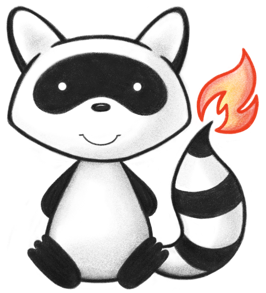
001/*- 002 * #%L 003 * HAPI FHIR JPA Server 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.jpa.term.loinc; 021 022import ca.uhn.fhir.jpa.term.IZipContentsHandlerCsv; 023import jakarta.annotation.Nonnull; 024import org.apache.commons.csv.CSVRecord; 025 026import java.util.List; 027 028import static org.apache.commons.lang3.StringUtils.isBlank; 029import static org.apache.commons.lang3.StringUtils.trim; 030 031public class LoincLinguisticVariantsHandler implements IZipContentsHandlerCsv { 032 033 private final List<LinguisticVariant> myLinguisticVariants; 034 035 public LoincLinguisticVariantsHandler(List<LinguisticVariant> thelinguisticVariants) { 036 myLinguisticVariants = thelinguisticVariants; 037 } 038 039 @Override 040 public void accept(CSVRecord theRecord) { 041 042 String id = trim(theRecord.get("ID")); 043 if (isBlank(id)) { 044 return; 045 } 046 047 String isoLanguage = trim(theRecord.get("ISO_LANGUAGE")); 048 if (isBlank(isoLanguage)) { 049 return; 050 } 051 052 String isoCountry = trim(theRecord.get("ISO_COUNTRY")); 053 if (isBlank(isoCountry)) { 054 return; 055 } 056 057 String languageName = trim(theRecord.get("LANGUAGE_NAME")); 058 if (isBlank(languageName)) { 059 return; 060 } 061 062 LinguisticVariant linguisticVariant = new LinguisticVariant(id, isoLanguage, isoCountry, languageName); 063 myLinguisticVariants.add(linguisticVariant); 064 } 065 066 public static class LinguisticVariant { 067 068 private String myId; 069 private String myIsoLanguage; 070 private String myIsoCountry; 071 private String myLanguageName; 072 073 public LinguisticVariant( 074 @Nonnull String theId, 075 @Nonnull String theIsoLanguage, 076 @Nonnull String theIsoCountry, 077 @Nonnull String theLanguageName) { 078 this.myId = theId; 079 this.myIsoLanguage = theIsoLanguage; 080 this.myIsoCountry = theIsoCountry; 081 this.myLanguageName = theLanguageName; 082 } 083 084 public String getLinguisticVariantFileName() { 085 return myIsoLanguage + myIsoCountry + myId + "LinguisticVariant.csv"; 086 } 087 088 public String getLanguageName() { 089 return myLanguageName; 090 } 091 092 public String getLanguageCode() { 093 return myIsoLanguage + "-" + myIsoCountry; 094 } 095 } 096}