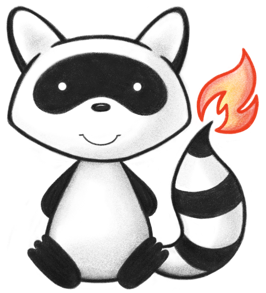
001package org.hl7.fhir.convertors.misc; 002 003import java.io.FileInputStream; 004import java.io.FileOutputStream; 005 006import org.hl7.fhir.r4.formats.IParser.OutputStyle; 007import org.hl7.fhir.r4.model.BooleanType; 008import org.hl7.fhir.r4.model.CodeSystem; 009import org.hl7.fhir.r4.model.CodeSystem.ConceptDefinitionComponent; 010import org.hl7.fhir.r4.model.CodeSystem.PropertyType; 011import org.hl7.fhir.r4.model.StringType; 012import org.hl7.fhir.r4.terminologies.CodeSystemUtilities; 013import org.hl7.fhir.utilities.CommaSeparatedStringBuilder; 014import org.hl7.fhir.utilities.TextFile; 015import org.hl7.fhir.utilities.Utilities; 016import org.hl7.fhir.utilities.json.model.JsonObject; 017import org.hl7.fhir.utilities.json.parser.JsonParser; 018 019public class SPDXImporter { 020 021 private StringBuilder b; 022 023 024 public static void main(String[] args) throws Exception { 025 new SPDXImporter().generate(args); 026 } 027 028 public void generate(String[] args) throws Exception { 029 JsonObject json = JsonParser.parseObjectFromUrl("https://raw.githubusercontent.com/spdx/license-list-data/main/json/licenses.json"); 030 CodeSystem cs = (CodeSystem) new org.hl7.fhir.r4.formats.JsonParser().parse(new FileInputStream(args[0])); 031 cs.getConcept().clear(); 032 cs.getProperty().clear(); 033 cs.addProperty().setCode("reference").setType(PropertyType.STRING); 034 cs.addProperty().setCode("isOsiApproved").setType(PropertyType.BOOLEAN); 035 cs.addProperty().setCode("status").setType(PropertyType.CODE).setUri("http://hl7.org/fhir/concept-properties#status"); 036 cs.addProperty().setCode("seeAlso").setType(PropertyType.STRING); 037 cs.setVersion(json.asString("licenseListVersion")); 038 ConceptDefinitionComponent cc = cs.addConcept(); 039 cc.setCode("not-open-source"); 040 cc.setDisplay("Not open source"); 041 cc.setDefinition("Not an open source license."); 042 for (JsonObject l : json.getJsonObjects("licenses")) { 043 cc = cs.addConcept(); 044 cc.setCode(l.asString("licenseId")); 045 cc.setDisplay(l.asString("name")); 046 cc.setDefinition(l.asString("name")); 047 if (l.has("reference")) { 048 cc.addProperty().setCode("reference").setValue(new StringType(l.asString("reference"))); 049 } 050 if (l.has("isOsiApproved")) { 051 cc.addProperty().setCode("isOsiApproved").setValue(new BooleanType(l.asBoolean("isOsiApproved"))); 052 } 053 if (l.asBoolean("isDeprecatedLicenseId")) { 054 cc.addProperty().setCode("status").setValue(new BooleanType(l.asBoolean("deprecated"))); 055 } 056 for (String s : l.getStrings("seeAlso")) { 057 cc.addProperty().setCode("seeAlso").setValue(new StringType(s)); 058 } 059 } 060 CodeSystemUtilities.sortAllCodes(cs); 061 new org.hl7.fhir.r4.formats.JsonParser().setOutputStyle(OutputStyle.PRETTY).compose(new FileOutputStream(args[1]), cs); 062 b = new StringBuilder(); 063 generateEnum("SPDXLicense", cs); 064 TextFile.stringToFile(b.toString(), Utilities.changeFileExt(args[1], ".java")); 065 } 066 067 private void write(String s) { 068 b.append(s); 069 } 070 071 private boolean allPlusMinus(String cc) { 072 for (char c : cc.toCharArray()) 073 if (!(c == '-' || c == '+')) 074 return false; 075 return true; 076 } 077 078 protected String makeConst(String cc) { 079 if (cc.equals("*")) 080 cc = "ASTERISK"; 081 if (Utilities.isOid(cc) && Utilities.charCount(cc, '.') > 2) 082 cc = "OID_"+cc; 083 if (cc.equals("%")) 084 cc = "pct"; 085 else if (cc.equals("<")) 086 cc = "less_Than"; 087 else if (cc.equals("<=")) 088 cc = "less_Or_Equal"; 089 else if (cc.equals(">")) 090 cc = "greater_Than"; 091 else if (cc.equals(">=")) 092 cc = "greater_Or_Equal"; 093 else if (cc.equals("=")) 094 cc = "equal"; 095 else if (cc.equals("!=")) 096 cc = "not_equal"; 097 else if (allPlusMinus(cc)) 098 cc = cc.replace("-", "Minus").replace("+", "Plus"); 099 else 100 cc = cc.replace("-", "_").replace("+", "PLUS"); 101 cc = cc.replace("(", "_").replace(")", "_"); 102 cc = cc.replace("{", "_").replace("}", "_"); 103 cc = cc.replace("<", "_").replace(">", "_"); 104 cc = cc.replace(".", "_").replace("/", "_"); 105 cc = cc.replace(":", "_"); 106 cc = cc.replace("%", "pct"); 107 if (Utilities.isInteger(cc.substring(0, 1))) 108 cc = "_"+cc; 109 cc = cc.toUpperCase(); 110 return cc; 111 } 112 113 114 private void generateEnum(String name, CodeSystem cs) throws Exception { 115 String url = cs.getUrl(); 116 CommaSeparatedStringBuilder el = new CommaSeparatedStringBuilder(); 117 118 write(" public enum "+name+" {\r\n"); 119 int l = cs.getConcept().size(); 120 int i = 0; 121 for (ConceptDefinitionComponent c : cs.getConcept()) { 122 i++; 123 String cc = Utilities.camelCase(c.getCode()); 124 cc = makeConst(cc); 125 el.append(cc); 126 127 write(" /**\r\n"); 128 write(" * "+c.getDefinition()+"\r\n"); 129 write(" */\r\n"); 130 write(" "+cc.toUpperCase()+", \r\n"); 131 } 132 write(" /**\r\n"); 133 write(" * added to help the parsers\r\n"); 134 write(" */\r\n"); 135 write(" NULL;\r\n"); 136 el.append("NULL"); 137 138 139 write(" public static "+name+" fromCode(String codeString) throws FHIRException {\r\n"); 140 write(" if (codeString == null || \"\".equals(codeString))\r\n"); 141 write(" return null;\r\n"); 142 for (ConceptDefinitionComponent c : cs.getConcept()) { 143 String cc = Utilities.camelCase(c.getCode()); 144 cc = makeConst(cc); 145 write(" if (\""+c.getCode()+"\".equals(codeString))\r\n"); 146 write(" return "+cc+";\r\n"); 147 } 148 write(" throw new FHIRException(\"Unknown "+name+" code '\"+codeString+\"'\");\r\n"); 149 write(" }\r\n"); 150 151 write(" public static boolean isValidCode(String codeString) {\r\n"); 152 write(" if (codeString == null || \"\".equals(codeString))\r\n"); 153 write(" return false;\r\n"); 154 write(" return Utilities.existsInList(codeString"); 155 for (ConceptDefinitionComponent c : cs.getConcept()) { 156 write(", \""+c.getCode()+"\""); 157 } 158 write(");\r\n"); 159 write(" }\r\n"); 160 161 write(" public String toCode() {\r\n"); 162 write(" switch (this) {\r\n"); 163 for (ConceptDefinitionComponent c : cs.getConcept()) { 164 String cc = Utilities.camelCase(c.getCode()); 165 cc = makeConst(cc); 166 write(" case "+cc+": return \""+c.getCode()+"\";\r\n"); 167 } 168 write(" case NULL: return null;\r\n"); 169 write(" default: return \"?\";\r\n"); 170 write(" }\r\n"); 171 write(" }\r\n"); 172 173 write(" public String getSystem() {\r\n"); 174 write(" switch (this) {\r\n"); 175 for (ConceptDefinitionComponent c : cs.getConcept()) { 176 String cc = Utilities.camelCase(c.getCode()); 177 cc = makeConst(cc); 178 write(" case "+cc+": return \""+cs.getUrl()+"\";\r\n"); 179 } 180 write(" case NULL: return null;\r\n"); 181 write(" default: return \"?\";\r\n"); 182 write(" }\r\n"); 183 write(" }\r\n"); 184 185 write(" public String getDefinition() {\r\n"); 186 write(" switch (this) {\r\n"); 187 for (ConceptDefinitionComponent c : cs.getConcept()) { 188 String cc = Utilities.camelCase(c.getCode()); 189 cc = makeConst(cc); 190 write(" case "+cc+": return \""+Utilities.escapeJava(c.getDefinition())+"\";\r\n"); 191 } 192 write(" case NULL: return null;\r\n"); 193 write(" default: return \"?\";\r\n"); 194 write(" }\r\n"); 195 write(" }\r\n"); 196 197 write(" public String getDisplay() {\r\n"); 198 write(" switch (this) {\r\n"); 199 for (ConceptDefinitionComponent c : cs.getConcept()) { 200 String cc = Utilities.camelCase(c.getCode()); 201 cc = makeConst(cc); 202 write(" case "+cc+": return \""+Utilities.escapeJava(Utilities.noString(c.getDisplay()) ? c.getCode() : c.getDisplay())+"\";\r\n"); 203 } 204 write(" case NULL: return null;\r\n"); 205 write(" default: return \"?\";\r\n"); 206 write(" }\r\n"); 207 write(" }\r\n"); 208 209 write(" }\r\n"); 210 write("\r\n"); 211 212 213 write(" public static class "+name+"EnumFactory implements EnumFactory<"+name+"> {\r\n"); 214 write(" public "+name+" fromCode(String codeString) throws IllegalArgumentException {\r\n"); 215 216 write(" if (codeString == null || \"\".equals(codeString))\r\n"); 217 write(" if (codeString == null || \"\".equals(codeString))\r\n"); 218 write(" return null;\r\n"); 219 for (ConceptDefinitionComponent c : cs.getConcept()) { 220 String cc = Utilities.camelCase(c.getCode()); 221 cc = makeConst(cc); 222 write(" if (\""+c.getCode()+"\".equals(codeString))\r\n"); 223 write(" return "+name+"."+cc+";\r\n"); 224 } 225 write(" throw new IllegalArgumentException(\"Unknown "+name+" code '\"+codeString+\"'\");\r\n"); 226 write(" }\r\n"); 227 write("\r\n"); 228 write(" public Enumeration<"+name+"> fromType(PrimitiveType<?> code) throws FHIRException {\r\n"); 229 write(" if (code == null)\r\n"); 230 write(" return null;\r\n"); 231 write(" if (code.isEmpty())\r\n"); 232 write(" return new Enumeration<"+name+">(this, "+name+".NULL, code);\r\n"); 233 write(" String codeString = ((PrimitiveType) code).asStringValue();\r\n"); 234 write(" if (codeString == null || \"\".equals(codeString))\r\n"); 235 write(" return new Enumeration<"+name+">(this, "+name+".NULL, code);\r\n"); 236 for (ConceptDefinitionComponent c : cs.getConcept()) { 237 String cc = Utilities.camelCase(c.getCode()); 238 cc = makeConst(cc); 239 write(" if (\""+c.getCode()+"\".equals(codeString))\r\n"); 240 write(" return new Enumeration<"+name+">(this, "+name+"."+cc+", code);\r\n"); 241 } 242 write(" throw new FHIRException(\"Unknown "+name+" code '\"+codeString+\"'\");\r\n"); 243 write(" }\r\n"); 244 write(" public String toCode("+name+" code) {\r\n"); 245 for (ConceptDefinitionComponent c : cs.getConcept()) { 246 String cc = Utilities.camelCase(c.getCode()); 247 cc = makeConst(cc); 248 write(" if (code == "+name+"."+cc+")\r\n return \""+c.getCode()+"\";\r\n"); 249 } 250 write(" return \"?\";\r\n"); 251 write(" }\r\n"); 252 253 write(" public String toSystem("+name+" code) {\r\n"); 254 write(" return code.getSystem();\r\n"); 255 write(" }\r\n"); 256 257 write(" }\r\n"); 258 write("\r\n"); 259 } 260 261} 262