embeddable; 025 026import java.io.Serializable; 027import java.util.Objects; 028 029@Embeddable 030public class PersistedResourceModifiedMessageEntityPK implements IPersistedResourceModifiedMessagePK, Serializable { 031 032 @Column(name = "RES_ID", length = 256, nullable = false) 033 private String myResourcePid; 034 035 @Column(name = "RES_VER", length = 8, nullable = false) 036 private String myResourceVersion; 037 038 public String getResourcePid() { 039 return myResourcePid; 040 } 041 042 public PersistedResourceModifiedMessageEntityPK setResourcePid(String theResourcePid) { 043 myResourcePid = theResourcePid; 044 return this; 045 } 046 047 public String getResourceVersion() { 048 return myResourceVersion; 049 } 050 051 public PersistedResourceModifiedMessageEntityPK setResourceVersion(String theResourceVersion) { 052 myResourceVersion = theResourceVersion; 053 return this; 054 } 055 056 public static PersistedResourceModifiedMessageEntityPK with(String theResourcePid, String theResourceVersion) { 057 return new PersistedResourceModifiedMessageEntityPK() 058 .setResourcePid(theResourcePid) 059 .setResourceVersion(theResourceVersion); 060 } 061 062 @Override 063 public boolean equals(Object theO) { 064 if (this == theO) return true; 065 if (theO == null || getClass() != theO.getClass()) return false; 066 PersistedResourceModifiedMessageEntityPK that = (PersistedResourceModifiedMessageEntityPK) theO; 067 return myResourcePid.equals(that.myResourcePid) && myResourceVersion.equals(that.myResourceVersion); 068 } 069 070 @Override 071 public int hashCode() { 072 return Objects.hash(myResourcePid, myResourceVersion); 073 } 074 075 @Override 076 public String toString() { 077 return myResourcePid + "/" + myResourceVersion; 078 } 079}