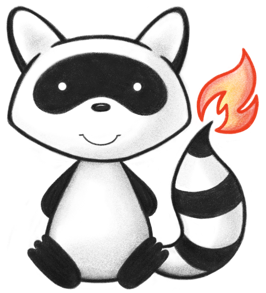
001package org.hl7.fhir.convertors.misc; 002 003import java.io.FileNotFoundException; 004import java.io.FileOutputStream; 005import java.io.IOException; 006 007import org.hl7.fhir.r5.formats.JsonParser; 008import org.hl7.fhir.r5.model.Resource; 009import org.hl7.fhir.r5.utils.ResourceMinifier; 010import org.hl7.fhir.utilities.npm.FilesystemPackageCacheManager; 011import org.hl7.fhir.utilities.npm.NpmPackage; 012import org.hl7.fhir.utilities.npm.NpmPackage.PackageResourceInformation; 013 014public class PackageMinifier { 015 016 public static void main(String[] args) throws IOException { 017 new PackageMinifier().process(args[0], args[1]); 018 } 019 020 private void process(String source, String target) throws FileNotFoundException, IOException { 021 FilesystemPackageCacheManager pcm = new FilesystemPackageCacheManager(true); 022 NpmPackage src = pcm.loadPackage(source); 023 NpmPackage tgt = NpmPackage.empty(); 024 tgt.setNpm(src.getNpm().deepCopy()); 025 tgt.getNpm().set("name", tgt.getNpm().asString("name")+".min"); 026 027 ResourceMinifier min = new ResourceMinifier(); 028 for (PackageResourceInformation pri : src.listIndexedResources()) { 029 if (min.isMinified(pri.getResourceType())) { 030 Resource res = new JsonParser().parse(src.load(pri)); 031 if (min.minify(res)) { 032 tgt.addFile("package", res.fhirType()+"-"+res.getIdPart()+".json", new JsonParser().composeBytes(res), null); 033 } 034 } 035 } 036 tgt.save(new FileOutputStream(target)); 037 } 038 039}