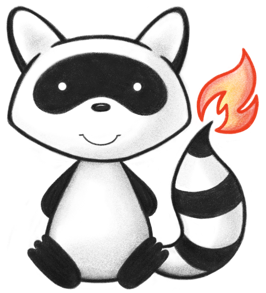
001 002package ca.uhn.fhir.jpa.rp.r5; 003 004import java.util.*; 005 006import org.apache.commons.lang3.StringUtils; 007 008import ca.uhn.fhir.jpa.searchparam.SearchParameterMap; 009import ca.uhn.fhir.model.api.Include; 010import ca.uhn.fhir.model.api.annotation.*; 011import org.hl7.fhir.r5.model.*; 012import ca.uhn.fhir.rest.annotation.*; 013import ca.uhn.fhir.rest.param.*; 014import ca.uhn.fhir.rest.api.SortSpec; 015import ca.uhn.fhir.rest.api.SummaryEnum; 016import ca.uhn.fhir.rest.api.SearchTotalModeEnum; 017import ca.uhn.fhir.rest.api.SearchContainedModeEnum; 018 019public class LocationResourceProvider extends 020 ca.uhn.fhir.jpa.provider.BaseJpaResourceProvider<Location> 021 { 022 023 @Override 024 public Class<Location> getResourceType() { 025 return Location.class; 026 } 027 028 @Search(allowUnknownParams=true) 029 public ca.uhn.fhir.rest.api.server.IBundleProvider search( 030 jakarta.servlet.http.HttpServletRequest theServletRequest, 031 jakarta.servlet.http.HttpServletResponse theServletResponse, 032 033 ca.uhn.fhir.rest.api.server.RequestDetails theRequestDetails, 034 035 @Description(shortDefinition="Search the contents of the resource's data using a filter") 036 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_FILTER) 037 StringAndListParam theFtFilter, 038 039 @Description(shortDefinition="Search the contents of the resource's data using a fulltext search") 040 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_CONTENT) 041 StringAndListParam theFtContent, 042 043 @Description(shortDefinition="Search the contents of the resource's narrative using a fulltext search") 044 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_TEXT) 045 StringAndListParam theFtText, 046 047 @Description(shortDefinition="Search for resources which have the given tag") 048 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_TAG) 049 TokenAndListParam theSearchForTag, 050 051 @Description(shortDefinition="Search for resources which have the given security labels") 052 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_SECURITY) 053 TokenAndListParam theSearchForSecurity, 054 055 @Description(shortDefinition="Search for resources which have the given profile") 056 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_PROFILE) 057 UriAndListParam theSearchForProfile, 058 059 @Description(shortDefinition="Search the contents of the resource's data using a list") 060 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_LIST) 061 StringAndListParam theList, 062 063 @Description(shortDefinition="The language of the resource") 064 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_LANGUAGE) 065 TokenAndListParam theResourceLanguage, 066 067 @Description(shortDefinition="Search for resources which have the given source value (Resource.meta.source)") 068 @OptionalParam(name=ca.uhn.fhir.rest.api.Constants.PARAM_SOURCE) 069 UriAndListParam theSearchForSource, 070 071 @Description(shortDefinition="Return resources linked to by the given target") 072 @OptionalParam(name="_has") 073 HasAndListParam theHas, 074 075 076 077 @Description(shortDefinition="The ID of the resource") 078 @OptionalParam(name="_id") 079 TokenAndListParam the_id, 080 081 082 @Description(shortDefinition="Search on the narrative of the resource") 083 @OptionalParam(name="_text") 084 SpecialAndListParam the_text, 085 086 087 @Description(shortDefinition="A (part of the) address of the location") 088 @OptionalParam(name="address") 089 StringAndListParam theAddress, 090 091 092 @Description(shortDefinition="A city specified in an address") 093 @OptionalParam(name="address-city") 094 StringAndListParam theAddress_city, 095 096 097 @Description(shortDefinition="A country specified in an address") 098 @OptionalParam(name="address-country") 099 StringAndListParam theAddress_country, 100 101 102 @Description(shortDefinition="A postal code specified in an address") 103 @OptionalParam(name="address-postalcode") 104 StringAndListParam theAddress_postalcode, 105 106 107 @Description(shortDefinition="A state specified in an address") 108 @OptionalParam(name="address-state") 109 StringAndListParam theAddress_state, 110 111 112 @Description(shortDefinition="A use code specified in an address") 113 @OptionalParam(name="address-use") 114 TokenAndListParam theAddress_use, 115 116 117 @Description(shortDefinition="One of the Location's characteristics") 118 @OptionalParam(name="characteristic") 119 TokenAndListParam theCharacteristic, 120 121 122 @Description(shortDefinition="Select locations that contain the specified co-ordinates") 123 @OptionalParam(name="contains") 124 SpecialAndListParam theContains, 125 126 127 @Description(shortDefinition="Technical endpoints providing access to services operated for the location") 128 @OptionalParam(name="endpoint", targetTypes={ } ) 129 ReferenceAndListParam theEndpoint, 130 131 132 @Description(shortDefinition="An identifier for the location") 133 @OptionalParam(name="identifier") 134 TokenAndListParam theIdentifier, 135 136 137 @Description(shortDefinition="A portion of the location's name or alias") 138 @OptionalParam(name="name") 139 StringAndListParam theName, 140 141 142 @Description(shortDefinition="Search for locations where the location.position is near to, or within a specified distance of, the provided coordinates expressed as [latitude]|[longitude]|[distance]|[units] (using the WGS84 datum, see notes).Servers which support the near parameter SHALL support the unit string 'km' for kilometers and SHOULD support '[mi_us]' for miles, support for other units is optional. If the units are omitted, then kms should be assumed. If the distance is omitted, then the server can use its own discretion as to what distances should be considered near (and units are irrelevant).If the server is unable to understand the units (and does support the near search parameter), it MIGHT return an OperationOutcome and fail the search with a http status 400 BadRequest. If the server does not support the near parameter, the parameter MIGHT report the unused parameter in a bundled OperationOutcome and still perform the search ignoring the near parameter.Note: The algorithm to determine the distance is not defined by the specification, and systems might have different engines that calculate things differently. They could consider geographic point to point, or path via road, or including current traffic conditions, or just simple neighboring postcodes/localities if that's all it had access to.") 143 @OptionalParam(name="near") 144 SpecialAndListParam theNear, 145 146 147 @Description(shortDefinition="Searches for locations (typically bed/room) that have an operational status (e.g. contaminated, housekeeping)") 148 @OptionalParam(name="operational-status") 149 TokenAndListParam theOperational_status, 150 151 152 @Description(shortDefinition="Searches for locations that are managed by the provided organization") 153 @OptionalParam(name="organization", targetTypes={ } ) 154 ReferenceAndListParam theOrganization, 155 156 157 @Description(shortDefinition="A location of which this location is a part") 158 @OptionalParam(name="partof", targetTypes={ } ) 159 ReferenceAndListParam thePartof, 160 161 162 @Description(shortDefinition="Searches for locations with a specific kind of status") 163 @OptionalParam(name="status") 164 TokenAndListParam theStatus, 165 166 167 @Description(shortDefinition="A code for the type of location") 168 @OptionalParam(name="type") 169 TokenAndListParam theType, 170 171 @RawParam 172 Map<String, List<String>> theAdditionalRawParams, 173 174 @Description(shortDefinition="Only return resources which were last updated as specified by the given range") 175 @OptionalParam(name="_lastUpdated") 176 DateRangeParam theLastUpdated, 177 178 @IncludeParam 179 Set<Include> theIncludes, 180 181 @IncludeParam(reverse=true) 182 Set<Include> theRevIncludes, 183 184 @Sort 185 SortSpec theSort, 186 187 @ca.uhn.fhir.rest.annotation.Count 188 Integer theCount, 189 190 @ca.uhn.fhir.rest.annotation.Offset 191 Integer theOffset, 192 193 SummaryEnum theSummaryMode, 194 195 SearchTotalModeEnum theSearchTotalMode, 196 197 SearchContainedModeEnum theSearchContainedMode 198 199 ) { 200 startRequest(theServletRequest); 201 try { 202 SearchParameterMap paramMap = new SearchParameterMap(); 203 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_FILTER, theFtFilter); 204 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_CONTENT, theFtContent); 205 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_TEXT, theFtText); 206 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_TAG, theSearchForTag); 207 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_SECURITY, theSearchForSecurity); 208 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_PROFILE, theSearchForProfile); 209 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_SOURCE, theSearchForSource); 210 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_LIST, theList); 211 paramMap.add(ca.uhn.fhir.rest.api.Constants.PARAM_LANGUAGE, theResourceLanguage); 212 213 paramMap.add("_has", theHas); 214 paramMap.add("_id", the_id); 215 paramMap.add("_text", the_text); 216 paramMap.add("address", theAddress); 217 paramMap.add("address-city", theAddress_city); 218 paramMap.add("address-country", theAddress_country); 219 paramMap.add("address-postalcode", theAddress_postalcode); 220 paramMap.add("address-state", theAddress_state); 221 paramMap.add("address-use", theAddress_use); 222 paramMap.add("characteristic", theCharacteristic); 223 paramMap.add("contains", theContains); 224 paramMap.add("endpoint", theEndpoint); 225 paramMap.add("identifier", theIdentifier); 226 paramMap.add("name", theName); 227 paramMap.add("near", theNear); 228 paramMap.add("operational-status", theOperational_status); 229 paramMap.add("organization", theOrganization); 230 paramMap.add("partof", thePartof); 231 paramMap.add("status", theStatus); 232 paramMap.add("type", theType); 233 paramMap.setRevIncludes(theRevIncludes); 234 paramMap.setLastUpdated(theLastUpdated); 235 paramMap.setIncludes(theIncludes); 236 paramMap.setSort(theSort); 237 paramMap.setCount(theCount); 238 paramMap.setOffset(theOffset); 239 paramMap.setSummaryMode(theSummaryMode); 240 paramMap.setSearchTotalMode(theSearchTotalMode); 241 paramMap.setSearchContainedMode(theSearchContainedMode); 242 243 getDao().translateRawParameters(theAdditionalRawParams, paramMap); 244 245 ca.uhn.fhir.rest.api.server.IBundleProvider retVal = getDao().search(paramMap, theRequestDetails, theServletResponse); 246 return retVal; 247 } finally { 248 endRequest(theServletRequest); 249 } 250 } 251 252}